Background Analysis
First look at a piece of js code. When adding, first determine whether it exists through an asynchronous request. If it does not exist, add it:
function add(url,data) { var isExited = isExited(data); if(!isExited){ addRequest(url, data); } }
When I add a piece of data, I first judge whether it exists in the database (of course, if the front-end and back-end are completely separated, the front-end should not judge the business logic, the front-end should only be used to display the data), first , the request of isExited() is an ajax request implementation. This is asynchronous. Obviously, the interface is likely to execute the following function when no result is returned (usually yes), making the value of isExited undefined. , this is obviously not what you want. If you want to achieve similar functions, you can use a callback function. A case is introduced below.
The process is as follows
The front-end jsp interface is as follows:
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8" %> <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %> <head> <meta charset="utf-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1"> <title>JS回调函数案例</title> <!-- Bootstrap --> <link href="<c:url value='/lib/bootstrap/css/bootstrap.min.css'/>" rel="stylesheet"> <script type="text/javascript"> /** * 删除的请求 */ function supplierDelete(element) { var id = element.parentNode.parentNode.cells[0].innerHTML; modalDeleteRequest('${pageContext.request.contextPath}/oms/supplier/remove/', id); } </script> </head> <body> <!-- 顶部导航 --> <div class="navbar navbar-inverse navbar-fixed-top" role="navigation" id="menu-nav"> </div> <div class="container partner-table-container textFont"> <table class="table table-striped detailTableSet"> <caption><h2 id="JS回调函数案例">JS回调函数案例</h2></caption> <br> <tr class="table-hover form-horizontal"> <td class="info">123</td> <td class="info">123</td> <td class="info">123</td> <td class="info">123</td> <td class="info">123</td> </tr> <tr> <td>123</td> <td>123</td> <td>123</td> <td>123</td> <td>123</td> <td> <a onclick="supplierUpdate(this)">修改</a> <a onclick="supplierDelete(this)">删除</a> </td> </tr> </table> </div> <!--显示成功失败的modal--> <%@include file="/modal-custom.jsp" %> <script src="<c:url value='/lib/jquery-1.8.3.min.js'/>"></script> <script src="<c:url value='/lib/bootstrap/js/bootstrap.min.js'/>"></script> <script type="text/javascript" src="<c:url value='/js/modal-operate.js'/>"></script> </body> </html>
The main js code is as follows:
<script type="text/javascript"> /** * 删除的请求 */ function supplierDelete(element) { var id = element.parentNode.parentNode.cells[0].innerHTML; modalDeleteRequest('${pageContext.request.contextPath}/oms/supplier/remove/', id); } </script>
Here is when the button is clicked to delete, but I want to pop up a confirmation deletion dialog box. If the confirmation is selected after the pop-up, the specific deletion method will be called. There is also a modal box (bootstrap) referenced here. Frame), mainly used to display pop-up box information, the code is as follows:
<%@ page language="java" pageEncoding="UTF-8" %> <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %> <!-- 模态框(Modal) --> <div class="modal fade" id="modal-result" tabindex="-1" role="dialog" aria-labelledby="myModalLabel" aria-hidden="true"> <div class="modal-dialog"> <div class="modal-content"> <div class="modal-header"> <button type="button" class="close" data-dismiss="modal" aria-hidden="true"> × </button> <h4 class="modal-title" id="myModalLabel"> 信息 </h4> </div> <div class="modal-body" id="modal-add-result-text"> </div> <div class="modal-footer"> <button type="button" class="btn btn-default" data-dismiss="modal">关闭 </button> </div> </div> <!-- /.modal-content --> </div> <!-- /.modal --> </div>
The following is today’s protagonist:
/** * 删除请求的操作 * @param url 删除请求的url * @param id 删除的id */ function modalDeleteRequest(url, id) { confirmIsDelete(url, id, deleteRequest); } /** * 在删除警告框确认之后调用的回调函数 * @param url * @param id */ function deleteRequest(url, id) { $.get(url + id, function (result) { $("#modal-add-result-text").text(result.msg); $("#modal-result").modal('show'); }, "json"); } /** * 弹出对话框确认是否删除 * @param url 删除请求的url * @param id 删除请求的id * @param callback 回调函数,在最后的时候需要进行回调的函数 */ function confirmIsDelete(url, id, callback) { var confirmDeleteDialog = $('<div class="modal fade"><div class="modal-dialog">' + '<div class="modal-content"><div class="modal-header"><button type="button" class="close" ' + 'data-dismiss="modal" aria-hidden="true">×</button>' + '<h4 id="确认删除">确认删除</h4></div><div class="modal-body">' + '<div class="alert alert-warning">确认要删除吗?删除之后无法恢复哦!</div></div><div class="modal-footer">' + '<button type="button" class="btn btn-default" data-dismiss="modal">取消</button>' + '<button type="button" class="btn btn-success" id="deleteOK">删除</button></div></div>' + '</div></div>'); confirmDeleteDialog.modal({ keyboard: false }).on({ 'hidden.bs.modal': function () { $(this).remove(); } }); var deleteConfirm = confirmDeleteDialog.find('#deleteOK'); deleteConfirm.on('click', function () { confirmDeleteDialog.modal('hide'); //隐藏dialog //需要回调的函数 callback(deleteRequest(url, id)); }); }
Write picture description here
Write picture description here
Since there is a lot of code above, let’s look at a simple framework below:
/** * 回调函数测试方法 * * @param callback * 回调的方法 */ function testCallback(callback) { alert('come in!'); callback(); } /** * 被回调的函数 */ function a() { alert('a'); } /** * 开始测试方法 */ function start() { testCallback(a); }
This is the end of the callback. I hope it will be helpful for everyone to learn. The editor also has a deeper understanding of js custom callback functions.
