


XML file is a commonly used file format. This article mainly introduces three implementation methods for C# to read XML, mainly XmlDocument, XmlTextReader, and Linq to Xml. Those who are interested can learn more.
Preface
XML file is a commonly used file format, such as app.config in WinForm and web.config in Web programs It is found in documents and many important places. (Similar to Json) Microsoft also provides a series of class libraries to help us store XML files in applications.
There are generally two models for accessing and operating XML files in programs:
DOM (Document Object Model):The benefits of using DOM are that it allows editing and updating of XML documents, random access to data in the document, and XPath querying, but The disadvantage of DOM is that it needs to load the entire document into memory at once, which can cause resource problems for large documents.
Stream model:The stream model solves this problem very well, because it uses the concept of stream to access XML files, that is to say, only the current node, but it also has its shortcomings, it is read-only, forward-only, and cannot perform backward navigation operations in the document.
The three methods of reading XML files in C# are as follows:
1. Use XmlDocument (DOM mode)
2 .Use XmlTextReader (stream mode)
3.Use Linq to Xml (Linq mode)
Use XmlDocument mode to read
Using XmlDocument is a way to read XML files based on the document structure model. In an XML file, we can think of XML as consisting of a document declaration (Declare), an element (Element), and an attribute (Attribute). , a tree composed of text (Text), etc. The first node is called the root node, and each node can have its own child nodes. After getting a node, it can be obtained through a series of attributes or methods The value of this node or some other attributes. For example:
xn 代表一个结点 xn.Name;//这个结点的名称 xn.Value;//这个结点的值 xn.ChildNodes;//这个结点的所有子结点 xn.ParentNode;//这个结点的父结点
Read all data
When using it, first declare an XmlDocument object, and then call the Load method to load the XML file from the specified path.
BookModel is a book model
#region XmlDocument读取 public static void XmlDocumentReadDemo() { //list List<BookModel> bookModeList = new List<BookModel>(); //使用的时候,首先声明一个XmlDocument对象,然后调用Load方法,从指定的路径加载XML文件. XmlDocument doc = new XmlDocument(); XmlReaderSettings settings = new XmlReaderSettings(); settings.IgnoreComments = true;//忽略文档里面的注释 using (XmlReader reader = XmlReader.Create(@"d:/demo.xml", settings)) { doc.Load(reader); //doc.Load(@"d:/demo.xml"); //然后可以通过调用SelectSingleNode得到指定的结点,通过GetAttribute得到具体的属性值.参看下面的代码 // 得到根节点bookstore XmlNode xn = doc.SelectSingleNode("bookstore"); // 得到根节点的所有子节点 XmlNodeList xnl = xn.ChildNodes; foreach (XmlNode xn1 in xnl) { BookModel bookModel = new BookModel(); // 将节点转换为元素,便于得到节点的属性值 XmlElement xe = (XmlElement)xn1; // 得到Type和ISBN两个属性的属性值 bookModel.BookISBN = xe.GetAttribute("ISBN").ToString(); bookModel.BookType = xe.GetAttribute("Type").ToString(); // 得到Book节点的所有子节点 XmlNodeList xnl0 = xe.ChildNodes; bookModel.BookName = xnl0.Item(0).InnerText; bookModel.BookAuthor = xnl0.Item(1).InnerText; bookModel.BookPrice = Convert.ToDouble(xnl0.Item(2).InnerText); bookModeList.Add(bookModel); } } bookModeList.Add(new BookModel()); } #endregion XmlDocument读取
The running results are as follows:
Use XmlTextReader to read
When using XmlTextReader to read data, first create a stream, and then use the read() method to continuously read downwards, and perform corresponding operations according to the type of node read. As follows:
#region XmlTextReaderDemo public static void XmlTextReaderDemo() { XmlTextReader reader = new XmlTextReader(@"d:/demo.xml"); List<BookModel> modelList = new List<BookModel>(); BookModel model = new BookModel(); while (reader.Read()) { if (reader.NodeType == XmlNodeType.Element) { if (reader.Name == "book") { model.BookType = reader.GetAttribute("Type"); model.BookISBN = reader.GetAttribute("ISBN"); } if (reader.Name == "title") { model.BookName = reader.ReadElementContentAsString(); } if (reader.Name == "author") { model.BookAuthor = reader.ReadElementString().Trim(); } if (reader.Name == "price") { model.BookPrice = Convert.ToDouble(reader.ReadElementString().Trim()); } //for(int i=0;i<reader.AttributeCount;i++) //{ // reader.MoveToAttribute(i); //} } if (reader.NodeType == XmlNodeType.EndElement) { modelList.Add(model); model = new BookModel(); } } reader.Close(); modelList.Add(new BookModel()); } #endregion XmlTextReaderDemo
Using Linq to Xml to read
Linq is a new feature that appeared in C# 3.0 , using it can easily operate many data sources, including XML files. Using Linq to operate XML files is very convenient and relatively simple.
