Note: Please read "Understanding javascript_13_Detailed Explanation of Execution Model" before reading this blog post
Note: Part of the content of this article is some of my own inferences, and there is no official document as a basis. If there are any errors, please correct me.
Jerky code
Let’s take a look at a relatively jerky code first:
function say(msg,other,garbage){
alert(arguments[1]);//world
var other = 'nice to meet you!' ;
var msg;
alert(arguments.length);
alert(msg);//hello
alert(other);//nice to meet you!
alert(arguments[ 1]);//nice to meet you!
alert(garbage);//undefined
}
say('hello','world');
Can you correctly explain the execution results of the code? Think about it. I think the result of running the code will be very different from your imagination! Why does msg normally output hello instead of undefined? What will happen if the parameters defined by the function and the variables defined inside the function are repeated? What is the relationship between arguments and parameters when defining a function? Let’s answer them one by one:
Simple memory map
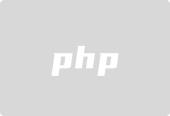
Note: The dotted line indicates the point that was once referenced.
Answer
First, let’s understand two concepts, formal parameters and actual parameters. Formal parameters refer to the parameters explicitly specified when defining a method. Due to the flexibility of the Javascript language, JavaScript does not require that the number of parameters passed when calling the method is consistent with the formal parameters. The parameters passed when JavaScript is actually called are actual parameters. arguments refers to the actual parameters. As can be seen from the say method, say defines three formal parameters, but only two values are passed when it is actually called. Therefore, the value of arguments.length is 2, not 3. Next, let’s take a look at the special behavior of arguments. Personally, I feel that arguments will treat all actual parameters as objects, and actual parameters of basic data types will be converted to Its corresponding object type. This is judged by defining a variable with the same name as the formal parameter in the function and assigning a value, and the value corresponding to the arguments will change accordingly.
Then let’s analyze the process of constructing the execution context of the say method. Since the logic is relatively complicated, I will write some 'pseudocode' here to illustrate:
function say(msg,other,garbage){
//First 'pre-parse' the variables declared by the function and execute it internally Process, it is invisible
var msg = undefined;
var other = undefined;
var garbage = undefined;
//Then 'pre-parse' the variables defined inside the function
var other = undefined;//Obviously, this definition is meaningless at this time.
var msg = undefined;//meaningless
//assign actual parameters
msg = new String('hello');//arguments will treat all actual parameters as objects
other = new String('world');
//Formally enter the function code part
alert(arguments[1]);//world
other = 'nice to meet you!';
//var msg; This has been pre-parsed, so it will not be executed again
alert(arguments.length);//2
alert(msg);//hello
alert(other) ;//nice to meet you!
alert(arguments[1]);//nice to meet you!
alert(garbage);//undefined
}
This code can already explain all the problems on one side. I won’t say much more.
The only point to emphasize is that it is meaningless to use var internally to define variables with the same names as formal parameters, because after the program is 'pre-parsed', they will be regarded as the same variable.
Others
There are many features about arguments. I mentioned arguments in the article "Pseudo Arrays". Interested readers can take a look. You can also refer to this article for the practical application of arguments:
http://www.gracecode.com/archives/2551/
Okay, that’s all. I hope everyone can correct me and give me more opinions.