Eager Loading can resolve N 1 query issues in Laravel. 1) Use the with method to preload relevant model data, such as User::with('posts')->get(). 2) For nested relationships, use with('posts.comments'). 3) Avoid overuse, selective loading, and use the load method as needed. Through these methods, the number of queries can be significantly reduced and the application performance can be improved.
introduction
In Laravel development, the N 1 query problem is a common performance bottleneck, which can cause a surge in database queries, seriously affecting the response speed of the application. Today we will discuss how to solve this problem through Eager Loading. After reading this article, you will master the basic concepts and usage methods of Eager Loading, which can effectively optimize your Laravel application and improve its performance.
Review of basic knowledge
In Laravel, the relationships between models are managed through Eloquent ORM. Eloquent provides convenient ways to define and query the relationship between models, such as one-to-one, one-to-many, many-to-many, etc. However, when we accidentally use Lazy Loading, we can easily fall into the trap of N 1 query.
Lazy loading is when data is loaded for the relevant model only when needed, which sounds efficient, but actually causes each parent model to trigger an additional query. For example, if you have a User
model where each user has multiple Post
, when you iterate through all users and access their posts, each user will trigger an additional query to get the post, which is the N 1 query problem.
Core concept or function analysis
The definition and function of Eager Loading
Eager Loading is a preloading technology that allows you to load data from all relevant models in one query, thus avoiding N 1 query problems. By using Eager Loading, you can significantly reduce the number of database queries and improve application performance.
Let's look at a simple example:
$users = User::with('posts')->get();
In this example, with('posts')
tells Laravel to load their posts while querying users. This way, all post data will be loaded in a query instead of triggering an additional query for each user.
How Eager Loading works
The implementation principle of Eager Loading is to obtain all relevant data at once by using JOIN
or subqueries. Specifically, Laravel generates an SQL query containing all the necessary data based on the relationship you specify.
For example, the example above might generate SQL queries similar to the following:
SELECT * FROM users; SELECT * FROM posts WHERE user_id IN (1, 2, 3, ...);
This way, all users and their post data will be loaded in two queries instead of each user triggering an additional query.
Example of usage
Basic usage
Let's look at a more specific example, suppose we have a User
model and a Post
model, and the user and post are a one-to-many relationship. We want to get all users and their posts:
$users = User::with('posts')->get(); foreach ($users as $user) { echo $user->name . " has " . $user->posts->count() . " posts."; }
In this example, with('posts')
ensures that all users' post data are loaded in a single query.
Advanced Usage
Eager Loading can also be used for more complex relationships, such as nested relationships. Assuming each post has multiple comments, we want to get all users and their posts and comments:
$users = User::with('posts.comments')->get(); foreach ($users as $user) { foreach ($user->posts as $post) { echo $post->title . " has " . $post->comments->count() . " comments."; } }
In this example, with('posts.comments')
ensures that all users' posts and comment data are loaded in a single query.
Common Errors and Debugging Tips
When using Eager Loading, a common mistake is to forget to use the with
method, resulting in still using lazy loading. To avoid this problem, you can define the default Eager Loading relationship in the model:
class User extends Model { protected $with = ['posts']; }
In this way, every time the User
model is queryed, posts
relationship will be automatically loaded.
Another common mistake is over-use of Eager Loading, which causes queries to become too complex and affect performance. In this case, you can use the load
method to load the relationship on demand:
$users = User::all(); $users->load('posts');
This way, you can load relationships as needed, avoiding loading all data at once.
Performance optimization and best practices
In practical applications, Eager Loading can significantly improve performance, but you should also pay attention to the following points:
- Avoid overuse : Although Eager Loading can reduce the number of queries, if the amount of data loaded at one time is too large, it may increase memory usage and affect performance.
- Selective loading : Selectively load relationships based on actual needs, rather than loading all relationships at once.
- Use the
load
method : Useload
method to load relationships as needed when needed, rather than loading all relationships at once when querying.
Let's look at an example of performance comparison:
// Lazy loading $users = User::all(); foreach ($users as $user) { $user->posts; // Trigger N 1 query} // Eager Loading $users = User::with('posts')->get(); foreach ($users as $user) { $user->posts; // Loaded, no additional query is triggered}
By using Eager Loading, we can reduce the number of queries from N 1 to 2, significantly improving performance.
When writing code, it is also very important to keep the code readable and maintained. When using Eager Loading, make sure your code is clear and well-commented so that other developers can easily understand and maintain your code.
In short, Eager Loading is a powerful tool that can help you solve N 1 query problems in Laravel. By using Eager Loading properly, you can significantly improve the performance of your application and provide a better user experience.
The above is the detailed content of Laravel N 1 query problem: How to solve it with Eager Loading?. For more information, please follow other related articles on the PHP Chinese website!

