PHP Design Patterns: Factory Pattern_PHP Tutorial
In the article "Do you know PHP design patterns", we briefly introduced the factory pattern. Today we will take a detailed look at the application scenarios of the factory pattern in PHP development.
To learn more about PHP design patterns, please visit: Talk about PHP design patterns
Originally in the book Design Patterns, many design patterns encourage the use of loose coupling. To understand this concept, it's best to talk about the arduous journey that many developers go through working on large systems. When you change one piece of code, problems can occur, and cascading breaks can occur in other parts of the system—parts you once thought were completely unrelated.
The problem is tight coupling. Functions and classes in one part of the system are heavily dependent on the behavior and structure of functions and classes in other parts of the system. You want a set of patterns that allow these classes to communicate with each other, but you don't want to tie them tightly together to avoid interlocking.
In large systems, a lot of code depends on a few key classes. Difficulties may arise when these classes need to be changed. For example, suppose you have a User class that reads from a file. You want to change it to a different class that reads from the database, however, all your code references the original class that reads from the file. At this time, it will be very convenient to use factory mode.
Factory pattern is a class that has certain methods that create objects for you. You can use a factory class to create objects without using new directly. This way, if you want to change the type of object created, you only need to change the factory. All code using this factory is automatically changed.
Example 1: Display a column of the factory class. The server side of the equation consists of two parts: a database and a set of PHP pages that allow you to add feedback, request a list of feedback, and get articles related to a specific feedback.
<ol class="dp-c"><li class="alt"><span><span><?php </span></span><li> <span class="keyword">interface</span><span> IUser </span> </li> <li class="alt"><span>{ </span></li> <li> <span> </span><span class="keyword">function</span><span> getName(); </span> </li> <li class="alt"><span>} </span></li> <li><span> </span></li> <li class="alt"> <span class="keyword">class</span><span> User </span><span class="keyword">implements</span><span> IUser </span> </li> <li><span>{ </span></li> <li class="alt"> <span> </span><span class="keyword">public</span><span> </span><span class="keyword">function</span><span> __construct( </span><span class="vars">$id</span><span> ) { } </span> </li> <li><span> </span></li> <li class="alt"> <span> </span><span class="keyword">public</span><span> </span><span class="keyword">function</span><span> getName() </span> </li> <li><span> { </span></li> <li class="alt"> <span> </span><span class="keyword">return</span><span> </span><span class="string">"Jack"</span><span>; </span> </li> <li><span> } </span></li> <li class="alt"><span>} </span></li> <li><span> </span></li> <li class="alt"> <span class="keyword">class</span><span> UserFactory </span> </li> <li><span>{ </span></li> <li class="alt"> <span> </span><span class="keyword">public</span><span> </span><span class="keyword">static</span><span> </span><span class="keyword">function</span><span> Create( </span><span class="vars">$id</span><span> ) </span> </li> <li><span> { </span></li> <li class="alt"> <span> </span><span class="keyword">return</span><span> </span><span class="keyword">new</span><span> User( </span><span class="vars">$id</span><span> ); </span> </li> <li><span> } </span></li> <li class="alt"><span>} </span></li> <li><span> </span></li> <li class="alt"> <span class="vars">$uo</span><span> = UserFactory::Create( 1 ); </span> </li> <li> <span class="func">echo</span><span>( </span><span class="vars">$uo</span><span>->getName().</span><span class="string">"\n"</span><span> ); </span> </li> <li class="alt"><span>?> </span></li></span></li></ol>
The IUser interface defines what operations the user object should perform. The implementation of IUser is called User, and the UserFactory factory class creates IUser objects. This relationship can be represented by UML in Figure 1.
Figure 1. Factory class and its related IUser interface and user class
If you run this code on the command line using the php interpreter, you will get the following result:
<ol class="dp-c"> <li class="alt"><span><span>% php factory1.php </span></span></li> <li><span>Jack </span></li> <li class="alt"><span>% </span></li> </ol>
The test code will request the User object from the factory and output the result of the getName method.
There is a variant of the factory pattern that uses factory methods. These public static methods in a class construct objects of that type. This method is useful if it is important to create objects of this type. For example, suppose you need to create an object first and then set a number of properties. This version of the factory pattern encapsulates the process in a single location, so you don't have to copy complex initialization code and paste it all over the code base.
Example 2 shows an example of using factory methods.
