Python Basic Tutorial Usage of Packages and Classes
Create a folder filePackage
Create __init__ in the filePackage folder. py
With __init__.py, filePackage is considered a package, otherwise it is just an ordinary folder.
Create file.py in the filePackage folder
file.py code is as follows:
#!/usr/bin/env python3 # -*- coding: utf-8 -*- from datetime import datetime class MyFile(): def __init__(self, filepath): print('MyFile init...') self.filepath = filepath def printFilePath(self): print(self.filepath) def testReadFile(self): with open(self.filepath, 'r') as f: s = f.read() print('open for read...') print(s) def testWriteFile(self): with open('test.txt', 'w') as f: f.write('今天是 ') f.write(datetime.now().strftime('%Y-%m-%d'))
__init__ The .py code is as follows:
from file import MyFile
Expose the public class methods in this module
and then outside There is no need to find the specific actual location of the reference, just find the __init__ of the package.
Create main.py and filePackage level,
main.py code is as follows:
#!/usr/bin/env python3 # -*- coding: utf-8 -*- from filePackage import MyFile if __name__ == '__main__': a = MyFile("./filePackage/test.txt") a.printFilePath(); a.testReadFile();
Directory structure:
If nothing is written in __init__.py, then in You can also write like this in main.py:
import filePackage.file if __name__ == '__main__': a = filePackage.file.MyFile("./filePackage/test.txt") a.printFilePath();
But it is not recommended to write like this. It is recommended to expose the public classes in the module according to the above method and quote them directly. .
Thank you for reading, I hope it can help you, thank you for your support of this site!
For more articles related to the usage of Python packages and classes, please pay attention to the PHP Chinese website!
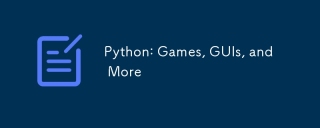
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
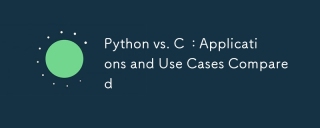
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
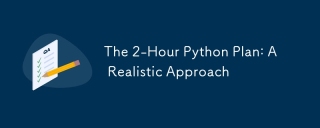
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
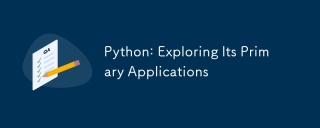
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
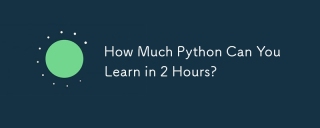
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
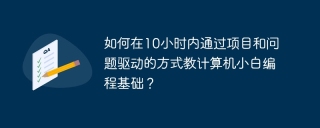
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
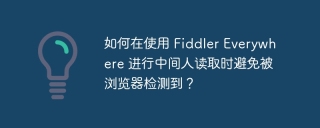
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
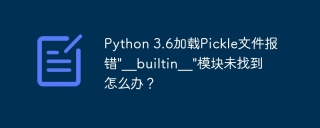
Error loading Pickle file in Python 3.6 environment: ModuleNotFoundError:Nomodulenamed...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
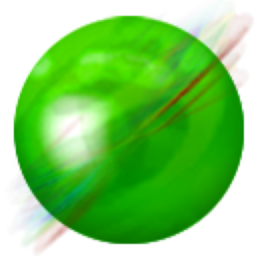
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Chinese version
Chinese version, very easy to use
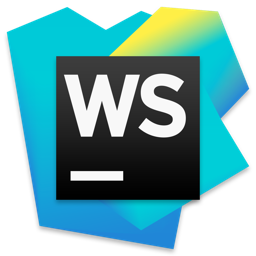
WebStorm Mac version
Useful JavaScript development tools
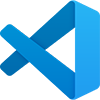
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft