Laravel Soft Delete: Enable, Restore, and Query Deleted Records
In Laravel, enabling soft deletion requires the use of SoftDeletes trait in the model, the restore method is used to restore deleted records, and the withTrashed or onlyTrashed methods are used to query deleted records. 1. Use SoftDeletes trait in the model and specify the deleted_at field. 2. Use the restore method to restore soft deleted records. 3. Use withTrashed to query all records or onlyTrashed to query records that are softly deleted. Soft deletion preserves historical data but does not reduce storage space. Pay attention to the correct method when maintaining indexes and querying.
When processing database records, it is often necessary to delete data, but sometimes we want these deletions to be reversible. This is where the Soft Delete function in the Laravel framework comes in. In this article, we will dive into how to enable soft deletion, how to recover deleted records, and how to query these marked deleted records.
Enabling soft deletion is very simple in Laravel, you just need to use SoftDeletes
trait in your model. Here is a simple code example:
use Illuminate\Database\Eloquent\Model; use Illuminate\Database\Eloquent\SoftDeletes; class User extends Model { use SoftDeletes; protected $dates = ['deleted_at']; }
In this code snippet, we added SoftDeletes
trait to the User
model and specified the deleted_at
field to mark the deleted time. When we call the delete
method, the record is not really deleted from the database, but instead sets deleted_at
field to the current time.
Recovering deleted records is also quite intuitive. We can use the restore
method to restore soft deleted records. Suppose we have a user record that was softly deleted, we can recover it like this:
$user = User::withTrashed()->find(1); $user->restore();
Here we use the withTrashed
method to query records that have been softly deleted, and then restore the records through restore
method.
Querying deleted records is equally simple. We can use the withTrashed
method to query all records, including soft deleted records, or use the onlyTrashed
method to query only soft deleted records. Here are two examples:
// Query all records, including $users that were softly deleted = User::withTrashed()->get(); // Query only soft deleted records $deletedUsers = User::onlyTrashed()->get();
In practical applications, the soft deletion function is very useful, especially when it is necessary to preserve historical data or implement the "Recycle Bin" function. However, there are some things to note when using soft deletion. First, soft deletion does not reduce the storage space of the database, because records still exist in the database. Secondly, if you accidentally forget to use withTrashed
or onlyTrashed
when querying, some logical errors may result, because by default, Laravel will not return soft deleted records.
Regarding performance optimization, index maintenance needs to be taken into account when using soft deletion. The deleted_at
field should be indexed to improve query performance. Additionally, soft deletion can cause performance issues when large-scale data operations, as each deletion operation is actually an update operation.
In practice, I once used soft deletion in an e-commerce platform project to implement the "cancel" function of orders. Users can cancel orders, but order data remains in the system for subsequent audits and analysis. This function greatly improves the flexibility of the system and the integrity of the data.
In short, Laravel's soft deletion feature provides us with a flexible and powerful way to manage the life cycle of our data. Through rational use and optimization, we can make full use of this function to improve the user experience and data management capabilities of the application.
The above is the detailed content of Laravel Soft Delete: Enable, Restore, and Query Deleted Records. For more information, please follow other related articles on the PHP Chinese website!

LaravelmigrationsstreamlinedatabasemanagementbyallowingschemachangestobedefinedinPHPcode,whichcanbeversion-controlledandshared.Here'showtousethem:1)Createmigrationclassestodefineoperationslikecreatingormodifyingtables.2)Usethe'phpartisanmigrate'comma

To find the latest version of Laravel, you can visit the official website laravel.com and click the "Docs" button in the upper right corner, or use the Composer command "composershowlaravel/framework|grepversions". Staying updated can help improve project security and performance, but the impact on existing projects needs to be considered.

YoushouldupdatetothelatestLaravelversionforperformanceimprovements,enhancedsecurity,newfeatures,bettercommunitysupport,andlong-termmaintenance.1)Performance:Laravel9'sEloquentORMoptimizationsenhanceapplicationspeed.2)Security:Laravel8introducedbetter

WhenyoumessupamigrationinLaravel,youcan:1)Rollbackthemigrationusing'phpartisanmigrate:rollback'ifit'sthelastone,or'phpartisanmigrate:reset'forall;2)Createanewmigrationtocorrecterrorsifalreadyinproduction;3)Editthemigrationfiledirectly,butthisisrisky;

ToboostperformanceinthelatestLaravelversion,followthesesteps:1)UseRedisforcachingtoimproveresponsetimesandreducedatabaseload.2)OptimizedatabasequerieswitheagerloadingtopreventN 1queryissues.3)Implementroutecachinginproductiontospeeduprouteresolution.

Laravel10introducesseveralkeyfeaturesthatenhancewebdevelopment.1)Lazycollectionsallowefficientprocessingoflargedatasetswithoutloadingallrecordsintomemory.2)The'make:model-and-migration'artisancommandsimplifiescreatingmodelsandmigrations.3)Integration

LaravelMigrationsshouldbeusedbecausetheystreamlinedevelopment,ensureconsistencyacrossenvironments,andsimplifycollaborationanddeployment.1)Theyallowprogrammaticmanagementofdatabaseschemachanges,reducingerrors.2)Migrationscanbeversioncontrolled,ensurin

Yes,LaravelMigrationisworthusing.Itsimplifiesdatabaseschemamanagement,enhancescollaboration,andprovidesversioncontrol.Useitforstructured,efficientdevelopment.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
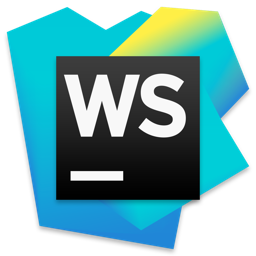
WebStorm Mac version
Useful JavaScript development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
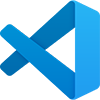
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
