Introduction
Descriptors (descriptors) are a profound but important black magic in the Python language. They are widely used in the core of the Python language. Mastering descriptors will help Python Adds an extra trick to the programmer's toolbox. In this article, I will describe the definition of descriptors and some common scenarios, and at the end of the article I will add __getattr,
__getattribute__,
__getitem__, which also involve attributes. Access the
Magic Method.
descr__get__(self, obj, objtype=None) --> value
descr.__set__(self, obj, value) --> None
descr.__delete__(self, obj) --> None
As long as an object attribute (object attribute) defines any one of the above three methods, then this class can be called Descriptor class.
RevealAcess class and implement the
__get__ method. Now this class can be called is a descriptor class.
class RevealAccess(object): def __get__(self, obj, objtype): print('self in RevealAccess: {}'.format(self)) print('self: {}\nobj: {}\nobjtype: {}'.format(self, obj, objtype)) class MyClass(object): x = RevealAccess() def test(self): print('self in MyClass: {}'.format(self))
EX1 instance attributes
Next let’s take a look at the meaning of each parameter of the__get__ method. In the following example,
self is the instance x of the RevealAccess class,
obj is the instance m of the MyClass class,
objtype as the name suggests is the MyClass class itself. As can be seen from the output statement,
m.xaccess descriptor
x will call the
__get__ method.
>>> m = MyClass() >>> m.test() self in MyClass: <__main__.MyClass object at 0x7f19d4e42160> >>> m.x self in RevealAccess: <__main__.RevealAccess object at 0x7f19d4e420f0> self: <__main__.RevealAccess object at 0x7f19d4e420f0> obj: <__main__.MyClass object at 0x7f19d4e42160> objtype: <class '__main__.MyClass'>
EX2 class attribute
If the attributex is directly accessed through the class, then
obj is directly None, which is still It's easier to understand, because there is no instance of MyClass.
>>> MyClass.x self in RevealAccess: <__main__.RevealAccess object at 0x7f53651070f0> self: <__main__.RevealAccess object at 0x7f53651070f0> obj: None objtype: <class '__main__.MyClass'>The principle of descriptorDescriptor triggerIn the above example, we enumerated the usage of descriptors from the perspective of instance attributes and class attributes. Below we Let’s carefully analyze the internal principle:
- If you access the
instance attribute
, the __getattribute__ method of the base class object is actually called. In this method Obj.d is translated into
type(obj).__dict__['d'].__get__(obj, type(obj)).
- If you access the
class attribute
, it is equivalent to calling the __getattribute__ method of the metaclass type, which translates cls.d into
cls.__dict__['d'].__get__(None, cls), here the obj of __get__() is None because there is no instance.
__getattribute__ magic method. This method will be called unconditionally when we access the attributes of an object. The detailed details are such as
__getattr I will make an additional supplement at the end of the article about the difference between ,
__getitem__, but we will not delve into it for now.
- If an object defines both __get__() and __set__ () method, this descriptor is called
data descriptor
.
- If an object only defines the __get__() method, this descriptor is called
non-data descriptor
.
- data descriptor
- instance dict
- non-data descriptor
- __getattr__()
data descriptor > instance dict > non-data descriptor > __getattr__()What does this mean? That is to say, if the
data descriptor->d and
instance attribute->d with the same name appear in the instance object obj,
obj.d will pair with the attribute
dWhen accessing, since the data descriptor has a higher priority, Python will call
type(obj).__dict__['d'].__get__(obj, type(obj)) instead of calling obj.__dict__['d']. But if the descriptor is a non-data descriptor, Python will call
obj.__dict__['d'].
property(fget=None, fset=None, fdel=None, doc=None) -> property attributefget, fset and fdel are the getter, setter and deleter methods of the class respectively. We use the following example to illustrate how to use Property:
class Account(object): def __init__(self): self._acct_num = None def get_acct_num(self): return self._acct_num def set_acct_num(self, value): self._acct_num = value def del_acct_num(self): del self._acct_num acct_num = property(get_acct_num, set_acct_num, del_acct_num, '_acct_num property.')If acct is an instance of Account, acct.acct_num will call the getter, acct.acct_num = value will call the setter, and del acct_num.acct_num will call deleter.
