


Automate Repetitive Tasks via AI-Generated PowerShell Scripts - Make Tech Easier
I've always held the belief that computers should serve us, rather than the reverse. This belief was tested when I found myself dedicating endless hours to repetitive tasks. However, this changed when I began leveraging artificial intelligence (AI) to seamlessly generate PowerShell scripts for automating my Windows tasks.
Table of Contents
- PowerShell Fundamentals: Automating Windows Tasks
- Utilizing AI to Craft Tailored PowerShell Scripts
- Scheduling Your AI-Generated PowerShell Scripts with Task Scheduler
PowerShell Fundamentals: Automating Windows Tasks
PowerShell serves as both a command-line shell and a scripting language integrated into Windows, offering robust capabilities for system administration and automation, as described by Microsoft.
In practice, you can develop scripts—files that contain commands and instructions for automatic execution—ranging from basic file operations to sophisticated system management tasks.
Numerous pre-existing PowerShell scripts are available online for common tasks. For instance, the PowerShell Scripts Repository hosts over 500 free scripts that address tasks from adjusting system settings to automating file operations. These scripts can monitor drive space, catalog installed software, and even categorize photos by date.
Yet, the real potential of PowerShell is realized when you design custom scripts that meet your specific needs, and this is where AI proves invaluable.
Utilizing AI to Craft Tailored PowerShell Scripts
Let me share how I use AI to generate PowerShell scripts for automating tasks without needing to master PowerShell, illustrated with a practical example.
Recently, I used PhotoRec to recover data from a failing drive, resulting in thousands of files in a single directory, making manual sorting a daunting task. I wanted to automatically sort these files into major categories such as images, audio, documents, and others.
Instead of spending hours learning PowerShell or searching for the ideal pre-made script, I turned to ChatGPT with a detailed description of my needs. Here’s how I framed my request to the AI:
I ensured my prompt included:
- What to do with files that don’t fit my categories
- The exact task I wanted to achieve (organizing files by extension)
- The specific categories and file types for each category
I made it clear in my AI prompt that I was looking for a PowerShell script, not, for example, a Bash script.
Within moments, the AI produced this PowerShell script to automate tasks for me:
<code># Define the source folder as the current directory where the script is executed<br>$sourceFolder = $PSScriptRoot<br><br># Define category folders and their associated file extensions<br>$categories = @{<br> "Images" = @(".jpg", ".jpeg", ".png", ".gif", ".bmp", ".tiff", ".webp", ".svg", ".ico", ".raw", ".cr2", ".nef", ".heic", ".psd", ".ai", ".eps")<br> "Documents" = @(".pdf", ".docx", ".doc", ".txt", ".rtf", ".xlsx", ".xls", ".pptx", ".ppt", ".odt", ".ods", ".odp", ".md", ".csv", ".json", ".xml", ".html", ".htm", ".log", ".tex", ".epub", ".mobi")<br> "Audio" = @(".mp3", ".wav", ".aac", ".flac", ".ogg", ".wma", ".m4a", ".mid", ".midi", ".aiff", ".alac", ".ape", ".opus", ".amr", ".au", ".voc")<br> "Video" = @(".mp4", ".avi", ".mov", ".wmv", ".mkv", ".flv", ".webm", ".m4v", ".mpg", ".mpeg", ".3gp", ".3g2", ".ts", ".vob", ".ogv", ".divx", ".asf", ".rm", ".rmvb")<br> "Archives" = @(".zip", ".rar", ".7z", ".tar", ".gz", ".bz2", ".xz", ".cab", ".iso", ".tgz", ".tbz2", ".lzh", ".dmg", ".pkg", ".deb", ".rpm")<br> "Code" = @(".py", ".js", ".html", ".css", ".java", ".c", ".cpp", ".cs", ".php", ".rb", ".go", ".swift", ".sql", ".ps1", ".sh", ".bat", ".vbs", ".ts", ".jsx", ".r", ".pl", ".lua")<br> "Executables" = @(".exe", ".msi", ".app", ".apk", ".dll", ".bin", ".com", ".jar", ".gadget", ".wsf", ".appx", ".appxbundle")<br> "Other" = @()<br>}<br><br># Create category folders if they don't exist<br>foreach ($category in $categories.Keys) {<br> $categoryPath = Join-Path -Path $sourceFolder -ChildPath $category<br> if (-not (Test-Path -Path $categoryPath)) {<br> New-Item -Path $categoryPath -ItemType Directory | Out-Null<br> Write-Host "Created folder: $category"<br> }<br>}<br><br># Get all files in the source folder (non-recursive)<br>$files = Get-ChildItem -Path $sourceFolder -File | Where-Object { $_.Name -ne $MyInvocation.MyCommand.Name }<br><br># Counter for moved files<br>$movedFiles = 0<br>$totalFiles = $files.Count<br><br>Write-Host "Starting to organize $totalFiles files..."