1. Generate digital clock
<script src="http://code.jquery.com/jquery-latest.js"></script> <script> window.onload=function(){ var oBody=document.body; var oP=document.getElementById("time"); setInterval(fnTime,1000); fnTime(); function fnTime(){ var myTime=new Date(); var iHours=myTime.getHours(); var iMin=myTime.getMinutes(); var iSec=myTime.getSeconds(); var str=toTwo(iHours)+toTwo(iMin)+toTwo(iSec); oP.innerHTML=str; } } /*将数字转换为字符串且一位数显示为两位,*/ function toTwo(n){ return n<10?'0'+n:''+n; } </script> <p id="time"></p>
Effect:
2. Convert numbers into pictures
Method 1:
The picture name is a number, written in the simplest way.
Pictures used:
Write a function strToImg(str) to convert each number in a string str into the corresponding image, and then dynamically create the tag.
Note: The content in the
tag needs to be cleared each time it is called.
<script></script> <script> window.onload=function(){ var oBody=document.body; var oP=document.getElementById("time"); setInterval(fnTime,1000); fnTime(); function fnTime(){ var myTime=new Date(); var iHours=myTime.getHours(); var iMin=myTime.getMinutes(); var iSec=myTime.getSeconds(); var str=toTwo(iHours)+toTwo(iMin)+toTwo(iSec); //oP.innerHTML=str; strToImg(str); } } /*将数字转换为字符串且一位数显示为两位,*/ function toTwo(n){ return n<10?'0'+n:''+n; } function strToImg(str){ var str=str; $("#time").empty(); for(var i=0;i<str.length;i++){ var oImg=$("<img src="/static/imghwm/default1.png" data-src="http://files.jb51.net/file_images/article/201511/2015112483002530.gif?2015102483010?x-oss-process=image/resize,p_40" class="lazy" / alt="Create picture clock effects with jquery_jquery" >"); oImg.attr("src","images/"+str.charAt(i)+".png"); $("#time").append(oImg); } } </script> <p id="time"></p>
Method 2: [Not applicable]
If the image name is not a pure number, save it in an array.
This method operates too many DOMs and is very inefficient. Sometimes the 6 nodes are not fully displayed.
Because my original intention was to check the information and saw that writing like this involves image preloading, and I thought it could speed up the efficiency. I tried it, but now it seems that I still don’t understand the principle of preloading, leaving a pitfall.
<script src="http://code.jquery.com/jquery-latest.js"></script> <script> window.onload=function(){ var oBody=document.body; var oP=document.getElementById("time"); setInterval(fnTime,1000); fnTime(); } function fnTime(){ var myTime=new Date(); var iHours=myTime.getHours(); var iMin=myTime.getMinutes(); var iSec=myTime.getSeconds(); var str=toTwo(iHours)+toTwo(iMin)+toTwo(iSec); //oP.innerHTML=str; strToImg(str); } /*将数字转换为字符串且一位数显示为两位,*/ function toTwo(n){ return n<10?'0'+n:''+n; } function strToImg(str){ var str=str; var imageArray=[]; for(i=0;i<11;i++){ imageArray[i]=new Image(); } //将个图像定义给相应的数组元素,使数组元素下标与图像所对应的数字字符一一对应 imageArray[0].src="images/0.png"; imageArray[1].src="images/1.png"; imageArray[2].src="images/2.png"; imageArray[3].src="images/3.png"; imageArray[4].src="images/4.png"; imageArray[5].src="images/5.png"; imageArray[6].src="images/6.png"; imageArray[7].src="images/7.png"; imageArray[8].src="images/8.png"; imageArray[9].src="images/9.png"; imageArray[10].src="images/fh.png"; $("#time").empty(); for(var i=0;i<str.length;i++){ var oImg=imageArray[str.charAt(i)]; //oImg.attr("src",imageArray[i].src); $("#time").append(oImg); } } </script> <p id="time"></p>
Method 3:
Hardcode the tag in the html.