>>> acct = Account() >>> acct.acct_num = 1000 >>> acct.acct_num 1000Python also provides the
@property decorator, which can be used to create properties for simple application scenarios. A property object has getter, setter and delete decorator methods, which can be used to create a copy of the property through the accessor function of the corresponding decorated function.
class Account(object): def __init__(self): self._acct_num = None @property # the _acct_num property. the decorator creates a read-only property def acct_num(self): return self._acct_num @acct_num.setter # the _acct_num property setter makes the property writeable def set_acct_num(self, value): self._acct_num = value @acct_num.deleter def del_acct_num(self): del self._acct_numIf you want the property to be read-only, just remove the setter method. Create descriptors at runtimeWe can add property attributes at runtime:
class Person(object): def addProperty(self, attribute): # create local setter and getter with a particular attribute name getter = lambda self: self._getProperty(attribute) setter = lambda self, value: self._setProperty(attribute, value) # construct property attribute and add it to the class setattr(self.__class__, attribute, property(fget=getter, \ fset=setter, \ doc="Auto-generated method")) def _setProperty(self, attribute, value): print("Setting: {} = {}".format(attribute, value)) setattr(self, '_' + attribute, value.title()) def _getProperty(self, attribute): print("Getting: {}".format(attribute)) return getattr(self, '_' + attribute)
>>> user = Person() >>> user.addProperty('name') >>> user.addProperty('phone') >>> user.name = 'john smith' Setting: name = john smith >>> user.phone = '12345' Setting: phone = 12345 >>> user.name Getting: name 'John Smith' >>> user.__dict__ {'_phone': '12345', '_name': 'John Smith'}Static methods and class methodsWe can use descriptors To simulate the implementation of
@staticmethod and
@classmethod in Python. Let’s first browse the following table:
Transformation | Called from an Object | Called from a Class |
---|---|---|
function | f(obj, *args) | f(*args) |
staticmethod | f(*args) | f(*args) |
classmethod | f(type(obj), *args) | f(klass, *args) |
静态方法
对于静态方法f
。c.f
和C.f
是等价的,都是直接查询object.__getattribute__(c, ‘f’)
或者object.__getattribute__(C, ’f‘)
。静态方法一个明显的特征就是没有self
变量。
静态方法有什么用呢?假设有一个处理专门数据的容器类,它提供了一些方法来求平均数,中位数等统计数据方式,这些方法都是要依赖于相应的数据的。但是类中可能还有一些方法,并不依赖这些数据,这个时候我们可以将这些方法声明为静态方法,同时这也可以提高代码的可读性。
使用非数据描述符来模拟一下静态方法的实现:
class StaticMethod(object): def __init__(self, f): self.f = f def __get__(self, obj, objtype=None): return self.f
我们来应用一下:
class MyClass(object): @StaticMethod def get_x(x): return x print(MyClass.get_x(100)) # output: 100
类方法
Python的@classmethod
和@staticmethod
的用法有些类似,但是还是有些不同,当某些方法只需要得到类的引用
而不关心类中的相应的数据的时候就需要使用classmethod了。
使用非数据描述符来模拟一下类方法的实现:
class ClassMethod(object): def __init__(self, f): self.f = f def __get__(self, obj, klass=None): if klass is None: klass = type(obj) def newfunc(*args): return self.f(klass, *args) return newfunc
其他的魔术方法
首次接触Python魔术方法的时候,我也被__get__
, __getattribute__
, __getattr__
, __getitem__
之间的区别困扰到了,它们都是和属性访问相关的魔术方法,其中重写__getattr__
,__getitem__
来构造一个自己的集合类非常的常用,下面我们就通过一些例子来看一下它们的应用。
__getattr__
Python默认访问类/实例的某个属性都是通过__getattribute__
来调用的,__getattribute__
会被无条件调用,没有找到的话就会调用__getattr__
。如果我们要定制某个类,通常情况下我们不应该重写__getattribute__
,而是应该重写__getattr__
,很少看见重写__getattribute__
的情况。
从下面的输出可以看出,当一个属性通过__getattribute__
无法找到的时候会调用__getattr__
。
In [1]: class Test(object): ...: def __getattribute__(self, item): ...: print('call __getattribute__') ...: return super(Test, self).__getattribute__(item) ...: def __getattr__(self, item): ...: return 'call __getattr__' ...: In [2]: Test().a call __getattribute__ Out[2]: 'call __getattr__'
应用
对于默认的字典,Python只支持以obj['foo']
形式来访问,不支持obj.foo
的形式,我们可以通过重写__getattr__
让字典也支持obj['foo']
的访问形式,这是一个非常经典常用的用法:
class Storage(dict): """ A Storage object is like a dictionary except `obj.foo` can be used in addition to `obj['foo']`. """ def __getattr__(self, key): try: return self[key] except KeyError as k: raise AttributeError(k) def __setattr__(self, key, value): self[key] = value def __delattr__(self, key): try: del self[key] except KeyError as k: raise AttributeError(k) def __repr__(self): return '<Storage ' + dict.__repr__(self) + '>'
我们来使用一下我们自定义的加强版字典:
>>> s = Storage(a=1) >>> s['a'] 1 >>> s.a 1 >>> s.a = 2 >>> s['a'] 2 >>> del s.a >>> s.a ... AttributeError: 'a'
__getitem__
getitem用于通过下标[]
的形式来获取对象中的元素,下面我们通过重写__getitem__
来实现一个自己的list。
class MyList(object): def __init__(self, *args): self.numbers = args def __getitem__(self, item): return self.numbers[item] my_list = MyList(1, 2, 3, 4, 6, 5, 3) print my_list[2]
这个实现非常的简陋,不支持slice和step等功能,请读者自行改进,这里我就不重复了。
应用
下面是参考requests库中对于__getitem__
的一个使用,我们定制了一个忽略属性大小写的字典类。
程序有些复杂,我稍微解释一下:由于这里比较简单,没有使用描述符的需求,所以使用了@property
装饰器来代替,lower_keys
的功能是将实例字典
中的键全部转换成小写并且存储在字典self._lower_keys
中。重写了__getitem__
方法,以后我们访问某个属性首先会将键转换为小写的方式,然后并不会直接访问实例字典,而是会访问字典self._lower_keys
去查找。赋值/删除操作的时候由于实例字典会进行变更,为了保持self._lower_keys
和实例字典同步,首先清除self._lower_keys
的内容,以后我们重新查找键的时候再调用__getitem__
的时候会重新新建一个self._lower_keys
。
class CaseInsensitiveDict(dict): @property def lower_keys(self): if not hasattr(self, '_lower_keys') or not self._lower_keys: self._lower_keys = dict((k.lower(), k) for k in self.keys()) return self._lower_keys def _clear_lower_keys(self): if hasattr(self, '_lower_keys'): self._lower_keys.clear() def __contains__(self, key): return key.lower() in self.lower_keys def __getitem__(self, key): if key in self: return dict.__getitem__(self, self.lower_keys[key.lower()]) def __setitem__(self, key, value): dict.__setitem__(self, key, value) self._clear_lower_keys() def __delitem__(self, key): dict.__delitem__(self, key) self._lower_keys.clear() def get(self, key, default=None): if key in self: return self[key] else: return default
我们来调用一下这个类:
>>> d = CaseInsensitiveDict() >>> d['ziwenxie'] = 'ziwenxie' >>> d['ZiWenXie'] = 'ZiWenXie' >>> print(d) {'ZiWenXie': 'ziwenxie', 'ziwenxie': 'ziwenxie'} >>> print(d['ziwenxie']) ziwenxie # d['ZiWenXie'] => d['ziwenxie'] >>> print(d['ZiWenXie']) ziwenxie
The above is the detailed content of Magic descriptors in Python. For more information, please follow other related articles on the PHP Chinese website!
