This article mainly introduces a brief discussion of React high-order components. Now I will share it with you and give you a reference.
Some time ago when I was writing a Hybrid page at work, I encountered such a scenario. The company needed a series of active components, and when each component was registered, it needed to call an interface provided by the App. Several methods were considered at the beginning, including mixins, component inheritance, and react higher-order components. But after various considerations, we finally chose to use high-level components.
So what are advanced components? First of all, you must first understand that requesting classes in ES6 is just syntactic sugar, and the essence is prototypal inheritance. To better illustrate this, we will not modify the component's code. Instead, it provides components that wrap components and enhance them with additional functionality. We call such components higher-order components (Higher-Order Component).
1. Disadvantages of Mixins
React officially does not recommend the use of Mixins technology to achieve code reuse. Mixins technology has a series of shortcomings. First, Mixins will cause naming problems. Conflict, we inject Mixins in the following way:
var myMixins = require('myMixins'); var Button = React.createClass({ mixins: [myMixins], // ... })
If you need to inject multiple mixins, one of them is your own, and the other may be a third party of. It is possible to use a method with the same name in two mixins, which will cause one of them to not work, and all you can do is change the name of one of the methods. On the other hand, a mixins may be very simple at first, only needing to implement a certain function, but when the business becomes more complex and more methods need to be added, it will become very complicated. To learn more about the shortcomings of mixins, you can check out the official blog.
2. Component inheritance
For myself, this method has been used more often. First, create a BaseComponent and implement a series of public methods in it. Each subsequent component inherits from this component, but the disadvantage is that it is not flexible enough. Only some relatively fixed methods can be implemented in the basic components, and there are great restrictions on the customization of each component.
3. React high-order components
Due to a series of shortcomings of mixins, React officials also realized that the pain points caused by using mixins are far higher than those caused by the technology itself. Advantages, high-order components can replace mixins, and it has richer uses when you go deeper.
Higher-order components (HOC) are advanced technologies in React that reuse component logic. But higher-order components themselves are not React APIs. It's just a pattern that arises inevitably from the compositional nature of React itself.
Higher-order function
Speaking of high-order components, we must first talk about high-order functions. High-order functions are functions that at least meet the following conditions :
1. Accept one or more functions as input
2. Output a function
In JavaScript, a language where functions are first-class citizens, higher-order functions There are still many uses, such as our usual callback functions, etc., which all use the knowledge of high-order functions. Let's first look at a simple higher-order function
var fun = function(x, y) { return x + y; }
fun is a function. Next we pass the entire function as a parameter to another function
var comp = function(x, y, f) { return f(x,y); }
Verify it
comp(1,2,fun) // 3
Higher-order component definition
Analogy to the definition of a higher-order function, a higher-order component accepts a component as a parameter, performs a series of processes on the component in the function, and then returns a new component as the return value.
We first define a high-order component BaseActivity
const BaseActivity = (WrappedComponent) => { return class extends Component { render() { return ( <section> <p>我的包裹组件</p> <WrappedComponent /> </section> ) } } }
The component accepts a wrapped component as a parameter and returns a processed anonymous components.
Use this high-order component in other components
class Example extends React.PureComponent { constructor(props) { super(props); this.state = { width: '100%', height: '100%' } } componentWillMount() { if ((navigator.userAgent.match(/(phone|pad|pod|iPhone|iPod|ios|iPad|Android|Mobile|BlackBerry|IEMobile|MQQBrowser|JUC|Fennec|wOSBrowser|BrowserNG|WebOS|Symbian|Windows Phone)/i))) { return; } else { this.setState({ width: '375px', height: '640px' }) } } render() { let { width, height } = this.state; return ( <p className="activity"> <p className="activity-content" style={{ width, height }}> <button className="btn">参加活动</button> </p> </p> ) } } export default BaseActivity(Example);
The specific usage is to use the BaseActivity function when exporting the component. Wrap this component and look at the output react dom content
An anonymous component is wrapped outside the Example component.
Parameters
Since the higher-order component is a function, we can pass the parameters we need to it
const BaseActivity = (WrappedComponent, title) => { return class extends Component { render() { return ( <section> <p>{title}</p> <WrappedComponent /> </section> ) } } }
Export like this in Example
export default BaseActivity(Example, '这是高阶组件的参数');
Let’s take a look at the output react dom
You can see that the parameters have been passed in.
Of course you can also use it like this (currying)
const BaseActivity (title) => (WrappedComponent) => { return class extends Component { render() { return ( <section> <p>{title}</p> <WrappedComponent /> </section> ) } } }
Export like this in Example
export default BaseActivity('这是高阶组件的参数')(Example);
We can see this usage in the form of ant-design and the connect of redux
// ant const WrappedDemo = Form.create()(Demo) // redux export default connect(mapStateToProps, mapDispatchToProps)(Counter)
High-order components You can also extend the props attribute of the original component, as shown below:
const BaseActivity (title) => (WrappedComponent) => { return class extends Component { render() { const newProps = { id: Math.random().toString(8) } return ( <section> <p>{title}</p> <WrappedComponent {...this.props} {...newProps}/> </section> ) } } }
Look at the output react dom
Disadvantages of higher-order components
High-order components also have a series of disadvantages. The first is that the static method of the wrapped component will Disappear, this is actually very easy to understand. When we pass the component into the function as a parameter, what is returned is not the original component, but a new component. The original static method naturally no longer exists. If we need to retain it, we can manually copy the methods of the original component to the new component, or use a library such as hoist-non-react-statics to copy.
The above is what I compiled for everyone. I hope it will be helpful to everyone in the future.
Related articles:
Ajax method to obtain response content length
Ajax method to regularly update a certain piece of content on the page Method
Ajax method of reading properties resource file data
The above is the detailed content of A brief discussion of React high-order components. For more information, please follow other related articles on the PHP Chinese website!
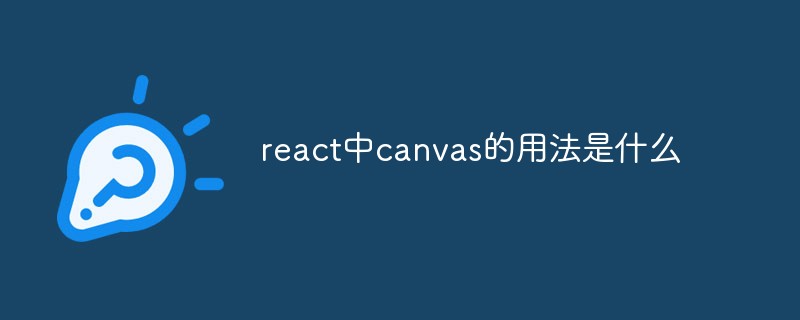
在react中,canvas用于绘制各种图表、动画等;可以利用“react-konva”插件使用canvas,该插件是一个canvas第三方库,用于使用React操作canvas绘制复杂的画布图形,并提供了元素的事件机制和拖放操作的支持。
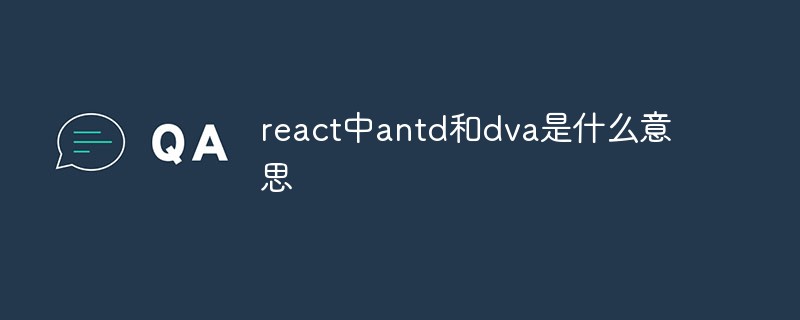
在react中,antd是基于Ant Design的React UI组件库,主要用于研发企业级中后台产品;dva是一个基于redux和“redux-saga”的数据流方案,内置了“react-router”和fetch,可理解为应用框架。
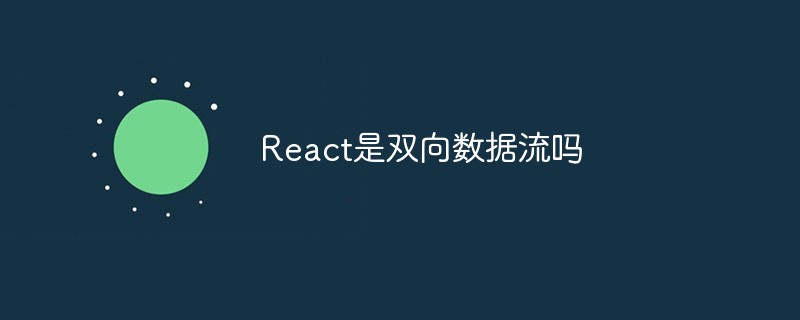
React不是双向数据流,而是单向数据流。单向数据流是指数据在某个节点被改动后,只会影响一个方向上的其他节点;React中的表现就是数据主要通过props从父节点传递到子节点,若父级的某个props改变了,React会重渲染所有子节点。
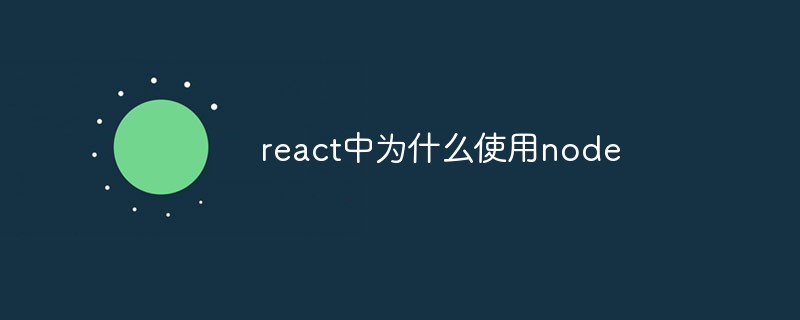
