This article mainly introduces in detail the five-step code to easily implement the JavaScript HTML clock effect. It has certain reference value. Interested friends can refer to it. I hope it can help everyone.
After learning HTML, CSS and JS for a period of time, I will make a beautiful HTML clock that does not look like a powerful one. Take a look at the picture first:
Next, we use 5 steps to make it
step1, Prepare HTML
First, we need to prepare the HTML structure, background, dial, hands (hour hand, minute hand, second hand), and numbers.<p id="clock"> <p class="bg"> <p class="point"> <p id="hour"></p> <p id="minute"></p> <p id="second"></p> <p class="circle"></p> </p> </p> < /p>
step2, Prepare CSS
Define the color and size of the pointer. What needs to be explained is transform: rotate(-90deg ); is used to rotate the pointer, transform-origin:0 6px; is used to set the rotation center point.<style> *{ margin: 0; padding: 0; } #clock{ margin: 5% auto; width: 400px; height: 400px; border-radius: 10px; background: #aaa; position: relative; transform: rotate(-90deg); } #clock .bg{ width: 360px; height: 360px; border-radius: 50%; background: #fff; position: absolute; left: 50%; top: 50%; margin-left: -180px; margin-top: -180px; } #clock .point{ position: absolute; left: 50%; top: 50%; margin-left: -14px; margin-top: -14px; } #clock #hour{ width: 80px; height: 16px; background: #000; margin: 6px 0 0 14px; /*transform: rotate(30deg);*/ transform-origin:0 8px; /*animation: hour 3s linear 100!* alternate*!;*/ border-radius: 16px; } #clock #minute{ width: 120px; height: 12px; background: #000; margin: -14px 0 0 14px; transform-origin:0 6px; border-radius: 12px; } #clock #second{ width: 160px; height: 6px; background: #f00; margin: -9px 0 0 14px; transform-origin:0 3px; border-radius: 6px; } #clock .point .circle{ width: 28px; height: 28px; border-radius: 50%; background: #000; position: absolute; left: 0; top: 0; } @keyframes hour { from {transform: rotate(0deg);} to {transform: rotate(360deg);} } #clock .number{ position: absolute; font-size: 34px; width: 50px; height: 50px; line-height: 50px; text-align: center; transform: rotate(90deg); } < /style>
step3, Calculate the hour hand position
JS obtains the current time through the Date object, getHours obtains the hour, and getMinutes obtains Minutes, getSeconds gets seconds. One rotation of the hour hand is 12 divisions, and the angle of each division is 360°/12. The minutes and seconds are both 60 divisions, and the angle of each division is 360°/60.function clock(){ var date = new Date();//获取当前时间 //时(0-23) 分(0-59)秒(0-59) //计算转动角度 var hourDeg = date.getHours()*360/12; var minuteDeg = date.getMinutes()*360/60; var secondDeg = date.getSeconds()*360/60; //console.log(hourDeg, minuteDeg, secondDeg); //设置指针 hour.style.transform = 'rotate('+hourDeg+'deg)'; minute.style.transform = 'rotate('+minuteDeg+'deg)'; second.style.transform = 'rotate('+secondDeg+'deg)'; }
step4, Clock rotation
Set the timer through setInterval and refresh it every second. Note that the initialization needs to be executed once, otherwise the effect will not be visible until 1s later.//初始化执行一次 clock(); setInterval(clock,1000);
step5, Drawing digital time
Digital time is also on a circle, we only need to determine the radius , and then get the coordinates on the radius. Just position each number by the left side. Take a look at the calculation rules of the circular coordinate system:* x = pointX + r*cos(angle);
* y = pointY + r*sin(angle);
But note that the coordinates we calculate are the coordinates of the point on the arc. When positioning each number, it is positioned based on the upper left corner point of the element, so that our numbers There will be an offset. That is to say, the center point of the number is not on the arc. The solution is to translate the coordinate point (x, y) by half of itself (x-w/2, y-h/2)
, so that the center point of the number is on the arc. .