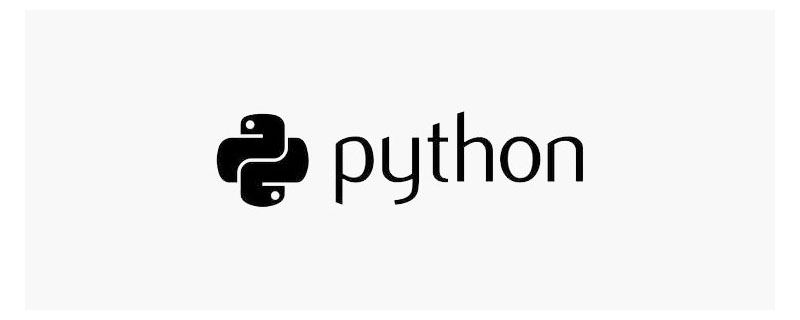
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于Seaborn的相关问题,包括了数据可视化处理的散点图、折线图、条形图等等内容,下面一起来看一下,希望对大家有帮助。
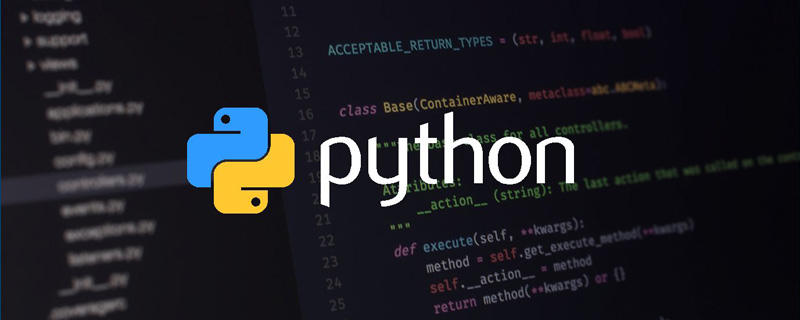
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于进程池与进程锁的相关问题,包括进程池的创建模块,进程池函数等等内容,下面一起来看一下,希望对大家有帮助。
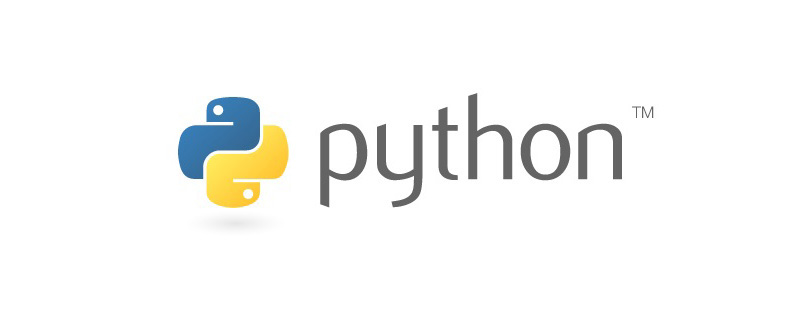
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于简历筛选的相关问题,包括了定义 ReadDoc 类用以读取 word 文件以及定义 search_word 函数用以筛选的相关内容,下面一起来看一下,希望对大家有帮助。
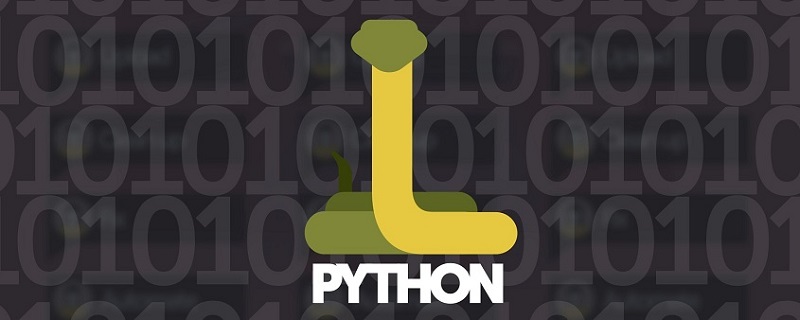
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于数据类型之字符串、数字的相关问题,下面一起来看一下,希望对大家有帮助。
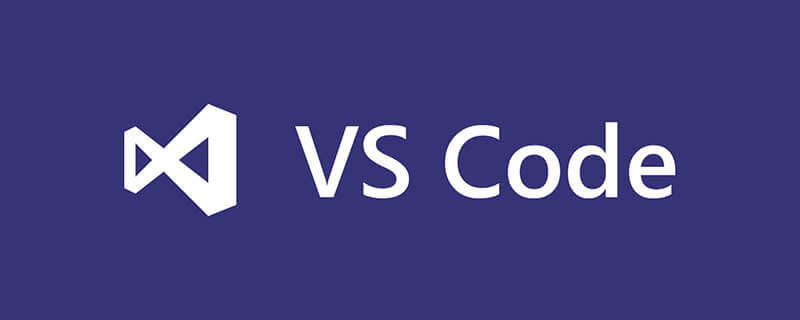
