


Laravel Middleware (Middleware) Practical combat: Permission control and logging
In Laravel, middleware is used to implement permission control and logging. 1) Create permission control middleware and decide whether to allow access by checking user permissions. 2) Create logging middleware to record detailed information about requests and responses.
introduction
In Laravel development, Middleware is a powerful and flexible tool that can execute specific logic before or after the request reaches the application. Today we will dive into how to use middleware to implement permission control and logging, two features that are very common and important in real projects. Through this article, you will learn how to create and use middleware, understand how it works, and master some practical tips and best practices.
Review of basic knowledge
In Laravel, middleware is the middle layer that handles HTTP requests. They can be used to filter requests, modify requests and responses, and execute some common logic. The concept of middleware is similar to a pipeline. When requests pass through this pipeline, they can be intercepted and processed by middleware.
Laravel provides several built-in middleware, such as auth
middleware to verify whether the user is logged in, csrf
middleware to prevent cross-site request forgery attacks. We can easily create custom middleware to meet specific needs.
Core concept or function analysis
Definition and function of middleware
Middleware is a class in Laravel that implements handle
methods. This method receives the request object and a closure (representing the next processing step of the request), which can process the request and then decide whether to pass the request to the next middleware or return the response directly.
Middleware has a very wide function, from simple request logging to complex permission control, it can be implemented through middleware. Its advantage is that it can be pulled out of the controller, making the code clearer and more maintainable.
A simple middleware example:
namespace App\Http\Middleware; use Closure; use Illuminate\Http\Request; class LogRequestMiddleware { public function handle(Request $request, Closure $next) { // Log the log before the request is processed\Log::info('Request received: ' . $request->method() . ' ' . $request->url()); // Pass the request to the next middleware or controller return $next($request); } }
How it works
When a request enters a Laravel application, it passes through a middleware pipeline. Each middleware can process the request and then decide whether to pass the request to the next middleware or return the response directly.
The execution order of middleware is defined by $middleware
and $routeMiddleware
arrays in the Kernel.php
file. Requests are passed through the middleware in the order in these arrays.
When processing a request, the middleware can:
- Modify the request object
- Execute some logic (such as logging)
- Decide whether to pass the request to the next middleware or controller
- Modify the response object (in the
terminate
method)
The working principle of middleware is similar to the onion model. Requests enter from the outer layer, processed by multiple middleware, and finally arrive at the controller, and then pass it from the inner layer to the outer layer, and then return it to the client after processing by the middleware.
Example of usage
Permission Control Middleware
In actual projects, permission control is a common requirement. We can create a middleware to check whether the user has permission to access a certain route.
namespace App\Http\Middleware; use Closure; use Illuminate\Http\Request; use Illuminate\Support\Facades\Auth; class CheckPermissionMiddleware { public function handle(Request $request, Closure $next, $permission) { if (Auth::user()->can($permission)) { return $next($request); } return response()->json(['error' => 'Unauthorized'], 403); } }
When using this middleware, you can specify the required permissions in the routing definition:
Route::get('/admin', function () { // Only users with 'manage-admin' permission can access it})->middleware('permission:manage-admin');
Logging middleware
Logging is also a common requirement, we can create a middleware to record the details of each request.
namespace App\Http\Middleware; use Closure; use Illuminate\Http\Request; use Illuminate\Support\Facades\Log; class LogRequestMiddleware { public function handle(Request $request, Closure $next) { // Log request information Log::info('Request received', [ 'method' => $request->method(), 'url' => $request->url(), 'headers' => $request->headers->all(), 'body' => $request->all(), ]); return $next($request); } public function terminate(Request $request, $response) { // Record response information Log::info('Response sent', [ 'status' => $response->getStatusCode(), 'content' => $response->getContent(), ]); } }
Common Errors and Debugging Tips
Some common problems may occur when using middleware:
- Middleware order problem : If the middleware is executed incorrectly, it may lead to logical errors. For example, permission checking middleware should be executed before logging middleware to avoid logging unauthorized requests.
- Middleware parameter passing error : When using middleware with parameters, make sure the parameter passing is correct. For example, in
CheckPermissionMiddleware
, the$permission
parameter must be passed correctly. - Middleware not registered : Make sure the middleware is registered correctly in the
Kernel.php
file, otherwise the middleware will not be executed.
When debugging these problems, you can use Laravel's logging system to record the middleware execution, or use debugging tools (such as Xdebug) to track the process of requests.
Performance optimization and best practices
There are some performance optimizations and best practices worth noting when using middleware:
- Avoid performing time-consuming operations in middleware : The middleware should be as light as possible to avoid performing database queries or other time-consuming operations in the middleware to avoid affecting the response time of the request.
- Using Cache : In the permission check middleware, you can use cache to store user permission information to avoid querying the database every time you request.
- Optimization of logging : In the logging middleware, the log detail level can be adjusted according to the environment (such as the production environment or the development environment) to avoid recording too much log information in the production environment.
When writing middleware, you should also pay attention to the readability and maintainability of the code:
- Use clear naming : The middleware's class and method names should clearly express their functions.
- Add comments : Add comments to the key parts of the middleware to explain its role and implementation principles.
- Keep the middleware single responsibility : Each middleware should be responsible for only one function, avoiding putting multiple irrelevant logic in the same middleware.
Through this article, you should have mastered how to use middleware in Laravel to implement permission control and logging. Hopefully these knowledge and techniques will work in your project and help you write more efficient and easier to maintain code.
The above is the detailed content of Laravel Middleware (Middleware) Practical combat: Permission control and logging. For more information, please follow other related articles on the PHP Chinese website!

