But unfortunately, the data returned by many services is still in XML format.
jquery has built-in support for processing data such as xml, and there is no problem with this. But the premise is that the returned data does not have any namespace. For example, the following data
< /Employee>
To process such data, the jquery code is roughly as follows
var div = $("#placeholder");
// Process xml without namespace
$.get("data.xml", null, function (data) {
var employees = $("Employee", data); //Find all Employee nodes
var ul = $("
");
employees.each(function ( ) {
$("
").text($(this).attr("firstName") " " $(this).attr("lastName")).appendTo(ul); // Construct a new li tag for each row of data and insert it into ul
});
ul.appendTo(div);
});
But if our XML data has a namespace, the above code will be invalid. The reason is that jquery cannot handle the namespace
xml version="1.0" encoding="utf-8" ?>
< d:Employee id="1" firstName="bill" lastName="gates">
In order to solve this problem, some enthusiastic netizens, I wrote a jquery plug-in called jquery.xmlns.js. If you are interested, you can learn about and download it from the following
http://www.rfk.id.au/blog/entry/xmlns-selectors-jquery/Then, we can use the following method to solve the problem
$.xmlns["d"] = "http://tech.xizhang.com";
// Process xml with namespace
$.get("datawithnamespace.xml", null , function (data) {
var employees = $("d|Employee", data); //Find all Employee nodes
var ul = $("
");
employees.each(function () {
$("
").text($(this).attr("firstName") " " $(this).attr("lastName") ).appendTo(ul);
});
ul.appendTo(div);
});
I have to say that the namespace in the XML technical specification is really a very bad design. It adds more trouble than it brings.
The complete code of the example in this article is as follows
<% @ Page Language="C#" AutoEventWireup="true" CodeBehind="WebForm1.aspx.cs" Inherits="WebApplication1.WebForm1" %>
< ;script language="javascript" type="text/javascript">
$(function () {
var div = $("#placeholder");
// Processing without namespace xml
$.get("data.xml", null, function (data) {
var employees = $("Employee", data); //Find all Employee nodes
var ul = $ ("
");
employees.each(function () {
$("
").text($(this).attr("firstName") " " $(this).attr("lastName")).appendTo(ul);// Construct a new li tag for each row of data and insert it into ul
});
ul .appendTo(div);
});
$("
").appendTo(div);
$.xmlns["d"] = "http://tech .xizhang.com";
// Process xml with namespace
$.get("datawithnamespace.xml", null, function (data) {
var employees = $("d|Employee" , data); //Find all Employee nodes
var ul = $("
");
employees.each(function () {
$("
").text($(this).attr("firstName") " " $(this).attr("lastName")).appendTo(ul);
});
ul.appendTo (div);
});
});
html>
Finally, the effect seen in the browser is as follows. There are pictures and the truth
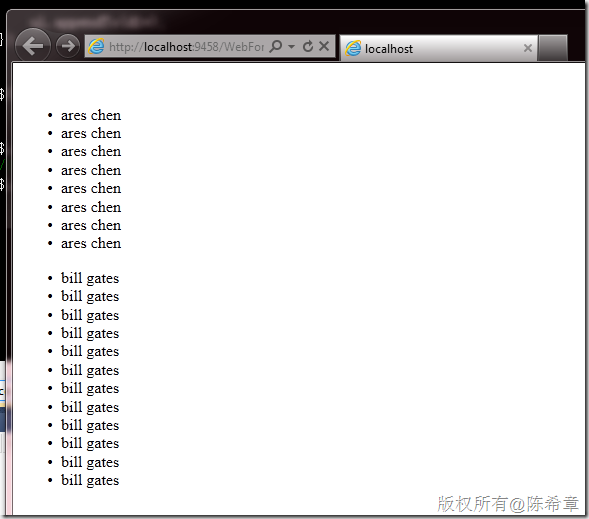