At a basic level, it's important to understand how JavaScript timers work. The execution of timers is often different from our intuitive imagination. That is because the JavaScript engine is single-threaded. Let's first understand how the following three functions control timers.
Recommended reading by Script House: 10 Mini Tips for JavaScript Beginners with No Skills
var id = setTimeout(fn, delay);
Initialize a timer, and then Executed after the specified time interval. This function returns a unique flag ID (Number type), which we can use to cancel the timer.
var id = setInterval(fn, delay);
is somewhat similar to setTimeout, but it continuously calls a function (the time interval is the delay parameter) until it is canceled.
clearInterval(id);, clearTimeout(id);
Use the timer ID (the return value of setTimeout and setInterval) to cancel the occurrence of the timer callback.
In order to understand the inner execution principle of timers, there is an important concept that needs to be discussed: the delay of timers cannot be guaranteed. Since all JavaScript code is executed in a thread, all asynchronous events (such as mouse clicks and timers) will only be executed when they have a chance to execute. Illustrate it with a nice diagram:
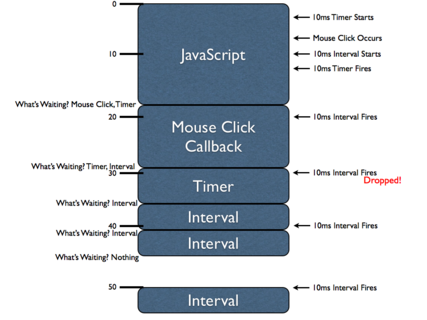
There is a lot of information to understand in this diagram, and if you understand it completely, you will have a good idea of how the JavaScript engine implements asynchronous events. This is a one-dimensional icon: the vertical direction represents time, and the blue blocks represent blocks of JavaScript code execution. For example, the first JavaScript code execution block takes about 18ms, the code execution block triggered by a mouse click takes 11ms, and so on.
Since the JavaScript engine only executes one code at a time (this is due to the single-threaded nature of JavaScript), each JavaScript code execution block will "block" the execution of other asynchronous events. This means that when an asynchronous event occurs (for example, a mouse click, a timer is triggered, or an Ajax asynchronous request), the callback functions of these events will be queued at the end of the execution queue waiting to be executed (actually, the queuing method depends on the browser Different processors, so here is just a simplification);
Start from the first JavaScript execution block. In the first execution block, two timers are initialized: a 10ms setTimeout() and A 10ms setInterval(). Depending on when and where the timer is initialized (it will start counting after the timer is initialized), the timer will actually be triggered before the first code block completes execution. However, the function bound to the timer will not be executed immediately (the reason why it is not executed immediately is that JavaScript is single-threaded). In fact, the delayed functions will be queued at the end of the execution queue, waiting for the next appropriate time to execute.
In addition, in the first JavaScript execution block we see a "mouse click" event occurring. A JavaScript callback function is bound to this asynchronous event (we never know when the user executes this (click) event, so it is considered asynchronous). This function will not be executed immediately, like the timer above, It will be queued at the end of the execution queue, waiting for execution at the next appropriate time.
When the first JavaScript execution block is executed, the browser will immediately ask a question: Which function (statement) is waiting to be executed? At this time, a "mouse click event handler function" and a "timer callback function" are waiting to be executed. The browser will select one (actually select the "handler function for mouse click event", because it can be seen from the picture that it is queued first) and execute it immediately. The "timer callback function" will wait for the next appropriate time to execute.
Note that when the "mouse click event handler" is executed, the setInterval callback function is triggered for the first time. Like the setTimeout callback function, it will be queued to the end of the execution queue and wait for execution. However, be sure to pay attention to this: when the setInterval callback function is triggered for the second time (the setTimeout function is still executing at this time) the first trigger of setTimeout will be discarded. When a long code block is executed, all setInterval callback functions may be queued at the back of the execution queue. After the code block is executed, the result will be a large series of setInterval callback functions waiting to be executed, and between these functions No intervals until it's all done. Therefore, browsers tend to queue the next handler to the end of the queue when there are no more interval handlers in the queue (this is due to the interval issue).
We can find that when the third setInterval callback function is triggered, the previous setInterval callback function is still executing. This illustrates a very important fact: setInterval does not consider what is currently being executed, but queues all blocked functions to the end of the queue. This means that the time interval between two setInterval callback functions will be sacrificed (reduced).
Finally, when the second setInterval callback function is executed, we can see that there is no program waiting for the JavaScript engine to execute. This means that the browser is now waiting for a new asynchronous event to occur. At 50ms, a new setInterval callback function is triggered again. At this time, no execution block blocks its execution. So it will be executed immediately. Let us use an example to clarify the difference between setTimeout and setInterval:
setTimeout(function(){
/* Some long block of code... */
setTimeout(arguments.callee, 10);
}, 10);
setInterval(function(){
/* Some long block of code... */
}, 10);
setTimeout(function(){
/* Some long block of code... */
setTimeout(arguments.callee, 10);
}, 10);
setInterval(function(){
/* Some long block of code... */
}, 10);
There is no difference between these two codes at first glance, but they are different. The interval between the execution of the setTimeout callback function and the previous execution is at least 10ms (maybe more, but not less than 10ms), while the setInterval callback function will try to execute every 10ms, regardless of whether the last execution is completed.
Summary
◆The JavaScript engine is single-threaded, forcing all asynchronous events to be queued for execution;
◆setTimeout and setInterval are fundamentally different when executing asynchronous code ;
◆If a timer is blocked and cannot be executed immediately, it will delay execution until the next possible execution time point (which is longer than the expected time interval);
◆If the execution time of the setInterval callback function will be long enough (longer than the specified time interval), they will be executed continuously and there will be no time interval between each other.
The above knowledge points are very important. Understanding how the JavaScript engine works, especially when a large number of asynchronous events occur (continuously), can lay the foundation for building advanced applications.
Original author: John Resig
Original link: http://ejohn.org/blog/how-javascript-timers-work/