Taskmanagementtoolsareessentialforeffectiveremoteprojectmanagementbyprioritizingtasksandtrackingprogress.1)UsetoolslikeTrelloandAsanatosetprioritieswithlabelsortags.2)EmploytoolslikeJiraandMonday.comforvisualtrackingwithGanttchartsandprogressbars.3)K

Laravel10enhancesperformancethroughseveralkeyfeatures.1)Itintroducesquerybuildercachingtoreducedatabaseload.2)ItoptimizesEloquentmodelloadingwithlazyloadingproxies.3)Itimprovesroutingwithanewcachingsystem.4)ItenhancesBladetemplatingwithviewcaching,al

The best full-stack Laravel application deployment strategies include: 1. Zero downtime deployment, 2. Blue-green deployment, 3. Continuous deployment, and 4. Canary release. 1. Zero downtime deployment uses Envoy or Deployer to automate the deployment process to ensure that applications remain available when updated. 2. Blue and green deployment enables downtime deployment by maintaining two environments and allows for rapid rollback. 3. Continuous deployment Automate the entire deployment process through GitHubActions or GitLabCI/CD. 4. Canary releases through Nginx configuration, gradually promoting the new version to users to ensure performance optimization and rapid rollback.

ToscaleaLaravelapplicationeffectively,focusondatabasesharding,caching,loadbalancing,andmicroservices.1)Implementdatabaseshardingtodistributedataacrossmultipledatabasesforimprovedperformance.2)UseLaravel'scachingsystemwithRedisorMemcachedtoreducedatab

Toovercomecommunicationbarriersindistributedteams,use:1)videocallsforface-to-faceinteraction,2)setclearresponsetimeexpectations,3)chooseappropriatecommunicationtools,4)createateamcommunicationguide,and5)establishpersonalboundariestopreventburnout.The

LaravelBladeenhancesfrontendtemplatinginfull-stackprojectsbyofferingcleansyntaxandpowerfulfeatures.1)Itallowsforeasyvariabledisplayandcontrolstructures.2)Bladesupportscreatingandreusingcomponents,aidinginmanagingcomplexUIs.3)Itefficientlyhandleslayou

Laravelisidealforfull-stackapplicationsduetoitselegantsyntax,comprehensiveecosystem,andpowerfulfeatures.1)UseEloquentORMforintuitivebackenddatamanipulation,butavoidN 1queryissues.2)EmployBladetemplatingforcleanfrontendviews,beingcautiousofoverusing@i

Forremotework,IuseZoomforvideocalls,Slackformessaging,Trelloforprojectmanagement,andGitHubforcodecollaboration.1)Zoomisreliableforlargemeetingsbuthastimelimitsonthefreeversion.2)Slackintegrateswellwithothertoolsbutcanleadtonotificationoverload.3)Trel


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SublimeText3 Chinese version
Chinese version, very easy to use
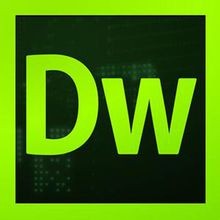
Dreamweaver CS6
Visual web development tools
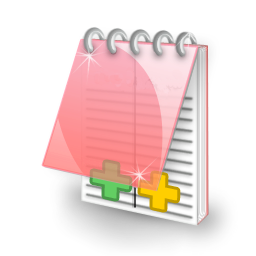
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
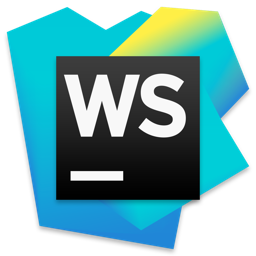
WebStorm Mac version
Useful JavaScript development tools