var pointX = 200; var pointY = 200; var r = 150; function drawNumber(){ var deg = Math.PI*2/12;//360° for (var i = 1; i <= 12; i++) { //计算每格的角度 var angle = deg*i; //计算圆上的坐标 var x = pointX + r*Math.cos(angle); var y = pointY + r*Math.sin(angle); //创建p,写入数字 var number = document.createElement('p'); number.className = 'number'; number.innerHTML = i; //减去自身的一半, 让p的中心点在圆弧上 number.style.left = x - 25 + 'px'; number.style.top = y - 25 + 'px'; //添加到页面 myClock.appendChild(number); } }Complete JS code:
<script> /*** * 时钟: * 1> 旋转: rotate(90deg) * 2> 旋转中心点: transform-origin * */ //TODO step1: 获取时钟的指针 var hour = document.getElementById('hour');//时针 var minute = document.getElementById('minute');//分针 var second = document.getElementById('second');//秒针 var myClock = document.getElementById('clock');//时钟 //TODO step2: 获取当前时间,把指针放在正确的位置 function clock(){ var date = new Date();//获取当前时间 //时(0-23) 分(0-59)秒(0-59) //计算转动角度 var hourDeg = date.getHours()*360/12; var minuteDeg = date.getMinutes()*360/60; var secondDeg = date.getSeconds()*360/60; //console.log(hourDeg, minuteDeg, secondDeg); //设置指针 hour.style.transform = 'rotate('+hourDeg+'deg)'; minute.style.transform = 'rotate('+minuteDeg+'deg)'; second.style.transform = 'rotate('+secondDeg+'deg)'; } //初始化执行一次 clock(); //TODO step3: 设置定时器 setInterval(clock,1000); /*** * 圆半径坐标计算: * x = pointX + r*cos(angle); * y = pointY + r*sin(angle); * */ var pointX = 200; var pointY = 200; var r = 150; //TODO step4: 画时钟数字 function drawNumber(){ var deg = Math.PI*2/12;//360° for (var i = 1; i <= 12; i++) { //计算每格的角度 var angle = deg*i; //计算圆上的坐标 var x = pointX + r*Math.cos(angle); var y = pointY + r*Math.sin(angle); //创建p,写入数字 var number = document.createElement('p'); number.className = 'number'; number.innerHTML = i; //减去自身的一半, 让p的中心点在圆弧上 number.style.left = x - 25 + 'px'; number.style.top = y - 25 + 'px'; //添加到页面 myClock.appendChild(number); } } drawNumber(); < /script>How about it? Do you know it? Related recommendations:
Detailed explanation of the creative clock project in JavaScript
Steps to implement the clock using canvas
JavaScript implements the "Creative Clock" project
The above is the detailed content of Simple method to implement JavaScript HTML clock effect. For more information, please follow other related articles on the PHP Chinese website!
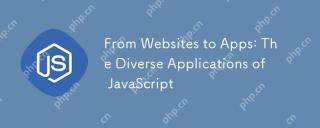
JavaScript is widely used in websites, mobile applications, desktop applications and server-side programming. 1) In website development, JavaScript operates DOM together with HTML and CSS to achieve dynamic effects and supports frameworks such as jQuery and React. 2) Through ReactNative and Ionic, JavaScript is used to develop cross-platform mobile applications. 3) The Electron framework enables JavaScript to build desktop applications. 4) Node.js allows JavaScript to run on the server side and supports high concurrent requests.
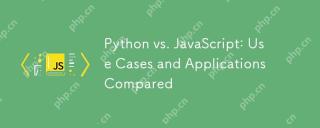
Python is more suitable for data science and automation, while JavaScript is more suitable for front-end and full-stack development. 1. Python performs well in data science and machine learning, using libraries such as NumPy and Pandas for data processing and modeling. 2. Python is concise and efficient in automation and scripting. 3. JavaScript is indispensable in front-end development and is used to build dynamic web pages and single-page applications. 4. JavaScript plays a role in back-end development through Node.js and supports full-stack development.
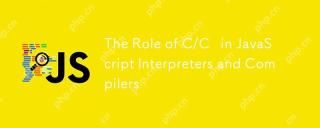
C and C play a vital role in the JavaScript engine, mainly used to implement interpreters and JIT compilers. 1) C is used to parse JavaScript source code and generate an abstract syntax tree. 2) C is responsible for generating and executing bytecode. 3) C implements the JIT compiler, optimizes and compiles hot-spot code at runtime, and significantly improves the execution efficiency of JavaScript.
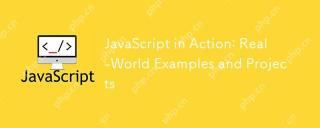
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
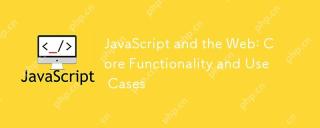
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
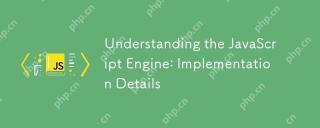
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
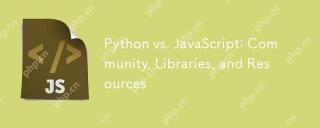
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
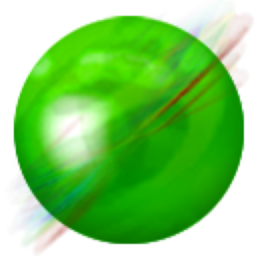
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment