


This article mainly introduces you to the relevant information on how to use the Laravel event system to implement login logging. The introduction in the article is very detailed and has certain reference and learning value for everyone. Friends who need it can take a look below.
This article introduces the relevant content of using the Laravel event system to implement login logging. It is shared for your reference. Let’s take a look at the detailed introduction:
Clear requirements
To record a login log, the following information is usually required:
Client Agent information
-
Client IP address
Access IP location
Login time
Login user Information
Establish tools
After clarifying your needs, find the tools you need based on each need.
Requirement 1 jenssegers/agent can meet our requirements
Requirement 2 Directly under Laravel
Request::getClientIp( )
Requirement 3 zhuzhichao/ip-location-zhThis package can meet the requirements
-
Requirement 4 time()
Requirement 5 Login user model
Start working
Using Laravel To implement an event subscription system, you need to implement a login event and a login event listener.
Generating events and listeners
Laravel command line supports automatically generating events and listeners. Add the events that need to be implemented in App\Providers\EventServiceProvider:
protected $listen = [ ..., //添加登录事件及对应监听器,一个事件可绑定多个监听器 'App\Events\LoginEvent' => [ 'App\Listeners\LoginListener', ], ];
Run the command: php artisan event:generate
The events and listeners will be automatically generated. Existing events and listeners will not be Changes will occur.
Login Event (Event)
Looking back at the requirements, our login event requires 5 points of information. This information needs to be recorded in the event, so the event The design is as follows:
namespace App\Events; use Illuminate\Broadcasting\Channel; use Illuminate\Queue\SerializesModels; use Illuminate\Broadcasting\PrivateChannel; use Illuminate\Foundation\Events\Dispatchable; use Illuminate\Broadcasting\InteractsWithSockets; use App\Models\User; use Jenssegers\Agent\Agent; class LoginEvent { use Dispatchable, InteractsWithSockets, SerializesModels; /** * @var User 用户模型 */ protected $user; /** * @var Agent Agent对象 */ protected $agent; /** * @var string IP地址 */ protected $ip; /** * @var int 登录时间戳 */ protected $timestamp; /** * 实例化事件时传递这些信息 */ public function __construct($user, $agent, $ip, $timestamp) { $this->user = $user; $this->agent = $agent; $this->ip = $ip; $this->timestamp = $timestamp; } public function getUser() { return $this->user; } public function getAgent() { return $this->agent; } public function getIp() { return $this->ip; } public function getTimestamp() { return $this->timestamp; } /** * Get the channels the event should broadcast on. * * @return Channel|array */ public function broadcastOn() { return new PrivateChannel('channel-default'); } }
Record the required information in the event and implement the get method of this information.
Login Listener(Listener)
In the listener, obtain the information passed by the event and record the information into the database. The implementation is as follows :
namespace App\Listeners; use App\Events\LoginEvent; class LoginListener { // handle方法中处理事件 public function handle(LoginEvent $event) { //获取事件中保存的信息 $user = $event->getUser(); $agent = $event->getAgent(); $ip = $event->getIp(); $timestamp = $event->getTimestamp(); //登录信息 $login_info = [ 'ip' => $ip, 'login_time' => $timestamp, 'user_id' => $user->id ]; // zhuzhichao/ip-location-zh 包含的方法获取ip地理位置 $addresses = \Ip::find($ip); $login_info['address'] = implode(' ', $addresses); // jenssegers/agent 的方法来提取agent信息 $login_info['device'] = $agent->device(); //设备名称 $browser = $agent->browser(); $login_info['browser'] = $browser . ' ' . $agent->version($browser); //浏览器 $platform = $agent->platform(); $login_info['platform'] = $platform . ' ' . $agent->version($platform); //操作系统 $login_info['language'] = implode(',', $agent->languages()); //语言 //设备类型 if ($agent->isTablet()) { // 平板 $login_info['device_type'] = 'tablet'; } else if ($agent->isMobile()) { // 便捷设备 $login_info['device_type'] = 'mobile'; } else if ($agent->isRobot()) { // 爬虫机器人 $login_info['device_type'] = 'robot'; $login_info['device'] = $agent->robot(); //机器人名称 } else { // 桌面设备 $login_info['device_type'] = 'desktop'; } //插入到数据库 DB::table('login_log')->insert($login_info); } }
In this way, the listener is completed. Every time the login event is triggered, a piece of login information will be added to the database.
Trigger events
Trigger events through the global event()
method, event()
method The parameters are event instances:
namespace App\Controllers; ... use App\Events\LoginEvent; use Jenssegers\Agent\Agent; class AuthControoler extends Controller { ... public function login(Request $request) { //登录实现 ... //登录成功,触发事件 event(new LoginEvent($this->guard()->user(), new Agent(), \Request::getClientIp(), time())); ... } }
Queue listener
Sometimes the listener will perform some time-consuming operations , at this time, the listener should be queued in conjunction with Laravel's queue system, provided that the queue has been configured and the queue processor has been enabled.
