When you mess up a migration in Laravel, you can: 1) Roll back the migration using 'php artisan migrate:rollback' if it's the last one, or 'php artisan migrate:reset' for all; 2) Create a new migration to correct errors if already in production; 3) Edit the migration file directly, but this is risky; 4) Use 'php artisan migrate:fresh' to start over, but be cautious in production. Always test migrations in a staging environment first to avoid issues in a live setting.
When you mess up a migration in Laravel, it can feel like you're stuck in a coding quagmire. But fear not, I've been there and I'll walk you through some strategies to get you back on track.
So, you've messed up a migration. What can you do? You have several options, each with its pros and cons, and I'll dive into them to help you choose the best path forward.
Let's start with understanding what a migration is in Laravel. Migrations are like version control for your database schema. They allow you to modify and share the database schema with your team. When you run a migration, Laravel executes the up
method to apply changes, and the down
method to roll back those changes. But what if you've made a mistake?
One common approach is to roll back the migration using php artisan migrate:rollback
. This command will undo the last migration, which is great if you've just realized your error. However, if you've made multiple migrations since the problematic one, you'll need to roll back multiple times, which can be tedious and risky if you're not careful.
Here's a bit of code to illustrate how to roll back migrations:
// Roll back the last migration php artisan migrate:rollback // Roll back all migrations php artisan migrate:reset
Rolling back is straightforward, but what if you've already pushed the migration to production? That's where things get tricky. You might need to create a new migration that corrects the error. This is where I've learned the hard way that it's crucial to test migrations thoroughly in a staging environment before deploying to production.
Let's say you've added a column that should have been a nullable field. You can create a new migration to fix this:
use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; use Illuminate\Support\Facades\Schema; class FixNullableColumn extends Migration { public function up() { Schema::table('users', function (Blueprint $table) { $table->string('email')->nullable()->change(); }); } public function down() { Schema::table('users', function (Blueprint $table) { $table->string('email')->nullable(false)->change(); }); } }
This approach allows you to fix the issue without losing data, but it can lead to a cluttered migration history if you're not careful. Always remember to keep your migrations clean and well-commented.
Another method is to edit the migration file directly. This is a quick fix, but it's fraught with danger. If you've already run the migration, you'll need to roll it back first, edit the file, and then re-run it. This can lead to inconsistencies if other team members have already run the original migration. Here's how you might edit a migration:
use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; use Illuminate\Support\Facades\Schema; class CreateUsersTable extends Migration { public function up() { Schema::create('users', function (Blueprint $table) { $table->id(); $table->string('name'); $table->string('email')->unique(); // Changed from non-unique to unique $table->timestamp('email_verified_at')->nullable(); $table->string('password'); $table->rememberToken(); $table->timestamps(); }); } public function down() { Schema::dropIfExists('users'); } }
Editing migrations directly can be a slippery slope. It's tempting to do, especially under pressure, but it can lead to issues if not everyone on the team is aware of the changes.
If you're really in a bind, you might consider using php artisan migrate:fresh
. This command will drop all tables and re-run all migrations. It's a nuclear option, but it can be useful if you're in a development environment and need to start fresh. Just be very careful with this in production!
// Drop all tables and re-run all migrations php artisan migrate:fresh
In my experience, the best practice is to test migrations thoroughly in a staging environment before pushing to production. This can save you from the headache of having to fix migrations in a live environment. Also, always keep your migration history clean by merging migrations when possible and commenting them well.
To wrap up, when you mess up a migration, you have several tools at your disposal. Roll back if you can, create a new migration to fix errors if you've already pushed to production, and be very cautious about editing migrations directly. And remember, always test in a staging environment first. With these strategies, you'll be able to navigate the murky waters of migration mishaps with confidence.
The above is the detailed content of Laravel: I messed up my migration, what can I do?. For more information, please follow other related articles on the PHP Chinese website!

LaravelmigrationsstreamlinedatabasemanagementbyallowingschemachangestobedefinedinPHPcode,whichcanbeversion-controlledandshared.Here'showtousethem:1)Createmigrationclassestodefineoperationslikecreatingormodifyingtables.2)Usethe'phpartisanmigrate'comma

To find the latest version of Laravel, you can visit the official website laravel.com and click the "Docs" button in the upper right corner, or use the Composer command "composershowlaravel/framework|grepversions". Staying updated can help improve project security and performance, but the impact on existing projects needs to be considered.

YoushouldupdatetothelatestLaravelversionforperformanceimprovements,enhancedsecurity,newfeatures,bettercommunitysupport,andlong-termmaintenance.1)Performance:Laravel9'sEloquentORMoptimizationsenhanceapplicationspeed.2)Security:Laravel8introducedbetter

WhenyoumessupamigrationinLaravel,youcan:1)Rollbackthemigrationusing'phpartisanmigrate:rollback'ifit'sthelastone,or'phpartisanmigrate:reset'forall;2)Createanewmigrationtocorrecterrorsifalreadyinproduction;3)Editthemigrationfiledirectly,butthisisrisky;

ToboostperformanceinthelatestLaravelversion,followthesesteps:1)UseRedisforcachingtoimproveresponsetimesandreducedatabaseload.2)OptimizedatabasequerieswitheagerloadingtopreventN 1queryissues.3)Implementroutecachinginproductiontospeeduprouteresolution.

Laravel10introducesseveralkeyfeaturesthatenhancewebdevelopment.1)Lazycollectionsallowefficientprocessingoflargedatasetswithoutloadingallrecordsintomemory.2)The'make:model-and-migration'artisancommandsimplifiescreatingmodelsandmigrations.3)Integration

LaravelMigrationsshouldbeusedbecausetheystreamlinedevelopment,ensureconsistencyacrossenvironments,andsimplifycollaborationanddeployment.1)Theyallowprogrammaticmanagementofdatabaseschemachanges,reducingerrors.2)Migrationscanbeversioncontrolled,ensurin

Yes,LaravelMigrationisworthusing.Itsimplifiesdatabaseschemamanagement,enhancescollaboration,andprovidesversioncontrol.Useitforstructured,efficientdevelopment.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
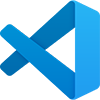
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
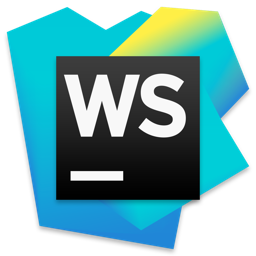
WebStorm Mac version
Useful JavaScript development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Notepad++7.3.1
Easy-to-use and free code editor
