This article learns functional programming from the following aspects:
1. What is the relationship between mathematical formulas and functional programming
Let’s take a simple example. There is a concept in mathematics called mapping (y=f(x)). To put it simply, it is a function. The most familiar one should be the quadratic function (parabola y=a*x*x b*x c)
Now coding implements the value of a certain point on the parabola. We know that a, b, c are parameters, and x is Independent variable, y is the dependent variable. If it were in the past, I might implement it like this (in order to commemorate the c that I haven’t written for a long time, I will write it in c)
double getParabola(double a,double b,double c,double x) { return a*x*x+b*x+c; }
Question 1. Given a parabola, find x =2, x=3, x=4, the value is the following approach
resultA = getParabola(a,b,c,2) resultB = getParabola(a,b,c,2) resultC = getParabola(a,b,c,2)
This is a normal approach in the program. However, from a mathematical perspective, is there any way to become consistent with mathematical formula thinking? The following is another implementation of mine (implemented here using go, because I know how to write c),
func getParabola(aa,bb,cc float32){ var a = aa var b = bb var c = cc a := func(x float32) { return a*x*x+b*x+c } return a }
Then, also for question 1, the solution is as follows
parabola := getParabola(a,b,c) resultA := parabola(2) resultB := parabola(3) resultC := parabola(4)
is Isn't it the same as finding the value of a function? Therefore, mathematical relationships are well represented in functional programming.
2. What are the characteristics of functional programming and what concepts does go support?
Functional programming has three major characteristics
1. Immutability of variables: Once a variable is assigned a value, it cannot be changed. If changes are needed, they must be copied and then modified. In go, once a string variable is assigned a value, it cannot be modified like c, c[2]='a', but it must be explicitly converted to []byte and then modified. But it is already another piece of memory.
2. Functional first-class citizens: Functions are also variables and can be passed as parameters, return values, etc. in the program. This feature should be supported by both c and go.
3. Tail recursion: The concept of recursion was learned in the Fibonacci sequence. If the recursion is very deep, the stack may explode and cause a significant performance degradation. As for tail recursion optimization technology, if the compiler supports it, the stack can be reused in each recursion (tail recursion means that the recursive call occurs in the last step. At this time, the previous results are passed as parameters to the last step of the call, so the previous The state has no effect anymore, so the stack can be reused).
Commonly used techniques in functional programming
1. map&reduce&filter
map is used to call the same function for each input to produce an output, such as for_each in c, map in hadoop, map in python, etc.
reduce is used to add each input to the previous output to get the next output, such as reduce in python and hadoop,
filter is used for filtering, such as c's count_if, etc. .
2. Recursion
3. Pipeline
Put the function instance into an array or list, and then pass the data to the action list, and the input is sequentially passed to each The function operates (meaning that the output of each function is used as the input of another function, the data is flowing, and the calculation is fixed, similar to the concept of storm), and finally we get the result we want.
4. Others (to be further studied)
3. Functional programming and operating efficiency
The most important concept of functional programming is function Equations are first-class citizens, functions and variables are the same. Can be used as parameters, return values, etc. The use of assignment statements is not favored, so recursion is used more often, so the efficiency of functional programming will definitely be lower.
Recently I use closures more. The concept of closure is an environment (one or more variables) plus a function. Every time the closure expression is evaluated, an isolation is obtained. The result is different from an ordinary function. An ordinary function is a piece of executable code. As long as the entrance is determined, the calling position is also determined. For example, in the parabola example above, calling
a:=getParabola(0.2,0.1,0.3) b:=getParabola(0.1,0.1,0.4)
will result in two parabolas. The reason why I think the efficiency will be reduced is because the closure itself is a process of evaluation and assignment, involving the creation and destruction of variables. Of course, I didn't actually test the performance. If subsequent release server efficiency decreases, perhaps this is something to consider.
For more go language knowledge, please pay attention to the go language tutorial column on the PHP Chinese website.
The above is the detailed content of Talking about functional programming from Go language closure. For more information, please follow other related articles on the PHP Chinese website!
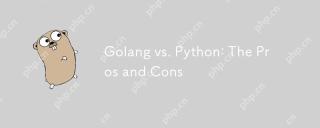
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
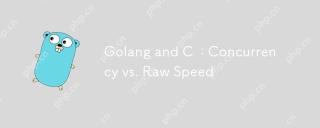
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
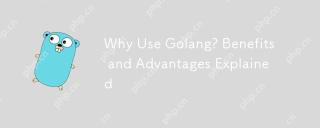
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
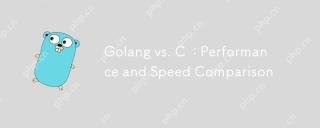
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
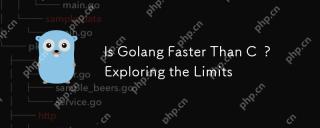
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
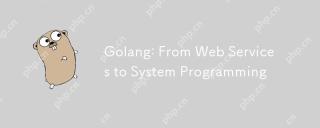
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
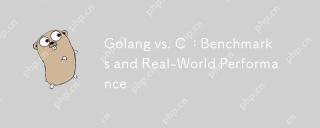
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
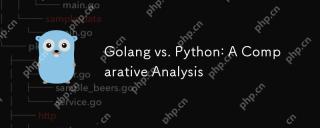
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
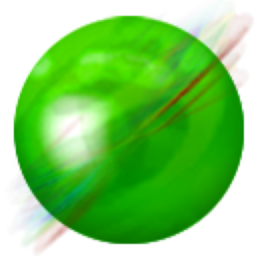
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
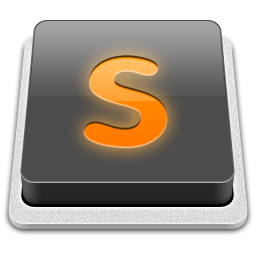
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
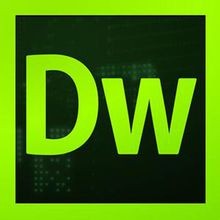
Dreamweaver CS6
Visual web development tools