


Example code for using python to implement the functions of wc command program
The basic code of python is used here to implement the basic functions of the wc command program under the Linux system.
#!/usr/bin/env python #encoding: utf-8 # Author: liwei # Function: wc program by python from optparse import OptionParser import sys,os def opt(): parser = OptionParser() parser.add_option('-c', '--char', dest='chars', action='store_true', default=False, help='only count chars') parser.add_option('-w', '--word', dest='words', action='store_true', default=False, help='only count words') parser.add_option('-l', '--line', dest='lines', action='store_true', default=False, help='only count lines') parser.add_option('-n', '--nototal', dest='nototal', action='store_true', default=False, help='don\'t print total information') options, args = parser.parse_args() return options, args #print options def get_count(data): chars = len(data) words = len(data.split()) lines = data.count('\n') return lines, words, chars #if not options.chars and not options.words and not options def print_wc(options, lines, words, chars, fn): if options.lines: print lines, if options.words: print words, if options.chars: print chars, print fn def main(): options, args = opt() if not (options.lines or options.words or options.chars): options.lines, options.words, options.chars = True, True, True if args: total_lines, total_words, total_chars = 0, 0, 0 for fn in args: if os.path.isfile(fn): with open(fn) as fd: data = fd.read() lines, words, chars = get_count(data) print_wc(options, lines, words, chars, fn) total_lines += lines total_words += words total_chars += chars elif os.path.isdir(fn): print >> sys.stderr, '%s is a directory' %fn else: sys.stderr.write('%s: No such file or directory\n' % fn) # 只有多个文件的时候会计算出total字段 if len(args) > 1 and not options.nototal: print_wc(options, total_lines, total_words, total_chars, 'total') else: fn = '' data = sys.stdin.read() lines, words, chars = get_count(data) print_wc(options, lines, words, chars, fn) if __name__ == '__main__': main()
The above is the detailed content of Example code for using python to implement the functions of wc command program. For more information, please follow other related articles on the PHP Chinese website!
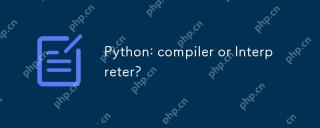
Python is an interpreted language, but it also includes the compilation process. 1) Python code is first compiled into bytecode. 2) Bytecode is interpreted and executed by Python virtual machine. 3) This hybrid mechanism makes Python both flexible and efficient, but not as fast as a fully compiled language.
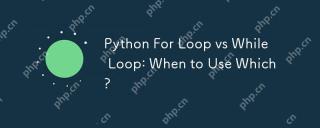
Useaforloopwheniteratingoverasequenceorforaspecificnumberoftimes;useawhileloopwhencontinuinguntilaconditionismet.Forloopsareidealforknownsequences,whilewhileloopssuitsituationswithundeterminediterations.
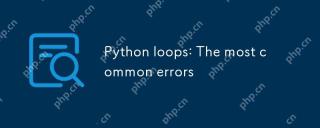
Pythonloopscanleadtoerrorslikeinfiniteloops,modifyinglistsduringiteration,off-by-oneerrors,zero-indexingissues,andnestedloopinefficiencies.Toavoidthese:1)Use'i
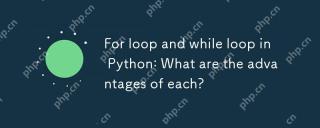
Forloopsareadvantageousforknowniterationsandsequences,offeringsimplicityandreadability;whileloopsareidealfordynamicconditionsandunknowniterations,providingcontrolovertermination.1)Forloopsareperfectforiteratingoverlists,tuples,orstrings,directlyacces
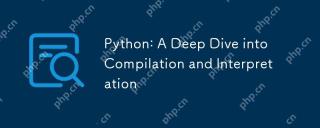
Pythonusesahybridmodelofcompilationandinterpretation:1)ThePythoninterpretercompilessourcecodeintoplatform-independentbytecode.2)ThePythonVirtualMachine(PVM)thenexecutesthisbytecode,balancingeaseofusewithperformance.
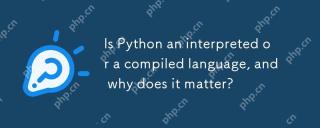
Pythonisbothinterpretedandcompiled.1)It'scompiledtobytecodeforportabilityacrossplatforms.2)Thebytecodeistheninterpreted,allowingfordynamictypingandrapiddevelopment,thoughitmaybeslowerthanfullycompiledlanguages.
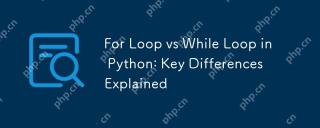
Forloopsareidealwhenyouknowthenumberofiterationsinadvance,whilewhileloopsarebetterforsituationswhereyouneedtoloopuntilaconditionismet.Forloopsaremoreefficientandreadable,suitableforiteratingoversequences,whereaswhileloopsoffermorecontrolandareusefulf
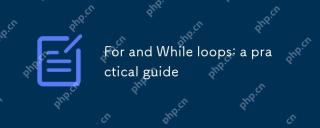
Forloopsareusedwhenthenumberofiterationsisknowninadvance,whilewhileloopsareusedwhentheiterationsdependonacondition.1)Forloopsareidealforiteratingoversequenceslikelistsorarrays.2)Whileloopsaresuitableforscenarioswheretheloopcontinuesuntilaspecificcond


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
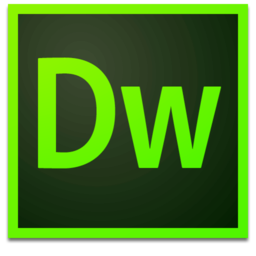
Dreamweaver Mac version
Visual web development tools
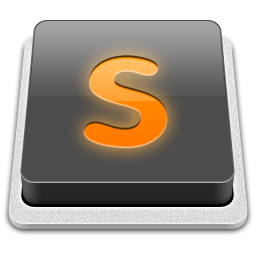
SublimeText3 Mac version
God-level code editing software (SublimeText3)
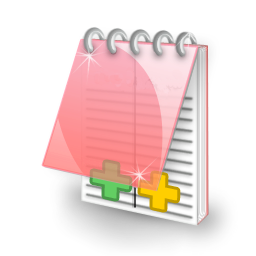
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
