JavaScript study notes (10) js object inheritance_basic knowledge
1. Prototype chain
//Rarely used alone
View Code
//Define the SuperClass class, which has an attribute property and a method getSuperValue
function SuperClass() {
this.property = true;
}
SuperClass.prototype.getSuperValue = function() {
return this.property;
}
//Define the SubClass class, which has an attribute subproperty and a method getSubValue added later
function SubClass() {
this.subproperty = false;
}
//SubClass class inherits SuperClass class
SubClass.prototype = new SuperClass();
//SubClass class adds a method getSubValue
SubClass.prototype.getSubValue = function() {
return this.subproperty;
}
//Create an instance of the SubClass class
var instance = new SubClass();
alert(instance.getSuperValue());
2. Determine the relationship between the prototype and the instance
The first way is to use the instanceof operator to test the instance and prototype chain. Constructor
alert(instance instanceof Object); //true , is instance an instance of Object?
alert(instance instanceof SuperClass); //true, is instance an instance of SuperClass?
alert(instance instanceof SubClass); //true, is instance an instance of SubClass?
The second way is to use the isPrototypeOf() method to test the prototype that appears in the prototype chain
alert(Object.prototype.isPrototypeOf(instance)); //true
alert(SuperClass.prototype.isPrototypeOf(instance)); //true
alert(SubClass.prototype.isPrototypeOf(instance)); //true
3. Points to note when defining methods using prototype chain inheritance
The order in which methods are defined:
View Code
function SuperClass() {
this.property = true;
}
SuperClass.prototype.getSuperValue = function() {
return this.property;
}
function SubClass() {
this.subproperty = false ;
}
//SubClass inherits SuperClass
SubClass.prototype = new SuperClass(); //This should be written first, and the newly added methods and methods of overriding the superclass should be written later , otherwise the overridden superclass method will never be able to call
//Add new method
SubClass.prototype.getSubValue = function() {
return this.subproperty;
}
//Override the super class method
SubClass.prototype.getSuperValue = function() {
return false;
}
var instance = new SubClass();
alert(instance. getSuperValue()); //fales, the instance of SubClass here calls the getSuperValue() method of SubClass, but blocks the getSuperValue() method of SuperClass.
//Using the SuperClass method will call the getSuperValue() method of SuperClass
Disadvantages of prototype chain inheritance: 1) Sharing properties in the super class, 2) You cannot pass parameters to the constructor of the super class when creating a subclass. All prototype chains are rarely used alone
4. Borrowing constructors
//Rarely used alone
Advantages: Parameters can be passed to the super class. Disadvantages: functions cannot be reused, all classes must use the constructor pattern
View Code
function SuperClass(name) {
this.name = name;
}
function SubClass(){
SuperClass.call(this,"RuiLiang"); / /Inherited SuperClass and passed parameters to SuperClass
this.age = 29; //Instance attributes
}
var instance = new SubClass();
alert(instance.name ); //RuiLiang
alert(instance.age); //29
6. Combination inheritance
//The most commonly used inheritance pattern
View Code
//Create SuperClass
function SuperClass(name) {
this.name = name;
this.colors = ["red","blue"," green"];
}
SuperClass.prototype.sayName = function() {
alert(this.name);
}
////Create SubClass
function SubClass(name,age) {
SuperClass.call(this,name); //Inherited attributes
this.age = age; //Own attributes
}
SubClass. prototype = new SuperClass(); //Inherited method
SubClass.prototype.sayAge = function() { //SubClass adds new method
alert(this.age);
};
//Use
var instance1 = new SubClass("RuiLiang",30);
instance1.colors.push("black");
alert(instance1.colors); //"red,blue ,green,black"
instance1.sayName(); //"RuiLiang"
instance1.sayAge(); //30
var instance2 = new SubClass("XuZuNan",26);
alert(instance2.colors); //"red,blue,green"
instance2.sayName(); //"RuiLiang"
instance2.sayAge(); //30
7. Other inheritance patterns
Prototypal inheritance, parasitic inheritance, parasitic combined inheritance
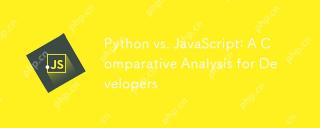
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
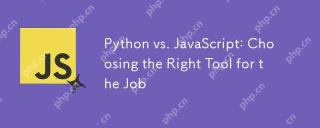
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
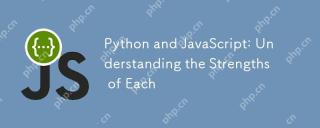
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
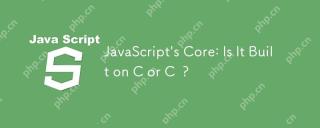
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
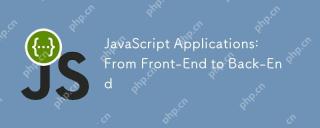
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
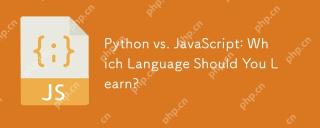
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
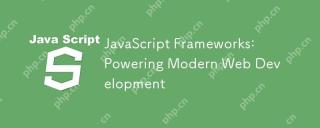
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
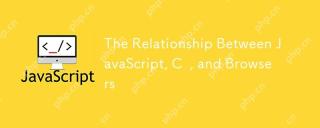
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 English version
Recommended: Win version, supports code prompts!
