PHP uses strpos function to block keyword programs_PHP tutorial
When we make message boards, we often need to block keywords. Below I will introduce how to use txt to save the keywords to be blocked, and then filter them based on the data submitted by the user.
Look at the strpos function first
The strpos() function returns the position of the first occurrence of a string in another string.
If the string is not found, return false.
Grammar
strpos(string,find,start) parameter description
string required. Specifies the string to be searched for.
find required. Specifies the characters to search for.
start is optional. Specifies the location from which to start the search.
Tips and Notes
Note: This function is case sensitive. For case-insensitive searches, use the stripos() function.
Example
The code is as follows | Copy code | ||||
|
2. PHP reads the keyword text and stores it in an array
3. Traverse the keyword array and use the strpos function one by one to see if there are keywords in the content. If there are keywords, return true, if not, return false
代码如下 | 复制代码 |
/**
|
The code is as follows | Copy code |
/** * Use strpos function to filter keywords in PHP * Qiongtai Blog */ // Keyword filter function function keyWordCheck($content){ // Remove white space $content = trim($content); //Read keyword text $content = @file_get_contents('keyWords.txt'); // Convert to array $arr = explode("n", $content); // Traversal detection for($i=0,$k=count($arr);$i // If the array element is empty, skip this loop If($arr[$i]==''){ Continue; } // If a keyword is detected, return the matching keyword and terminate the operation If(@strpos($str,$arr[$i])!==false){ //$i=$k; return $arr[$i]; } // Return false if no keyword is detected Return false; } $content = 'Here is the text content to be published. . . '; // Filter keywords $keyWord = keyWordCheck($content); // Determine whether the keyword exists if($keyWord){ echo 'The content you posted contains keywords'.$keyWord; }else{ echo 'Congratulations! By keyword detection'; // You can perform the operation of the database to complete the release action. } |
After writing the code, I deliberately wrote a keyword content in the variable $content, and then ran it and found that no keyword was detected. The execution result was passed, and other prohibited keywords were passed.
Depressed, I started to judge if there was something wrong
Encoding problem? Immediately open the keyWord.txt file again with Notepad, and then save it in UTF-8 format. The result still didn't work.
Didn't get the text content of keyWord.txt? Immediately print_r() found that it was read normally and converted into an array line by line.
So, I declared the keyword array directly in the program
The code is as follows | Copy code | ||||
|
If you declare a keyword array in PHP, it will work, but if you read a text file, it will not work. What the hell?
When I was puzzled, I thought, could it be caused by the content read from the text file having spaces or line breaks not being filtered? So I added a trim function
After adding the trim() function to filter out the blanks, the test passed. It turned out that the problem was here after messing around for a long time.
The code is as follows
|
Copy code | ||||
/**
Continue;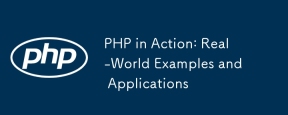
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
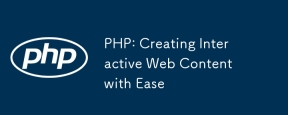
PHP makes it easy to create interactive web content. 1) Dynamically generate content by embedding HTML and display it in real time based on user input or database data. 2) Process form submission and generate dynamic output to ensure that htmlspecialchars is used to prevent XSS. 3) Use MySQL to create a user registration system, and use password_hash and preprocessing statements to enhance security. Mastering these techniques will improve the efficiency of web development.
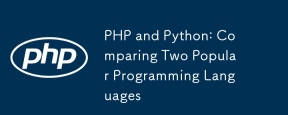
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
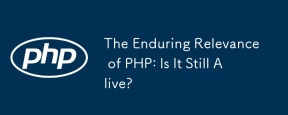
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.
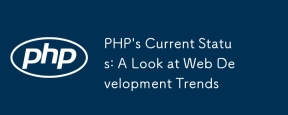
PHP remains important in modern web development, especially in content management and e-commerce platforms. 1) PHP has a rich ecosystem and strong framework support, such as Laravel and Symfony. 2) Performance optimization can be achieved through OPcache and Nginx. 3) PHP8.0 introduces JIT compiler to improve performance. 4) Cloud-native applications are deployed through Docker and Kubernetes to improve flexibility and scalability.
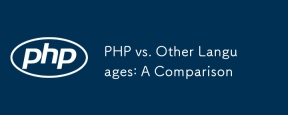
PHP is suitable for web development, especially in rapid development and processing dynamic content, but is not good at data science and enterprise-level applications. Compared with Python, PHP has more advantages in web development, but is not as good as Python in the field of data science; compared with Java, PHP performs worse in enterprise-level applications, but is more flexible in web development; compared with JavaScript, PHP is more concise in back-end development, but is not as good as JavaScript in front-end development.
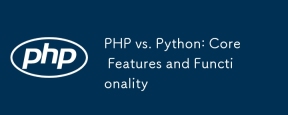
PHP and Python each have their own advantages and are suitable for different scenarios. 1.PHP is suitable for web development and provides built-in web servers and rich function libraries. 2. Python is suitable for data science and machine learning, with concise syntax and a powerful standard library. When choosing, it should be decided based on project requirements.
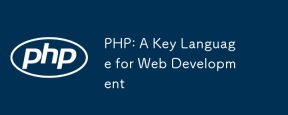
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
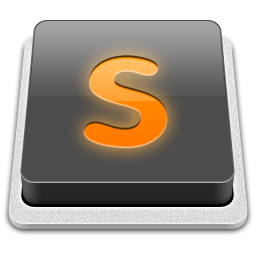
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
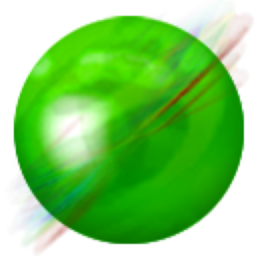
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment