Use session control to implement page login and logout functions
First is an ordinary login page implementation
Login pagelogin.php
<!DOCTYPE html> <html> <head> <title>登陆页</title> <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.1.3/css/bootstrap.min.css" integrity="sha384-MCw98/SFnGE8fJT3GXwEOngsV7Zt27NXFoaoApmYm81iuXoPkFOJwJ8ERdknLPMO" crossorigin="anonymous"> </head> <body> <div> <div class="card col-12 mt-5"> <div> <h4> 用户登录 </h4> <div class="col-12 mt-4 d-flex justify-content-center"> <form method="post" action="action.php"> <input type="hidden" name="action" value="login"> <div> <label for="username">用户名</label> <input type="text" class="form-control" id="username" name="username" placeholder="请输入用户名"> </div> <div> <label for="password">密码</label> <input type="password" class="form-control" id="password" name="password" placeholder="请输入密码"> </div> <div class="form-group form-check"> <input type="checkbox" class="form-check-input" id="remember" name="remember"> <label for="remember"> 在这台电脑上记住我的登录状态 </label> </div> <button type="submit" class="btn btn-primary"> 登录 </button> </form> </div> </div> </div> </div> </body> </html>
Login function implementationaction.php
##
<?php session_start(); switch ($_REQUEST['action']) { case 'login': $username = $_POST['username']; $password = $_POST['password']; $remember = $_POST['remember']; $user = getUser(); if ($username != $user['username']) { // 登录失败 sendLoginFailedResponse(); } if ($password != $user['password']) { // 登录失败 sendLoginFailedResponse(); } if ($remember) { rememberLogin($username); } $_SESSION['username'] = $username; header("location:index.php"); break; case 'logout': session_unset(); setcookie("username", "", time() - 1); header("location:login.php"); break; } function getUser() { return array( "username" => "cyy", "password" => "123456" ); } function sendLoginFailedResponse() { $response = "<script> alert('用户名或密码错误!'); window.location='login.php'; </script>"; echo $response; die; } function rememberLogin($username) { setcookie("username", $username, time() + 7 * 24 * 3600); }
Homepageindex.php
<?php session_start(); if (rememberedLogin()) { $_SESSION['username'] = $_COOKIE['username']; } if (!hasLoggedIn()) { header("location:login.php"); die; } function hasLoggedIn() { return isset($_SESSION['username']) && validateUsername($_SESSION['username']); } function validateUsername($username) { return $username == "cyy"; } function rememberedLogin() { return isset($_COOKIE['username']) && validateUsername($_COOKIE['username']); } ?> <!DOCTYPE html> <html> <head> <title>主页</title> <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.1.3/css/bootstrap.min.css" integrity="sha384-MCw98/SFnGE8fJT3GXwEOngsV7Zt27NXFoaoApmYm81iuXoPkFOJwJ8ERdknLPMO" crossorigin="anonymous"> </head> <body> <div> <nav class="navbar navbar-light bg-light"> <a> 使用 Cookie 和 Session 实现会话控制 </a> <a href="action.php?action=logout"> <button class="btn btn-outline-danger my-2 my-sm-0" type="button"> 注销 </button> </a> </nav> <div class="d-flex justify-content-around mt-5"> <div class="card col-5"> <div> <h5> 会话控制实战内容一 </h5> <h6 class="card-subtitle mb-2 text-muted"> SESSION 部分 </h6> <p> 实现用户认证功能,用户登录、退出与身份识别 </p> </div> </div> <div class="card col-5"> <div> <h5> 会话控制实战内容二 </h5> <h6 class="card-subtitle mb-2 text-muted"> COOKIE 部分 </h6> <p> 实现登录记住用户功能,七天免登录认证 </p> </div> </div> </div> <div class="d-flex justify-content-around mt-4"> <div class="card col-5"> <div> <h5> 会话控制实战内容一 </h5> <h6 class="card-subtitle mb-2 text-muted"> SESSION 部分 </h6> <p> 实现用户认证功能,用户登录、退出与身份识别 </p> </div> </div> <div class="card col-5"> <div> <h5> 会话控制实战内容二 </h5> <h6 class="card-subtitle mb-2 text-muted"> COOKIE 部分 </h6> <p> 实现登录记住用户功能,七天免登录认证 </p> </div> </div> </div> </div> </body> </html>
