Using Smarty in PHP Part One: Using Variables_PHP Tutorial
All access in Smarty is based on variables. The following is an example to illustrate.
Example idea: The main file introduces the template initialization configuration file (init.inc.php) and a class, and assigns values to the variables in the template.
First, set the init.inc.php file as the initialization configuration file of the Smarty template
init.inc.php
define('ROOT_PATH', dirname(__FILE__)); //Define the website root directory
require ROOT_PATH.'/libs/Smarty.class.php'; //Load Smarty file
$_tpl = new Smarty(); //Instantiate an object
$_tpl->template_dir = ROOT_PATH.'/tpl/'; //Reset the template directory to the tpl directory under the root directory
$_tpl->compile_dir = ROOT_PATH.'./com/'; //Reset the compilation directory to the com directory under the root directory
$_tpl->left_delimiter = '
$_tpl->right_delimiter = '}>'; //Reset the left delimiter to '}>'
?>
Main file index.php
require 'init.inc.php'; //Introduce template initialization file
require 'Persion.class.php'; //Load object file
global $_tpl;
$title = 'This is a title!';
$content = 'This is body content!';
/*
* 1. Assign to template variables from PHP;
* Dynamic data (PHP from database or file, and variables generated by algorithms)
* Any type of data can be allocated from PHP, mainly including the following
* Scalar: string, int, double, boolean
* Composite: array, object
* NULL
* The index array is accessed directly through the index
* For associative arrays, instead of using [associated subscript], use . subscript
* The object is accessed directly through ->
* */
$_tpl->assign('title',$title);
$_tpl->assign('content',$content); //Assignment of variables
$_tpl->assign('arr1',array('abc','def','ghi')); //Assignment of index array
$_tpl->assign('arr2',array(array('abc','def','ghi'),array('jkl','mno','pqr'))); //Index two-dimensional Array assignment
$_tpl->assign('arr3',array('one'=>'111','two'=>'222','three'=>'333')); //Associative array Assignment
$_tpl->assign('arr4',array('one'=>array('one'=>'111','two'=>'222'),'two'=>array( 'three'=>'333','four'=>'444'))); //Assignment of associated two-dimensional array
$_tpl->assign('arr5',array('one'=>array('111','222'),array('three'=>'333','444'))); / /Assignment of mixed arrays of associative and indexed arrays
$_tpl->assign('object',new Persion('Xiaoyi', 10)); //Object assignment
//Numbers in Smarty can also be operated (+-*/^...)
$_tpl->assign('num1',10);
$_tpl->assign('num2',20);
$_tpl->display('index.tpl');
?>
The template file index.tpl of the main file index.php (stored in the /tpl/ directory)
ttp://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd" >http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
http://www.w3.org/1999/xhtml">
Variable access:
Index array access:
Access to indexed two-dimensional array: < ;{$arr2[1][0]}>
Access to associative array:
Access to associative two-dimensional arrays:
Access to mixed associative and indexed arrays:
Access to member variables in the object: name}> age}>
Access to methods in objects: hello()}>
Variable operations:
Mixed operations of variables:
Persion.class.php
class Persion {
public $name; //For ease of access, set to public
Public $age;
//Define a construction method
Public function __construct($name,$age) {
$this->name = $name;
$this->age = $age;
}
//Define a hello() method to output name and age
Public function hello() {
return 'Hello! My name is '.$this->name.', and I am '.$this->age.' this year. ';
}
}
?>
Execution result:
Variable access: This is body content!
Index array access: abc def ghi
Access to indexed two-dimensional array: abc def ghi jkl mno pqr
Access to associative array: 111 222 333
Access to associative two-dimensional array: 111 222 333 444
Access to mixed associative and indexed arrays: 111 222 333 444
Access to member variables in objects: Xiaoyi 10
Access to methods in objects: Hello! My name is Xiao Yi, I am 10 years old this year.
Variable operations: 30
Mixed operations on variables: 94
Excerpted from: lee's column
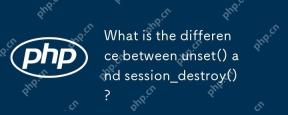
Thedifferencebetweenunset()andsession_destroy()isthatunset()clearsspecificsessionvariableswhilekeepingthesessionactive,whereassession_destroy()terminatestheentiresession.1)Useunset()toremovespecificsessionvariableswithoutaffectingthesession'soveralls
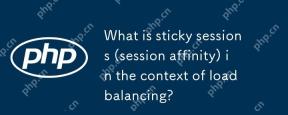
Stickysessionsensureuserrequestsareroutedtothesameserverforsessiondataconsistency.1)SessionIdentificationassignsuserstoserversusingcookiesorURLmodifications.2)ConsistentRoutingdirectssubsequentrequeststothesameserver.3)LoadBalancingdistributesnewuser
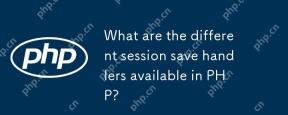
PHPoffersvarioussessionsavehandlers:1)Files:Default,simplebutmaybottleneckonhigh-trafficsites.2)Memcached:High-performance,idealforspeed-criticalapplications.3)Redis:SimilartoMemcached,withaddedpersistence.4)Databases:Offerscontrol,usefulforintegrati
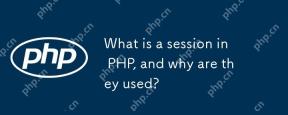
Session in PHP is a mechanism for saving user data on the server side to maintain state between multiple requests. Specifically, 1) the session is started by the session_start() function, and data is stored and read through the $_SESSION super global array; 2) the session data is stored in the server's temporary files by default, but can be optimized through database or memory storage; 3) the session can be used to realize user login status tracking and shopping cart management functions; 4) Pay attention to the secure transmission and performance optimization of the session to ensure the security and efficiency of the application.
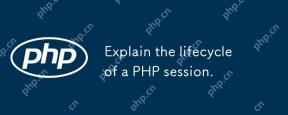
PHPsessionsstartwithsession_start(),whichgeneratesauniqueIDandcreatesaserverfile;theypersistacrossrequestsandcanbemanuallyendedwithsession_destroy().1)Sessionsbeginwhensession_start()iscalled,creatingauniqueIDandserverfile.2)Theycontinueasdataisloade
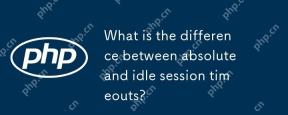
Absolute session timeout starts at the time of session creation, while an idle session timeout starts at the time of user's no operation. Absolute session timeout is suitable for scenarios where strict control of the session life cycle is required, such as financial applications; idle session timeout is suitable for applications that want users to keep their session active for a long time, such as social media.
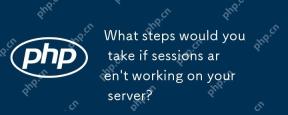
The server session failure can be solved through the following steps: 1. Check the server configuration to ensure that the session is set correctly. 2. Verify client cookies, confirm that the browser supports it and send it correctly. 3. Check session storage services, such as Redis, to ensure that they are running normally. 4. Review the application code to ensure the correct session logic. Through these steps, conversation problems can be effectively diagnosed and repaired and user experience can be improved.
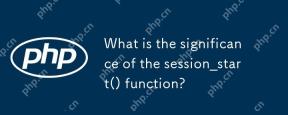
session_start()iscrucialinPHPformanagingusersessions.1)Itinitiatesanewsessionifnoneexists,2)resumesanexistingsession,and3)setsasessioncookieforcontinuityacrossrequests,enablingapplicationslikeuserauthenticationandpersonalizedcontent.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Notepad++7.3.1
Easy-to-use and free code editor
