The timestamp refers to the total number of seconds from Greenwich Mean Time on January 1, 1970 (00:00:00 GMT) to the current time. It is also called Unix Timestamp.
Baidu Encyclopedia defines a timestamp as a piece of complete and verifiable data that can represent that a piece of data existed before a specific time, usually A sequence of characters that uniquely identifies a moment in time.
In python, a timestamp is a string of numbers. When we want to convert it into time data with a certain format, such as '2018-08-08 11:11:11', we need to use to the time module. Likewise, time data can be converted to timestamps.
The specific operations are as follows:
Convert time to timestamp
Reformat time
Convert timestamp to time
Get the current time and convert it to a timestamp (actual requirement)
Convert time to timestamp
Convert the time data 2018-08-08 11:11:11 into a timestamp.
The specific operation process is:
Use the strptime() function to convert the time into a time array! !
Use the mktime() function to convert the time array into a timestamp!!
import time dt = '2018-08-08 11:11:11' # 将时间转换成时间数组 timeArray = time.strptime(dt, "%Y-%m-%d %H:%M:%S") print(timeArray) #time.struct_time(tm_year=2018, tm_mon=8, tm_mday=8, tm_hour=11, tm_min=11,tm_sec=11,tm_wday=2,tm_yday=220,tm_isdst=-1) print(timeArray[0]) # 2018 # 将时间数组转换成时间戳 timestamp = time.mktime(timeArray) print(timestamp) # 1533697871.0
Reformat the time
Reformatting the time requires the following Two steps:
Use the strptime() function to convert the time into a time array
Use the strftime() function to reformat the time
import time dt = '2018-08-08 11:11:11' # 利用strptime()函数将时间转换成时间数组 timeArray = time.strptime(dt, '%Y-%m-%d %H:%M:%S') # 利用strftime()函数重新格式化时间 dt_new = time.strftime('%Y-%m-%d - %H:%M:%S',timeArray) print(dt_new) # 2018-08-08 - 11:11:11
Convert the timestamp Convert to time
In converting timestamp to time, you first need to convert the timestamp into localtime, and then convert it into the specific format of time:
Use the localtime() function to convert the time Convert the stamp to the localtime format
Use the strftime() function to reformat the time
import time timestamp = 1533697871.0 # 利用localtime()函数将时间戳转化成时间数组 localtime = time.localtime(timestamp) print(localtime) #time.struct_time(tm_year=2018, tm_mon=8, tm_mday=8,tm_hour=11,tm_min=11,tm_sec=11, tm_wday=2, tm_yday=220, tm_isdst=0) # 利用strftime()函数重新格式化时间 dt = time.strftime('%Y:%m:%d %H:%M:%S',localtime) print(dt) # 2018:08:08 11:11:11
Get the current time in the specified format
Basic steps:
Use time() to obtain the current time,
then use the localtime() function to convert it to a localtime (local) time array,
finally use the strftime() function to reformat it time.
import time # 获取当前时间 current_time = int(time.time()) print(current_time) # 1537873862 # 转换为localtime localtime = time.localtime(current_time) # 利用strftime()函数重新格式化时间 dt = time.strftime('%Y:%m:%d %H:%M:%S', localtime) print(dt) # 返回当前时间:2019:07:19 4:14:34
The above is the detailed content of What is python timestamp. For more information, please follow other related articles on the PHP Chinese website!
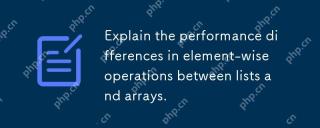
Arraysarebetterforelement-wiseoperationsduetofasteraccessandoptimizedimplementations.1)Arrayshavecontiguousmemoryfordirectaccess,enhancingperformance.2)Listsareflexiblebutslowerduetopotentialdynamicresizing.3)Forlargedatasets,arrays,especiallywithlib
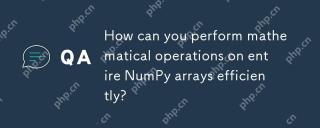
Mathematical operations of the entire array in NumPy can be efficiently implemented through vectorized operations. 1) Use simple operators such as addition (arr 2) to perform operations on arrays. 2) NumPy uses the underlying C language library, which improves the computing speed. 3) You can perform complex operations such as multiplication, division, and exponents. 4) Pay attention to broadcast operations to ensure that the array shape is compatible. 5) Using NumPy functions such as np.sum() can significantly improve performance.
