How can PHP and HTML interact?
PHP and HTML interact primarily through the integration of PHP code within HTML documents. PHP is a server-side scripting language, which means it runs on the server before the page is sent to the client's browser. This allows PHP to generate dynamic content that can be seamlessly embedded within an HTML structure.
When a user requests a PHP-enabled webpage, the server processes the PHP code first, which may include logic, database queries, or other operations. The result of the PHP execution is then merged with the HTML, replacing the PHP code with its output. This output can include plain text, HTML tags, or even JavaScript, allowing for a fully interactive and dynamic web experience.
For example, a simple interaction between PHP and HTML might look like this:
<!DOCTYPE html> <html> <body> <?php echo "<h1 id="Hello-World">Hello World</h1>"; ?> </body> </html>
In this case, the PHP code echo "<h1 id="Hello-World">Hello World</h1>";
will be executed on the server and replaced with the <h1 id="Hello-World">Hello World</h1>
HTML tag, which is then sent to the client's browser.
What are the best practices for embedding PHP within HTML?
When embedding PHP within HTML, following best practices can significantly improve code readability, maintainability, and performance. Here are some key practices:
- Separate Concerns: Keep your HTML structure and PHP logic as separate as possible. Use PHP to handle data processing and logic, and let HTML take care of the presentation. This can be achieved by using PHP includes or templating engines like Twig or Blade.
-
Use PHP Tags Sparingly: Avoid opening and closing PHP tags excessively. Instead, try to group PHP code together to reduce the number of tags. For example:
<?php $name = "John"; $age = 30; ?> <p>Name: <?php echo $name; ?>, Age: <?php echo $age; ?></p>
Could be better written as:
<?php $name = "John"; $age = 30; ?> <p>Name: <?php echo $name; ?>, Age: <?php echo $age; ?></p>
- Avoid Mixing PHP and HTML in Complex Logic: For complex logic, consider separating PHP code into functions or separate files. This keeps the HTML clean and makes the PHP code easier to manage.
-
Use PHP Short Tags Carefully: PHP short tags
=
are convenient for echoing values but should be used cautiously as they might not be enabled on all servers. -
Security Considerations: Always sanitize and validate any user input to prevent security vulnerabilities like SQL injection and XSS attacks. Use PHP's built-in functions like
htmlspecialchars()
to escape output.
How can PHP dynamically generate HTML content?
PHP can dynamically generate HTML content through several methods, enabling the creation of responsive and personalized web experiences. Here’s how:
-
Direct Output: PHP can directly output HTML content using functions like
echo
orprint
. This is straightforward but can become messy in complex scenarios.<?php $name = "Alice"; echo "<h1 id="Hello-name">Hello, $name!</h1>"; ?>
-
Conditional Statements and Loops: PHP can use conditional statements (like
if
,else
) and loops (likeforeach
,while
) to generate HTML content based on certain conditions or data sets.<?php $users = ["Alice", "Bob", "Charlie"]; foreach ($users as $user) { echo "<li>$user</li>"; } ?>
-
Functions and Includes: PHP functions can generate HTML, and these functions can be included in multiple pages, promoting code reuse.
<?php function generateHeader($title) { echo "<header><h1 id="title">$title</h1></header>"; } generateHeader("Welcome to My Site"); ?>
-
Templating Engines: Using templating engines like Twig or Blade can separate PHP logic from HTML, making it easier to manage complex dynamic content.
// Using Twig $loader = new \Twig\Loader\FilesystemLoader('path/to/templates'); $twig = new \Twig\Environment($loader); echo $twig->render('user_profile.html', ['name' => 'Alice']);
-
Database Integration: PHP can query databases and use the results to dynamically generate HTML. For instance, retrieving user data from a MySQL database and displaying it on the page.
<?php $conn = mysqli_connect("localhost", "username", "password", "dbname"); $result = mysqli_query($conn, "SELECT name FROM users"); while($row = mysqli_fetch_assoc($result)) { echo "<li>" . htmlspecialchars($row['name']) . "</li>"; } mysqli_close($conn); ?>
What tools or IDEs are recommended for developing with PHP and HTML?
Several tools and IDEs are well-suited for developing with PHP and HTML, each offering different features and benefits. Here are some of the most recommended:
- PhpStorm: This is one of the most comprehensive IDEs for PHP development. It offers advanced features like code completion, debugging, version control integration, and support for frameworks like Laravel and Symfony. PhpStorm also has robust HTML and CSS support, making it ideal for full-stack development.
- Visual Studio Code (VS Code): VS Code is a lightweight yet powerful code editor with extensive plugin support. With extensions like PHP Intelephense, you can get advanced PHP features, while HTML and CSS tools are built-in. VS Code is highly customizable and widely used in the web development community.
- Sublime Text: A popular choice for its speed and efficiency, Sublime Text supports PHP and HTML out of the box. With plugins like Emmet and PHPIntel, it can be transformed into a powerful development environment.
- NetBeans: An open-source IDE, NetBeans provides a comprehensive development environment with strong support for PHP, HTML, and other web technologies. It offers features like code completion, debugging, and project management.
- Atom: Atom is another versatile text editor that supports PHP and HTML development. With its vast library of packages, developers can extend its capabilities to include advanced PHP debugging and HTML/CSS editing features.
- Eclipse with PDT (PHP Development Tools): Eclipse is a well-known IDE that, when combined with the PDT plugin, provides a robust environment for PHP and HTML development. It includes code assistance, debugging, and project management tools.
Each of these tools offers different advantages depending on your specific needs and preferences. Choosing the right one depends on factors such as project complexity, preferred workflow, and the level of customization desired.
The above is the detailed content of How can PHP and HTML interact?. For more information, please follow other related articles on the PHP Chinese website!
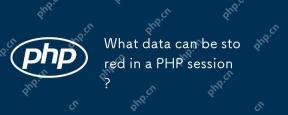
PHPsessionscanstorestrings,numbers,arrays,andobjects.1.Strings:textdatalikeusernames.2.Numbers:integersorfloatsforcounters.3.Arrays:listslikeshoppingcarts.4.Objects:complexstructuresthatareserialized.
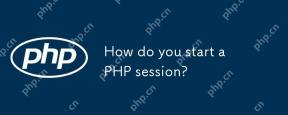
TostartaPHPsession,usesession_start()atthescript'sbeginning.1)Placeitbeforeanyoutputtosetthesessioncookie.2)Usesessionsforuserdatalikeloginstatusorshoppingcarts.3)RegeneratesessionIDstopreventfixationattacks.4)Considerusingadatabaseforsessionstoragei
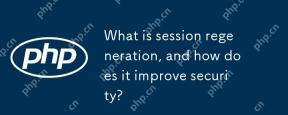
Session regeneration refers to generating a new session ID and invalidating the old ID when the user performs sensitive operations in case of session fixed attacks. The implementation steps include: 1. Detect sensitive operations, 2. Generate new session ID, 3. Destroy old session ID, 4. Update user-side session information.
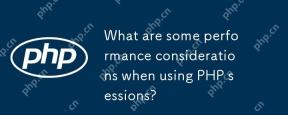
PHP sessions have a significant impact on application performance. Optimization methods include: 1. Use a database to store session data to improve response speed; 2. Reduce the use of session data and only store necessary information; 3. Use a non-blocking session processor to improve concurrency capabilities; 4. Adjust the session expiration time to balance user experience and server burden; 5. Use persistent sessions to reduce the number of data read and write times.
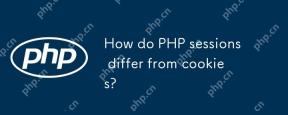
PHPsessionsareserver-side,whilecookiesareclient-side.1)Sessionsstoredataontheserver,aremoresecure,andhandlelargerdata.2)Cookiesstoredataontheclient,arelesssecure,andlimitedinsize.Usesessionsforsensitivedataandcookiesfornon-sensitive,client-sidedata.
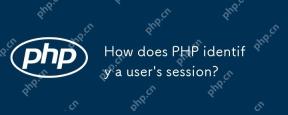
PHPidentifiesauser'ssessionusingsessioncookiesandsessionIDs.1)Whensession_start()iscalled,PHPgeneratesauniquesessionIDstoredinacookienamedPHPSESSIDontheuser'sbrowser.2)ThisIDallowsPHPtoretrievesessiondatafromtheserver.
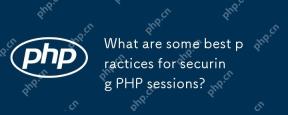
The security of PHP sessions can be achieved through the following measures: 1. Use session_regenerate_id() to regenerate the session ID when the user logs in or is an important operation. 2. Encrypt the transmission session ID through the HTTPS protocol. 3. Use session_save_path() to specify the secure directory to store session data and set permissions correctly.
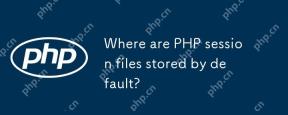
PHPsessionfilesarestoredinthedirectoryspecifiedbysession.save_path,typically/tmponUnix-likesystemsorC:\Windows\TemponWindows.Tocustomizethis:1)Usesession_save_path()tosetacustomdirectory,ensuringit'swritable;2)Verifythecustomdirectoryexistsandiswrita


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Chinese version
Chinese version, very easy to use

SublimeText3 Linux new version
SublimeText3 Linux latest version

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