<p id="time"><img src="/static/imghwm/default1.png" data-src="images/0.png" class="lazy"/ alt="Create picture clock effects with jquery_jquery" ><img src="/static/imghwm/default1.png" data-src="images/0.png" class="lazy"/ alt="Create picture clock effects with jquery_jquery" ><img src="/static/imghwm/default1.png" data-src="images/0.png" class="lazy"/ alt="Create picture clock effects with jquery_jquery" ><img src="/static/imghwm/default1.png" data-src="images/0.png" class="lazy"/ alt="Create picture clock effects with jquery_jquery" ><img src="/static/imghwm/default1.png" data-src="images/0.png" class="lazy"/ alt="Create picture clock effects with jquery_jquery" ><img src="/static/imghwm/default1.png" data-src="images/0.png" class="lazy"/ alt="Create picture clock effects with jquery_jquery" ></p> <script src="http://code.jquery.com/jquery-latest.js"></script> <script> window.onload=function(){ var oBody=document.body; var oP=document.getElementById("time"); setInterval(fnTime,1000); fnTime(); } function fnTime(){ var myTime=new Date(); var iHours=myTime.getHours(); var iMin=myTime.getMinutes(); var iSec=myTime.getSeconds(); var str=toTwo(iHours)+toTwo(iMin)+toTwo(iSec); //oP.innerHTML=str; strToImg(str); } var imageArray=[]; //将个图像定义给相应的数组元素,使数组元素下标与图像所对应的数字字符一一对应 imageArray[0]="images/0.png"; imageArray[1]="images/1.png"; imageArray[2]="images/2.png"; imageArray[3]="images/3.png"; imageArray[4]="images/4.png"; imageArray[5]="images/5.png"; imageArray[6]="images/6.png"; imageArray[7]="images/7.png"; imageArray[8]="images/8.png"; imageArray[9]="images/9.png"; imageArray[10]="images/fh.png"; /*将数字转换为字符串且一位数显示为两位,*/ function toTwo(n){ return n<10?'0'+n:''+n; } function strToImg(str){ var str=str; for(var i=0;i<str.length;i++){ $("#time").find("img").eq(i).attr("src",imageArray[str.charAt(i)]); } } </script>
Method 4: [Recommended]
Dynamicly generate tags and write them efficiently.
<p id="time"></p> <script></script> <script> window.onload=function(){ var oBody=document.body; var oP=document.getElementById("time"); setInterval(fnTime,1000); fnTime(); } function fnTime(){ var myTime=new Date(); var iHours=myTime.getHours(); var iMin=myTime.getMinutes(); var iSec=myTime.getSeconds(); var str=toTwo(iHours)+toTwo(iMin)+toTwo(iSec); //oP.innerHTML=str; strToImg(str); } /*将数字转换为字符串且一位数显示为两位,*/ function toTwo(n){ return n<10?'0'+n:''+n; } var imageArray=[]; //将个图像定义给相应的数组元素,使数组元素下标与图像所对应的数字字符一一对应 imageArray[0]="images/0.png"; imageArray[1]="images/1.png"; imageArray[2]="images/2.png"; imageArray[3]="images/3.png"; imageArray[4]="images/4.png"; imageArray[5]="images/5.png"; imageArray[6]="images/6.png"; imageArray[7]="images/7.png"; imageArray[8]="images/8.png"; imageArray[9]="images/9.png"; imageArray[10]="images/fh.png"; function strToImg(str){ var str=str; var tempHtml=''; for(var i=0;i<str.length;i++){ var imgHtml="<img src="/static/imghwm/default1.png" data-src="+imageArray[str.charAt(i)]+" class="lazy"/ alt="Create picture clock effects with jquery_jquery" >"; tempHtml+=imgHtml; } $("#time").html(tempHtml); } </script>
The above is all the code for creating picture clock effects with jquery. I hope you like it.
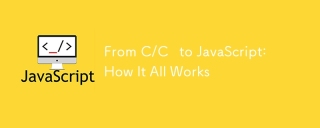
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
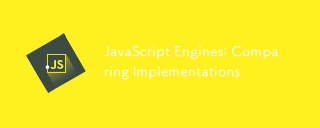
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
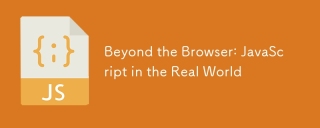
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.
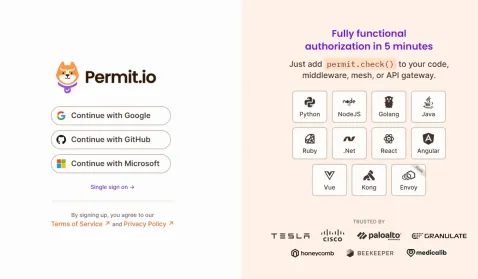
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
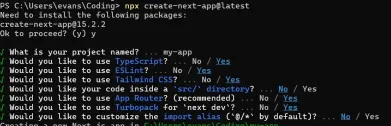
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
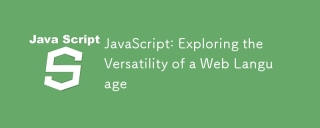
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
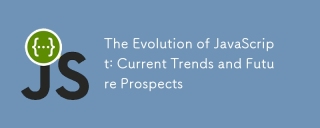
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
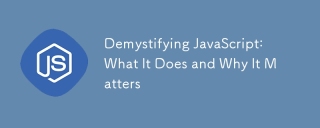
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
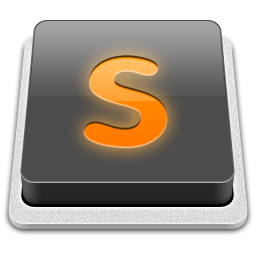
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
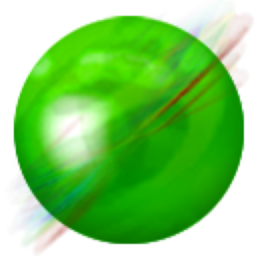
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment