Laravel migrations streamline database management by allowing schema changes to be defined in PHP code, which can be version-controlled and shared. Here's how to use them: 1) Create migration classes to define operations like creating or modifying tables. 2) Use the 'php artisan migrate' command to apply migrations and 'php artisan migrate:rollback' to reverse them. 3) Follow best practices such as testing in development and using seeders for initial data.
Laravel migrations are an essential tool for managing database schema changes in a clean and version-controlled manner. If you've ever found yourself manually altering your database structure, you know how tedious and error-prone it can be. Migrations solve this by allowing you to define your database schema in PHP code, which can be version controlled and easily shared across your development team. Let's dive into how you can leverage Laravel migrations to streamline your database management process.
When I first started using Laravel, I was amazed at how migrations could simplify my workflow. No more SQL scripts scattered around my project or worrying about whether my teammates had the latest schema changes. Migrations became my go-to solution for database management, and I'm excited to share with you how to harness their power effectively.
To start using Laravel migrations, you'll need to understand a few key concepts. Migrations are essentially classes that represent a set of database operations. These operations can be creating tables, modifying existing ones, or even dropping them. The beauty of migrations lies in their reversibility; you can roll back changes if needed, which is incredibly useful during development.
Let's jump into a practical example to see how this works. Suppose you want to create a new table for storing user information. Here's how you'd do it:
use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; use Illuminate\Support\Facades\Schema; class CreateUsersTable extends Migration { public function up() { Schema::create('users', function (Blueprint $table) { $table->id(); $table->string('name'); $table->string('email')->unique(); $table->timestamp('email_verified_at')->nullable(); $table->string('password'); $table->rememberToken(); $table->timestamps(); }); } public function down() { Schema::dropIfExists('users'); } }
This migration class defines the structure of the users
table. The up
method is where you specify what to do when the migration is run, and the down
method defines how to reverse those changes. Running this migration will create the users
table with the specified columns.
Now, to run this migration, you'd use the following Artisan command:
php artisan migrate
This command will execute all pending migrations, bringing your database schema up to date. If you need to roll back the last migration, you can use:
php artisan migrate:rollback
One of the things I love about Laravel migrations is their flexibility. You can modify existing tables just as easily. Let's say you want to add a new column to the users
table for storing a user's phone number:
use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; use Illuminate\Support\Facades\Schema; class AddPhoneToUsersTable extends Migration { public function up() { Schema::table('users', function (Blueprint $table) { $table->string('phone')->nullable()->after('email'); }); } public function down() { Schema::table('users', function (Blueprint $table) { $table->dropColumn('phone'); }); } }
This migration will add a phone
column to the users
table when run. The after
method ensures the new column is placed right after the email
column, which can be useful for maintaining a logical order in your table structure.
When working with migrations, it's crucial to keep a few best practices in mind. Always test your migrations in a development environment before applying them to production. This helps catch any issues early on. Also, consider using seeders to populate your database with initial data after migrations, which can be particularly useful for setting up test environments or providing default data for your application.
One potential pitfall to be aware of is the naming of your migration files. Laravel uses timestamps in the filename to determine the order of execution, so it's essential to ensure your migrations are named correctly to maintain the correct sequence. Additionally, be cautious when rolling back migrations in production, as this can lead to data loss if not handled carefully.
In terms of performance, migrations are generally efficient, but for large-scale applications with many migrations, you might want to consider using the --pretend
flag to see what SQL would be executed without actually running it:
php artisan migrate --pretend
This can help you review the changes before committing to them, especially in complex scenarios.
Overall, Laravel migrations are a powerful tool that can significantly enhance your database management workflow. By following the steps and best practices outlined here, you'll be well on your way to mastering migrations and keeping your database schema in sync with your application's evolving needs.
The above is the detailed content of How to Use Laravel Migrations: A Step-by-Step Tutorial. For more information, please follow other related articles on the PHP Chinese website!

LaravelmigrationsstreamlinedatabasemanagementbyallowingschemachangestobedefinedinPHPcode,whichcanbeversion-controlledandshared.Here'showtousethem:1)Createmigrationclassestodefineoperationslikecreatingormodifyingtables.2)Usethe'phpartisanmigrate'comma

To find the latest version of Laravel, you can visit the official website laravel.com and click the "Docs" button in the upper right corner, or use the Composer command "composershowlaravel/framework|grepversions". Staying updated can help improve project security and performance, but the impact on existing projects needs to be considered.

YoushouldupdatetothelatestLaravelversionforperformanceimprovements,enhancedsecurity,newfeatures,bettercommunitysupport,andlong-termmaintenance.1)Performance:Laravel9'sEloquentORMoptimizationsenhanceapplicationspeed.2)Security:Laravel8introducedbetter

WhenyoumessupamigrationinLaravel,youcan:1)Rollbackthemigrationusing'phpartisanmigrate:rollback'ifit'sthelastone,or'phpartisanmigrate:reset'forall;2)Createanewmigrationtocorrecterrorsifalreadyinproduction;3)Editthemigrationfiledirectly,butthisisrisky;

ToboostperformanceinthelatestLaravelversion,followthesesteps:1)UseRedisforcachingtoimproveresponsetimesandreducedatabaseload.2)OptimizedatabasequerieswitheagerloadingtopreventN 1queryissues.3)Implementroutecachinginproductiontospeeduprouteresolution.

Laravel10introducesseveralkeyfeaturesthatenhancewebdevelopment.1)Lazycollectionsallowefficientprocessingoflargedatasetswithoutloadingallrecordsintomemory.2)The'make:model-and-migration'artisancommandsimplifiescreatingmodelsandmigrations.3)Integration

LaravelMigrationsshouldbeusedbecausetheystreamlinedevelopment,ensureconsistencyacrossenvironments,andsimplifycollaborationanddeployment.1)Theyallowprogrammaticmanagementofdatabaseschemachanges,reducingerrors.2)Migrationscanbeversioncontrolled,ensurin

Yes,LaravelMigrationisworthusing.Itsimplifiesdatabaseschemamanagement,enhancescollaboration,andprovidesversioncontrol.Useitforstructured,efficientdevelopment.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
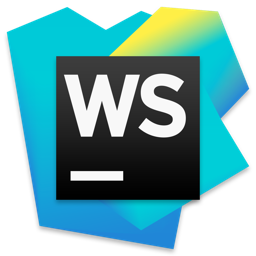
WebStorm Mac version
Useful JavaScript development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
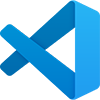
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
