


More examples of vue2.0 mobile terminal implementing pull-down refresh and pull-up loading
This article mainly introduces examples of how to implement pull-down refresh and pull-up loading on vue2.0 mobile terminal. The content is quite good. I will share it with you now and give it as a reference.
I am building a front-end architecture based on vue2.0 webpack es6 and sorting out some plug-ins. The following is a pull-down update and a pull-up for more. It is very easy to use. I would like to share it with you.
Go directly to the code. If you don’t understand, read it a few times. I will tell you how to use it below.
<template lang="html"> <p class="yo-scroll" :class="{'down':(state===0),'up':(state==1),refresh:(state===2),touch:touching}" @touchstart="touchStart($event)" @touchmove="touchMove($event)" @touchend="touchEnd($event)" @scroll="(onInfinite || infiniteLoading) ? onScroll($event) : undefined"> <section class="inner" :style="{ transform: 'translate3d(0, ' + top + 'px, 0)' }"> <header class="pull-refresh"> <slot name="pull-refresh"> <span class="down-tip">下拉更新</span> <span class="up-tip">松开更新</span> <span class="refresh-tip">更新中</span> </slot> </header> <slot></slot> <footer class="load-more"> <slot name="load-more"> <span>加载中……</span> </slot> </footer> </section> </p> </template> <script> export default { props: { offset: { type: Number, default: 40 }, enableInfinite: { type: Boolean, default: true }, enableRefresh: { type: Boolean, default: true }, onRefresh: { type: Function, default: undefined, required: false }, onInfinite: { type: Function, default: undefined, require: false } }, data() { return { top: 0, state: 0, startY: 0, touching: false, infiniteLoading: false } }, methods: { touchStart(e) { this.startY = e.targetTouches[0].pageY this.startScroll = this.$el.scrollTop || 0 this.touching = true }, touchMove(e) { if (!this.enableRefresh || this.$el.scrollTop > 0 || !this.touching) { return } let diff = e.targetTouches[0].pageY - this.startY - this.startScroll if (diff > 0) e.preventDefault() this.top = Math.pow(diff, 0.8) + (this.state === 2 ? this.offset : 0) if (this.state === 2) { // in refreshing return } if (this.top >= this.offset) { this.state = 1 } else { this.state = 0 } }, touchEnd(e) { if (!this.enableRefresh) return this.touching = false if (this.state === 2) { // in refreshing this.state = 2 this.top = this.offset return } if (this.top >= this.offset) { // do refresh this.refresh() } else { // cancel refresh this.state = 0 this.top = 0 } }, refresh() { this.state = 2 this.top = this.offset this.onRefresh(this.refreshDone) }, refreshDone() { this.state = 0 this.top = 0 }, infinite() { this.infiniteLoading = true this.onInfinite(this.infiniteDone) }, infiniteDone() { this.infiniteLoading = false }, onScroll(e) { if (!this.enableInfinite || this.infiniteLoading) { return } let outerHeight = this.$el.clientHeight let innerHeight = this.$el.querySelector('.inner').clientHeight let scrollTop = this.$el.scrollTop let ptrHeight = this.onRefresh ? this.$el.querySelector('.pull-refresh').clientHeight : 0 let infiniteHeight = this.$el.querySelector('.load-more').clientHeight let bottom = innerHeight - outerHeight - scrollTop - ptrHeight if (bottom < infiniteHeight) this.infinite() } } } </script> <style> .yo-scroll { position: absolute; top: 2.5rem; right: 0; bottom: 0; left: 0; overflow: auto; -webkit-overflow-scrolling: touch; background-color: #ddd } .yo-scroll .inner { position: absolute; top: -2rem; width: 100%; transition-duration: 300ms; } .yo-scroll .pull-refresh { position: relative; left: 0; top: 0; width: 100%; height: 2rem; display: flex; align-items: center; justify-content: center; } .yo-scroll.touch .inner { transition-duration: 0ms; } .yo-scroll.down .down-tip { display: block; } .yo-scroll.up .up-tip { display: block; } .yo-scroll.refresh .refresh-tip { display: block; } .yo-scroll .down-tip, .yo-scroll .refresh-tip, .yo-scroll .up-tip { display: none; } .yo-scroll .load-more { height: 3rem; display: flex; align-items: center; justify-content: center; } </style>
Copy the above component, save it as a component with the suffix .vue and put it under your component, and then introduce it to the page. The following is what I quoted. Code on demo
: There are comments inside, please leave me a message if you have any questions!