VS Code的确是一款非常热门、有强大用户基础的一款开发工具。本文给大家介绍一下10款高效、好用的插件,能够让原本单薄的VS Code如虎添翼,开发效率顿时提升到一个新的阶段。
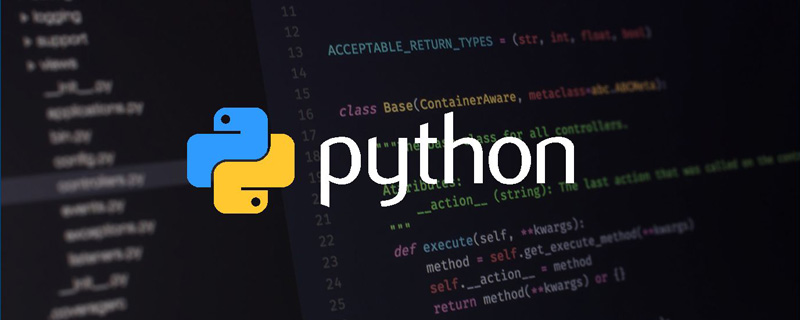
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于numpy模块的相关问题,Numpy是Numerical Python extensions的缩写,字面意思是Python数值计算扩展,下面一起来看一下,希望对大家有帮助。
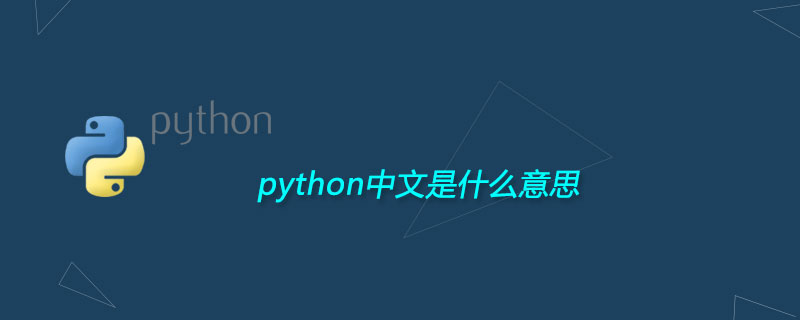
pythn的中文意思是巨蟒、蟒蛇。1989年圣诞节期间,Guido van Rossum在家闲的没事干,为了跟朋友庆祝圣诞节,决定发明一种全新的脚本语言。他很喜欢一个肥皂剧叫Monty Python,所以便把这门语言叫做python。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Atom editor mac version download
The most popular open source editor
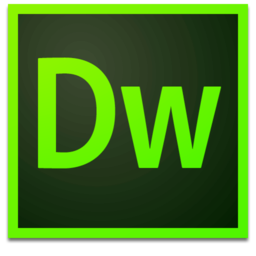
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 English version
Recommended: Win version, supports code prompts!