Must reference using System.Linq;using System.Xml.Linq;
##
#region 读取所有的数据 XElement xe = XElement.Load(@"d:/demoLinq.xml"); //xe.Descendants var elements = from ele in xe.Elements() select ele; List<BookModel> modelList = new List<BookModel>(); foreach (var ele in elements) { BookModel model = new BookModel(); model.BookAuthor = ele.Element("author").Value; model.BookName = ele.Element("title").Value; model.BookPrice = Convert.ToDouble(ele.Element("price").Value); model.BookISBN = ele.Attribute("ISBN").Value; model.BookType = ele.Attribute("Type").Value; modelList.Add(model); } modelList.Add(new BookModel()); #endregion 读取所有的数据
Summary
1.The advantage of the XmlDocument method is that it is easy to find2.The XmlTextReader method is a stream-reading method that uses less memory temporarily3.Linq to The latest method of Xml is also the recommended method. The code is small and easy to understand. The above is the detailed code explanation of the three implementation methods of C# reading XML. For more related content, please pay attention to the PHP Chinese website (www.php.cn) !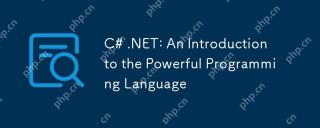
The combination of C# and .NET provides developers with a powerful programming environment. 1) C# supports polymorphism and asynchronous programming, 2) .NET provides cross-platform capabilities and concurrent processing mechanisms, which makes them widely used in desktop, web and mobile application development.
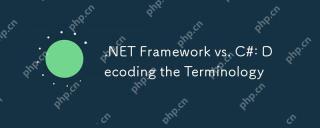
.NETFramework is a software framework, and C# is a programming language. 1..NETFramework provides libraries and services, supporting desktop, web and mobile application development. 2.C# is designed for .NETFramework and supports modern programming functions. 3..NETFramework manages code execution through CLR, and the C# code is compiled into IL and runs by CLR. 4. Use .NETFramework to quickly develop applications, and C# provides advanced functions such as LINQ. 5. Common errors include type conversion and asynchronous programming deadlocks. VisualStudio tools are required for debugging.
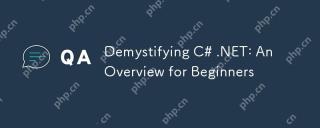
C# is a modern, object-oriented programming language developed by Microsoft, and .NET is a development framework provided by Microsoft. C# combines the performance of C and the simplicity of Java, and is suitable for building various applications. The .NET framework supports multiple languages, provides garbage collection mechanisms, and simplifies memory management.
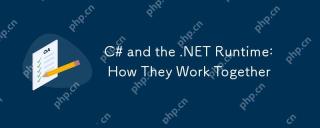
C# and .NET runtime work closely together to empower developers to efficient, powerful and cross-platform development capabilities. 1) C# is a type-safe and object-oriented programming language designed to integrate seamlessly with the .NET framework. 2) The .NET runtime manages the execution of C# code, provides garbage collection, type safety and other services, and ensures efficient and cross-platform operation.
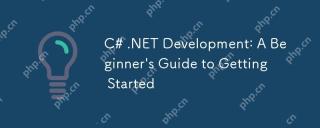
To start C#.NET development, you need to: 1. Understand the basic knowledge of C# and the core concepts of the .NET framework; 2. Master the basic concepts of variables, data types, control structures, functions and classes; 3. Learn advanced features of C#, such as LINQ and asynchronous programming; 4. Be familiar with debugging techniques and performance optimization methods for common errors. With these steps, you can gradually penetrate the world of C#.NET and write efficient applications.
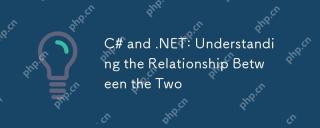
The relationship between C# and .NET is inseparable, but they are not the same thing. C# is a programming language, while .NET is a development platform. C# is used to write code, compile into .NET's intermediate language (IL), and executed by the .NET runtime (CLR).
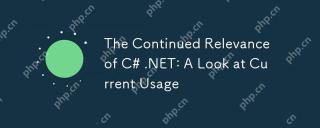
C#.NET is still important because it provides powerful tools and libraries that support multiple application development. 1) C# combines .NET framework to make development efficient and convenient. 2) C#'s type safety and garbage collection mechanism enhance its advantages. 3) .NET provides a cross-platform running environment and rich APIs, improving development flexibility.
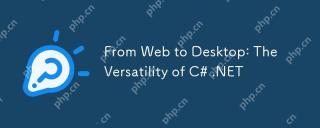
C#.NETisversatileforbothwebanddesktopdevelopment.1)Forweb,useASP.NETfordynamicapplications.2)Fordesktop,employWindowsFormsorWPFforrichinterfaces.3)UseXamarinforcross-platformdevelopment,enablingcodesharingacrossWindows,macOS,Linux,andmobiledevices.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
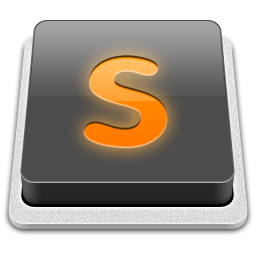
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor