


HTML example to be used in this section
- Project one
- Project two
- Project three
1. Create element node
The document.createElement() method is used to create elements. It accepts a parameter, which is the tag name of the element to be created, and returns the created element. Node
var div = document.createElement("div") ; //Create a div element
div.id = "myDiv"; //Set the div's id
div.className = "box"; //Set the div's class
After creating the element, you must add the element to the document tree
2. Add element node
The appendChild() method is used to add a node to the end of the childNodes list and return the element node to be added
var ul = document.getElementById("myList"); //Get ul
var li = document.createElement("li"); //Create li
li.innerHTML = "Item 4"; //Add text to li
ul.appendChild(li); / /Add li to the end of the ul child node
After adding:
- Project One
- Project Two
- Project Three
- Project Four
appendChild() method can also be added Elements that already exist will be moved from the original position to the new position
var ul = document.getElementById("myList"); //Get ul
ul.appendChild(ul.firstChild); //Move the first element node of ul to the end of the ul child node
After running (IE):
- Project Two
- Project Three
- Project One
insertBefore() method, if you do not insert the node at the end, but want to place it at a specific position, use this method, this method Accepts 2 parameters, the first is the node to be inserted, the second is the reference node, returns the element node to be added
var ul = document.getElementById("myList"); //Get ul
var li = document.createElement("li"); //Create li
li.innerHTML= "Item Four"; //Add text to li
ul.insertBefore(li,ul.firstChild); //Add li before the first child node of ul
After adding:
- Item four
- Item one
- Item two
- Project 3
var ul = document.getElementById("myList"); //Get ul
var li = document.createElement("li"); //Create li
li.innerHTML= "Item 4"; //Add text to li
ul.insertBefore(li,ul.null); //Add li to the end of the child node of ul
After adding:
- Item one
- Item two
- Item three
< ;li>Project 4
var ul = document.getElementById("myList"); //Get ul
var li = document.createElement("li"); //Create li
li.innerHTML= "Item 4"; //Add text to li
var lis = ul.getElementsByTagName("li") //Get the collection of all li's in ul
ul.insertBefore(li,lis[ 1]); //Add li before the second li node in ul
After adding:
- Project 1
- Item 4
- Item 2
- Item 3
removeChild() method, used to remove nodes, accepts a parameter, which is the node to be removed, and returns the removed node. Note that the removed node is still in the document , but its position is no longer in the document
ul.removeChild(lis[0]); //Remove the first li, Different from the above, you need to consider the differences between browsers
4. Replace element node
replaceChild() method, used to replace nodes, accepts two parameters, the first parameter is to insert node, the second one is the node to be replaced, return the replaced node
li.innerHTML= "Item Four"; //Add to li Text
var lis = ul.getElementsByTagName("li") //Get the collection of all li in ul
var returnNode = ul.replaceChild(li,lis[1]); //Replace the original with the created li The second li
5. Copy node
cloneNode() method, used to copy nodes, accepts a Boolean parameter, true means deep copy (copy the node and all its child nodes) , false means shallow copy (copy the node itself, do not copy the child nodes)
When operating nodes, please pay attention to the differences between IE and other browsers (discussed in Section 18)
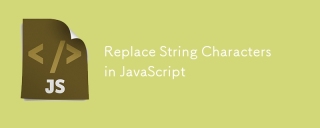
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
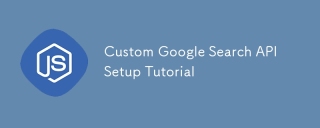
This tutorial shows you how to integrate a custom Google Search API into your blog or website, offering a more refined search experience than standard WordPress theme search functions. It's surprisingly easy! You'll be able to restrict searches to y
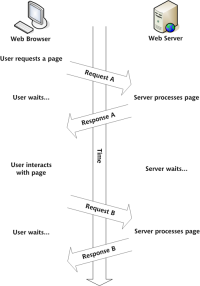
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
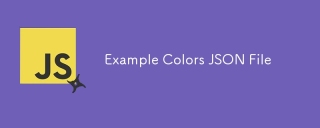
This article series was rewritten in mid 2017 with up-to-date information and fresh examples. In this JSON example, we will look at how we can store simple values in a file using JSON format. Using the key-value pair notation, we can store any kind
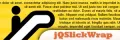
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
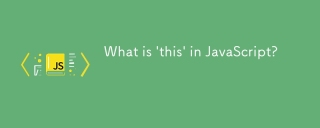
Core points This in JavaScript usually refers to an object that "owns" the method, but it depends on how the function is called. When there is no current object, this refers to the global object. In a web browser, it is represented by window. When calling a function, this maintains the global object; but when calling an object constructor or any of its methods, this refers to an instance of the object. You can change the context of this using methods such as call(), apply(), and bind(). These methods call the function using the given this value and parameters. JavaScript is an excellent programming language. A few years ago, this sentence was

jQuery is a great JavaScript framework. However, as with any library, sometimes it’s necessary to get under the hood to discover what’s going on. Perhaps it’s because you’re tracing a bug or are just curious about how jQuery achieves a particular UI
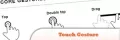
This post compiles helpful cheat sheets, reference guides, quick recipes, and code snippets for Android, Blackberry, and iPhone app development. No developer should be without them! Touch Gesture Reference Guide (PDF) A valuable resource for desig


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 Linux new version
SublimeText3 Linux latest version
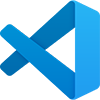
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Atom editor mac version download
The most popular open source editor
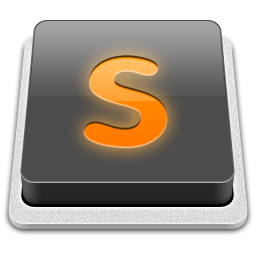
SublimeText3 Mac version
God-level code editing software (SublimeText3)
