


The content of this article is an introduction to relevant knowledge about python classes (with examples). It has certain reference value. Friends in need can refer to it. I hope it will be helpful to you.
Python-class attributes, instance attributes, class methods, static methods, instance methods
Class attributes and instance attributes
#coding:utf-8 class Student(object): name = 'I am a class variable' #类变量 >>> s = Student() # 创建实例s >>> print(s.name) # 打印name属性,因为实例并没有name属性,所以会继续查找class的name属性 Student >>> print(Student.name) # 打印类的name属性 Student >>> s.name = 'Michael' # 给实例绑定name属性 >>> print(s.name) # 由于实例属性优先级比类属性高,因此,它会屏蔽掉类的name属性 Michael >>> print(Student.name) # 但是类属性并未消失,用Student.name仍然可以访问 Student >>> del s.name # 如果删除实例的name属性 >>> print(s.name) # 再次调用s.name,由于实例的name属性没有找到,类的name属性就显示出来了 Student
Class methods, instances Method, static method
Instance method, the first parameter must be passed by default to the instance object, and it is generally customary to use self.
Static method, no parameters required.
Class method, the first parameter must be passed by default, and cls is generally used.
# coding:utf-8 class Foo(object): """类三种方法语法形式""" def instance_method(self): print("是类{}的实例方法,只能被实例对象调用".format(Foo)) @staticmethod def static_method(): print("是静态方法") @classmethod def class_method(cls): print("是类方法") foo = Foo() foo.instance_method() foo.static_method() foo.class_method() print('----------------') Foo.static_method() Foo.class_method()
Running result:
是类<class>的实例方法,只能被实例对象调用 是静态方法 是类方法 ---------------- 是静态方法 是类方法</class>
Class method
Since there can only be one initialization method in a python class, the class cannot be initialized according to different situations. Class methods are mainly used when a class is used to define multiple constructors.
Special note, static methods can also implement the above functions. When static methods have to write the name of the class every time, it is inconvenient.
# coding:utf-8 class Book(object): def __init__(self, title): self.title = title @classmethod def class_method_create(cls, title): book = cls(title=title) return book @staticmethod def static_method_create(title): book= Book(title) return book book1 = Book("use instance_method_create book instance") book2 = Book.class_method_create("use class_method_create book instance") book3 = Book.static_method_create("use static_method_create book instance") print(book1.title) print(book2.title) print(book3.title)
The above is the detailed content of Introduction to relevant knowledge of python classes (with examples). For more information, please follow other related articles on the PHP Chinese website!
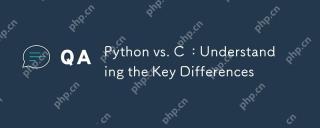
Python and C each have their own advantages, and the choice should be based on project requirements. 1) Python is suitable for rapid development and data processing due to its concise syntax and dynamic typing. 2)C is suitable for high performance and system programming due to its static typing and manual memory management.
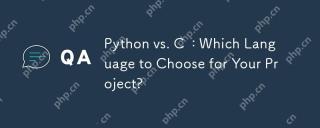
Choosing Python or C depends on project requirements: 1) If you need rapid development, data processing and prototype design, choose Python; 2) If you need high performance, low latency and close hardware control, choose C.
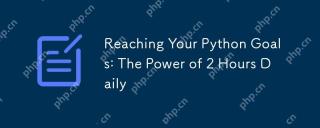
By investing 2 hours of Python learning every day, you can effectively improve your programming skills. 1. Learn new knowledge: read documents or watch tutorials. 2. Practice: Write code and complete exercises. 3. Review: Consolidate the content you have learned. 4. Project practice: Apply what you have learned in actual projects. Such a structured learning plan can help you systematically master Python and achieve career goals.
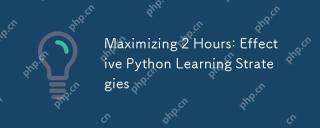
Methods to learn Python efficiently within two hours include: 1. Review the basic knowledge and ensure that you are familiar with Python installation and basic syntax; 2. Understand the core concepts of Python, such as variables, lists, functions, etc.; 3. Master basic and advanced usage by using examples; 4. Learn common errors and debugging techniques; 5. Apply performance optimization and best practices, such as using list comprehensions and following the PEP8 style guide.

Python is suitable for beginners and data science, and C is suitable for system programming and game development. 1. Python is simple and easy to use, suitable for data science and web development. 2.C provides high performance and control, suitable for game development and system programming. The choice should be based on project needs and personal interests.
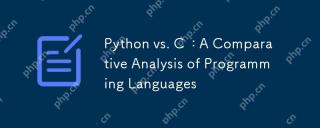
Python is more suitable for data science and rapid development, while C is more suitable for high performance and system programming. 1. Python syntax is concise and easy to learn, suitable for data processing and scientific computing. 2.C has complex syntax but excellent performance and is often used in game development and system programming.
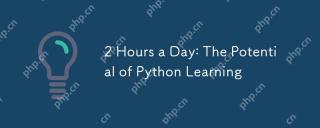
It is feasible to invest two hours a day to learn Python. 1. Learn new knowledge: Learn new concepts in one hour, such as lists and dictionaries. 2. Practice and exercises: Use one hour to perform programming exercises, such as writing small programs. Through reasonable planning and perseverance, you can master the core concepts of Python in a short time.
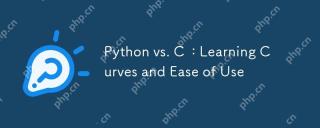
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 English version
Recommended: Win version, supports code prompts!
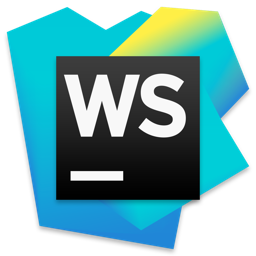
WebStorm Mac version
Useful JavaScript development tools
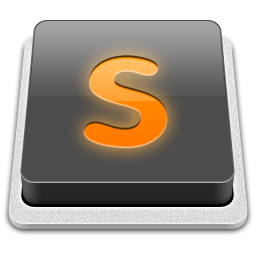
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Linux new version
SublimeText3 Linux latest version