QR code is a barcode image that uses black and white flat geometric figures to record text, pictures, URLs and other information through corresponding encoding algorithms. As shown below
Characteristics of QR code:
1. High-density coding, large information capacity
It can accommodate up to 1850 uppercase letters or 2710 numbers or 1108 bytes, or more than 500 Chinese characters, which is about dozens of times higher than the information capacity of ordinary barcodes.
2. Wide encoding range
This barcode can encode pictures, sounds, text, signatures, fingerprints and other digitized information and express it with barcodes; it can Represents text in multiple languages; can represent image data.
3. Strong fault tolerance and error correction function
This allows the 2D barcode to be read correctly even if it is partially damaged due to perforations, stains, etc. , information can still be recovered if 50% of the damaged area is damaged.
4. High decoding reliability
It is much lower than ordinary barcode decoding error rate of 2 parts per million, and the bit error rate does not exceed 10 million one part.
5. Encryption measures can be introduced
Good confidentiality and anti-counterfeiting properties.
6. Low cost, easy to make, durable
Because of the above characteristics, QR codes are becoming more and more popular and their applications are becoming more and more widespread ( For detailed information, please see Baidu Encyclopedia (the introduction is not the focus of this article), so knowing how to develop QR codes is a very good knowledge reserve, so this article will explain how to generate and parse QR codes.
1. Java
Required jar package: QRCode.jar
http://sourceforge.jp/projects/qrcode/
TwoDimensionCode class: QR code operation core class
package qrcode; import java.awt.Color; import java.awt.Graphics2D; import java.awt.image.BufferedImage; import java.io.File; import java.io.IOException; import java.io.InputStream; import java.io.OutputStream; import javax.imageio.ImageIO; import jp.sourceforge.qrcode.QRCodeDecoder; import jp.sourceforge.qrcode.exception.DecodingFailedException; import com.swetake.util.Qrcode; public class TwoDimensionCode { /** * 生成二维码(QRCode)图片 * @param content 存储内容 * @param imgPath 图片路径 */ public void encoderQRCode(String content, String imgPath) { this.encoderQRCode(content, imgPath, "png", 7); } /** * 生成二维码(QRCode)图片 * @param content 存储内容 * @param output 输出流 */ public void encoderQRCode(String content, OutputStream output) { this.encoderQRCode(content, output, "png", 7); } /** * 生成二维码(QRCode)图片 * @param content 存储内容 * @param imgPath 图片路径 * @param imgType 图片类型 */ public void encoderQRCode(String content, String imgPath, String imgType) { this.encoderQRCode(content, imgPath, imgType, 7); } /** * 生成二维码(QRCode)图片 * @param content 存储内容 * @param output 输出流 * @param imgType 图片类型 */ public void encoderQRCode(String content, OutputStream output, String imgType) { this.encoderQRCode(content, output, imgType, 7); } /** * 生成二维码(QRCode)图片 * @param content 存储内容 * @param imgPath 图片路径 * @param imgType 图片类型 * @param size 二维码尺寸 */ public void encoderQRCode(String content, String imgPath, String imgType, int size) { try { BufferedImage bufImg = this.qRCodeCommon(content, imgType, size); File imgFile = new File(imgPath); // 生成二维码QRCode图片 ImageIO.write(bufImg, imgType, imgFile); } catch (Exception e) { e.printStackTrace(); } } /** * 生成二维码(QRCode)图片 * @param content 存储内容 * @param output 输出流 * @param imgType 图片类型 * @param size 二维码尺寸 */ public void encoderQRCode(String content, OutputStream output, String imgType, int size) { try { BufferedImage bufImg = this.qRCodeCommon(content, imgType, size); // 生成二维码QRCode图片 ImageIO.write(bufImg, imgType, output); } catch (Exception e) { e.printStackTrace(); } } /** * 生成二维码(QRCode)图片的公共方法 * @param content 存储内容 * @param imgType 图片类型 * @param size 二维码尺寸 * @return */ private BufferedImage qRCodeCommon(String content, String imgType, int size) { BufferedImage bufImg = null; try { Qrcode qrcodeHandler = new Qrcode(); // 设置二维码排错率,可选L(7%)、M(15%)、Q(25%)、H(30%),排错率越高可存储的信息越少,但对二维码清晰度的要求越小 qrcodeHandler.setQrcodeErrorCorrect('M'); qrcodeHandler.setQrcodeEncodeMode('B'); // 设置设置二维码尺寸,取值范围1-40,值越大尺寸越大,可存储的信息越大 qrcodeHandler.setQrcodeVersion(size); // 获得内容的字节数组,设置编码格式 byte[] contentBytes = content.getBytes("utf-8"); // 图片尺寸 int imgSize = 67 + 12 * (size - 1); bufImg = new BufferedImage(imgSize, imgSize, BufferedImage.TYPE_INT_RGB); Graphics2D gs = bufImg.createGraphics(); // 设置背景颜色 gs.setBackground(Color.WHITE); gs.clearRect(0, 0, imgSize, imgSize); // 设定图像颜色> BLACK gs.setColor(Color.BLACK); // 设置偏移量,不设置可能导致解析出错 int pixoff = 2; // 输出内容> 二维码 if (contentBytes.length > 0 && contentBytes.length < 800) { boolean[][] codeOut = qrcodeHandler.calQrcode(contentBytes); for (int i = 0; i < codeOut.length; i++) { for (int j = 0; j < codeOut.length; j++) { if (codeOut[j][i]) { gs.fillRect(j * 3 + pixoff, i * 3 + pixoff, 3, 3); } } } } else { throw new Exception("QRCode content bytes length = " + contentBytes.length + " not in [0, 800]."); } gs.dispose(); bufImg.flush(); } catch (Exception e) { e.printStackTrace(); } return bufImg; } /** * 解析二维码(QRCode) * @param imgPath 图片路径 * @return */ public String decoderQRCode(String imgPath) { // QRCode 二维码图片的文件 File imageFile = new File(imgPath); BufferedImage bufImg = null; String content = null; try { bufImg = ImageIO.read(imageFile); QRCodeDecoder decoder = new QRCodeDecoder(); content = new String(decoder.decode(new TwoDimensionCodeImage(bufImg)), "utf-8"); } catch (IOException e) { System.out.println("Error: " + e.getMessage()); e.printStackTrace(); } catch (DecodingFailedException dfe) { System.out.println("Error: " + dfe.getMessage()); dfe.printStackTrace(); } return content; } /** * 解析二维码(QRCode) * @param input 输入流 * @return */ public String decoderQRCode(InputStream input) { BufferedImage bufImg = null; String content = null; try { bufImg = ImageIO.read(input); QRCodeDecoder decoder = new QRCodeDecoder(); content = new String(decoder.decode(new TwoDimensionCodeImage(bufImg)), "utf-8"); } catch (IOException e) { System.out.println("Error: " + e.getMessage()); e.printStackTrace(); } catch (DecodingFailedException dfe) { System.out.println("Error: " + dfe.getMessage()); dfe.printStackTrace(); } return content; } public static void main(String[] args) { String imgPath = "G:/TDDOWNLOAD/Michael_QRCode.png"; String encoderContent = "Hello 大大、小小,welcome to QRCode!" + "\nMyblog [ http://sjsky.iteye.com ]" + "\nEMail [ sjsky007@gmail.com ]"; TwoDimensionCode handler = new TwoDimensionCode(); handler.encoderQRCode(encoderContent, imgPath, "png"); // try { // OutputStream output = new FileOutputStream(imgPath); // handler.encoderQRCode(content, output); // } catch (Exception e) { // e.printStackTrace(); // } System.out.println("========encoder success"); String decoderContent = handler.decoderQRCode(imgPath); System.out.println("解析结果如下:"); System.out.println(decoderContent); System.out.println("========decoder success!!!"); } }
TwoDimensionCodeImage class: QR code image object
For more java QR code generation and parsing sample code related articles, please pay attention to PHP Chinese website!
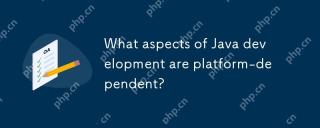
Javadevelopmentisnotentirelyplatform-independentduetoseveralfactors.1)JVMvariationsaffectperformanceandbehavioracrossdifferentOS.2)NativelibrariesviaJNIintroduceplatform-specificissues.3)Filepathsandsystempropertiesdifferbetweenplatforms.4)GUIapplica
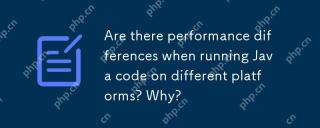
Java code will have performance differences when running on different platforms. 1) The implementation and optimization strategies of JVM are different, such as OracleJDK and OpenJDK. 2) The characteristics of the operating system, such as memory management and thread scheduling, will also affect performance. 3) Performance can be improved by selecting the appropriate JVM, adjusting JVM parameters and code optimization.
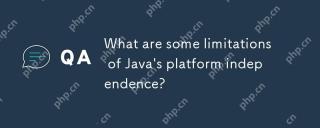
Java'splatformindependencehaslimitationsincludingperformanceoverhead,versioncompatibilityissues,challengeswithnativelibraryintegration,platform-specificfeatures,andJVMinstallation/maintenance.Thesefactorscomplicatethe"writeonce,runanywhere"
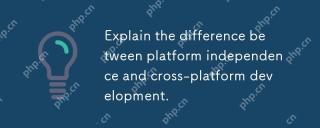
Platformindependenceallowsprogramstorunonanyplatformwithoutmodification,whilecross-platformdevelopmentrequiressomeplatform-specificadjustments.Platformindependence,exemplifiedbyJava,enablesuniversalexecutionbutmaycompromiseperformance.Cross-platformd
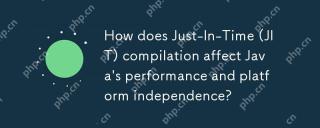
JITcompilationinJavaenhancesperformancewhilemaintainingplatformindependence.1)Itdynamicallytranslatesbytecodeintonativemachinecodeatruntime,optimizingfrequentlyusedcode.2)TheJVMremainsplatform-independent,allowingthesameJavaapplicationtorunondifferen
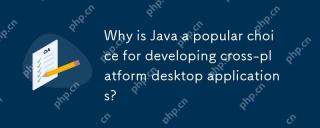
Javaispopularforcross-platformdesktopapplicationsduetoits"WriteOnce,RunAnywhere"philosophy.1)ItusesbytecodethatrunsonanyJVM-equippedplatform.2)LibrarieslikeSwingandJavaFXhelpcreatenative-lookingUIs.3)Itsextensivestandardlibrarysupportscompr
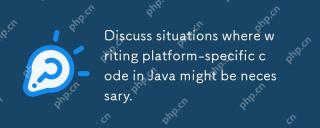
Reasons for writing platform-specific code in Java include access to specific operating system features, interacting with specific hardware, and optimizing performance. 1) Use JNA or JNI to access the Windows registry; 2) Interact with Linux-specific hardware drivers through JNI; 3) Use Metal to optimize gaming performance on macOS through JNI. Nevertheless, writing platform-specific code can affect the portability of the code, increase complexity, and potentially pose performance overhead and security risks.
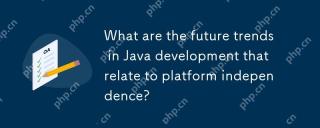
Java will further enhance platform independence through cloud-native applications, multi-platform deployment and cross-language interoperability. 1) Cloud native applications will use GraalVM and Quarkus to increase startup speed. 2) Java will be extended to embedded devices, mobile devices and quantum computers. 3) Through GraalVM, Java will seamlessly integrate with languages such as Python and JavaScript to enhance cross-language interoperability.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
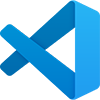
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
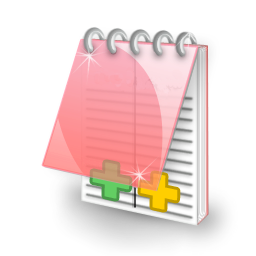
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
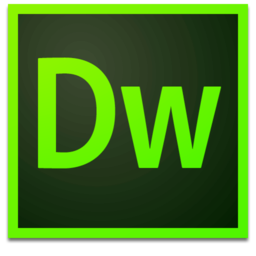
Dreamweaver Mac version
Visual web development tools