<ol class="dp-c"><li class="alt"><span><span><?php </span></span><li> <span class="keyword">interface</span><span> IUser </span> </li> <li class="alt"><span>{ </span></li> <li> <span> </span><span class="keyword">function</span><span> getName(); </span> </li> <li class="alt"><span>} </span></li> <li><span> </span></li> <li class="alt"> <span class="keyword">class</span><span> User </span><span class="keyword">implements</span><span> IUser </span> </li> <li><span>{ </span></li> <li class="alt"> <span> </span><span class="keyword">public</span><span> </span><span class="keyword">static</span><span> </span><span class="keyword">function</span><span> Load( </span><span class="vars">$id</span><span> ) </span> </li> <li><span> { </span></li> <li class="alt"> <span> </span><span class="keyword">return</span><span> </span><span class="keyword">new</span><span> User( </span><span class="vars">$id</span><span> ); </span> </li> <li><span> } </span></li> <li class="alt"><span> </span></li> <li> <span> </span><span class="keyword">public</span><span> </span><span class="keyword">static</span><span> </span><span class="keyword">function</span><span> Create( ) </span> </li> <li class="alt"><span> { </span></li> <li> <span> </span><span class="keyword">return</span><span> </span><span class="keyword">new</span><span> User( null ); </span> </li> <li class="alt"><span> } </span></li> <li><span> </span></li> <li class="alt"> <span> </span><span class="keyword">public</span><span> </span><span class="keyword">function</span><span> __construct( </span><span class="vars">$id</span><span> ) { } </span> </li> <li><span> </span></li> <li class="alt"> <span> </span><span class="keyword">public</span><span> </span><span class="keyword">function</span><span> getName() </span> </li> <li><span> { </span></li> <li class="alt"> <span> </span><span class="keyword">return</span><span> </span><span class="string">"Jack"</span><span>; </span> </li> <li><span> } </span></li> <li class="alt"><span>} </span></li> <li><span> </span></li> <li class="alt"> <span class="vars">$uo</span><span> = User::Load( 1 ); </span> </li> <li> <span class="func">echo</span><span>( </span><span class="vars">$uo</span><span>->getName().</span><span class="string">"\n"</span><span> ); </span> </li> <li class="alt"><span>?> </span></li></span></li></ol>
This code is much simpler. It has only one interface IUser and a User class that implements this interface. The User class has two static methods for creating objects. This relationship can be represented by UML in Figure 2.
Figure 2. IUser interface and user class with factory method
Running the script on the command line produces the same results as Listing 1, as follows:
<ol class="dp-c"> <li class="alt"><span><span>% php factory2.php </span></span></li> <li><span>Jack </span></li> <li class="alt"><span>% </span></li> </ol>
As mentioned above, sometimes such modes can seem overkill in smaller environments. However, it's best to learn this solid form of coding that you can apply to projects of any size.
- Discussion on PHP Design Patterns: Proxy Pattern
- Chat of Responsibility Model on PHP Design Patterns
- Structural Patterns of PHP Design Patterns
- Command Pattern of PHP Design Patterns
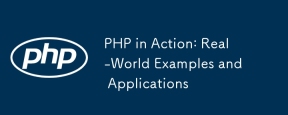
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
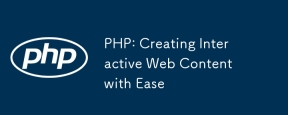
PHP makes it easy to create interactive web content. 1) Dynamically generate content by embedding HTML and display it in real time based on user input or database data. 2) Process form submission and generate dynamic output to ensure that htmlspecialchars is used to prevent XSS. 3) Use MySQL to create a user registration system, and use password_hash and preprocessing statements to enhance security. Mastering these techniques will improve the efficiency of web development.
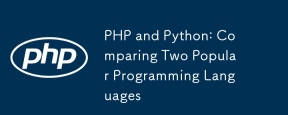
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
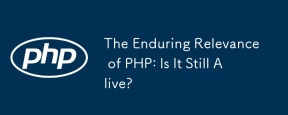
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.
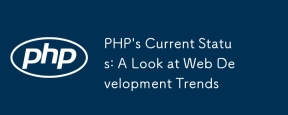
PHP remains important in modern web development, especially in content management and e-commerce platforms. 1) PHP has a rich ecosystem and strong framework support, such as Laravel and Symfony. 2) Performance optimization can be achieved through OPcache and Nginx. 3) PHP8.0 introduces JIT compiler to improve performance. 4) Cloud-native applications are deployed through Docker and Kubernetes to improve flexibility and scalability.
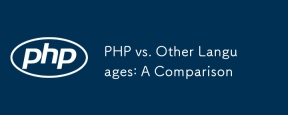
PHP is suitable for web development, especially in rapid development and processing dynamic content, but is not good at data science and enterprise-level applications. Compared with Python, PHP has more advantages in web development, but is not as good as Python in the field of data science; compared with Java, PHP performs worse in enterprise-level applications, but is more flexible in web development; compared with JavaScript, PHP is more concise in back-end development, but is not as good as JavaScript in front-end development.
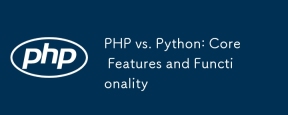
PHP and Python each have their own advantages and are suitable for different scenarios. 1.PHP is suitable for web development and provides built-in web servers and rich function libraries. 2. Python is suitable for data science and machine learning, with concise syntax and a powerful standard library. When choosing, it should be decided based on project requirements.
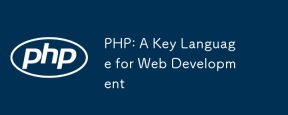
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),