<br><br># Process each file<br>foreach ($file in $files) {<br> $extension = $file.Extension.ToLower()<br> $targetCategory = "Other"<br><br> # Determine which category the file belongs to<br> foreach ($category in $categories.Keys) {<br> if ($category -eq "Other") { continue }<br> if ($categories[$category] -contains $extension) {<br> $targetCategory = $category<br> break<br> }<br> }<br><br> # Move the file to the appropriate category folder<br> $targetPath = Join-Path -Path $sourceFolder -ChildPath $targetCategory<br> $targetFile = Join-Path -Path $targetPath -ChildPath $file.Name<br><br> # Check if target file already exists and handle accordingly<br> if (Test-Path -Path $targetFile) {<br> $baseName = [System.IO.Path]::GetFileNameWithoutExtension($file.Name)<br> $newName = "$baseName`_$(Get-Random)$extension"<br> $targetFile = Join-Path -Path $targetPath -ChildPath $newName<br> }<br><br> Move-Item -Path $file.FullName -Destination $targetFile<br> $movedFiles <br><br> # Show progress<br> $percentComplete = [math]::Round(($movedFiles / $totalFiles) * 100, 2)<br> Write-Progress -Activity "Organizing Files" -Status "$movedFiles of $totalFiles files processed ($percentComplete%)" -PercentComplete $percentComplete<br>}<br><br>Write-Host "File organization complete! Moved $movedFiles files into categories."</code>
The script is thoroughly commented, making it easier to understand and modify if you wish to learn PowerShell and make manual adjustments.
To transform this code into a functional script, I simply copied the entire code block from the AI chat and opened Windows Notepad (or any text editor).
After pasting the code, I saved the file with a descriptive name and the .ps1 extension (such as "organize-files.ps1"). I placed this script directly in the folder with my recovered files and executed it by right-clicking on the file and selecting Run with PowerShell.
In no time, thousands of scattered files were neatly organized into appropriate folders.
Scheduling Your AI-Generated PowerShell Scripts with Task Scheduler
An AI-generated PowerShell script that automates your Windows tasks without requiring manual intervention is even more valuable!
I spend long hours working on my computer and often forget to take breaks, leading to eye strain and reduced productivity. I decided to create a script that would remind me to take screen breaks at regular intervals. Again, I sought help from AI with a clear request:
The AI swiftly generated this eye-saving script:
<code># Script to remind user to take regular screen breaks<br><br># Load required assemblies for notifications<br>Add-Type -AssemblyName System.Windows.Forms<br><br># Function to show break reminder notification<br>function Show-BreakReminder {<br> $motivationalMessages = @(<br> "Time for a 5-minute break! Rest your eyes and stretch.",<br> "Screen break time! Look at something 20 feet away for 20 seconds.",<br> "Break time! Stand up and move around for 5 minutes.",<br> "Your eyes need a rest! Take 5 minutes away from the screen.",<br> "Productivity hack: A 5-minute break now will boost your focus!"<br> )<br><br> # Select a random message<br> $randomMessage = $motivationalMessages | Get-Random<br><br> # Create and configure the notification<br> $notification = New-Object System.Windows.Forms.NotifyIcon<br> $notification.Icon = [System.Drawing.SystemIcons]::Information<br> $notification.BalloonTipTitle = "Wellness Reminder"<br> $notification.BalloonTipText = $randomMessage<br> $notification.Visible = $true<br><br> # Show notification for 10 seconds<br> $notification.ShowBalloonTip(10000)<br><br> # Clean up after a delay<br> Start-Sleep -Seconds 12<br> $notification.Dispose()<br>}<br><br># Display an initial notification<br>Show-BreakReminder<br><br>Write-Host "Break reminder displayed. Set this script to run hourly using Task Scheduler."</code>
This script was exactly what I needed, but I wanted it to run automatically every hour without my intervention. To achieve this, I used Windows Task Scheduler.
