Foreword
Currently, unit testing is receiving more and more attention from developers in software development. It can improve the efficiency of software development and ensure the quality of development. In the past, unit testing was often seen in server-side development, but as the division of labor in the field of Web programming gradually becomes clearer, relevant unit testing can also be carried out in the field of front-end Javascript development to ensure the quality of front-end development.
In server-side unit testing, there are various testing frameworks. There are also some excellent frameworks in JavaScript. But in this article, we will implement a simple unit testing framework step by step. .
JS unit testing has many aspects, the most common ones are method function checking and browser compatibility checking. This article mainly talks about the first type.
The JS code examined in this article is a JS date formatting method I wrote before. The original text is here (javascript date formatting function, similar to the usage in C#). The code is as follows:
Date.prototype.toString=function(format){
var time ={};
time.Year=this.getFullYear();
time.TYear=("" time.Year).substr(2);
time.Month=this.getMonth() 1 ;
time.TMonth=time.Month<10?"0" time.Month:time.Month;
time.Day=this.getDate();
time.TDay=time.Day<10 ?"0" time.Day:time.Day;
time.Hour=this.getHours();
time.THour=time.Hour<10?"0" time.Hour:time.Hour;
time.hour=time.Hour<13?time.Hour:time.Hour-12;
time.Thour=time.hour<10?"0" time.hour:time.hour;
time .Minute=this.getMinutes();
time.TMinute=time.Minute<10?"0" time.Minute:time.Minute;
time.Second=this.getSeconds();
time .TSecond=time.Second<10?"0" time.Second:time.Second;
time.Millisecond=this.getMilliseconds();
var oNumber=time.Millisecond/1000;
if( format!=undefined && format.replace(/s/g,"").length>0){
format=format
.replace(/yyyy/ig,time.Year)
.replace( /yyy/ig,time.Year)
.replace(/yy/ig,time.TYear)
.replace(/y/ig,time.TYear)
.replace(/MM/g, time.TMonth)
.replace(/M/g,time.Month)
.replace(/dd/ig,time.TDay)
.replace(/d/ig,time.Day)
.replace(/HH/g,time.THour)
.replace(/H/g,time.Hour)
.replace(/hh/g,time.Thour)
.replace( /h/g,time.hour)
.replace(/mm/g,time.TMinute)
.replace(/m/g,time.Minute)
.replace(/ss/ig, time.TSecond)
.replace(/s/ig,time.Second)
.replace(/fff/ig,time.Millisecond)
.replace(/ff/ig,oNumber.toFixed(2 )*100)
.replace(/f/ig,oNumber.toFixed(1)*10);
}
else{
format=time.Year "-" time.Month "- " time.Day " " time.Hour ":" time.Minute ":" time.Second;
}
return format;
}
This code currently does not exist A serious bug was found. For testing in this article, we changed .replace(/MM/g,time.TMonth) to .replace(/MM/g,time.Month). This error is useless when the month is less than 10. Two digits represent the month.
There is a saying now that good designs are refactored. It is the same in this article. Let’s start with the simplest one.