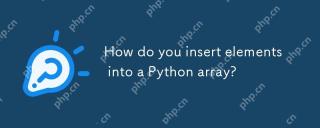
In Python, there are two main methods for inserting elements into a list: 1) Using the insert(index, value) method, you can insert elements at the specified index, but inserting at the beginning of a large list is inefficient; 2) Using the append(value) method, add elements at the end of the list, which is highly efficient. For large lists, it is recommended to use append() or consider using deque or NumPy arrays to optimize performance.
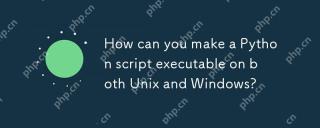
TomakeaPythonscriptexecutableonbothUnixandWindows:1)Addashebangline(#!/usr/bin/envpython3)andusechmod xtomakeitexecutableonUnix.2)OnWindows,ensurePythonisinstalledandassociatedwith.pyfiles,oruseabatchfile(run.bat)torunthescript.
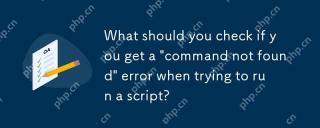
When encountering a "commandnotfound" error, the following points should be checked: 1. Confirm that the script exists and the path is correct; 2. Check file permissions and use chmod to add execution permissions if necessary; 3. Make sure the script interpreter is installed and in PATH; 4. Verify that the shebang line at the beginning of the script is correct. Doing so can effectively solve the script operation problem and ensure the coding process is smooth.
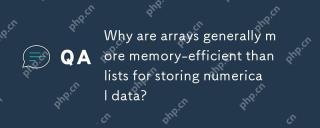
Arraysaregenerallymorememory-efficientthanlistsforstoringnumericaldataduetotheirfixed-sizenatureanddirectmemoryaccess.1)Arraysstoreelementsinacontiguousblock,reducingoverheadfrompointersormetadata.2)Lists,oftenimplementedasdynamicarraysorlinkedstruct
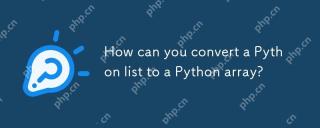
ToconvertaPythonlisttoanarray,usethearraymodule:1)Importthearraymodule,2)Createalist,3)Usearray(typecode,list)toconvertit,specifyingthetypecodelike'i'forintegers.Thisconversionoptimizesmemoryusageforhomogeneousdata,enhancingperformanceinnumericalcomp
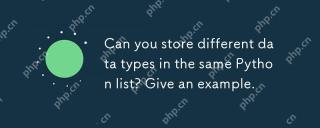
Python lists can store different types of data. The example list contains integers, strings, floating point numbers, booleans, nested lists, and dictionaries. List flexibility is valuable in data processing and prototyping, but it needs to be used with caution to ensure the readability and maintainability of the code.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
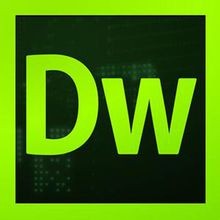
Dreamweaver CS6
Visual web development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
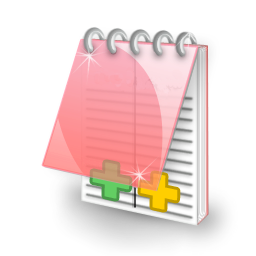
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
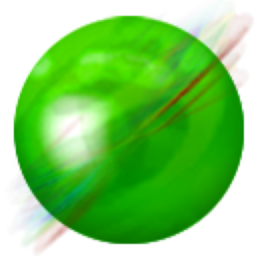
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