I saved the AI-generated PowerShell script as a .ps1 file, then opened Task Scheduler from the Start menu and created a new basic task with a daily trigger.
After setting up the basic task, I modified the trigger settings for hourly execution. In the Task Scheduler Library, I located my newly created task and right-clicked to select Properties. I went to the Triggers tab and selected Edit. In the Edit Trigger window, I checked the Repeat task every: option and set the repeat interval to 1 hour. Under duration, I selected Indefinitely, then clicked OK to save these settings.
I also needed to configure the action settings properly. In the Properties window, I navigated to the Actions tab and selected Edit. For the Program/script field, I entered powershell.exe instead of the direct path to my script. In the Add arguments field, I entered -ExecutionPolicy Bypass -WindowStyle Hidden -File "C:\Users\David\Desktop\eye-saver.ps1", which includes both the execution parameters and the full path to my script.
After making these adjustments, I clicked OK to save the action settings, then clicked OK again on the Properties window to finalize all changes. The effort was well worth it!
The advantage of this AI-driven approach is that once you’ve set up a few automated tasks via PowerShell scripts, your computer begins to work for you in the background, providing those helpful reminders that previously required you to remember or set external alarms.
If you're keen on exploring more PowerShell capabilities for your automation projects, check out our guide on essential PowerShell commands that every Windows user should be familiar with. These commands are the foundation of more complex scripts and will help you understand the mechanics behind your AI-generated automation.
All images and screenshots by David Morelo.
The above is the detailed content of Automate Repetitive Tasks via AI-Generated PowerShell Scripts - Make Tech Easier. For more information, please follow other related articles on the PHP Chinese website!
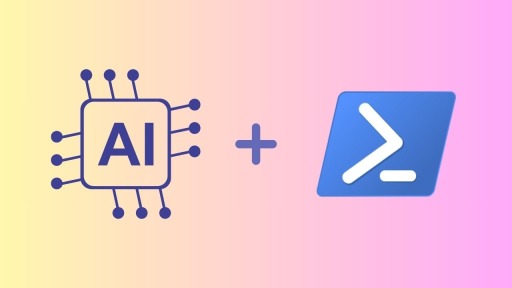
I've always held the belief that computers should serve us, rather than the reverse. This belief was tested when I found myself dedicating endless hours to repetitive tasks. However, this changed when I began leveraging artificial intelligence (AI) t
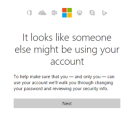
Similar to other large companies, Microsoft prioritizes your account security and protection from unauthorized access by individuals with harmful intentions.If Microsoft detects an unusual login attempt, it marks it as suspicious. You will receive an
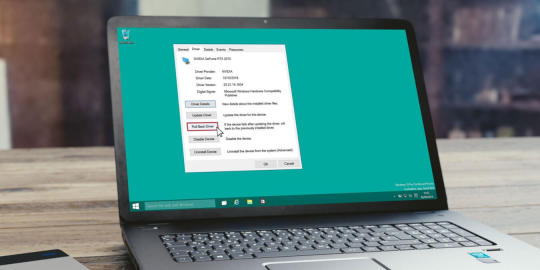
