这篇文章主要介绍了使用C#创建Windows服务的实例代码,小编觉得挺不错的,现在分享给大家,也给大家做个参考。一起跟随小编过来看看吧
本文介绍了使用C#创建Windows服务的实例代码,分享给大家
一、开发环境
操作系统:Windows 10 X64
开发环境:VS2015
编程语言:C#
.NET版本:.NET Framework 4.0
目标平台:X86
二、创建Windows Service
1、新建一个Windows Service,并将项目名称改为“MyWindowsService”,如下图所示:
2、在解决方案资源管理器内将Service1.cs改为MyService1.cs后并点击“查看代码”图标按钮进入代码编辑器界面,如下图所示:
3、在代码编辑器内如入以下代码,如下所示:
using System; using System.ServiceProcess; using System.IO; namespace MyWindowsService { public partial class MyService : ServiceBase { public MyService() { InitializeComponent(); } string filePath = @"D:\MyServiceLog.txt"; protected override void OnStart(string[] args) { using (FileStream stream = new FileStream(filePath,FileMode.Append)) using (StreamWriter writer = new StreamWriter(stream)) { writer.WriteLine($"{DateTime.Now},服务启动!"); } } protected override void OnStop() { using (FileStream stream = new FileStream(filePath, FileMode.Append)) using (StreamWriter writer = new StreamWriter(stream)) { writer.WriteLine($"{DateTime.Now},服务停止!"); } } } }
4、双击项目“MyWindowsService”进入“MyService”设计界面,在空白位置右击鼠标弹出上下文菜单,选中“添加安装程序”,如下图所示:
5、此时软件会生成两个组件,分别为“serviceInstaller1”及“serviceProcessInstaller1”,如下图所示:
6、点击“serviceInstaller1”,在“属性”窗体将ServiceName改为MyService,Description改为我的服务,StartType保持为Manual,如下图所示:
7、点击“serviceProcessInstaller1”,在“属性”窗体将Account改为LocalSystem(服务属性系统级别),如下图所示:
8、鼠标右键点击项目“MyWindowsService”,在弹出的上下文菜单中选择“生成”按钮,如下图所示:
9、至此,Windows服务已经创建完毕。
三、创建安装、启动、停止、卸载服务的Windows窗体
1、在同一个解决方案里新建一个Windows Form项目,并命名为WindowsServiceClient,如下图所示:
2、将该项目设置为启动项目,并在窗体内添加四个按钮,分别为安装服务、启动服务、停止服务及卸载服务,如下图所示:
3、按下F7进入代码编辑界面,引用“System.ServiceProcess”及“System.Configuration.Install”,并输入如下代码:
using System; using System.Collections; using System.Windows.Forms; using System.ServiceProcess; using System.Configuration.Install; namespace WindowsServiceClient { public partial class Form1 : Form { public Form1() { InitializeComponent(); } string serviceFilePath = $"{Application.StartupPath}\\MyWindowsService.exe"; string serviceName = "MyService"; //事件:安装服务 private void button1_Click(object sender, EventArgs e) { if (this.IsServiceExisted(serviceName)) this.UninstallService(serviceName); this.InstallService(serviceFilePath); } //事件:启动服务 private void button2_Click(object sender, EventArgs e) { if (this.IsServiceExisted(serviceName)) this.ServiceStart(serviceName); } //事件:停止服务 private void button4_Click(object sender, EventArgs e) { if (this.IsServiceExisted(serviceName)) this.ServiceStop(serviceName); } //事件:卸载服务 private void button3_Click(object sender, EventArgs e) { if (this.IsServiceExisted(serviceName)) { this.ServiceStop(serviceName); this.UninstallService(serviceFilePath); } } //判断服务是否存在 private bool IsServiceExisted(string serviceName) { ServiceController[] services = ServiceController.GetServices(); foreach (ServiceController sc in services) { if (sc.ServiceName.ToLower() == serviceName.ToLower()) { return true; } } return false; } //安装服务 private void InstallService(string serviceFilePath) { using (AssemblyInstaller installer = new AssemblyInstaller()) { installer.UseNewContext = true; installer.Path = serviceFilePath; IDictionary savedState = new Hashtable(); installer.Install(savedState); installer.Commit(savedState); } } //卸载服务 private void UninstallService(string serviceFilePath) { using (AssemblyInstaller installer = new AssemblyInstaller()) { installer.UseNewContext = true; installer.Path = serviceFilePath; installer.Uninstall(null); } } //启动服务 private void ServiceStart(string serviceName) { using (ServiceController control = new ServiceController(serviceName)) { if (control.Status == ServiceControllerStatus.Stopped) { control.Start(); } } } //停止服务 private void ServiceStop(string serviceName) { using (ServiceController control = new ServiceController(serviceName)) { if (control.Status == ServiceControllerStatus.Running) { control.Stop(); } } } } }
4、为了后续调试服务及安装卸载服务的需要,将已生成的MyWindowsService.exe引用到本Windows窗体,如下图所示:
5、由于需要安装服务,故需要使用UAC中Administrator的权限,鼠标右击项目“WindowsServiceClient”,在弹出的上下文菜单中选择“添加”->“新建项”,在弹出的选择窗体中选择“应用程序清单文件”并单击确定,如下图所示:
6、打开该文件,并将
7. After the IDE is started, the following form will pop up (some systems may not display it due to UAC configuration), which needs to be opened with administrator rights:
8. After reopening, run the WindowsServiceClient project in the IDE;
9. Use WIN+R to open the run form and enter services in the form. .msc and open the service, as shown in the figure below:
10. Click the "Install Service" button in the form, MyService will appear in the service, as shown in the figure below Display:
11. Click the "Run Service" button to start and run the service, as shown below:
Additional: How to debug the service
1. To debug the service, it is actually very simple. If you need to attach the service process to the project that needs to be debugged, if To debug the service you just built, set a breakpoint in the OnStop event as follows:The above is the detailed content of How to create a Windows service in C# (picture)?. For more information, please follow other related articles on the PHP Chinese website!
