


This article mainly introduces the dynamic Lambda expression generated by SqlDataReader. Friends who need it can refer to it
The previous article uses dynamic lambda expression to convert DataTable into entities. It is much faster than using reflection directly. few. The main reason is that the commission is dynamically generated when the first line is converted.
The subsequent conversions all call the delegate directly, eliminating the performance loss caused by multiple reflections.
Today we are processing the stream object SqlDataReader returned by SqlServer, and also converting entities by dynamically generating Lambda expressions.
First version of the code
using System; using System.Collections.Generic; using System.Data; using System.Data.Common; using System.Data.SqlClient; using System.Linq; using System.Linq.Expressions; using System.Reflection; using System.Text; using System.Threading.Tasks; namespace Demo1 { public static class EntityConverter { #region /// <summary> /// DataTable生成实体 /// </summary> /// <typeparam name="T"></typeparam> /// <param name="dataTable"></param> /// <returns></returns> public static List<T> ToList<T>(this DataTable dataTable) where T : class, new() { if (dataTable == null || dataTable.Rows.Count <= 0) throw new ArgumentNullException("dataTable", "当前对象为null无法生成表达式树"); Func<DataRow, T> func = dataTable.Rows[0].ToExpression<T>(); List<T> collection = new List<T>(dataTable.Rows.Count); foreach (DataRow dr in dataTable.Rows) { collection.Add(func(dr)); } return collection; } /// <summary> /// 生成表达式 /// </summary> /// <typeparam name="T"></typeparam> /// <param name="dataRow"></param> /// <returns></returns> public static Func<DataRow, T> ToExpression<T>(this DataRow dataRow) where T : class, new() { if (dataRow == null) throw new ArgumentNullException("dataRow", "当前对象为null 无法转换成实体"); ParameterExpression parameter = Expression.Parameter(typeof(DataRow), "dr"); List<MemberBinding> binds = new List<MemberBinding>(); for (int i = 0; i < dataRow.ItemArray.Length; i++) { String colName = dataRow.Table.Columns[i].ColumnName; PropertyInfo pInfo = typeof(T).GetProperty(colName); if (pInfo == null || !pInfo.CanWrite) continue; MethodInfo mInfo = typeof(DataRowExtensions).GetMethod("Field", new Type[] { typeof(DataRow), typeof(String) }).MakeGenericMethod(pInfo.PropertyType); MethodCallExpression call = Expression.Call(mInfo, parameter, Expression.Constant(colName, typeof(String))); MemberAssignment bind = Expression.Bind(pInfo, call); binds.Add(bind); } MemberInitExpression init = Expression.MemberInit(Expression.New(typeof(T)), binds.ToArray()); return Expression.Lambda<Func<DataRow, T>>(init, parameter).Compile(); } #endregion /// <summary> /// 生成lambda表达式 /// </summary> /// <typeparam name="T"></typeparam> /// <param name="reader"></param> /// <returns></returns> public static Func<SqlDataReader, T> ToExpression<T>(this SqlDataReader reader) where T : class, new() { if (reader == null || reader.IsClosed || !reader.HasRows) throw new ArgumentException("reader", "当前对象无效"); ParameterExpression parameter = Expression.Parameter(typeof(SqlDataReader), "reader"); List<MemberBinding> binds = new List<MemberBinding>(); for (int i = 0; i < reader.FieldCount; i++) { String colName = reader.GetName(i); PropertyInfo pInfo = typeof(T).GetProperty(colName); if (pInfo == null || !pInfo.CanWrite) continue; MethodInfo mInfo = reader.GetType().GetMethod("GetFieldValue").MakeGenericMethod(pInfo.PropertyType); MethodCallExpression call = Expression.Call(parameter, mInfo, Expression.Constant(i)); MemberAssignment bind = Expression.Bind(pInfo, call); binds.Add(bind); } MemberInitExpression init = Expression.MemberInit(Expression.New(typeof(T)), binds.ToArray()); return Expression.Lambda<Func<SqlDataReader, T>>(init, parameter).Compile(); } } }
Based on the previous article, the extension method of SqlDataReader is added
The following code is called
using System; using System.Collections.Generic; using System.Data; using System.Data.Common; using System.Data.SqlClient; using System.Diagnostics; using System.Linq; using System.Reflection; using System.Text; using System.Threading.Tasks; namespace Demo1 { class Program { static void Main(string[] args) { String conString = "Data Source=.; Initial Catalog=master; Integrated Security=true;"; Func<SqlDataReader, Usr> func = null; List<Usr> usrs = new List<Usr>(); using (SqlDataReader reader = GetReader(conString, "select object_id 'ID',name 'Name' from sys.objects", CommandType.Text, null)) { while (reader.Read()) { if (func == null) { func = reader.ToExpression<Usr>(); } Usr usr = func(reader); usrs.Add(usr); } } usrs.Clear(); Console.ReadKey(); } public static SqlDataReader GetReader(String conString, String sql, CommandType type, params SqlParameter[] pms) { SqlConnection conn = new SqlConnection(conString); SqlCommand cmd = new SqlCommand(sql, conn); cmd.CommandType = type; if (pms != null && pms.Count() > 0) { cmd.Parameters.AddRange(pms); } conn.Open(); return cmd.ExecuteReader(CommandBehavior.CloseConnection); } } class Usr { public Int32 ID { get; set; } public String Name { get; set; } } }
Currently only Ability to process objects returned by sqlserver and other databases. Originally, I wanted to add the extension method of DbDataReader, but I found an error in dynamically generating lambda expressions, so I recorded the current
solution first.