Driver issues are quite common in Windows systems. Sometimes, updates to new drivers may cause a Blue Screen of Death (BSOD) error message in Windows. Fortunately, this problem can be solved by rolling back the driver. You can use the Rollback Driver feature to restore the driver update to a previous version to check if it is functioning properly. Here is a detailed guide on how to roll back drivers in Windows. Directory Rollback Driver in Windows What to do if the Rollback Driver option is disabled? FAQ Rollback Driver in Windows Windows comes with some built-in tools designed to detect and resolve possible conflicts in the operating system. This pack
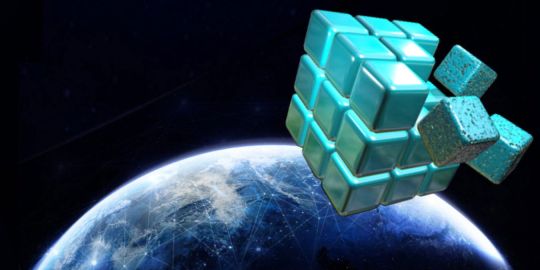
The Windows Registry is a central hub for storing all configurations related to the Windows operating system and its software. This is why numerous Windows tutorials often involve adding, modifying, or deleting Registry keys.However, you may encounte
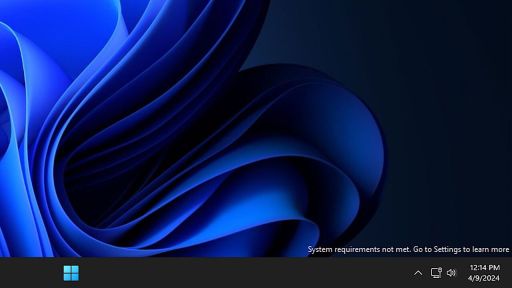
Windows 11 does have strict installation requirements. However, installing Windows 11 on unsupported devices is not difficult. If you have successfully installed it, don't rush to celebrate. You also need to clear the desktop "System Requirements Not Meeted" watermark that Microsoft introduced to prevent installation on unsupported hardware. This guide lists three ways to remove this watermark. Directory Group Policy Editor Windows Registry Editor Script Group Policy Editor If you are using Windows Pro or Enterprise and you have Group Policy Editor enabled, this method is the easiest. Follow the instructions below to disable the watermark through the Group Policy Editor. Enter "Group Policy" in Windows Search and click Edit Group in the results
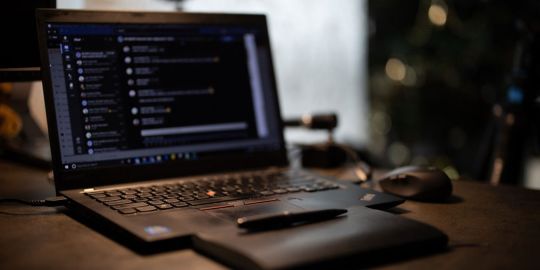
Microsoft Teams is a widely used platform for collaboration and communication within organizations. Despite its effectiveness, you might occasionally face issues with the camera during calls. This guide offers a range of solutions to resolve the came

If you plan to upgrade your RAM or test its performance, it is important to know your RAM type. This means that your laptop or PC needs to be evaluated to determine the DDR module it supports, as well as other details like the form, speed and capacity of RAM. This tutorial shows how to check RAM types using various Windows applications and third-party tools in Windows. Directory Check RAM type via command prompt Check RAM type via task manager Check RAM type in Windows Check RAM type in PowerShell Check RAM type using CPU-Z Check RAM type using Novabench Check RAM type via visual inspection of motherboard Check RAM type via command prompt Check RAM type
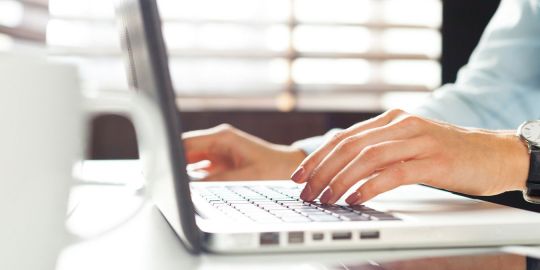
Local Security Authority (LSA) protection is a crucial security feature designed to safeguard a user's credentials on a Windows computer, preventing unauthorized access. Some users have encountered an error message stating that "Local Security A


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
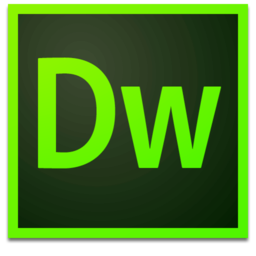
Dreamweaver Mac version
Visual web development tools

SublimeText3 Chinese version
Chinese version, very easy to use

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!