Next is the session control example: Wishing Wall source code
index.php
##
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml" xml:lang="en"> <head> <meta http-equiv="Content-Type" content="text/html;charset=UTF-8"> <title>许愿墙</title> <link rel="stylesheet" href="Css/index.css" /> <script type="text/javascript" src='Js/jquery-1.7.2.min.js'></script> <script type="text/javascript" src='Js/index.js'></script> </head> <body> <div id='top'> <a href="wish.php"><span id='send'></span></a> </div> <div id='main'> <?php //连接数据库 $connection=mysqli_connect('127.0.0.1','root','123456'); if(mysqli_connect_error()){ die(mysqli_connect_error()); } mysqli_select_db($connection,'wall'); mysqli_set_charset($connection,'utf8'); $sql="SELECT * FROM wall"; $result=mysqli_query($connection,$sql); //显示留言 while($row=mysqli_fetch_assoc($result)){ $wish_time=$row['wish_time']; $time=date('Y-m-d H:i:s',$wish_time); $id=$row['id']; //判断留言板颜色 switch($row['color']){ case 'a1': echo "<dl class='paper a1'>"; break; case 'a2': echo "<dl class='paper a2'>"; break; case 'a3': echo "<dl class='paper a3'>"; break; case 'a4': echo "<dl class='paper a4'>"; break; case 'a5': echo "<dl class='paper a5'>"; break; default: echo "<dl class='paper a1'>"; break; } echo "<dt>"; echo "<span>{$row['name']}</span>"; echo "<span>No.{$row['id']}</span>"; echo "</dt>"; echo "<dd>{$row['content']}</dd>"; echo "<dd>"; echo "<span>{$time}</span>"; echo "<a href=\"delete.php?num={$id}\"></a>"; echo "</dd>"; echo "</dl>"; } mysqli_close($connection); ?> </div> <!--[if IE 6]> <script type="text/javascript" src="./Js/iepng.js"></script> <script type="text/javascript"> DD_belatedPNG.fix('#send,#close,.close','background'); </script> <![endif]--> </body> </html>
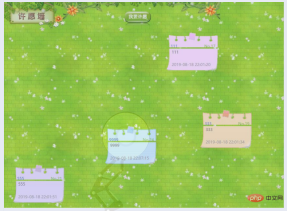
wish.php
<!DOCTYPE > <html xmlns="http://www.w3.org/1999/xhtml" xml:lang="en"> <head> <meta http-equiv="Content-Type" content="text/html;charset=UTF-8"> <title>许愿墙</title> <link rel="stylesheet" href="Css/index.css" /> <script type="text/javascript" src='Js/jquery-1.7.2.min.js'></script> <script type="text/javascript" src='Js/index.js'></script> <style type="text/css"> #content { width: 638px; height:650px; margin:0 auto; margin-top:100px; /*background-color:#F0FAFF; border:2px solid #C9F;*/ } #content .c-top{ width: 638px; height: 80px; background: url(./Images/content_top.jpg) no-repeat; } #content .c-bottom{ width: 638px; height: 50px; background: url(./Images/content_bottom.jpg) no-repeat; } .c-content{ width: 638px; height: 470px; background: url(./Images/content_bg.jpg) repeat; } .papercolor{ width:588px; height: 60px; margin-left: 35px; padding-top:15px; } .p-left{ float: left; width: 120px; line-height: 27px; }p-left .p-right{ float: left; } .color330{ float: left; margin-left: 20px; border-right: #404040 1px solid; border-top: #404040 1px solid; border-left:#404040 1px solid; width: 25px; cursor: pointer; border-bottom: #404040 1px solid; height: 25px; } .papercontent{ width: 588px; height: 210px; margin-left: 35px; } .left{ width: 294px; height:100px; float: left; } .right{ width: 294px; height:100px; float: left; } .left-top{ margin-bottom: 10px; } .left-bottom{ } .right-top{ margin-bottom: 10px; } .right-bottom{ width:200px; height:150px; border: 1px solid orange; margin-left:20px; background-color:#E8DEFF; } .name{ clear: both; width: 588px; height: 50px; margin-left: 35px; margin-top:10px; } .name-left{ width:60px; height: 26px; line-height: 26px; float: left; } .name-right{ float: left; } .name-right input{ width: 200px; height: 