


Detailed explanation of 4 types of type identification methods in JavaScript_javascript skills
具体内容如下:
1.typeof
【输出】首字母小写的字符串形式
【功能】
[a]可以识别标准类型(将Null识别为object)
[b]不能识别具体的对象类型(Function除外)
【实例】
console.log(typeof "jerry");//"string" console.log(typeof 12);//"number" console.log(typeof true);//"boolean" console.log(typeof undefined);//"undefined" console.log(typeof null);//"object" console.log(typeof {name: "jerry"});//"object" console.log(typeof function(){});//"function" console.log(typeof []);//"object" console.log(typeof new Date);//"object" console.log(typeof /\d/);//"object" function Person(){}; console.log(typeof new Person);//"object"
2.Object.prototype.toString
【输出】[object 数据类型]的字符串形式
【功能】
[a]可以识别标准类型及内置对象类型
[b]不能识别自定义类型
【构造方法】
function type(obj){ return Object.prototype.toString.call(obj).slice(8,-1).toLowerCase(); }
【实例1】
console.log(Object.prototype.toString.call("jerry"));//[object String] console.log(Object.prototype.toString.call(12));//[object Number] console.log(Object.prototype.toString.call(true));//[object Boolean] console.log(Object.prototype.toString.call(undefined));//[object Undefined] console.log(Object.prototype.toString.call(null));//[object Null] console.log(Object.prototype.toString.call({name: "jerry"}));//[object Object] console.log(Object.prototype.toString.call(function(){}));//[object Function] console.log(Object.prototype.toString.call([]));//[object Array] console.log(Object.prototype.toString.call(new Date));//[object Date] console.log(Object.prototype.toString.call(/\d/));//[object RegExp] function Person(){}; console.log(Object.prototype.toString.call(new Person));//[object Object]
【实例2】
function type(obj){ return Object.prototype.toString.call(obj).slice(8,-1).toLowerCase(); } console.log(type("jerry"));//"string" console.log(type(12));//"number" console.log(type(true));//"boolean" console.log(type(undefined));//"undefined" console.log(type(null));//"null" console.log(type({name: "jerry"}));//"object" console.log(type(function(){}));//"function" console.log(type([]));//"array" console.log(type(new Date));//"date" console.log(type(/\d/));//"regexp" function Person(){}; console.log(type(new Person));//"object"
3.constructor
【输出】function 数据类型(){[native code]}或者function 自定义类型(){}
【功能】
[a]可以识别标准类型、内置对象类型及自定义类型
[b]不能识别undefined、null,会报错
【构造方法】
function type(obj){ var temp = obj.constructor.toString(); return temp.replace(/^function (\w+)\(\).+$/,'$1'); }
【实例1】
console.log(("jerry").constructor);//function String(){[native code]} console.log((12).constructor);//function Number(){[native code]} console.log((true).constructor);//function Boolean(){[native code]} //console.log((undefined).constructor);//报错 //console.log((null).constructor);//报错 console.log(({name: "jerry"}).constructor);//function Object(){[native code]} console.log((function(){}).constructor);//function Function(){[native code]} console.log(([]).constructor);//function Array(){[native code]} console.log((new Date).constructor);//function Date(){[native code]} console.log((/\d/).constructor);//function RegExp(){[native code]} function Person(){}; console.log((new Person).constructor);//function Person(){}
【实例2】
function type(obj){ var temp = obj.constructor.toString().toLowerCase(); return temp.replace(/^function (\w+)\(\).+$/,'$1'); } console.log(type("jerry"));//"string" console.log(type(12));//"number" console.log(type(true));//"boolean" //console.log(type(undefined));//错误 //console.log(type(null));//错误 console.log(type({name: "jerry"}));//"object" console.log(type(function(){}));//"function" console.log(type([]));//"array" console.log(type(new Date));//"date" console.log(type(/\d/));//"regexp" function Person(){}; console.log(type(new Person));//"person"
4.instanceof
【输出】true或false
【功能】
[a]可以识别内置对象类型、自定义类型及其父类型
[b]不能识别标准类型,会返回false
[c]不能识别undefined、null,会报错
【实例】
console.log("jerry" instanceof String);//false console.log(12 instanceof Number);//false console.log(true instanceof Boolean);//false //console.log(undefined instanceof Undefined);//报错 //console.log(null instanceof Null);//报错 console.log({name: "jerry"} instanceof Object);//true console.log(function(){} instanceof Function);//true console.log([] instanceof Array);//true console.log(new Date instanceof Date);//true console.log(/\d/ instanceof RegExp);//true function Person(){}; console.log(new Person instanceof Person);//true console.log(new Person instanceof Object);//true
以上内容就是详解JavaScript中的4种类型识别方法,希望大家喜欢。
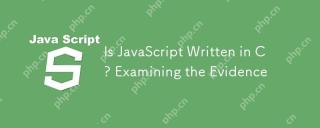
Yes, the engine core of JavaScript is written in C. 1) The C language provides efficient performance and underlying control, which is suitable for the development of JavaScript engine. 2) Taking the V8 engine as an example, its core is written in C, combining the efficiency and object-oriented characteristics of C. 3) The working principle of the JavaScript engine includes parsing, compiling and execution, and the C language plays a key role in these processes.