Queuing is very simple, just the listener needs to implement the ShouldQueue interface, that is:
namespace App\Listeners; ... use Illuminate\Contracts\Queue\ShouldQueue; class LoginListener implements ShouldQueue { /** * 失败重试次数 * @var int */ public $tries = 1; ... }
Summary
Laravel's event system is very elegant to implement. Various types of listeners can be easily added to the same event, and each listener does not interfere with each other and is decoupled. Sex is very strong. Coupled with the queue system, some follow-up tasks can be easily processed.
The above is the detailed content of Introduction to related methods of using Laravel event system to complete login logging. For more information, please follow other related articles on the PHP Chinese website!
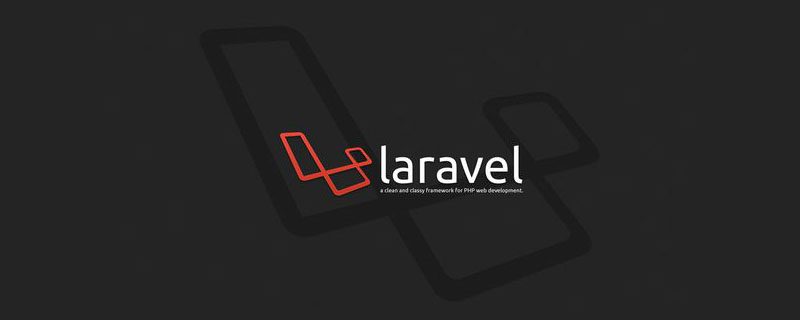
本篇文章给大家带来了关于laravel的相关知识,其中主要介绍了关于单点登录的相关问题,单点登录是指在多个应用系统中,用户只需要登录一次就可以访问所有相互信任的应用系统,下面一起来看一下,希望对大家有帮助。
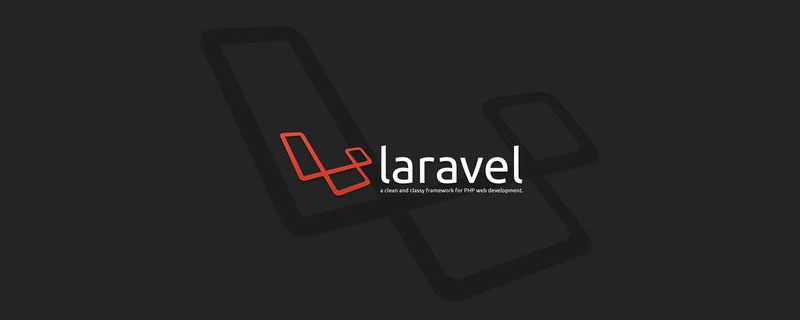
本篇文章给大家带来了关于laravel的相关知识,其中主要介绍了关于Laravel的生命周期相关问题,Laravel 的生命周期从public\index.php开始,从public\index.php结束,希望对大家有帮助。
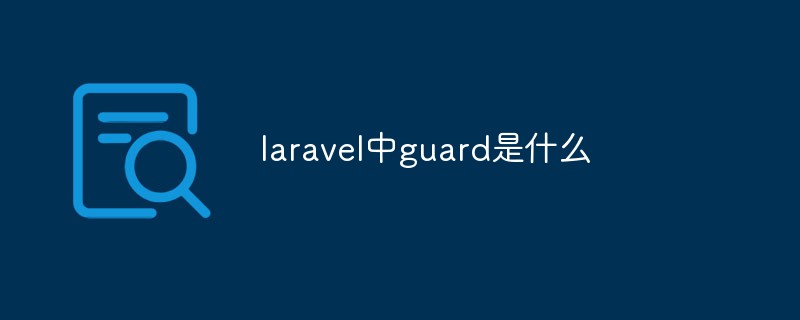