26px; } .code{ clear: both; width: 588px; height: 50px; margin-left: 35px; margin-top:10px; } .code-left{ width:50px; height: 26px; line-height: 26px; float: left; } .code-content{ width:100px; float: left; } .code-content input{ width: 100px; height: 26px; } .code-right{ float:left; margin-left: 10px; } .code-right input{ width: 40px; height: 26px; background-color: pink; } .submit{ width:174px; height:38px; background: url(./Images/pic_submit.gif) no-repeat; margin-left:217px; } .shuname{ width:80px; height:25px; margin-left: 120px; } span{ font-size: 13px; font-family: "微软雅黑"; } </style> </head> <body> <div id='top'></div> <div id="content"> <div></div> <form action="add.php" method="post" id="myfrom"> <div> <div> <div> <span>请选择纸条颜色:</span> </div> <div> <div id="a1" style="background:#FFDFFF"></div> <div id="a2" style="background:#C3FEC0"></div> <div id="a3" style="background:#FFE3b8"></div> <div id="a4" style="background:#CEECFF"></div> <div id="a5" style="background:#E8DEFF"></div> <input type="hidden" value="" name="idvalue" id="idvalue"> </div> </div> <div> <div> <div> <span>输入你的祝福纸条内容:</span> </div> <div> <textarea cols="25" rows="8" id="textfont" name="textfont"></textarea> </div> </div> <div> <div> <span>纸条效果预览:</span> </div> <div> <div style="height:15px"><span>第x条</span><br/></div> <div style="height:100px;margin-top:10px"><span id="font"></span></div> <div><span id="name">署名:</span></div> </div> </div> </div> <div> <div> <span>您的署名:</span> </div> <div> <input id="nameright" type="text" name="name" value=""> </div> </div> <div> <div> <span>验证码:</span> </div> <div> <input id="codeone" type="text" name="recode" value=""><span></span> </div> <div> <input id="codetwo" type="text" name="code" value="<?php echo mt_rand(1000,9999); ?>" readonly> </div> </div> <!--<div><button type="submit" style="width:174px;height:38px"></button></div>--> <input style="BORDER-RIGHT: #f33b78 1px outset; BORDER-TOP: #f33b78 1px outset; FONT-WEIGHT: bold; BORDER-LEFT: #f33b78 1px outset; COLOR: #ffffff; BORDER-BOTTOM: #f33b78 1px outset; BACKGROUND-COLOR: #70ae0b;margin-left: 225px" type="submit" value="→ 开始贴许愿小纸条 ←" name="submit" id="submit"> <a href="index.php"><input type="button" name="Submit2" value="返回"></a> </div> </form> <hr/ style="color:orange;width:550"> <div></div> </div> <!--[if IE 6]> <script type="text/javascript" src="./Js/iepng.js"></script> <script type="text/javascript"> DD_belatedPNG.fix('#send,#close,.close','background'); </script> <![endif]--> <script type="text/javascript"> //改变颜色 $(".color330").click(function(){ var value=$(this).css("background-color"); var idvalue=$(this).attr("id"); console.log(idvalue); $("#idvalue").attr("value",idvalue); $(".right-bottom").css("background-color",value); }) //改变值触发的事件 var textfont = document.getElementById('textfont'); var font = document.getElementById('font'); textfont.onchange=function(){ font.innerHTML=textfont.value; } //改变值触发的事件 var nameright = document.getElementById('nameright'); nameright.onchange=function(){ document.getElementById("name").innerText="署名: "+nameright.value; } //在填写完毕验证码之后验证是否一致 var codeone = document.getElementById('codeone'); var codetwo = document.getElementById('codetwo'); //表单时区焦点事件 codeone.onblur=function(){ //验证两次验证码是否一致 if(codeone.value != codetwo.value){ this.nextSibling.innerHTML='验证码不一致!' this.nextSibling.style.color='red'; } } $( '#submit' ).click( function () { window.location.href="add.php"; } ); </script> </body> </html>
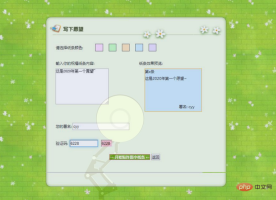
add.php