Taskmanagementtoolsareessentialforeffectiveremoteprojectmanagementbyprioritizingtasksandtrackingprogress.1)UsetoolslikeTrelloandAsanatosetprioritieswithlabelsortags.2)EmploytoolslikeJiraandMonday.comforvisualtrackingwithGanttchartsandprogressbars.3)K

Laravel10enhancesperformancethroughseveralkeyfeatures.1)Itintroducesquerybuildercachingtoreducedatabaseload.2)ItoptimizesEloquentmodelloadingwithlazyloadingproxies.3)Itimprovesroutingwithanewcachingsystem.4)ItenhancesBladetemplatingwithviewcaching,al

The best full-stack Laravel application deployment strategies include: 1. Zero downtime deployment, 2. Blue-green deployment, 3. Continuous deployment, and 4. Canary release. 1. Zero downtime deployment uses Envoy or Deployer to automate the deployment process to ensure that applications remain available when updated. 2. Blue and green deployment enables downtime deployment by maintaining two environments and allows for rapid rollback. 3. Continuous deployment Automate the entire deployment process through GitHubActions or GitLabCI/CD. 4. Canary releases through Nginx configuration, gradually promoting the new version to users to ensure performance optimization and rapid rollback.

ToscaleaLaravelapplicationeffectively,focusondatabasesharding,caching,loadbalancing,andmicroservices.1)Implementdatabaseshardingtodistributedataacrossmultipledatabasesforimprovedperformance.2)UseLaravel'scachingsystemwithRedisorMemcachedtoreducedatab

Toovercomecommunicationbarriersindistributedteams,use:1)videocallsforface-to-faceinteraction,2)setclearresponsetimeexpectations,3)chooseappropriatecommunicationtools,4)createateamcommunicationguide,and5)establishpersonalboundariestopreventburnout.The

LaravelBladeenhancesfrontendtemplatinginfull-stackprojectsbyofferingcleansyntaxandpowerfulfeatures.1)Itallowsforeasyvariabledisplayandcontrolstructures.2)Bladesupportscreatingandreusingcomponents,aidinginmanagingcomplexUIs.3)Itefficientlyhandleslayou

Laravelisidealforfull-stackapplicationsduetoitselegantsyntax,comprehensiveecosystem,andpowerfulfeatures.1)UseEloquentORMforintuitivebackenddatamanipulation,butavoidN 1queryissues.2)EmployBladetemplatingforcleanfrontendviews,beingcautiousofoverusing@i

Forremotework,IuseZoomforvideocalls,Slackformessaging,Trelloforprojectmanagement,andGitHubforcodecollaboration.1)Zoomisreliableforlargemeetingsbuthastimelimitsonthefreeversion.2)Slackintegrateswellwithothertoolsbutcanleadtonotificationoverload.3)Trel


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
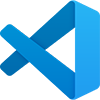
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Zend Studio 13.0.1
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
