array("key"=>"value");Create an array
// Display array
print_r($array);
//Use the compact() function to create a new array, and use the parameters as the units of the new array;
$newArray = compact("red","green","yellow","blue","array");
//Use the extract() function to convert the cells in the array into variables
Extract($exArray);
echo "$key1 $key2 $key3 $key4 $key5";
※Check values and keys
array_key_exists($key,$array);//Check array key
in_array($value,$array);//Check the value in the array
※Get the value
//Use array_values() to get the value of the array
$carValues = array_values($car);
//Get the key name of the array
$twoKeys = array_keys($two);
Key($array);//Output the key name of the current unit
//After the array is defined, use current() to obtain the value of the current unit
$red = current($array);
list($red,$green) = $array;//Assign the value in the array to the variable, $array = array("red", "green");
each($two);//Return the key and value of the current unit in the array
※Traverse the array
foreach($two as $subArray);//Traverse the array
while(list($key,$value) = each($array)){
echo "$key=>$value,";//Use each to traverse the array
}
※Fill array
// Fill the array to the left and right
array_pad($array,+3,"shuzhi");//The 2 parameters are filled from left to right, and will be filled only when the value is greater than the number of units
$array1 = array_fill(5,5,"test");//Use array_fill() to fill the value of this array, the value is test, start filling from the 5th unit, and fill 5 units in total
// Fill in the array key name
$keys = array(string, 5, 10, str);
$array3 = array_fill_keys($keys,"array value");
//Use the array_filp() function to exchange key names and values
$speed = array_flip($speed);
//Use the array_splice() function to replace the value of the 6th cell with 7
$output = array_splice($input,6,0,7);
//Use the array_splice() function to delete array cells and keep only the first 5 cells
$output = array_splice($input,5);
