Python's set is similar to other languages. It is an unordered set of non-repeating elements. Its basic functions include relationship testing and elimination of duplicate elements. Set objects also support union, intersection, difference and sysmmetric difference( Symmetric difference set) and other mathematical operations.
sets supports x in set, len(set), and for x in set. As an unordered collection, sets do not record element positions or insertion points. Therefore, sets do not support indexing, slicing, or other sequence-like operations.
Let’s give a simple example below.
>>> x = set('spam')
>>> y = set(['h','a','m'])
>>> x, y
(set([ 'a', 'p', 's', 'm']), set(['a', 'h', 'm']))
Here are some small applications.
>>> x & y # Intersection
set(['a', 'm'])
>>> x | y # Union
set(['a', 'p', 's ', 'h', 'm'])
>>> x - y # Difference set
set(['p', 's'])
I remember a netizen asked how to remove duplicate elements from a massive list , it's okay to use hash to solve it, but it doesn't feel very high in terms of performance. It's still very good to use set to solve it. The example is as follows:
>>> a = [11,22,33,44,11,22]
>>> b = set(a)
>>> b
set([33, 11, 44, 22])
>>> c = [i for i in b]
>>> c
[33, 11, 44, 22]
It’s very cool and can be done in just a few lines.
1.8 Sets
Collections are used to contain a group of unordered objects. To create a set, use the set() function and provide a series of items like this:
s = set([3,5,9,10]) #Create a numeric set
t = set("Hello" ) #Create a collection of unique characters
Unlike lists and tuples, collections are unordered and cannot be indexed numerically. Additionally, elements in the collection cannot be repeated. For example, if you check the value of the t set in the previous code, the result will be:
>>> t
set(['H', 'e', 'l', 'o'])
Note that only an 'l'.
Sets support a series of standard operations, including union, intersection, difference and symmetric difference, for example:
a = t | s # The union of t and s
b = t & s # The union of t and s Intersection
c = t – s # Find the difference set (the term is in t, but not in s)
d = t ^ s # Symmetrical difference set (the term is in t or s, but not both at the same time) Medium)
Basic operations:
t.add('x') . ) can delete one item:
t.remove('H')
len(s)
set length
x in s
test whether x is a member of s
x not in s
test Whether x is not a member of s
s.issubset(t)
s
Test whether every element in s is in t
s.issuperset(t)
s >= t
Tests whether every element in t is in s
s.union(t)
s | t
Returns a new set containing every element in s and t
s.intersection(t)
s & t
Returns a new set containing common elements in s and t
s.difference(t)
s - t
Returns a new set containing elements that are in s but not in t
s.symmetric_difference(t)
s ^ t
Returns a new set containing unique elements in s and t
s.copy()
Returns a shallow copy of set "s"
Please note : The non-operator (non-operator (that is, of the form s.union()) versions of union(), intersection(), difference() and symmetric_difference() will accept any iterable as argument. In contrast, their operator based counterparts require that the arguments must be sets. This avoids potential errors such as using set('abc') & 'cbs' instead of set('abc').intersection('cbs') for more readability. Changes from version 2.3.1: Previously all parameters had to be sets.
In addition, both Set and ImmutableSet support comparison between sets. Two sets are equal if and only if: the elements of each set are elements of the other (they are each other's subset). One set is smaller than another set only if the first set is a subset of the second set (a subset, but not equal). One set is stronger than another set only if the first set is a superset of the second set (a superset, but not equal).
Subsets and equality comparisons do not produce full sorting functionality. For example: any two sets are neither equal nor subsets of each other, so the following operations will return False: a
b. Therefore, sets does not provide a __cmp__ method. Because sets only defines part of the sorting function (subset relationship), the output of the list.sort() method is not defined for the list of sets.Operator
Operation result
hash(s)
Returns the hash value of s
The following table lists the operations available for Set but not available for ImmutableSet:
operator (voperator)
is equivalent to
operation result
s.update(t)
s |= t
Returns set "s"
s.intersection_update(t)
s &= t
after adding elements in set "t". Returns only set "s"
s containing elements in set "t". difference_update(t)
s -= t
Returns set "s" after deleting the elements contained in set "t"
s.symmetric_difference_update(t)
s ^= t
Returns set "t" ” or set “s” that has elements in set “s” but not both
s.add(x)
adds element x to set “s”
s.remove(x)
Removes the element Returns an unspecified element in set "s", or raises KeyError if empty
s.clear()
Removes all elements in set "s"
Please note: non-operator version of update() , intersection_update(), difference_update() and symmetric_difference_update() will accept any iterable as argument. Changes from version 2.3.1: Previously all parameters had to be sets.
Also please note: this module also contains a union_update() method, which is an alias of the update() method. This method is included for backward compatibility. Programmers should use the update() method more often, as this method is also supported by the built-in set() and frozenset() types.
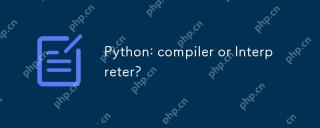
Python is an interpreted language, but it also includes the compilation process. 1) Python code is first compiled into bytecode. 2) Bytecode is interpreted and executed by Python virtual machine. 3) This hybrid mechanism makes Python both flexible and efficient, but not as fast as a fully compiled language.
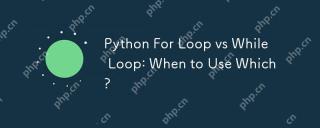
Useaforloopwheniteratingoverasequenceorforaspecificnumberoftimes;useawhileloopwhencontinuinguntilaconditionismet.Forloopsareidealforknownsequences,whilewhileloopssuitsituationswithundeterminediterations.
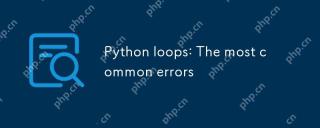
Pythonloopscanleadtoerrorslikeinfiniteloops,modifyinglistsduringiteration,off-by-oneerrors,zero-indexingissues,andnestedloopinefficiencies.Toavoidthese:1)Use'i
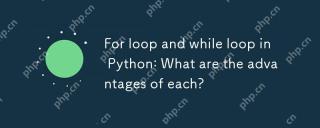
Forloopsareadvantageousforknowniterationsandsequences,offeringsimplicityandreadability;whileloopsareidealfordynamicconditionsandunknowniterations,providingcontrolovertermination.1)Forloopsareperfectforiteratingoverlists,tuples,orstrings,directlyacces
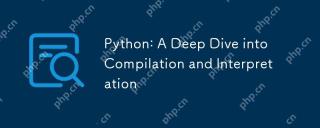
Pythonusesahybridmodelofcompilationandinterpretation:1)ThePythoninterpretercompilessourcecodeintoplatform-independentbytecode.2)ThePythonVirtualMachine(PVM)thenexecutesthisbytecode,balancingeaseofusewithperformance.
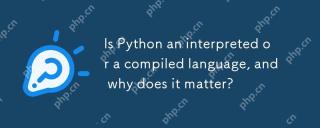
Pythonisbothinterpretedandcompiled.1)It'scompiledtobytecodeforportabilityacrossplatforms.2)Thebytecodeistheninterpreted,allowingfordynamictypingandrapiddevelopment,thoughitmaybeslowerthanfullycompiledlanguages.
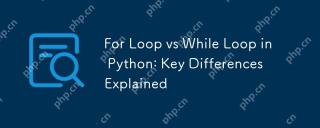
Forloopsareidealwhenyouknowthenumberofiterationsinadvance,whilewhileloopsarebetterforsituationswhereyouneedtoloopuntilaconditionismet.Forloopsaremoreefficientandreadable,suitableforiteratingoversequences,whereaswhileloopsoffermorecontrolandareusefulf
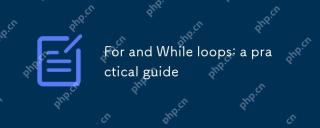
Forloopsareusedwhenthenumberofiterationsisknowninadvance,whilewhileloopsareusedwhentheiterationsdependonacondition.1)Forloopsareidealforiteratingoversequenceslikelistsorarrays.2)Whileloopsaresuitableforscenarioswheretheloopcontinuesuntilaspecificcond


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
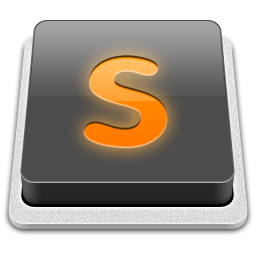
SublimeText3 Mac version
God-level code editing software (SublimeText3)
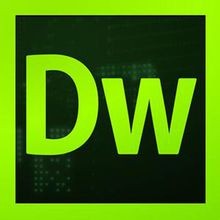
Dreamweaver CS6
Visual web development tools
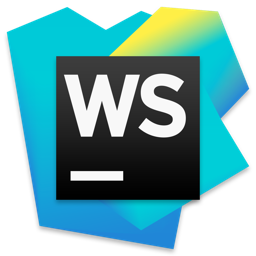
WebStorm Mac version
Useful JavaScript development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