The above is the detailed content of Detailed explanation of examples of dynamic Lambda expressions generated by SqlDataReader in ASP.NET. For more information, please follow other related articles on the PHP Chinese website!
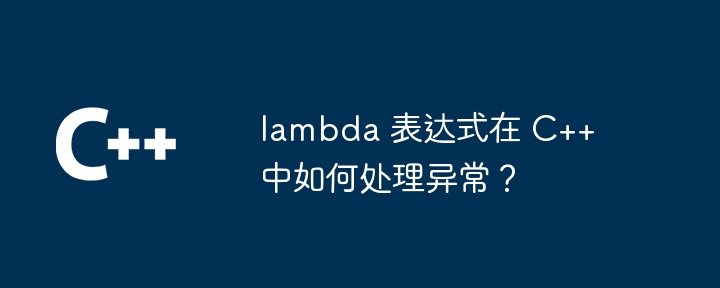
在C++中,使用Lambda表达式处理异常有两种方法:使用try-catch块捕获异常,并在catch块中处理或重新抛出异常。使用std::function类型的包装函数,其try_emplace方法可以捕获Lambda表达式中的异常。
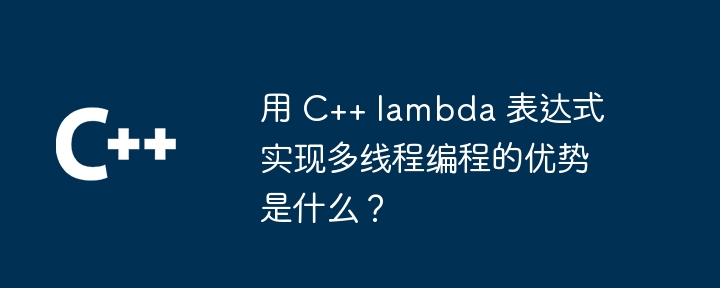
lambda表达式在C++多线程编程中的优势包括:简洁性、灵活性、易于传参和并行性。实战案例:使用lambda表达式创建多线程,在不同线程中打印线程ID,展示了该方法的简洁和易用性。
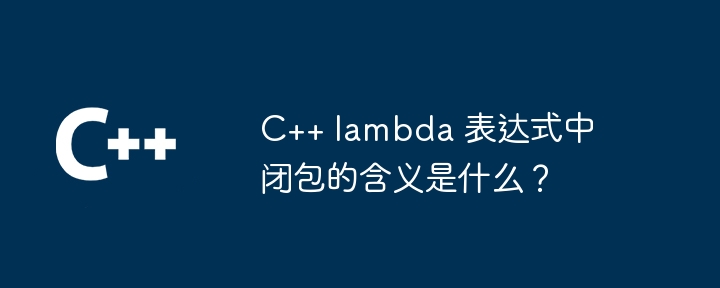
在C++中,闭包是能够访问外部变量的lambda表达式。要创建闭包,请捕获lambda表达式中的外部变量。闭包提供可复用性、信息隐藏和延迟求值等优势。它们在事件处理程序等实际情况中很有用,其中即使外部变量被销毁,闭包仍然可以访问它们。
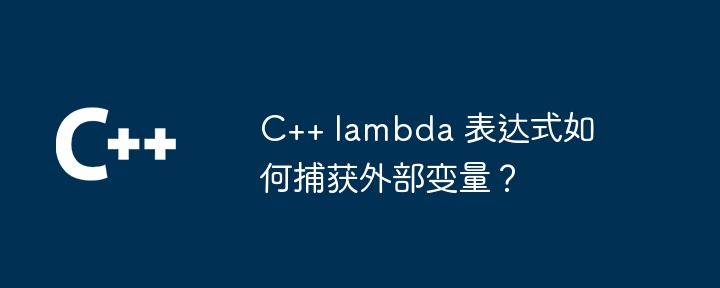
在C++中捕获外部变量的lambda表达式有三种方法:按值捕获:创建一个变量副本。按引用捕获:获得变量引用。同时按值和引用捕获:允许捕获多个变量,按值或按引用。
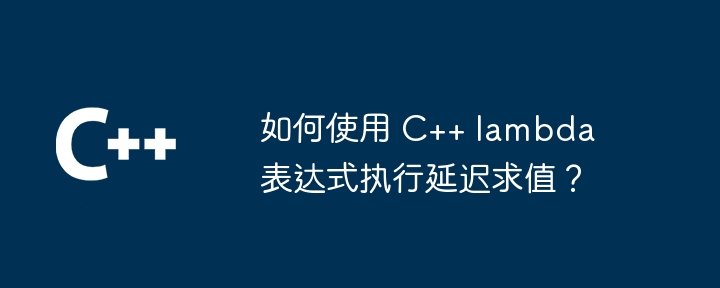
如何使用C++lambda表达式执行延迟求值?使用lambda表达式创建延迟求值的函数对象。延迟计算推迟到需要时才执行。仅当需要时才计算结果,提高性能。