<?php // 获取表单提交数据 $name=$_POST['name']; $textfont=$_POST['textfont']; $wish_time=time(); $color=$_POST['idvalue']; // 数据库操作 $connection=mysqli_connect('127.0.0.1','root','123456'); if(mysqli_connect_error()){ die(mysqli_connect_error()); } mysqli_select_db($connection,'wall'); mysqli_set_charset($connection,'utf8'); $sql="INSERT INTO wall(content,name,wish_time,color) VALUES('$textfont','$name',$wish_time,'$color')"; $result=mysqli_query($connection,$sql); if($result){ echo '<script>alert("发布成功!");document.location = "index.php";</script>'; }else{ echo '<script>alert("发布失败!");document.location = "index.php";</script>'; } mysqli_close($connection); ?>
Delete wishdelete .php
<?php //接受要删除的留言id $num=$_GET['num']; // 数据库操作 $connection=mysqli_connect('127.0.0.1','root','123456'); if(mysqli_connect_error()){ die(mysqli_connect_error()); } mysqli_select_db($connection,'wall'); mysqli_set_charset($connection,'utf8'); $sql="DELETE FROM wall WHERE id=$num"; $result=mysqli_query($connection,$sql); if($result){ echo '<script>alert("删除成功!");document.location = "index.php";</script>'; }else{ echo '<script>alert("删除失败!");document.location = "index.php";</script>'; } mysqli_close($connection); ?>
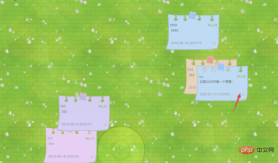
wall.sql
-- phpMyAdmin SQL Dump -- version 4.8.5 -- https://www.phpmyadmin.net/ -- -- 主机: localhost -- 生成日期: 2019-08-18 22:08:38 -- 服务器版本: 8.0.12 -- PHP 版本: 7.3.4 SET SQL_MODE = "NO_AUTO_VALUE_ON_ZERO"; SET AUTOCOMMIT = 0; START TRANSACTION; SET time_zone = "+00:00"; /*!40101 SET @OLD_CHARACTER_SET_CLIENT=@@CHARACTER_SET_CLIENT */; /*!40101 SET @OLD_CHARACTER_SET_RESULTS=@@CHARACTER_SET_RESULTS */; /*!40101 SET @OLD_COLLATION_CONNECTION=@@COLLATION_CONNECTION */; /*!40101 SET NAMES utf8mb4 */; -- -- 数据库: `wall` -- -- -------------------------------------------------------- -- -- 表的结构 `wall` -- CREATE TABLE `wall` ( `id` tinyint(4) NOT NULL COMMENT '留言编号', `content` varchar(200) CHARACTER SET utf8 COLLATE utf8_general_ci NOT NULL COMMENT '留言内容', `name` varchar(20) NOT NULL DEFAULT '匿名的宝宝' COMMENT '署名', `wish_time` int(11) NOT NULL COMMENT '留言时间', `color` char(2) CHARACTER SET utf8 COLLATE utf8_general_ci NOT NULL COMMENT '留言背景色' ) ENGINE=InnoDB DEFAULT CHARSET=utf8; -- -- 转存表中的数据 `wall` -- INSERT INTO `wall` (`id`, `content`, `name`, `wish_time`, `color`) VALUES (17, '111', '111', 1566136880, 'a1'), (19, '333', '333', 1566136894, 'a3'), (21, '555', '555', 1566136911, 'a5'), (24, '9999', '9999', 1566137235, 'a4'); -- -- 转储表的索引 -- -- -- 表的索引 `wall` -- ALTER TABLE `wall` ADD PRIMARY KEY (`id`); -- -- 在导出的表使用AUTO_INCREMENT -- -- -- 使用表AUTO_INCREMENT `wall` -- ALTER TABLE `wall` MODIFY `id` tinyint(4) NOT NULL AUTO_INCREMENT COMMENT '留言编号', AUTO_INCREMENT=26; COMMIT; /*!40101 SET CHARACTER_SET_CLIENT=@OLD_CHARACTER_SET_CLIENT */; /*!40101 SET CHARACTER_SET_RESULTS=@OLD_CHARACTER_SET_RESULTS */; /*!40101 SET COLLATION_CONNECTION=@OLD_COLLATION_CONNECTION */;
Supplementary knowledge points:
[Use COOKIE to achieve session control]
Used to store key user information
Saved on the client (browse Server)
Transmitted through HTTP request/response headers
【COOKIE EXPIRED】
● COOKIE EXPIRED
● User manually deletes COOKIE
● The server clears the validity of COOKIE
[Use SESSION to achieve session control]
● Used to store user-related information
● Saved on the server
● Locate the SESSION content through the SESSION ID saved on the client
【SESSION invalidation/clearance】
● COOKIE expires (close browser)
● User manually deletes COOKIE
● Server deletes SESSION file or clears SESSION content
For more related PHP knowledge, please visit
phptutorialThe above is the detailed content of Use session control to implement page login and logout functions. For more information, please follow other related articles on the PHP Chinese website!
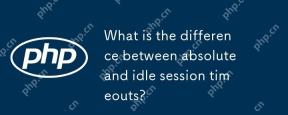
Absolute session timeout starts at the time of session creation, while an idle session timeout starts at the time of user's no operation. Absolute session timeout is suitable for scenarios where strict control of the session life cycle is required, such as financial applications; idle session timeout is suitable for applications that want users to keep their session active for a long time, such as social media.
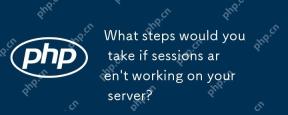
The server session failure can be solved through the following steps: 1. Check the server configuration to ensure that the session is set correctly. 2. Verify client cookies, confirm that the browser supports it and send it correctly. 3. Check session storage services, such as Redis, to ensure that they are running normally. 4. Review the application code to ensure the correct session logic. Through these steps, conversation problems can be effectively diagnosed and repaired and user experience can be improved.
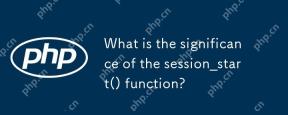
session_start()iscrucialinPHPformanagingusersessions.1)Itinitiatesanewsessionifnoneexists,2)resumesanexistingsession,and3)setsasessioncookieforcontinuityacrossrequests,enablingapplicationslikeuserauthenticationandpersonalizedcontent.
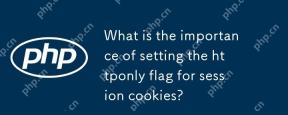
Setting the httponly flag is crucial for session cookies because it can effectively prevent XSS attacks and protect user session information. Specifically, 1) the httponly flag prevents JavaScript from accessing cookies, 2) the flag can be set through setcookies and make_response in PHP and Flask, 3) Although it cannot be prevented from all attacks, it should be part of the overall security policy.
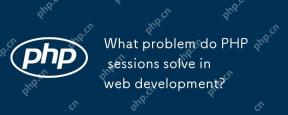
PHPsessionssolvetheproblemofmaintainingstateacrossmultipleHTTPrequestsbystoringdataontheserverandassociatingitwithauniquesessionID.1)Theystoredataserver-side,typicallyinfilesordatabases,anduseasessionIDstoredinacookietoretrievedata.2)Sessionsenhances
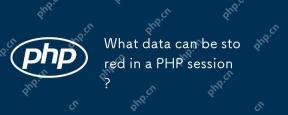
PHPsessionscanstorestrings,numbers,arrays,andobjects.1.Strings:textdatalikeusernames.2.Numbers:integersorfloatsforcounters.3.Arrays:listslikeshoppingcarts.4.Objects:complexstructuresthatareserialized.
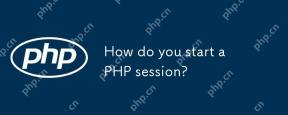
TostartaPHPsession,usesession_start()atthescript'sbeginning.1)Placeitbeforeanyoutputtosetthesessioncookie.2)Usesessionsforuserdatalikeloginstatusorshoppingcarts.3)RegeneratesessionIDstopreventfixationattacks.4)Considerusingadatabaseforsessionstoragei
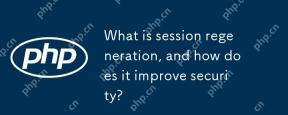
Session regeneration refers to generating a new session ID and invalidating the old ID when the user performs sensitive operations in case of session fixed attacks. The implementation steps include: 1. Detect sensitive operations, 2. Generate new session ID, 3. Destroy old session ID, 4. Update user-side session information.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Zend Studio 13.0.1
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor
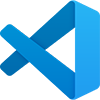
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