因为在react中需要利用到webpack,而webpack依赖nodejs;webpack是一个模块打包机,在执行打包压缩的时候是依赖nodejs的,没有nodejs就不能使用webpack,所以react需要使用nodejs。
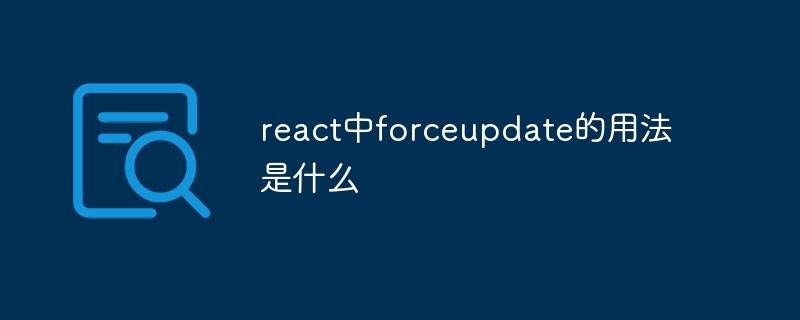
在react中,forceupdate()用于强制使组件跳过shouldComponentUpdate(),直接调用render(),可以触发组件的正常生命周期方法,语法为“component.forceUpdate(callback)”。
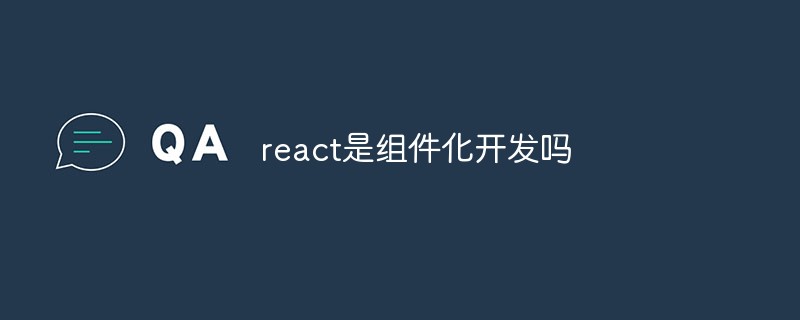
react是组件化开发;组件化是React的核心思想,可以开发出一个个独立可复用的小组件来构造应用,任何的应用都会被抽象成一颗组件树,组件化开发也就是将一个页面拆分成一个个小的功能模块,每个功能完成自己这部分独立功能。
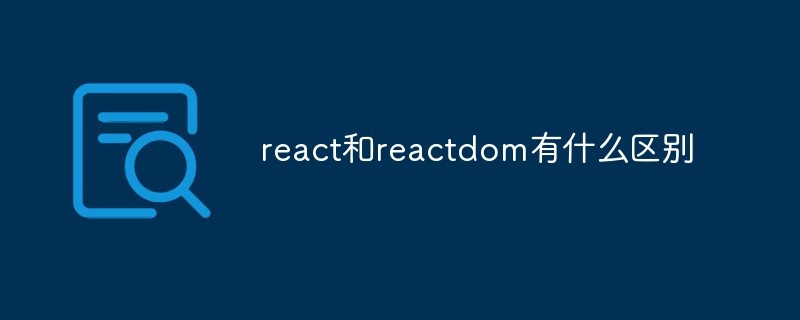
react和reactdom的区别是:ReactDom只做和浏览器或DOM相关的操作,例如“ReactDOM.findDOMNode()”操作;而react负责除浏览器和DOM以外的相关操作,ReactDom是React的一部分。
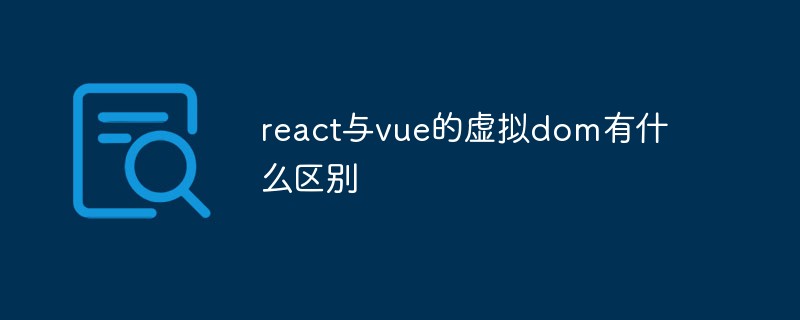
react与vue的虚拟dom没有区别;react和vue的虚拟dom都是用js对象来模拟真实DOM,用虚拟DOM的diff来最小化更新真实DOM,可以减小不必要的性能损耗,按颗粒度分为不同的类型比较同层级dom节点,进行增、删、移的操作。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
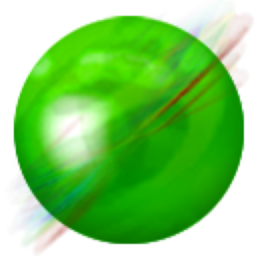
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version

Notepad++7.3.1
Easy-to-use and free code editor
