This article will introduce some knowledge related to custom functions and arrays in PHP development. PHP developers can pay attention to it.
1. Custom function
A custom function is a function that we define ourselves. The format of a custom function in PHP is as follows:
function funname(arg1, arg2, arg3......){ //TODO return values; } <?php function fun($m, $n){ if($m==0 || $n==0){ return 0; }else{ $a=$m*$n; return $a; } } $p=2; $h=3; echo $p."*".$h."=".fun($p,$h); ?>
Output result: 2*3=6
Here is another variable Parameter function
<?php /* function fun($m, $n){ if($m==0 || $n==0){ return 0; }else{ $a=$m*$n; return $a; } } $p=2; $h=3; echo $p."*".$h."=".fun($p,$h); */ function fun($m, $n=1, $x=2){ $a=$m*$n*$x; return $a; } $p=2; echo fun($p)."<br>"; // 2*1*2 = 4 echo fun($p, 3)."<br>"; // 2*3*2 = 12 echo fun($p, 3, 3)."<br>"; // 2*3*3 = 18 ?>
Output result:
4
12
18
Let’s take a look at custom function reference passing
<?php /* function fun($m, $n){ if($m==0 || $n==0){ return 0; }else{ $a=$m*$n; return $a; } } $p=2; $h=3; echo $p."*".$h."=".fun($p,$h); */ /* function fun($m, $n=1, $x=2){ $a=$m*$n*$x; return $a; } $p=2; echo fun($p)."<br>"; // 2*1*2 = 4 echo fun($p, 3)."<br>"; // 2*3*2 = 12 echo fun($p, 3, 3)."<br>"; // 2*3*3 = 18 */ function fun(&$n){ $n=$n*$n; } $p=2; fun($p); echo $p; ?>
Output result: 4
2. Array definition assignment
1. Array Basic writing format
Simple form: array(value 1, value 2, value 3, …….)
array(“aa”, 12, true, 2.2, “test”, 50); //By array subscript Get data
Full form: array(key 1=>value 1, key 2=>value 2, …)
array(“title”=>”aa”, “age”=>20) ; //Data can only be obtained through key names
2. Ways to create arrays
//First method
$arr1=array(11, 22, 33, "44");
// The second type
$arr2=array('a'=>'11', 'b'=>'22');
//The third type
$arr3[0]='20';
$arr3[1]='30';
3. Array operations
1. Modify
$arr=array(11, 22, 33, 44); $arr[0]=55; //数组变为$arr=array(55, 22, 33, 44);
2. Delete
$arr=array(11, 22, 33, 44); unset($arr[0]); //数组变为$arr=array(22, 33, 44);
3. Use
$arr=array(11, 22, 33, 44); echo $arr[0]; $arr=array('a'=>11, 'b'=>22, 'c'=>33, 'd'=>44); echo $arr['b']];
4. Traverse
$arr=array('a'=>11, 'b'=>22, 'c'=>33, 'd'=>44); foreach($arr as $value){ //无键名 echo $value."<br>"; } foreach($arr as $id=>$value){ //输出键和值 echo $id."__".$value."<br>"; }
4. Two-dimensional array
$arr=array(array("1","11","111"), array("2","22","222")); echo $arr[1][2];
5. Array function
(1) array_change_key_case(array, case)
array: required, array.
case: Optional, CASE_LOWER (default value, lowercase letters return the keys of the array), CASE_UPPER (uppercase letters return the keys of the array)
Function: Convert all KEYs of the array to uppercase or lowercase.
2.
$a=array("a"=>"Cat","b"=>"Dog","c"=>"Horse");
3.
print_r(array_change_key_case($a,CASE_UPPER));
4.
?>
5.
Result: Array ( [A] => Cat [B] => Dog [C] => Horse )
(2) array_chunk(array, size, preserve_key)
array: required.
size: required, specifies how many elements each new array contains.
preserve_key: optional, true (preserve key name), false (new index)
Function: Divide an array into new array blocks.
<?php //array_chunk(array,size,preserve_key) $a1=array("a"=>"Cat","b"=>"Dog","c"=>"Horse","d"=>"Cow"); print_r(array_chunk($a1,2)); $a2=array("a"=>"Cat","b"=>"Dog","c"=>"Horse","d"=>"Cow"); print_r(array_chunk($a2,2,true)); ?>
Result:
Array ( [0] => Array ( [0] => Cat [1] => Dog ) [1] => Array ( [0] => Horse [1 ] => Cow ) )
Array ( [0] => Array ( [a] => Cat [b] => Dog ) [1] => Array ( [c] => Horse [d] => Cow ) )
…….
