


What are the array data types of numpy in python? (detailed code explanation)
The content of this article is to introduce to you what are the array data types of numpy in python? (Detailed code explanation). It has certain reference value. Friends in need can refer to it. I hope it will be helpful to you.
import numpy as np #创建 # 创建一维数组 a = np.array([1, 2, 3]) print(a) ''' [1 2 3] ''' # 创建多维数组 b = np.array([(1, 2, 3), (4, 5, 6)]) print(b) ''' [[1 2 3] [4 5 6]] ''' # 创建等差一维数组 c = np.arange(1, 5, 0.5) print(c) ''' [1. 1.5 2. 2.5 3. 3.5 4. 4.5] ''' # 创建随机数数组 d = np.random.random((2, 2)) print(d) ''' [[0.65746941 0.09766114] [0.15024283 0.9212932 ]] ''' # 创建一个确定起始点和终止点和个数的等差一维数组 ##包含终止点 e = np.linspace(1, 2, 10) print(e) ''' [1. 1.11111111 1.22222222 1.33333333 1.44444444 1.55555556 1.66666667 1.77777778 1.88888889 2. ] ''' ##不包含终止点 f = np.linspace(1, 2, 10, endpoint=False) print(f) ''' [1. 1.1 1.2 1.3 1.4 1.5 1.6 1.7 1.8 1.9] ''' #创建一个全为‘1’的 数组 g = np.ones([2,3]) print(g) ''' [[1. 1. 1.] [1. 1. 1.]] ''' #创建一个全为‘0’的数组 h = np.zeros([2,3]) print(h) ''' [[0. 0. 0.] [0. 0. 0.]] ''' #通过函数创建数组 k = np.fromfunction(lambda i,j :(i+1)*(j+1),(9,9)) print(k) ''' [[ 1. 2. 3. 4. 5. 6. 7. 8. 9.] [ 2. 4. 6. 8. 10. 12. 14. 16. 18.] [ 3. 6. 9. 12. 15. 18. 21. 24. 27.] [ 4. 8. 12. 16. 20. 24. 28. 32. 36.] [ 5. 10. 15. 20. 25. 30. 35. 40. 45.] [ 6. 12. 18. 24. 30. 36. 42. 48. 54.] [ 7. 14. 21. 28. 35. 42. 49. 56. 63.] [ 8. 16. 24. 32. 40. 48. 56. 64. 72.] [ 9. 18. 27. 36. 45. 54. 63. 72. 81.]] ''' ############## #获取数组的相关属性 a = np.array([(1,2,3),(4,5,6)]) print(a) ##获取数组的形状 print(a.shape) ''' (2, 3) 表示:该数组为2行3列 ''' ## 改变数组的形状 b = a.reshape(3,2) print(b) ''' [[1 2] [3 4] [5 6]] 将a数组的数据由2行3列变成3行2列得到b数组,但是a数组没有发生改变 ''' a.resize(3,2) print(a) ''' [[1 2] [3 4] [5 6]] a数组由2行3列变成3行2列,此时,a数组的形状发生了改变 ''' ############## #数组切片操作 a = np.array([(1,2,3),(4,5,6)]) print(a) ''' [[1 2 3] [4 5 6]] ''' ##获取数组的第二行 print(a[1]) ''' [4 5 6] ''' ##获取数组的前两行 print(a[0:2]) ''' [[1 2 3] [4 5 6]] ''' ##获取数组的前两列的值 print(a[:,[0,1]]) ''' [[1 2] [4 5]] ''' ##获取数组的第1行的前两列的值 print(a[0,[0,1]]) ''' [1 2] ''' ##遍历数组 for row in a: print(row) ''' [1 2 3] [4 5 6] ''' ####################### ##数组拼接 a = np.array([1,2,3]) b = np.array([4,5,6]) #垂直方向的拼接 c = np.vstack((a,b)) print(c) ''' [[1 2 3] [4 5 6]] ''' #竖直方向的拼接 d = np.hstack((a,b)) print(d) ''' [1 2 3 4 5 6] ''' ##################### ##数组的计算 a = np.array([1,2,3]) b = np.array([4,5,6]) #加法 c = a+b print(c) ''' [5 7 9] ''' #减法 d= a - b print(d) ''' [-3 -3 -3] ''' #乘法 e = a * b print(e) ''' [ 4 10 18] ''' #求和 f = np.array([(1,2,3),(4,5,6)]) print(f.sum()) ''' 21 ''' #按列求和 print(f.sum(axis=0)) ''' [5 7 9] ''' #按行求和 print(f.sum(axis=1)) ''' [ 6 15] ''' #最小值的值 print(f.min()) ''' 1 ''' #最小值的索引 print(f.argmin()) ''' 0 ''' #最大值的值 print(f.max()) ''' 6 ''' print(f.argmax()) ''' 5 ''' #平均值 print(f.mean()) ''' 3.5 ''' #方差 print(f.var()) ''' 2.9166666666666665 ''' #标准差 print(f.std()) ''' 1.707825127659933 ''' ############# # 线性代数的运算 #矩阵内积 np.dot() #行列式 np.linalg.det() # 逆矩阵 np.linalg.inv() #多元一次方程组求根 np.linalg.solve() #求特征值和特征向量 np.linalg.eig()
The above is the detailed content of What are the array data types of numpy in python? (detailed code explanation). For more information, please follow other related articles on the PHP Chinese website!
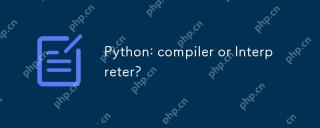
Python is an interpreted language, but it also includes the compilation process. 1) Python code is first compiled into bytecode. 2) Bytecode is interpreted and executed by Python virtual machine. 3) This hybrid mechanism makes Python both flexible and efficient, but not as fast as a fully compiled language.
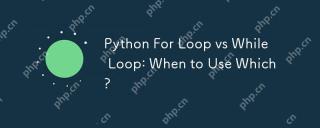
Useaforloopwheniteratingoverasequenceorforaspecificnumberoftimes;useawhileloopwhencontinuinguntilaconditionismet.Forloopsareidealforknownsequences,whilewhileloopssuitsituationswithundeterminediterations.
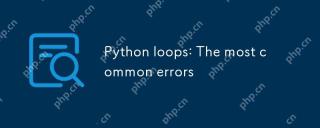
Pythonloopscanleadtoerrorslikeinfiniteloops,modifyinglistsduringiteration,off-by-oneerrors,zero-indexingissues,andnestedloopinefficiencies.Toavoidthese:1)Use'i
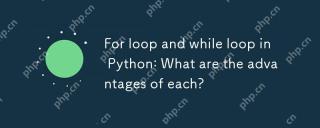
Forloopsareadvantageousforknowniterationsandsequences,offeringsimplicityandreadability;whileloopsareidealfordynamicconditionsandunknowniterations,providingcontrolovertermination.1)Forloopsareperfectforiteratingoverlists,tuples,orstrings,directlyacces
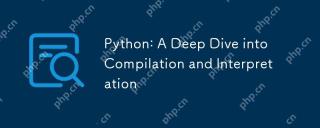
Pythonusesahybridmodelofcompilationandinterpretation:1)ThePythoninterpretercompilessourcecodeintoplatform-independentbytecode.2)ThePythonVirtualMachine(PVM)thenexecutesthisbytecode,balancingeaseofusewithperformance.
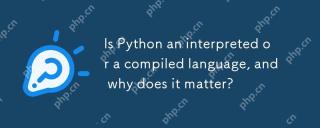
Pythonisbothinterpretedandcompiled.1)It'scompiledtobytecodeforportabilityacrossplatforms.2)Thebytecodeistheninterpreted,allowingfordynamictypingandrapiddevelopment,thoughitmaybeslowerthanfullycompiledlanguages.
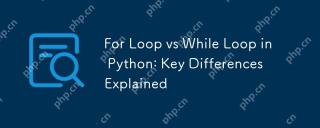
Forloopsareidealwhenyouknowthenumberofiterationsinadvance,whilewhileloopsarebetterforsituationswhereyouneedtoloopuntilaconditionismet.Forloopsaremoreefficientandreadable,suitableforiteratingoversequences,whereaswhileloopsoffermorecontrolandareusefulf
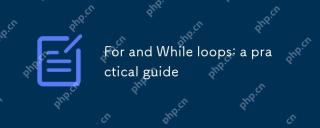
Forloopsareusedwhenthenumberofiterationsisknowninadvance,whilewhileloopsareusedwhentheiterationsdependonacondition.1)Forloopsareidealforiteratingoversequenceslikelistsorarrays.2)Whileloopsaresuitableforscenarioswheretheloopcontinuesuntilaspecificcond


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
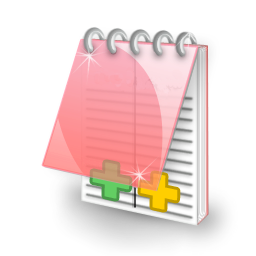
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
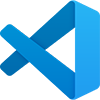
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
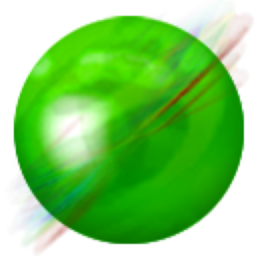
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