在laravel中,guard是一个用于用户认证的插件;guard的作用就是处理认证判断每一个请求,从数据库中读取数据和用户输入的对比,调用是否登录过或者允许通过的,并且Guard能非常灵活的构建一套自己的认证体系。
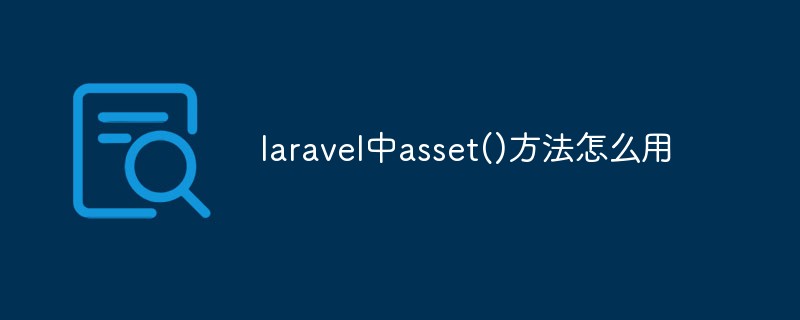
laravel中asset()方法的用法:1、用于引入静态文件,语法为“src="{{asset(‘需要引入的文件路径’)}}"”;2、用于给当前请求的scheme前端资源生成一个url,语法为“$url = asset('前端资源')”。
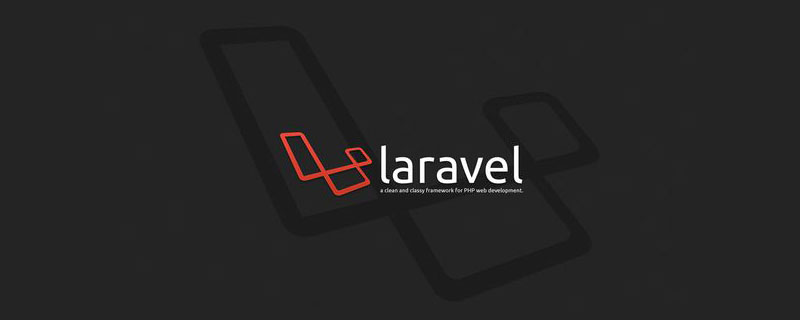
本篇文章给大家带来了关于laravel的相关知识,其中主要介绍了关于使用中间件记录用户请求日志的相关问题,包括了创建中间件、注册中间件、记录用户访问等等内容,下面一起来看一下,希望对大家有帮助。
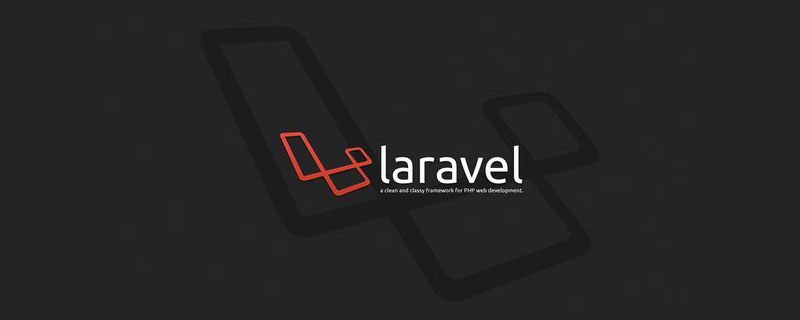
本篇文章给大家带来了关于laravel的相关知识,其中主要介绍了关于中间件的相关问题,包括了什么是中间件、自定义中间件等等,中间件为过滤进入应用的 HTTP 请求提供了一套便利的机制,下面一起来看一下,希望对大家有帮助。
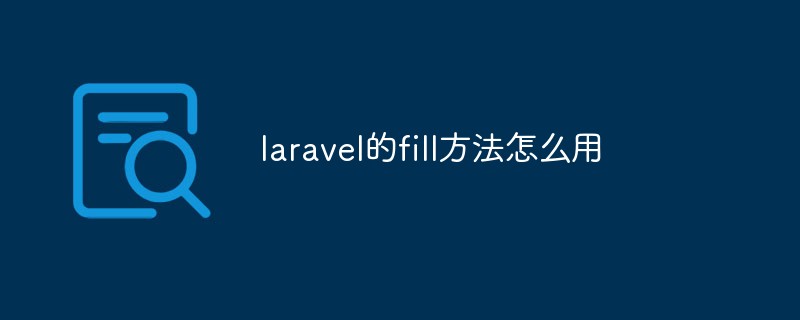
在laravel中,fill方法是一个给Eloquent实例赋值属性的方法,该方法可以理解为用于过滤前端传输过来的与模型中对应的多余字段;当调用该方法时,会先去检测当前Model的状态,根据fillable数组的设置,Model会处于不同的状态。
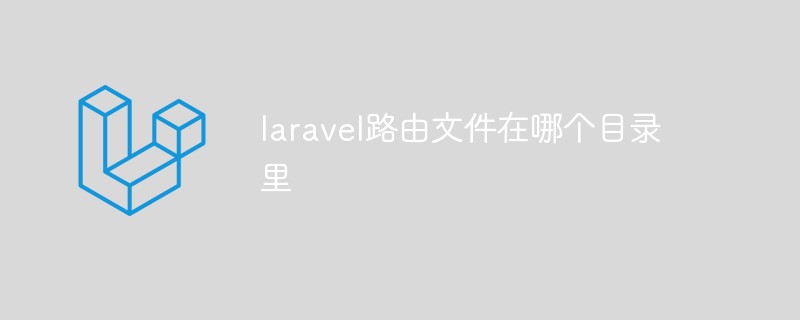
laravel路由文件在“routes”目录里。Laravel中所有的路由文件定义在routes目录下,它里面的内容会自动被框架加载;该目录下默认有四个路由文件用于给不同的入口使用:web.php、api.php、console.php等。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
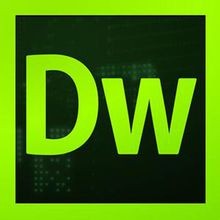
Dreamweaver CS6
Visual web development tools
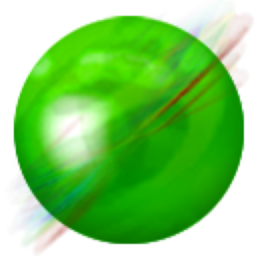
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor
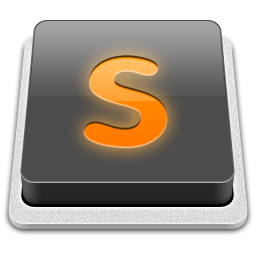
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
