php Generate signature and verify signature
<?php /** * 根据原文生成签名内容 * * @param string $data 原文内容 * * @return string */ function sign($data) { $filePath = 'test.p12'; if(!file_exists($filePath)) { return false; } $pkcs12 = file_get_contents($filePath); if (openssl_pkcs12_read($pkcs12, $certs, '读取证书所需要的密码')) { $privateKey = $certs['pkey']; //根据实际情况键值可能不同 $publicKey = $certs['cert']; //根据实际情况键值可能不同 $binary_signature = ""; if (openssl_sign($data, $binarySignature, $privateKey, OPENSSL_ALGO_SHA1)) { return $binarySignature; } else { return ''; } } else { return ''; } } /** * 验证签名自己生成的是否正确 * * @param string $data 签名的原文 * @param string $signature 签名 * * @return bool */ function verifySign($data, $signature) { $filePath = 'test.p12'; if(!file_exists($filePath)) { return false; } $pkcs12 = file_get_contents($filePath); if (openssl_pkcs12_read($pkcs12, $certs, '读取证书所需要的密码')) { $publicKey = $certs['cert']; $ok = openssl_verify($data, $signature, $publicKey); if ($ok == 1) { return true; } } return false; } /** * 验证返回的签名是否正确 * * @param string $data 要验证的签名原文 * @param string $signature 签名内容 * * @return bool */ function verifyRespondSign($data, $signature) { $filePath = 'allinpay-pds.pem'; if(!file_exists($filePath)) { return false; } $fp = fopen($filePath, "r"); $cert = fread($fp, 8192); fclose($fp); $pubkeyid = openssl_get_publickey($cert); if(!is_resource($pubkeyid)) { return false; } $ok = openssl_verify($data, $signature, $pubkeyid); if ($ok == 1) { openssl_free_key($pubkeyid); return true; } return false; } ?>
openssl_sign The default signature_alg parameter is OPENSSL_ALGO_SHA1
Needed if using DSA encryption method OPENSSL_ALGO_DSS1 parameter
Signature_alg Other parameters
OPENSSL_ALGO_DSS1 (integer)
OPENSSL_ALGO_SHA1 (integer)
OPENSSL_ALGO_SHA224 (integer)
OPENSSL_ALGO_SHA256 (integer)
OPENSSL_ALGO_SHA384 (integer)
OPENSSL_ALGO_SHA512 (integer)
OPENSSL_ALGO_RMD160 (integer)
OPENSSL_ALGO_MD5 (integer)
OPENSSL_ALGO_MD4 (integer)
OPENSSL_ALGO_MD2 (integer)
The above is the detailed content of How to generate and verify signature example code in php. For more information, please follow other related articles on the PHP Chinese website!
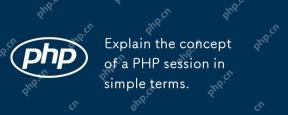
PHPsessionstrackuserdataacrossmultiplepagerequestsusingauniqueIDstoredinacookie.Here'showtomanagethemeffectively:1)Startasessionwithsession_start()andstoredatain$_SESSION.2)RegeneratethesessionIDafterloginwithsession_regenerate_id(true)topreventsessi
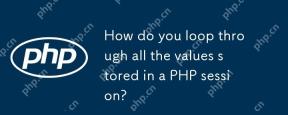
In PHP, iterating through session data can be achieved through the following steps: 1. Start the session using session_start(). 2. Iterate through foreach loop through all key-value pairs in the $_SESSION array. 3. When processing complex data structures, use is_array() or is_object() functions and use print_r() to output detailed information. 4. When optimizing traversal, paging can be used to avoid processing large amounts of data at one time. This will help you manage and use PHP session data more efficiently in your actual project.
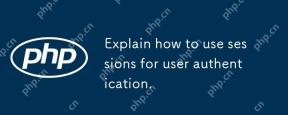
The session realizes user authentication through the server-side state management mechanism. 1) Session creation and generation of unique IDs, 2) IDs are passed through cookies, 3) Server stores and accesses session data through IDs, 4) User authentication and status management are realized, improving application security and user experience.
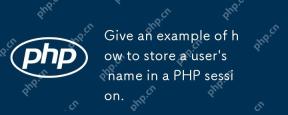
Tostoreauser'snameinaPHPsession,startthesessionwithsession_start(),thenassignthenameto$_SESSION['username'].1)Usesession_start()toinitializethesession.2)Assigntheuser'snameto$_SESSION['username'].Thisallowsyoutoaccessthenameacrossmultiplepages,enhanc
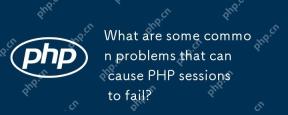
Reasons for PHPSession failure include configuration errors, cookie issues, and session expiration. 1. Configuration error: Check and set the correct session.save_path. 2.Cookie problem: Make sure the cookie is set correctly. 3.Session expires: Adjust session.gc_maxlifetime value to extend session time.
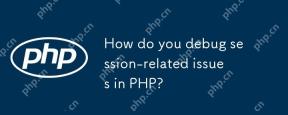
Methods to debug session problems in PHP include: 1. Check whether the session is started correctly; 2. Verify the delivery of the session ID; 3. Check the storage and reading of session data; 4. Check the server configuration. By outputting session ID and data, viewing session file content, etc., you can effectively diagnose and solve session-related problems.
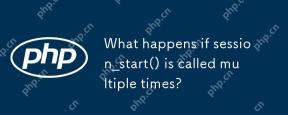
Multiple calls to session_start() will result in warning messages and possible data overwrites. 1) PHP will issue a warning, prompting that the session has been started. 2) It may cause unexpected overwriting of session data. 3) Use session_status() to check the session status to avoid repeated calls.
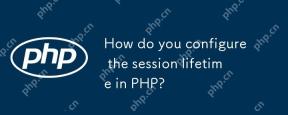
Configuring the session lifecycle in PHP can be achieved by setting session.gc_maxlifetime and session.cookie_lifetime. 1) session.gc_maxlifetime controls the survival time of server-side session data, 2) session.cookie_lifetime controls the life cycle of client cookies. When set to 0, the cookie expires when the browser is closed.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Atom editor mac version download
The most popular open source editor
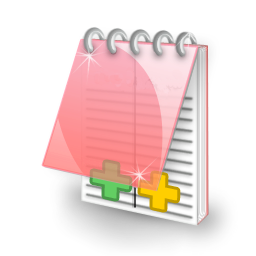
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 English version
Recommended: Win version, supports code prompts!

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