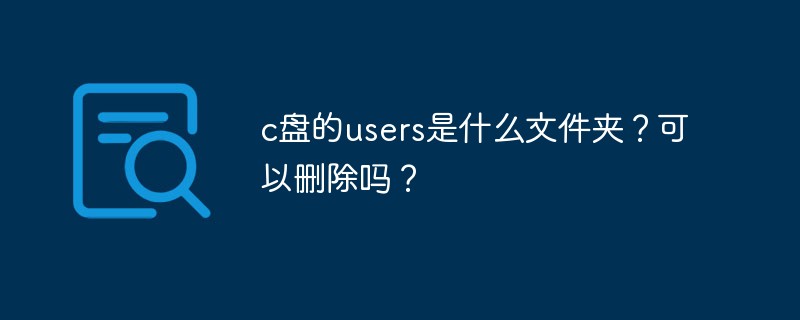
c盘的users是用户文件夹,主要存放用户的各项配置文件。users文件夹是windows系统的重要文件夹,不能随意删除;它保存了很多用户信息,一旦删除会造成数据丢失,严重的话会导致系统无法启动。
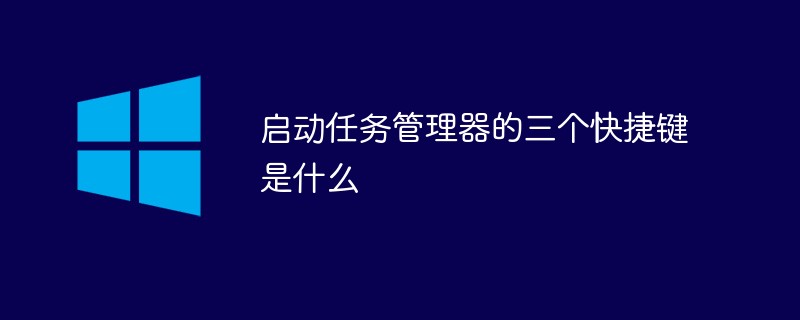
启动任务管理器的三个快捷键是:1、“Ctrl+Shift+Esc”,可直接打开任务管理器;2、“Ctrl+Alt+Delete”,会进入“安全选项”的锁定界面,选择“任务管理器”,即可以打开任务管理器;3、“Win+R”,会打开“运行”窗口,输入“taskmgr”命令,点击“确定”即可调出任务管理器。
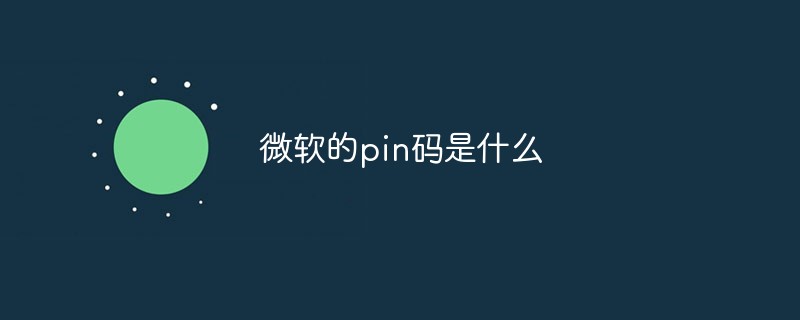
PIN码是Windows系统为了方便用户本地登录而独立于window账户密码的快捷登录密码,是Windows系统新添加的一套本地密码策略;在用户登陆了Microsoft账户后就可以设置PIN来代替账户密码,不仅提高安全性,而且也可以让很多和账户相关的操作变得更加方便。PIN码只能通过本机登录,无法远程使用,所以不用担心PIN码被盗。
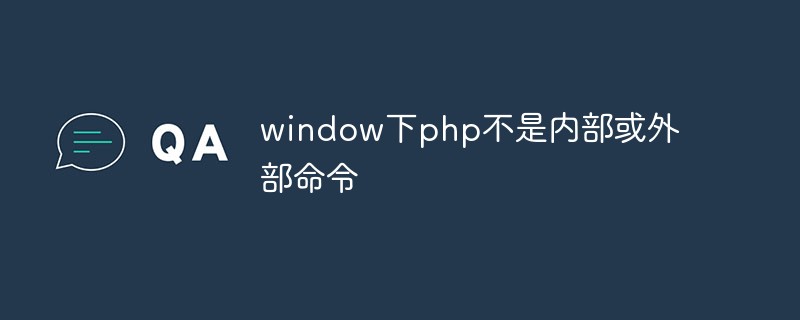
对于刚刚开始使用PHP的用户来说,如果在Windows操作系统中遇到了“php不是内部或外部命令”的问题,可能会感到困惑。这个错误通常是由于系统无法识别PHP的路径导致的。在本文中,我将为您提供一些可能会导致这个问题的原因和解决方法,以帮助您快速解决这个问题。
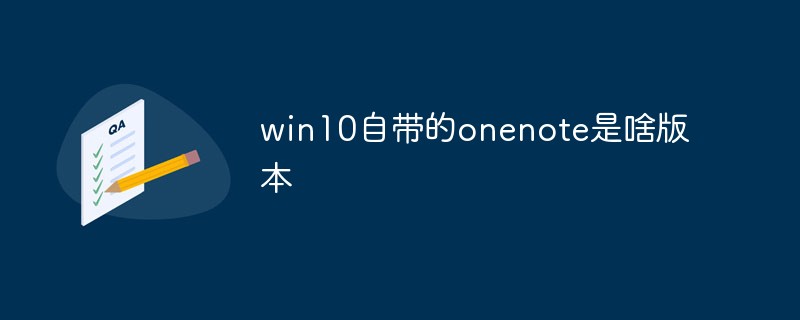
win10自带的onenote是UWP版本;onenote是一套用于自由形式的信息获取以及多用户协作工具,而UWP版本是“Universal Windows Platform”的简称,表示windows通用应用平台,不是为特定的终端设计的,而是针对使用windows系统的各种平台。
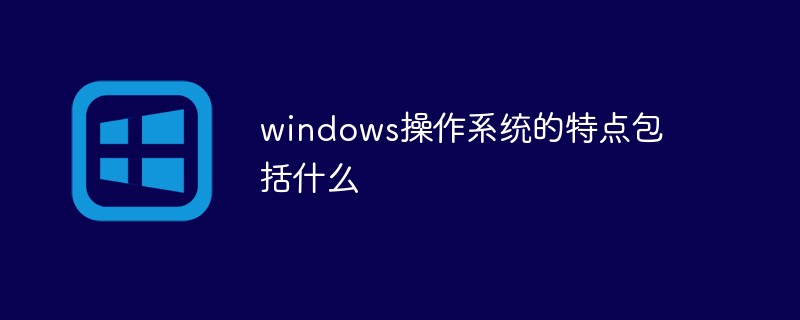
windows操作系统的特点包括:1、图形界面;直观高效的面向对象的图形用户界面,易学易用。2、多任务;允许用户同时运行多个应用程序,或在一个程序中同时做几件事情。3、即插即用。4、出色的多媒体功能。5、对内存的自动化管理。
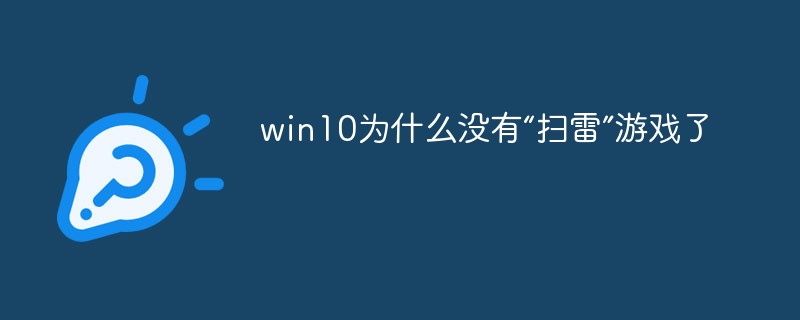
因为win10系统是不自带扫雷游戏的,需要用户自行手动安装。安装步骤:1、点击打开“开始菜单”;2、在打开的菜单中,找到“Microsoft Store”应用商店,并点击进入;3、在应用商店主页的搜索框中,搜索“minesweeper”;4、在搜索结果中,点击选择需要下载的“扫雷”游戏;5、点击“获取”按钮,等待获取完毕后自动完成安装游戏即可。
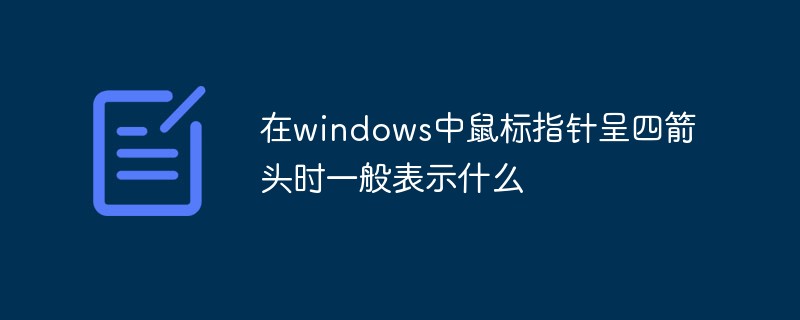
在windows中鼠标指针呈四箭头时一般表示选中对象可以上、下、左、右移动。在Windows中鼠标指针首次用不同的指针来表示不同的状态,如系统忙、移动中、拖放中;在Windows中使用的鼠标指针文件还被称为“光标文件”或“动态光标文件”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
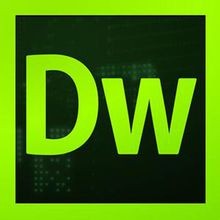
Dreamweaver CS6
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
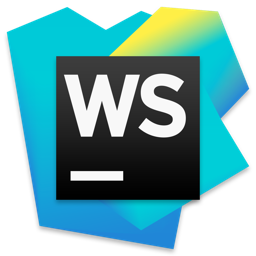
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