First version: Use the original alert
As the first version, we are very lazy and directly use alert to check. The complete code is as follows:
Demo
运行后会弹出 2012 和 4 ,观察结果我们知道 date.toString("MM")方法是有问题的。
这种方式很不方便,最大的问题是它只弹出了结果,并没有给出正确或错误的信息,除非对代码非常熟悉,否则很难知道弹出的结果是正是误,下面,我们写一个断言(assert)方法来进行测试,明确给出是正是误的信息。
第二版:用assert进行检查
断言是表达程序设计人员对于系统应该达到状态的一种预期,比如有一个方法用于把两个数字加起来,对于3 2,我们预期这个方法返回的结果是5,如果确实返回5那么就通过,否则给出错误提示。
断言是单元测试的核心,在各种单元测试的框架中都提供了断言功能,这里我们写一个简单的断言(assert)方法:
function assert(message,result){
if(!result){
throw new Error(message);
}
return true;
}
这个方法接受两个参数,第一个是错误后的提示信息,第二个是断言结果
用断言测试代码如下:
var date=new Date(2012,3,9);
try{
assert("yyyy should return full year",date.toString("yyyy")==="2012");
}catch(e){
alert("Test failed:" e.message);
}
try{
assert("MM should return full month",date.toString("MM")==="04");
}
catch(e){
alert("Test failed:" e.message);
}
运行后会弹出如下窗口:
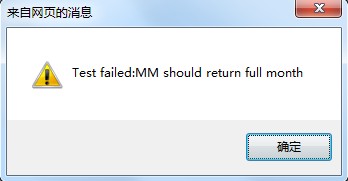
第三版:进行批量测试 在第二版中,assert方法可以给出明确的结果,但如果想进行一系列的测试,每个测试都要进行异常捕获,还是不够方便。另外,在一般的测试框架中都可以给出成功的个数,失败的个数,及失败的错误信息。
为了可以方便在看到测试结果,这里我们把结果用有颜色的文字显示的页面上,所以这里要写一个小的输出方法PrintMessage:
function PrintMessage(text,color){
var div=document.createElement("div");
div.innerHTML=text;
div.style.color=color;
document.body.appendChild(div);
delete div;
}
Next, we will write a TestCase method similar to jsTestDriver to perform batch testing:
function testCase(name,tests){
var successCount= 0;
var testCount=0;
for(var test in tests){
testCount ;
try{
tests[test]();
PrintMessage(test " success" ,"#080");
successCount ;
}
catch(e){
PrintMessage(test " failed:" e.message,"#800");
}
}
PrintMessage("Test result: " testCount " tests," successCount " success, " (testCount-successCount) " failures","#800");
}
Test code:
var date=new Date(2012,3 ,9);
testCase("date toString test",{
yyyy:function(){
assert("yyyy should return 2012",date.toString("yyyy")==="2012 ");
},
MM:function(){
assert("MM should return 04",date.toString("MM")==="04");
},
dd:function(){
assert("dd should return 09",date.toString("dd")==="09");
}
});
The result is:
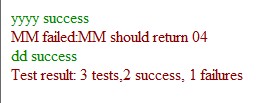
This way we can see at a glance which one is wrong. But is this perfect? We can see that in the last test, var date=new Date(2012,3,9) is defined outside testCase, and date is shared in the entire testCase test code. Because of the various The date value is not modified in the method, so there is no problem. If the date value is modified in a test method, the test results will be inaccurate, so many test frameworks provide setUp and tearDown methods. , used to provide and destroy test data uniformly. Next, we will add the setUp and tearDown methods to our testCase.
Version 4: Batch testing that provides test data uniformly
First we add the setUp and tearDown methods:
testCase("date toString",{
setUp:function(){
this.date=new Date(2012,3,9);
},
tearDown:function(){
delete this.date;
},
yyyy:function(){
assert("yyyy should return 2012",this. date.toString("yyyy")==="2012");
},
MM:function(){
assert("MM should return 04",this.date.toString("MM ")==="04");
},
dd:function(){
assert("dd should return 09",this.date.toString("dd")===" 09");
}
});
Since the setUp and tearDown methods do not participate in the test, we need to modify the testCase code:
function testCase(name,tests){
var successCount=0;
var testCount=0 ;
var hasSetUp=typeof tests.setUp == "function";
var hasTearDown=typeof tests.tearDown == "function";
for(var test in tests){
if(test ==="setUp"||test==="tearDown"){
continue;
}
testCount;
try{
if(hasSetUp){
tests.setUp ();
}
tests[test]();
PrintMessage(test " success","#080");
if(hasTearDown){
tests.tearDown ();
}
successCount ;
}
catch(e){
PrintMessage(test " failed:" e.message,"#800");
}
}
PrintMessage("Test result: " testCount " tests," successCount " success, " (testCount-successCount) " failures","#800");
}
The result after running is the same as the third version.
Summary and reference articles
As mentioned above, good design is the result of continuous reconstruction. Is the fourth version above perfect? It is far from it. Here is just an example. If you need knowledge in this area, I can write about the use of each testing framework later.
This article is just an entry-level example of JS unit testing. It allows beginners to have a preliminary concept of JS unit testing. It is an introduction to the topic. Experts are welcome to add their suggestions.
This article refers to the first chapter of the book "Test-Driven JavaScript Development" (I think it is pretty good, I recommend it). The test case in the book is also a time function, but it is more complicated to write and is not suitable for beginners. Too easy to understand.
Author: Artwl