<template> <p> <v-scroll :on-refresh="onRefresh" :on-infinite="onInfinite"> <ul> <li v-for="(item,index) in listdata" >{{item.name}}</li> <li v-for="(item,index) in downdata" >{{item.name}}</li> </ul> </v-scroll> </p> </template> <script> import Scroll from './y-scroll/scroll'; export default{ data () { return { counter : 1, //默认已经显示出15条数据 count等于一是让从16条开始加载 num : 15, // 一次显示多少条 pageStart : 0, // 开始页数 pageEnd : 0, // 结束页数 listdata: [], // 下拉更新数据存放数组 downdata: [] // 上拉更多的数据存放数组 } }, mounted : function(){ this.getList(); }, methods: { getList(){ let vm = this; vm.$http.get('https://api.github.com/repos/typecho-fans/plugins/contents/').then((response) => { vm.listdata = response.data.slice(0,15); }, (response) => { console.log('error'); }); }, onRefresh(done) { this.getList(); done() // call done }, onInfinite(done) { let vm = this; vm.$http.get('https://api.github.com/repos/typecho-fans/plugins/contents/').then((response) => { vm.counter++; vm.pageEnd = vm.num * vm.counter; vm.pageStart = vm.pageEnd - vm.num; let arr = response.data; let i = vm.pageStart; let end = vm.pageEnd; for(; i<end; i++){ let obj ={}; obj["name"] = arr[i].name; vm.downdata.push(obj); if((i + 1) >= response.data.length){ this.$el.querySelector('.load-more').style.display = 'none'; return; } } done() // call done }, (response) => { console.log('error'); }); } }, components : { 'v-scroll': Scroll } } </script>
The above is the entire content of this article. I hope it will be helpful to everyone’s study. For more related content, please pay attention to the PHP Chinese website!
Related recommendations:
v-for loading local static image method in vue
Using Vue to dynamically generate form forms introduce
The above is the detailed content of More examples of vue2.0 mobile terminal implementing pull-down refresh and pull-up loading. For more information, please follow other related articles on the PHP Chinese website!
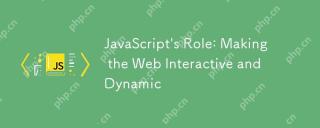
JavaScript is at the heart of modern websites because it enhances the interactivity and dynamicity of web pages. 1) It allows to change content without refreshing the page, 2) manipulate web pages through DOMAPI, 3) support complex interactive effects such as animation and drag-and-drop, 4) optimize performance and best practices to improve user experience.
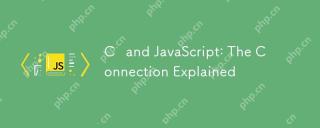
C and JavaScript achieve interoperability through WebAssembly. 1) C code is compiled into WebAssembly module and introduced into JavaScript environment to enhance computing power. 2) In game development, C handles physics engines and graphics rendering, and JavaScript is responsible for game logic and user interface.
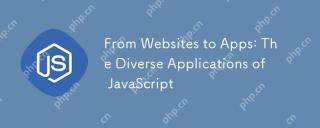
JavaScript is widely used in websites, mobile applications, desktop applications and server-side programming. 1) In website development, JavaScript operates DOM together with HTML and CSS to achieve dynamic effects and supports frameworks such as jQuery and React. 2) Through ReactNative and Ionic, JavaScript is used to develop cross-platform mobile applications. 3) The Electron framework enables JavaScript to build desktop applications. 4) Node.js allows JavaScript to run on the server side and supports high concurrent requests.
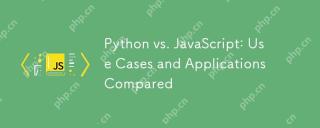
Python is more suitable for data science and automation, while JavaScript is more suitable for front-end and full-stack development. 1. Python performs well in data science and machine learning, using libraries such as NumPy and Pandas for data processing and modeling. 2. Python is concise and efficient in automation and scripting. 3. JavaScript is indispensable in front-end development and is used to build dynamic web pages and single-page applications. 4. JavaScript plays a role in back-end development through Node.js and supports full-stack development.
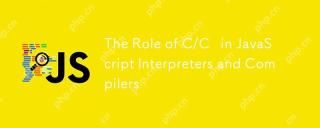
C and C play a vital role in the JavaScript engine, mainly used to implement interpreters and JIT compilers. 1) C is used to parse JavaScript source code and generate an abstract syntax tree. 2) C is responsible for generating and executing bytecode. 3) C implements the JIT compiler, optimizes and compiles hot-spot code at runtime, and significantly improves the execution efficiency of JavaScript.
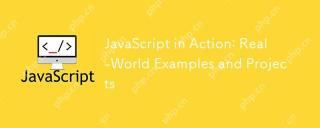
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
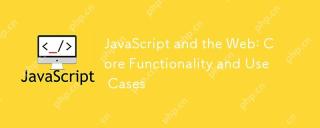
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
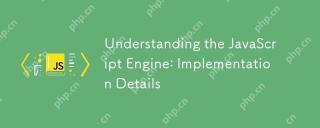
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
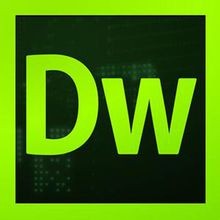
Dreamweaver CS6
Visual web development tools
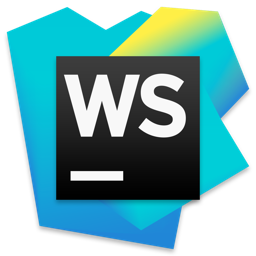
WebStorm Mac version
Useful JavaScript development tools
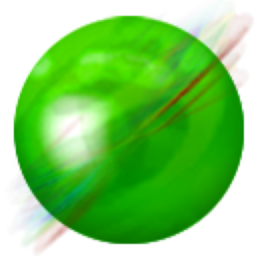
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
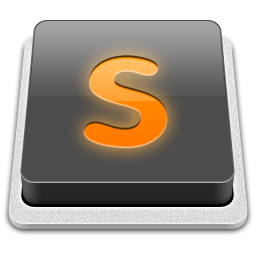
SublimeText3 Mac version
God-level code editing software (SublimeText3)