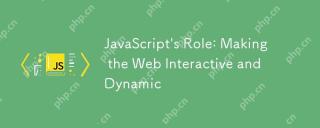
JavaScript is at the heart of modern websites because it enhances the interactivity and dynamicity of web pages. 1) It allows to change content without refreshing the page, 2) manipulate web pages through DOMAPI, 3) support complex interactive effects such as animation and drag-and-drop, 4) optimize performance and best practices to improve user experience.
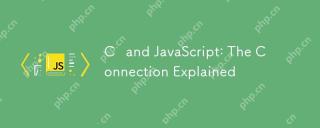
C and JavaScript achieve interoperability through WebAssembly. 1) C code is compiled into WebAssembly module and introduced into JavaScript environment to enhance computing power. 2) In game development, C handles physics engines and graphics rendering, and JavaScript is responsible for game logic and user interface.
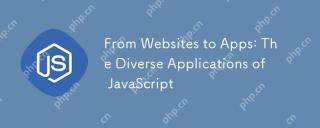
JavaScript is widely used in websites, mobile applications, desktop applications and server-side programming. 1) In website development, JavaScript operates DOM together with HTML and CSS to achieve dynamic effects and supports frameworks such as jQuery and React. 2) Through ReactNative and Ionic, JavaScript is used to develop cross-platform mobile applications. 3) The Electron framework enables JavaScript to build desktop applications. 4) Node.js allows JavaScript to run on the server side and supports high concurrent requests.
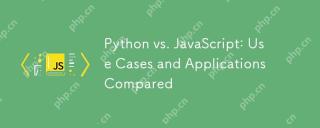
Python is more suitable for data science and automation, while JavaScript is more suitable for front-end and full-stack development. 1. Python performs well in data science and machine learning, using libraries such as NumPy and Pandas for data processing and modeling. 2. Python is concise and efficient in automation and scripting. 3. JavaScript is indispensable in front-end development and is used to build dynamic web pages and single-page applications. 4. JavaScript plays a role in back-end development through Node.js and supports full-stack development.
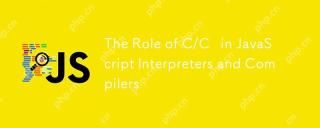
C and C play a vital role in the JavaScript engine, mainly used to implement interpreters and JIT compilers. 1) C is used to parse JavaScript source code and generate an abstract syntax tree. 2) C is responsible for generating and executing bytecode. 3) C implements the JIT compiler, optimizes and compiles hot-spot code at runtime, and significantly improves the execution efficiency of JavaScript.
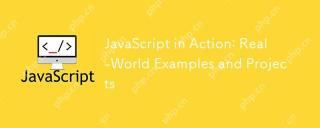
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
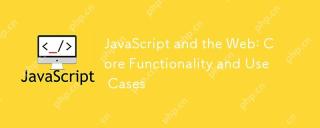
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Linux new version
SublimeText3 Linux latest version

Notepad++7.3.1
Easy-to-use and free code editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