$array1 = range(10,100,10); //Use the third parameter of the range() function to set the step value between units
※Sort
shuffle($array);//Shuffle the order of the array
//Use array_multisort() to sort three arrays
array_multisort($sort1,$sort2,$sort3);
// Sort this array and maintain the index relationship
asort($array);
// Sort the test array in reverse order and maintain the index relationship
arsort($array);
//Use ksort() to sort the array by key name
ksort($array);
//Use the krsort() function to sort by key name in reverse order
krsort($array);
//Use sort() to sort the test array [arranged by key name]
sort($array);
// Use natsort() to sort [natural sorting, numerical arrangement] which is case-sensitive for unit values
natsort($array);
//Use the natcasesort() function to sort [natural sorting] but ignore the case of values
natcasesort($array);
//Use the array_reverse() function to sort, and the array units are arranged in reverse order
$newArray = array_reverse($array,TRUE);//Retain the original key name when TRUE is set
※Intersection and difference
//Use array_diff() to calculate the difference set of three arrays [Compare array values]
$result = array_diff($dog1,$dog2,$dog3);
//Use array_diff_assoc() to calculate the difference set of three arrays [compare values and key names]
$result = array_diff_assoc($dog1,$dog2,$dog3);
//Use array_diff_key() to calculate the difference set of three arrays [Compare key names]
$result = array_diff_key($dog1,$dog2,$dog3);
//Use array_intersect() to calculate the intersection of three arrays [Compare array values]
$result = array_intersect($dog1,$dog2,$dog3);
//Use array_intersect_assoc() to calculate the intersection of three arrays [compare values and key names]
$result = array_intersect_assoc($dog1,$dog2,$dog3);
//Use array_intersect_key() to calculate the intersection of three arrays [Compare key names]
$result = array_intersect_key($dog1,$dog2,$dog3);
※Merge arrays
//Use the array_merge() function to merge arrays
$result = array_merge($array1,$array2,$array3,$array4,$array5);
array_rand($input,10);//Randomly take out 10 units
Count($array,COUNT_RECURSIVE);//Display the number of array units, the 2 parameters can only be 1 or COUNT_RECURSIVE, sometimes multi-dimensional arrays can be traversed
※In and out of stack
//The array is popped from the stack, last in first out, and the last unit of the array is popped
array_pop($array);
// Push the array onto the stack and add the two values 7 and 8 to the end of the array
array_push($array,7,8);
//Move the beginning element of the array out of the array
array_shift($array);
//Add 7 and 8 to the beginning of the array
array_unshift($array,7,8);
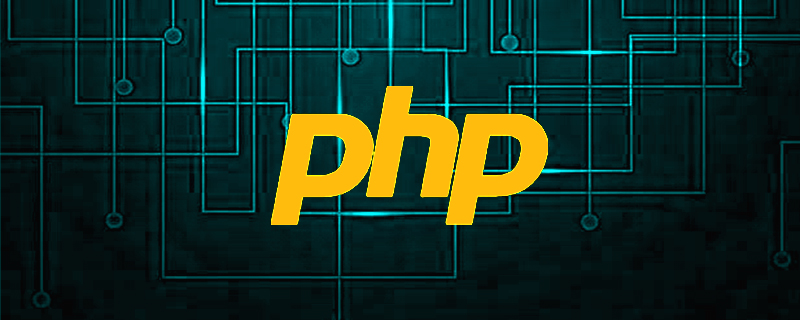
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
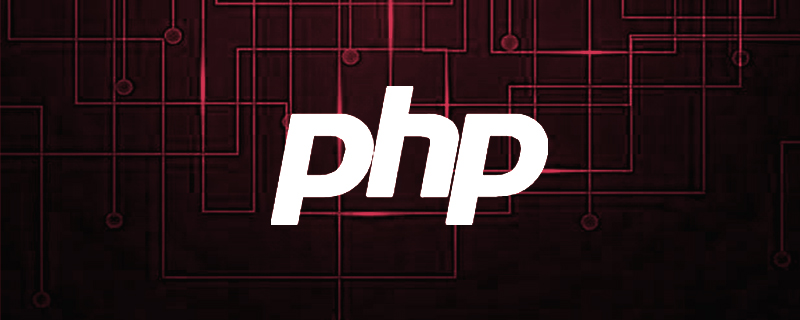
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
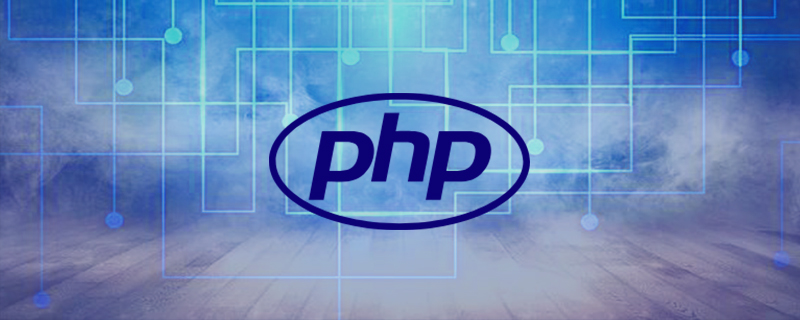
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
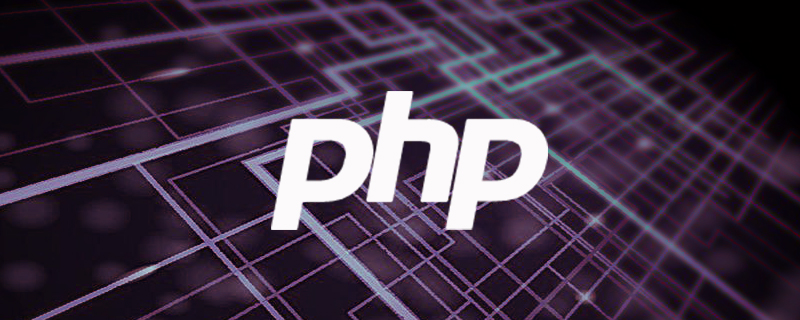
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
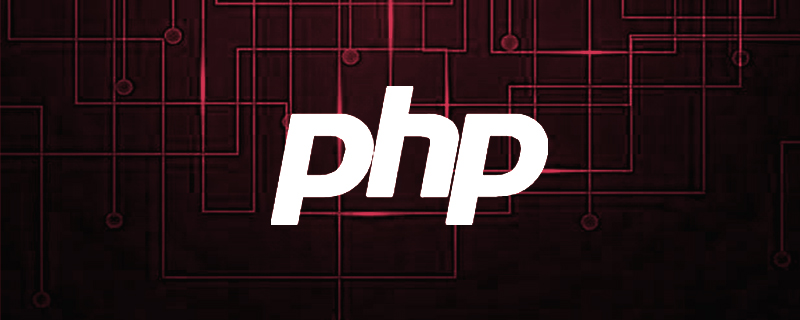
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
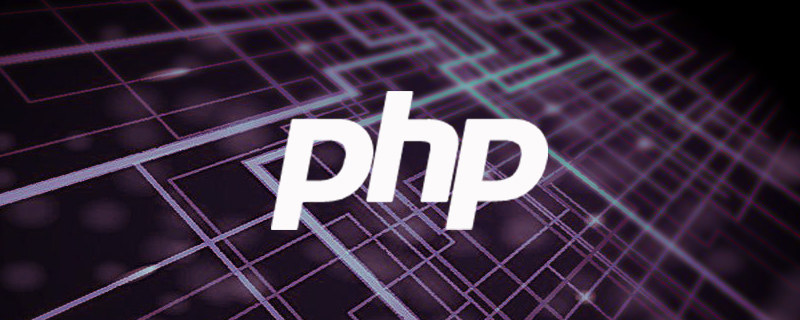
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。
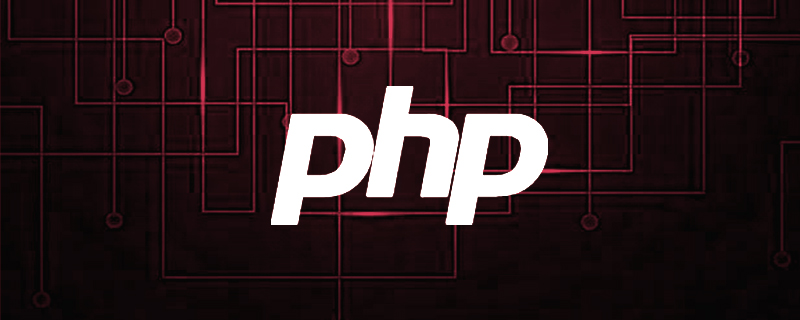
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
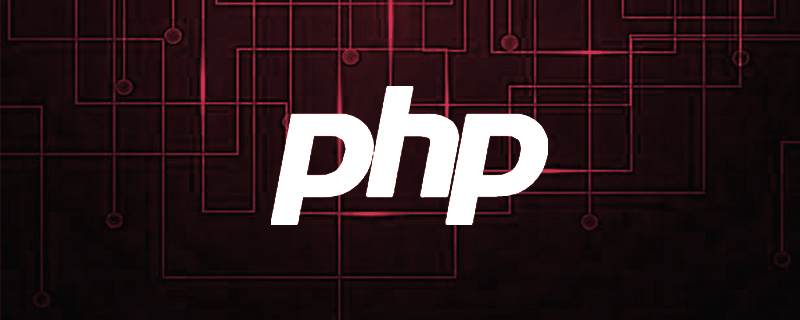
php判断有没有小数点的方法:1、使用“strpos(数字字符串,'.')”语法,如果返回小数点在字符串中第一次出现的位置,则有小数点;2、使用“strrpos(数字字符串,'.')”语句,如果返回小数点在字符串中最后一次出现的位置,则有。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
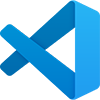
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
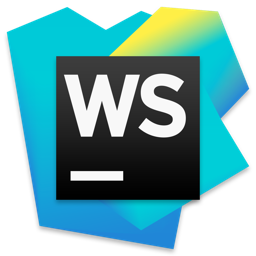
WebStorm Mac version
Useful JavaScript development tools
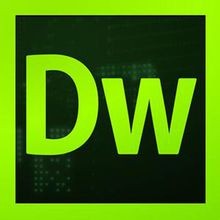
Dreamweaver CS6
Visual web development tools
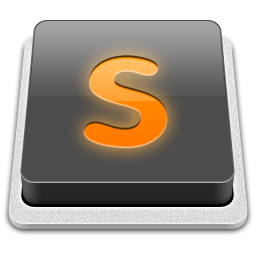
SublimeText3 Mac version
God-level code editing software (SublimeText3)