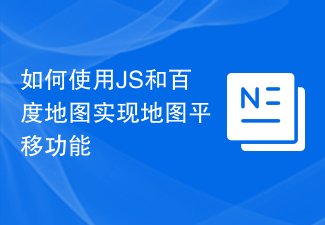
如何使用JS和百度地图实现地图平移功能百度地图是一款广泛使用的地图服务平台,在Web开发中经常用于展示地理信息、定位等功能。本文将介绍如何使用JS和百度地图API实现地图平移功能,并提供具体的代码示例。一、准备工作使用百度地图API前,首先需要在百度地图开放平台(http://lbsyun.baidu.com/)上申请一个开发者账号,并创建一个应用。创建完成
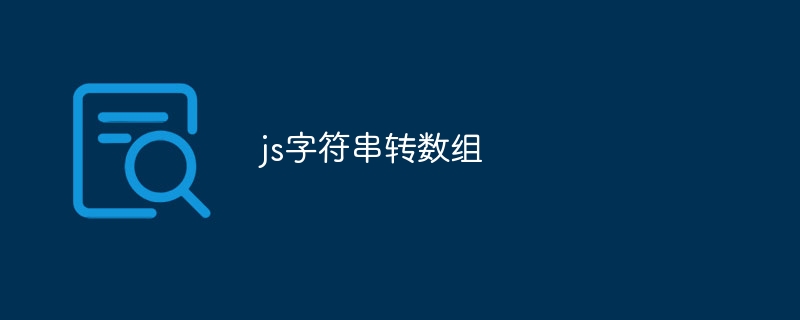
js字符串转数组的方法:1、使用“split()”方法,可以根据指定的分隔符将字符串分割成数组元素;2、使用“Array.from()”方法,可以将可迭代对象或类数组对象转换成真正的数组;3、使用for循环遍历,将每个字符依次添加到数组中;4、使用“Array.split()”方法,通过调用“Array.prototype.forEach()”将一个字符串拆分成数组的快捷方式。
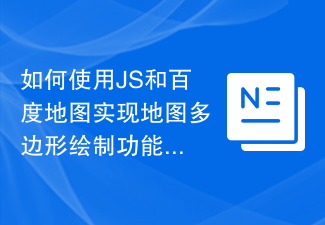
如何使用JS和百度地图实现地图多边形绘制功能在现代网页开发中,地图应用已经成为常见的功能之一。而地图上绘制多边形,可以帮助我们将特定区域进行标记,方便用户进行查看和分析。本文将介绍如何使用JS和百度地图API实现地图多边形绘制功能,并提供具体的代码示例。首先,我们需要引入百度地图API。可以利用以下代码在HTML文件中导入百度地图API的JavaScript
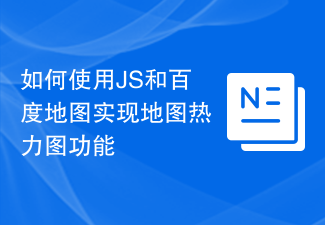
如何使用JS和百度地图实现地图热力图功能简介:随着互联网和移动设备的迅速发展,地图成为了一种普遍的应用场景。而热力图作为一种可视化的展示方式,能够帮助我们更直观地了解数据的分布情况。本文将介绍如何使用JS和百度地图API来实现地图热力图的功能,并提供具体的代码示例。准备工作:在开始之前,你需要准备以下事项:一个百度开发者账号,并创建一个应用,获取到相应的AP
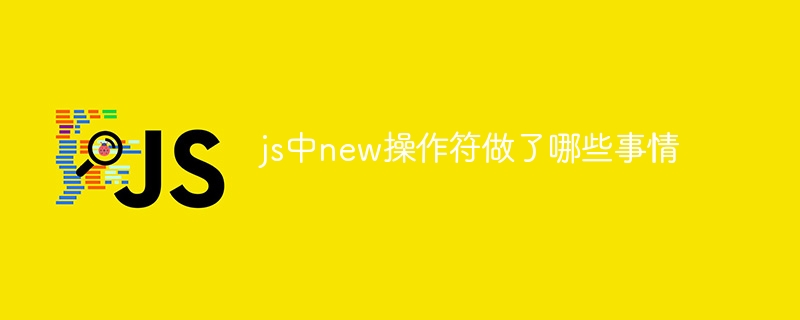
js中new操作符做了:1、创建一个空对象,这个新对象将成为函数的实例;2、将新对象的原型链接到构造函数的原型对象,这样新对象就可以访问构造函数原型对象中定义的属性和方法;3、将构造函数的作用域赋给新对象,这样新对象就可以通过this关键字来引用构造函数中的属性和方法;4、执行构造函数中的代码,构造函数中的代码将用于初始化新对象的属性和方法;5、如果构造函数中没有返回等等。
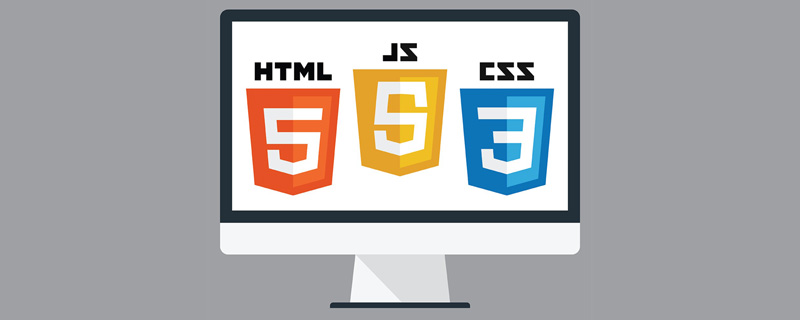
这篇文章主要为大家详细介绍了js实现打字小游戏,文中示例代码介绍的非常详细,具有一定的参考价值,感兴趣的小伙伴们可以参考一下。
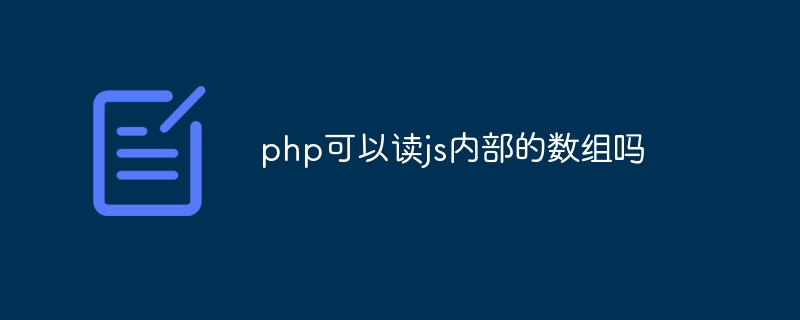
php在特定情况下可以读js内部的数组。其方法是:1、在JavaScript中,创建一个包含需要传递给PHP的数组的变量;2、使用Ajax技术将该数组发送给PHP脚本。可以使用原生的JavaScript代码或者使用基于Ajax的JavaScript库如jQuery等;3、在PHP脚本中,接收传递过来的数组数据,并进行相应的处理即可。
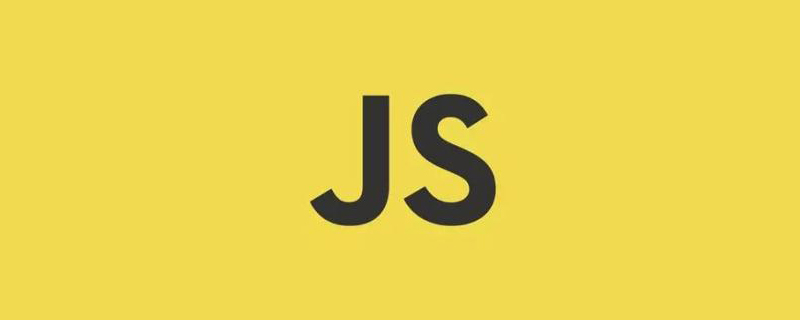
js全称JavaScript,是一种具有函数优先的轻量级,直译式、解释型或即时编译型的高级编程语言,是一种属于网络的高级脚本语言;JavaScript基于原型编程、多范式的动态脚本语言,并且支持面向对象、命令式和声明式,如函数式编程。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Zend Studio 13.0.1
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
