


Summary of PHP array declaration, traversal, and array global variable usage_PHP tutorial
PHP tutorial: array declaration, traversal, array global variables
/*
* 1. Overview of arrays
* 1. The essence of arrays: manage and operate a set of variables, batch processing
* 2. Arrays Composite type (can store multiple)
* 3. Arrays can store data of any length and any type of data
* 4. Arrays can complete the functions of other language data structures (linked lists, queues , stack, collection class)
*
*
*
* 2. Classification of arrays
* There are multiple units in the array, (units are called elements)
* Each Element (subscript [key] and value)
* When accessing an element individually, the element is accessed through the subscript (key)
* 1. One-dimensional array, two-dimensional array, three-dimensional array. . . Multidimensional array
* (an array of arrays means that other arrays are stored in the array)
* 2. There are two types of arrays in PHP
* Index array: It is an index whose subscript is a sequential integer
* Associative array: The subscript is a string as an index
*
* There are only two types of subscripts (integer, string)
*
*
* 3. Multiple arrays Declaration method
*
* 1. Directly assign a value to the array element declaration
* If the index subscript is not given, the sequential index will start from 0
* If the index subscript is given, the next It will increase by 1 starting from the largest
* If the previous subscript appears later, if it is an assignment, the previous element will be reassigned
* When mixed declaration, index and association do not affect each other (it does not affect the index subscript) Declaration)
*
* 2. Use the array() function to declare
* The default is an index array
* If you specify a subscript for an associative array and an index array, use key => value
* Use " , " to split
* between multiple members. 3. Use other function declarations
*
*
*
*
*/
//Index array
$user[0]=1;//User serial number
$user[1]="zhangsan";//User name
$user[2]=10;//Age
$ user[3]="nan";//Gender
echo '
'; <br>print_r($user); <br>echo '';
//Association Array
$user["id"]=1;
$user["name"]="zhangsan";
$user["age"]=10;
$user["sex "];
$user["age"]=90;//Assignment
echo $user["name"];//Output
//Use array() to declare the array
$user =array(1,"zhangsan",10,"nan");
//Use array() to declare an associative array
$user=array("id"=>1,"name"=> "zhangsan","age"=>10,"sex"=>"nan");
//Declare a multi-dimensional array (multiple records) to save multiple user information records in a table
$user=array(
//Use $user[0] to call this line, such as calling the name in this record, $user[0][1]
array(1,"zhangsan",10 ,"nan"),
//Use $user[1] to call this line, such as calling the name in this record, $user[1][1]
array(2,"lisi",20 ,"nv")
);
//Array saves multiple tables, each table has multiple records
$info=array(
"user"=>array(
array(1,"zhangsan",10,"nan"),
array(2,"lisi",20,"nv")
),
"score"=>array(
array(1,90,80,70),
array(2,60,40,70)
)
);
echo $info["score"][1 ][1];//Output 60,
?>
Array super global variable
/* Predefined array:
* Automatic global variable---super Global array
*
* 1. Contains data from the WEB server, client, operating environment and user input
* 2. These arrays are special
* 3. Automatically take effect in the global scope, These arrays can be used directly
* 4. Users cannot customize these arrays, but the operations of these arrays are the same as those defined by themselves
* 5. These arrays can be used directly in functions
*
* $_GET //Variables submitted to the script via URL request
* $_POST //Variables submitted to the script via HTTP POST method
* $_REQUEST //Via GET, POST and COOKIE mechanisms Variables submitted to the script
* $_FILES //Variables submitted to the script via http post method file upload
* $_COOKIE
* $_SESSION
* $_ENV //Execution environment submitted to the script Variables
* $_SERVER //Variables are set by the WEB server, or directly associated with the execution environment of the current script
* $GLOBALS //As long as the variables that are valid for the current script are here, the keys of the array The name of the global script
*
*
*/
//Super global array can be called directly inside the function
$arr=array(10,20);//General array
$_GET=array(50,90);//Super global array
function demo(){
global $arr;//When calling global variables, you must first include
print_r($arr);
print_r($_GET);//Directly call the super global array without including
}
?>
//Use the passed value directly as a variable. Useful when register_global=on in the php.ini configuration file.
echo $username."
";
echo $email."
";
echo $page."
";
//The most stable Value method
echo $_GET["username"]."
";
echo $_GET["email"]."
";
echo $_GET["page "]."
";
?>
this is a $_GET test
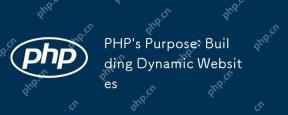
PHP is used to build dynamic websites, and its core functions include: 1. Generate dynamic content and generate web pages in real time by connecting with the database; 2. Process user interaction and form submissions, verify inputs and respond to operations; 3. Manage sessions and user authentication to provide a personalized experience; 4. Optimize performance and follow best practices to improve website efficiency and security.

PHP uses MySQLi and PDO extensions to interact in database operations and server-side logic processing, and processes server-side logic through functions such as session management. 1) Use MySQLi or PDO to connect to the database and execute SQL queries. 2) Handle HTTP requests and user status through session management and other functions. 3) Use transactions to ensure the atomicity of database operations. 4) Prevent SQL injection, use exception handling and closing connections for debugging. 5) Optimize performance through indexing and cache, write highly readable code and perform error handling.
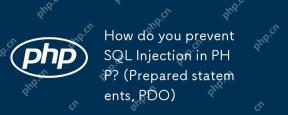
Using preprocessing statements and PDO in PHP can effectively prevent SQL injection attacks. 1) Use PDO to connect to the database and set the error mode. 2) Create preprocessing statements through the prepare method and pass data using placeholders and execute methods. 3) Process query results and ensure the security and performance of the code.
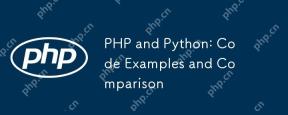
PHP and Python have their own advantages and disadvantages, and the choice depends on project needs and personal preferences. 1.PHP is suitable for rapid development and maintenance of large-scale web applications. 2. Python dominates the field of data science and machine learning.
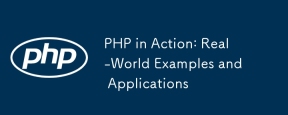
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
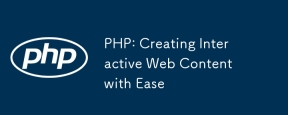
PHP makes it easy to create interactive web content. 1) Dynamically generate content by embedding HTML and display it in real time based on user input or database data. 2) Process form submission and generate dynamic output to ensure that htmlspecialchars is used to prevent XSS. 3) Use MySQL to create a user registration system, and use password_hash and preprocessing statements to enhance security. Mastering these techniques will improve the efficiency of web development.
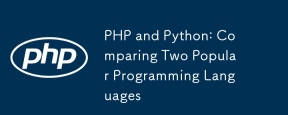
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
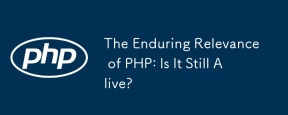
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
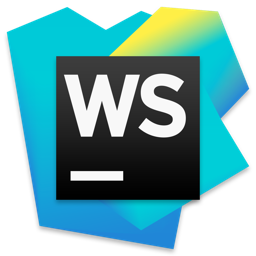
WebStorm Mac version
Useful JavaScript development tools
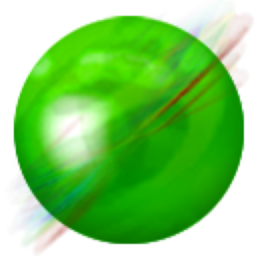
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment