Simple code
Let’s look at a simple code first:
function HumanCloning(){
}
HumanCloning.prototype ={
name: 'Idiot's motto'
}
var clone01 = new HumanCloning();
alert( clone01.name);//'Idiot's motto'
alert(clone01 instanceof HumanCloning);//true
HumanCloning.prototype = {};
alert(clone01.name);//'Idiot's motto Motto'
alert(clone01 instanceof HumanCloning);//false
var clone02 = new HumanCloning();
alert(clone02.name);//undefined
alert(clone02 instanceof HumanCloning);/ /true
Complex theory
Only function objects (functions) in JS have the concept of classes, so to create an object, you must use a function object. There are [[Construct]] method and [[Call]] method inside the function object. [[Construct]] is used to construct the object, [[Call]] is used for function calling. [[Construct] is only triggered when using the new operator. ]logic. Note: In this example, the custom function HumanCloning is a function object, so are local objects such as Object, String, Number, etc. function objects? The answer is yes, because local objects can be regarded as derived types of functions. , in this sense, they can be treated equivalently to user-defined functions. (Echoes the "Built-in Data Types" section in "Understanding Javascript_04_Data Model")
var obj=new Object(); uses the built-in Object function object to create the instantiated object obj. var obj={}; and var obj=[]; This kind of code will trigger the construction process of Object and Array by the JS engine. function fn(){}; var myObj=new fn(); creates an instantiated object using a user-defined type.
Note: The specific concept of function objects will be explained in subsequent articles. Now "function object" can be simply understood as the concept of "function" and its internal implementation.
Based on this example, the function object is HumanCloning, and the object instances created by the function object are clone01 and clone02. Now let’s take a look at the implementation details of var clone01 = new HumanCloning(); (Note: [[ of the function object The Construct]] method handles the logic to implement the creation of the object):
1. Create a build-in object object obj and initialize it
2. If HumanCloning.prototype is of Object type, clone01’s internal [[Prototype ]] is set to HumanCloning.prototype, otherwise clone01’s [[Prototype]] will be its initialization value (i.e. Object.prototype)
3. Use clone01 as this and use the args parameter to call HumanCloning’s internal [[Call]] method
3.1 The internal [[Call]] method creates the current execution context (Note: The execution context will be explained in a subsequent blog post, and has been partially explained in the article "Javascript Speed Up_01_Reference Variable Optimization")
3.2 Call the function body of HumanCloning
3.3 Destroy the current execution context
3.4 Return the return value of the HumanCloning function body. If the function body of HumanCloning has no return value, it will return undefined
4. If [[Call]] If the return value is of Object type, this value is returned, otherwise obj is returned.
Note that the following code is the code explanation for step 1, step 2 and step 3:
var clone01 = {};
//[[prototype]] is inaccessible outside the program and is only used for understanding
//clone01.[[prototype]] = HumanCloning.prototype;
HumanCloning.call(clone01);
2단계에서 프로토타입은 객체가 표시하는 프로토타입 속성을 참조하고 [[Prototype]]은 객체의 내부 프로토타입 속성(암시적)을 나타냅니다. 객체의 프로토타입 체인을 구성하는 것은 객체의 명시적인 프로토타입 속성이 아니라 내부의 암시적 [[Prototype]]입니다. 표시된 프로토타입은 함수 객체에서만 의미가 있습니다. 위의 생성 과정에서 볼 수 있듯이 함수의 프로토타입은 이러한 방식으로 파생 객체의 암시적 [[Prototype]] 속성에 할당됩니다. 규칙, 파생 객체의 프로토타입 객체, 함수 사이에는 속성과 메소드의 상속/공유 관계만 존재합니다. (즉, 프로토타입 상속의 구현 원리는 "Javascript_05_Prototypal 상속 원리의 이해"의 내용입니다.)
3.4단계의 설명에 주목하고, Yi Fei의 질문을 살펴보겠습니다.
이제 이 질문에 대한 대답은 쉬울 것 같습니다.
참고: 원래 주소http://www.planabc.net/2008/02/20/javascript_new_function/
메모리 분석
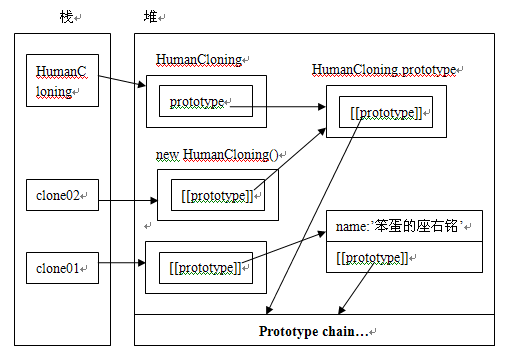
간단한 메모리 다이어그램과 함수 객체 개념의 도입도 위 코드를 설명합니다(비교적으로 다이어그램은 그다지 엄격하지는 않지만 이해하기 쉽습니다). 이는 또한 instanceof의 구현 원칙에 대한 의문을 제기합니다. 저는 모든 사람들이 몇 가지 징후를 본 적이 있다고 생각합니다. 구체적인 구현 세부 사항은 다음 블로그 게시물을 참조하세요.
로컬 속성 및 상속된 속성
객체는 암시적 프로토타입 체인을 통해 속성과 메서드를 상속할 수 있지만 프로토타입도 일반 객체입니다. 즉, 순전히 구조적 설명이 아닌 일반적인 인스턴스화된 객체입니다. . 따라서 지역 속성과 상속 속성에 문제가 있습니다.
먼저 객체 속성을 설정하는 과정을 살펴보겠습니다. JS는 속성이 JavaScript 코드에서 설정될 수 있는지, for in 등에 의해 열거될 수 있는지 여부를 나타내기 위해 객체의 속성을 설명하는 데 사용되는 속성 세트를 정의합니다.
obj.propName=value의 할당문을 처리하는 단계는 다음과 같습니다.
1. propName의 속성이 설정할 수 없는 경우
을 반환합니다. obj.propName이 존재하지 않는 경우. , obj에 대한 속성을 생성합니다. 이름은
입니다. obj.propName의 값을
값으로 설정합니다. 그 이유는 분명합니다. obj의 내부 [[Prototype]]은 인스턴스입니다. obj와 속성을 공유할 뿐만 아니라, 이를 수정하면 다른 객체에도 영향을 미칠 수 있습니다.
예제를 살펴보겠습니다.
function HumanCloning( ){
}
HumanCloning.prototype ={
name:'바보의 좌우명'
}
var clone01 = new HumanCloning()
clone01.name = 'jxl ';
alert(clone01.name);//jxl
var clone02 = new HumanCloning();
alert(clone02.name);//바보의 좌우명
결과는 훌륭합니다. 분명히 말하면, 객체의 속성은 프로토타입에 있는 동일한 이름의 속성을 수정할 수 없으며, 동일한 이름의 속성을 생성하고 여기에 값을 할당할 뿐입니다.
참고자료.
http://www.jb51.net/article/25008.htm