There are many functions like this, you can check them when you use them.
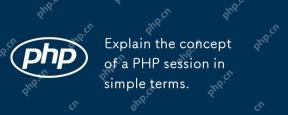
PHPsessionstrackuserdataacrossmultiplepagerequestsusingauniqueIDstoredinacookie.Here'showtomanagethemeffectively:1)Startasessionwithsession_start()andstoredatain$_SESSION.2)RegeneratethesessionIDafterloginwithsession_regenerate_id(true)topreventsessi
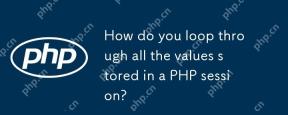
In PHP, iterating through session data can be achieved through the following steps: 1. Start the session using session_start(). 2. Iterate through foreach loop through all key-value pairs in the $_SESSION array. 3. When processing complex data structures, use is_array() or is_object() functions and use print_r() to output detailed information. 4. When optimizing traversal, paging can be used to avoid processing large amounts of data at one time. This will help you manage and use PHP session data more efficiently in your actual project.
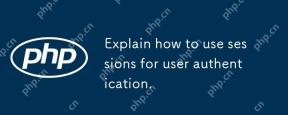
The session realizes user authentication through the server-side state management mechanism. 1) Session creation and generation of unique IDs, 2) IDs are passed through cookies, 3) Server stores and accesses session data through IDs, 4) User authentication and status management are realized, improving application security and user experience.
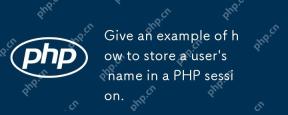
Tostoreauser'snameinaPHPsession,startthesessionwithsession_start(),thenassignthenameto$_SESSION['username'].1)Usesession_start()toinitializethesession.2)Assigntheuser'snameto$_SESSION['username'].Thisallowsyoutoaccessthenameacrossmultiplepages,enhanc
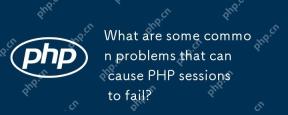
Reasons for PHPSession failure include configuration errors, cookie issues, and session expiration. 1. Configuration error: Check and set the correct session.save_path. 2.Cookie problem: Make sure the cookie is set correctly. 3.Session expires: Adjust session.gc_maxlifetime value to extend session time.
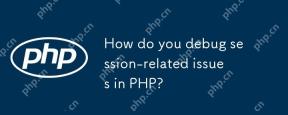
Methods to debug session problems in PHP include: 1. Check whether the session is started correctly; 2. Verify the delivery of the session ID; 3. Check the storage and reading of session data; 4. Check the server configuration. By outputting session ID and data, viewing session file content, etc., you can effectively diagnose and solve session-related problems.
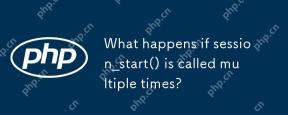
Multiple calls to session_start() will result in warning messages and possible data overwrites. 1) PHP will issue a warning, prompting that the session has been started. 2) It may cause unexpected overwriting of session data. 3) Use session_status() to check the session status to avoid repeated calls.
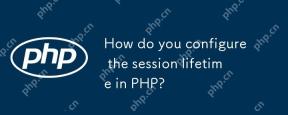
Configuring the session lifecycle in PHP can be achieved by setting session.gc_maxlifetime and session.cookie_lifetime. 1) session.gc_maxlifetime controls the survival time of server-side session data, 2) session.cookie_lifetime controls the life cycle of client cookies. When set to 0, the cookie expires when the browser is closed.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
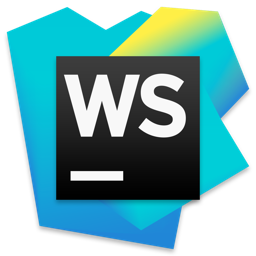
WebStorm Mac version
Useful JavaScript development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
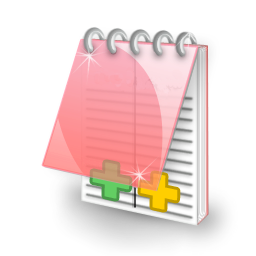
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 English version
Recommended: Win version, supports code prompts!