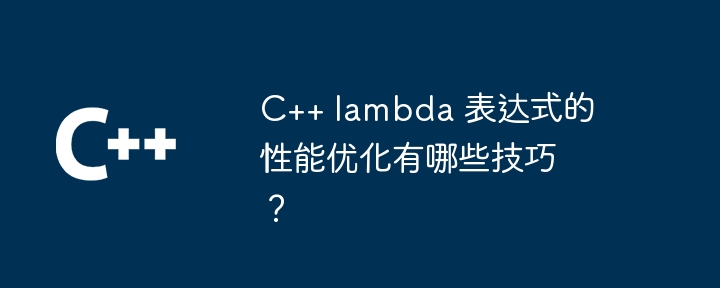
优化C++Lambda表达式的性能技巧包括:避免创建不必要的lambda对象通过std::bind显式捕获最小的对象使用std::move移动捕获的变量以避免复制优化lambda体,避免不必要的内存分配、重复计算和全局变量访问
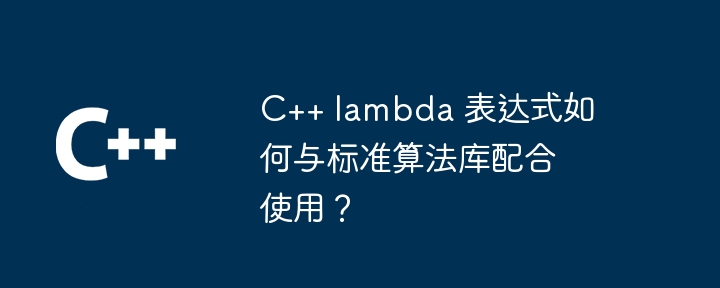
C++Lambda表达式与标准算法库紧密协作,允许创建匿名函数,简化对数据的处理。具体用途包括:排序向量:使用lambda表达式对元素进行排序。查找元素:使用lambda表达式在容器中查找特定元素。
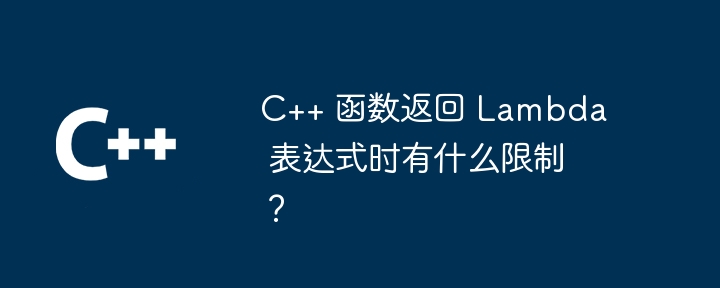
回答:C++函数可以返回Lambda表达式,但存在以下限制:限制:Lambda表达式应捕获存储类型(CapturesbyValue)Lambda表达式不能返回局部变量Lambda表达式不能返回Lambda表达式


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
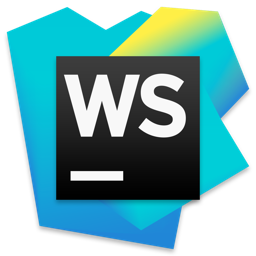
WebStorm Mac version
Useful JavaScript development tools
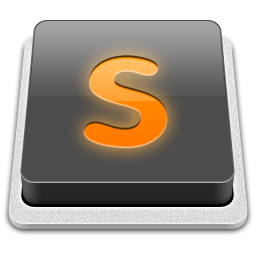
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Chinese version
Chinese version, very easy to use

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
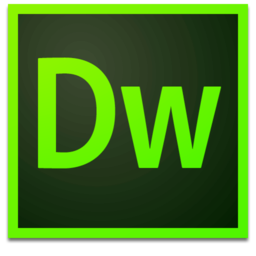
Dreamweaver Mac version
Visual web development tools
