


Discuss some issues to pay attention to when referencing the & symbol in PHP_PHP Tutorial
PHP reference & symbol is a difficult knowledge point to master. Novices must pay more attention to this when actually writing code, because if you misunderstand the use of the PHP reference & symbol, it will cause errors in the entire code you write.
- How to use PHP associative array to query results
- Specific ways to correct common PHP errors
- The specific method of querying a single component using the PHP function header()
- WordPress won the second place in the 2009 Open Source PHP Project
- Relation between converting PHP array to string and converting PHP string to array
<ol class="dp-xml"> <li class="alt"><span><span>$</span><span class="attribute"><font color="#ff0000">a</font></span><span> = </span><span class="attribute-value"><font color="#0000ff">array</font></span><span>('a','c'...'n'); </span></span></li> <li class=""> <span>$</span><span class="attribute"><font color="#ff0000">b</font></span><span> = $a; </span> </li> </ol>
If the program only executes here, $b and $b are the same, but they do not occupy different memory spaces like C. Instead, they point to the same memory space. This is php and c. The difference is that you don’t need to write $b=&$a to mean that $b points to the memory of $a. zend has already implemented the reference for you, and zend will be very smart to help you judge when to do this and when. It shouldn't be handled this way.
If you continue to write the following code later, add a function, pass parameters through PHP reference & symbol, and print out the array size.
<ol class="dp-xml"> <li class="alt"><span><span>function printArray(&$arr) //引用传递 </span></span></li> <li class=""><span> { </span></li> <li class="alt"><span> print(count($arr)); </span></li> <li class=""><span> } </span></li> <li class="alt"><span> </span></li> <li class=""><span> printArray($a); </span></li> </ol>
In the above code, we pass the $a array into the printArray() function through the PHP reference & symbol. The zend engine will think that printArray() may cause changes to $a, and it will automatically produce $b at this time. A data copy of $a, re-applying for a piece of memory for storage. This is the "copy-on-write" concept mentioned earlier.
If we change the above code to the following:
<ol class="dp-xml"> <li class="alt"><span><span>function printArray($arr) //值传递 </span></span></li> <li class=""><span> { </span></li> <li class="alt"><span> print(count($arr)); </span></li> <li class=""><span> } </span></li> <li class="alt"><span> </span></li> <li class=""><span> printArray($a); </span></li> </ol>
The above code directly passes the $a value to printArray(). There is no reference transfer at this time, so there is no copy-on-write.
You can test the execution efficiency of the above two lines of code. For example, add a loop outside 1000 times and see how long it takes to run. The results will let you know that incorrect use of the PHP reference & symbol will cause performance to drop by more than 30%.
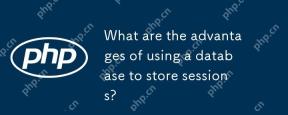
The main advantages of using database storage sessions include persistence, scalability, and security. 1. Persistence: Even if the server restarts, the session data can remain unchanged. 2. Scalability: Applicable to distributed systems, ensuring that session data is synchronized between multiple servers. 3. Security: The database provides encrypted storage to protect sensitive information.
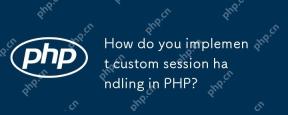
Implementing custom session processing in PHP can be done by implementing the SessionHandlerInterface interface. The specific steps include: 1) Creating a class that implements SessionHandlerInterface, such as CustomSessionHandler; 2) Rewriting methods in the interface (such as open, close, read, write, destroy, gc) to define the life cycle and storage method of session data; 3) Register a custom session processor in a PHP script and start the session. This allows data to be stored in media such as MySQL and Redis to improve performance, security and scalability.
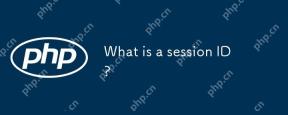
SessionID is a mechanism used in web applications to track user session status. 1. It is a randomly generated string used to maintain user's identity information during multiple interactions between the user and the server. 2. The server generates and sends it to the client through cookies or URL parameters to help identify and associate these requests in multiple requests of the user. 3. Generation usually uses random algorithms to ensure uniqueness and unpredictability. 4. In actual development, in-memory databases such as Redis can be used to store session data to improve performance and security.
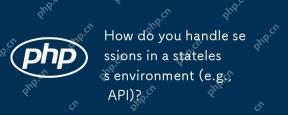
Managing sessions in stateless environments such as APIs can be achieved by using JWT or cookies. 1. JWT is suitable for statelessness and scalability, but it is large in size when it comes to big data. 2.Cookies are more traditional and easy to implement, but they need to be configured with caution to ensure security.
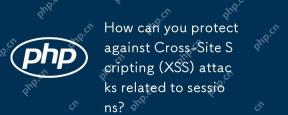
To protect the application from session-related XSS attacks, the following measures are required: 1. Set the HttpOnly and Secure flags to protect the session cookies. 2. Export codes for all user inputs. 3. Implement content security policy (CSP) to limit script sources. Through these policies, session-related XSS attacks can be effectively protected and user data can be ensured.
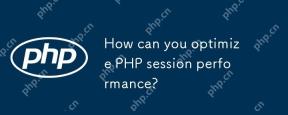
Methods to optimize PHP session performance include: 1. Delay session start, 2. Use database to store sessions, 3. Compress session data, 4. Manage session life cycle, and 5. Implement session sharing. These strategies can significantly improve the efficiency of applications in high concurrency environments.
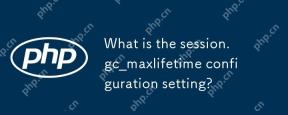
Thesession.gc_maxlifetimesettinginPHPdeterminesthelifespanofsessiondata,setinseconds.1)It'sconfiguredinphp.iniorviaini_set().2)Abalanceisneededtoavoidperformanceissuesandunexpectedlogouts.3)PHP'sgarbagecollectionisprobabilistic,influencedbygc_probabi

In PHP, you can use the session_name() function to configure the session name. The specific steps are as follows: 1. Use the session_name() function to set the session name, such as session_name("my_session"). 2. After setting the session name, call session_start() to start the session. Configuring session names can avoid session data conflicts between multiple applications and enhance security, but pay attention to the uniqueness, security, length and setting timing of session names.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
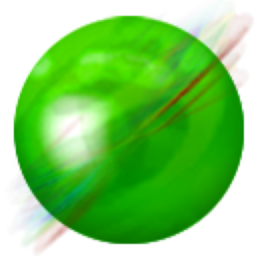
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
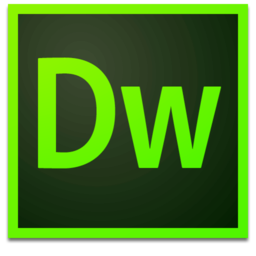
Dreamweaver Mac version
Visual web development tools
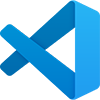
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Atom editor mac version download
The most popular open source editor

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
