在C++中实现无锁数据结构可以通过使用原子操作和CAS操作来实现。具体步骤包括:1.使用std::atomic保证head和tail的原子性操作;2.使用compare_exchange_strong进行CAS操作,确保数据一致性;3.使用std::shared_ptr管理节点数据,避免内存泄漏。
在C++中实现无锁数据结构是一项既具有挑战性又有趣的任务。无锁数据结构可以提高多线程程序的性能,因为它们消除了锁的开销,减少了线程之间的竞争和等待时间。然而,实现无锁数据结构需要深入理解原子操作、内存模型以及并发编程的各种陷阱。
让我们从一个基本的无锁队列开始探讨这个主题。无锁队列是一种常见的无锁数据结构,它允许多个线程同时进行入队和出队操作,而不需要锁来保护共享资源。
首先,我们需要了解原子操作和CAS(Compare-and-Swap)操作。CAS操作是无锁算法的核心,它允许我们以原子方式比较并交换内存中的值。如果当前值与预期值匹配,则将其替换为新值;否则,操作失败。C++提供了<atomic></atomic>
头文件来支持原子操作。
让我们来看看一个简单的无锁队列实现:
#include <atomic> #include <memory> template<typename T> class LockFreeQueue { private: struct Node { std::shared_ptr<T> data; Node* next; Node(T const& data_) : data(std::make_shared<T>(data_)), next(nullptr) {} }; std::atomic<Node*> head; std::atomic<Node*> tail; public: LockFreeQueue() : head(new Node(T())), tail(head.load()) {} ~LockFreeQueue() { while (Node* const old_head = head.load()) { head.store(old_head->next); delete old_head; } } void enqueue(T const& data) { Node* new_node = new Node(data); Node* old_tail = nullptr; Node* old_next = nullptr; while (true) { old_tail = tail.load(); old_next = old_tail->next.load(); if (old_tail == tail.load()) { if (old_next == nullptr) { if (old_tail->next.compare_exchange_strong(old_next, new_node)) { break; } } else { tail.compare_exchange_strong(old_tail, old_next); } } } tail.compare_exchange_strong(old_tail, new_node); } bool dequeue(T& result) { Node* old_head = head.load(); Node* old_tail = tail.load(); Node* new_head = old_head->next.load(); if (old_head == head.load()) { if (new_head == nullptr) { return false; } if (old_head == old_tail) { tail.compare_exchange_strong(old_tail, new_head); } result = *new_head->data; if (head.compare_exchange_strong(old_head, new_head)) { delete old_head; return true; } } return false; } };
这个无锁队列实现了一些关键点:
-
原子操作:使用
std::atomic
来保证head
和tail
的原子性操作。 -
CAS操作:使用
compare_exchange_strong
来进行CAS操作,确保在并发环境下数据的一致性。 -
内存管理:使用
std::shared_ptr
来管理节点数据的生命周期,避免内存泄漏。
然而,实现无锁数据结构也有一些挑战和需要注意的地方:
- ABA问题:CAS操作可能遇到ABA问题,即一个值被修改了两次后又恢复到原来的值,导致CAS操作成功但数据不一致。在某些情况下,可以使用带版本号的CAS操作来解决这个问题。
-
内存顺序:C++11引入的内存模型定义了不同类型的内存顺序(如
std::memory_order_relaxed
、std::memory_order_acquire
等),正确选择内存顺序对无锁算法的正确性至关重要。 - 性能调优:无锁数据结构的性能优化需要考虑很多因素,如缓存一致性、假共享等。需要通过性能测试和分析来找到最佳的实现方式。
在实际应用中,无锁数据结构的选择和实现需要根据具体的需求和场景来决定。有些情况下,简单的锁定机制可能更容易实现和维护,而在高并发环境下,无锁数据结构则能带来显著的性能提升。
总之,实现C++中的无锁数据结构需要深入理解并发编程的原理和技术,同时也需要不断地测试和优化。希望这个简单的无锁队列实现能为你提供一些启发和参考。
The above is the detailed content of How to implement lock-free data structure in C?. For more information, please follow other related articles on the PHP Chinese website!
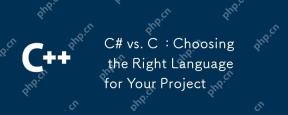
C# is suitable for projects that require development efficiency and type safety, while C is suitable for projects that require high performance and hardware control. 1) C# provides garbage collection and LINQ, suitable for enterprise applications and Windows development. 2)C is known for its high performance and underlying control, and is widely used in gaming and system programming.
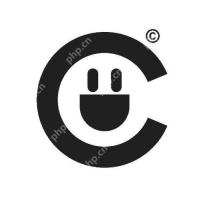
C code optimization can be achieved through the following strategies: 1. Manually manage memory for optimization use; 2. Write code that complies with compiler optimization rules; 3. Select appropriate algorithms and data structures; 4. Use inline functions to reduce call overhead; 5. Apply template metaprogramming to optimize at compile time; 6. Avoid unnecessary copying, use moving semantics and reference parameters; 7. Use const correctly to help compiler optimization; 8. Select appropriate data structures, such as std::vector.
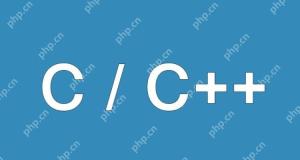
The volatile keyword in C is used to inform the compiler that the value of the variable may be changed outside of code control and therefore cannot be optimized. 1) It is often used to read variables that may be modified by hardware or interrupt service programs, such as sensor state. 2) Volatile cannot guarantee multi-thread safety, and should use mutex locks or atomic operations. 3) Using volatile may cause performance slight to decrease, but ensure program correctness.
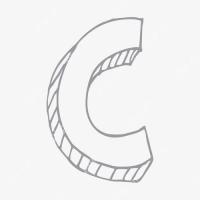
Measuring thread performance in C can use the timing tools, performance analysis tools, and custom timers in the standard library. 1. Use the library to measure execution time. 2. Use gprof for performance analysis. The steps include adding the -pg option during compilation, running the program to generate a gmon.out file, and generating a performance report. 3. Use Valgrind's Callgrind module to perform more detailed analysis. The steps include running the program to generate the callgrind.out file and viewing the results using kcachegrind. 4. Custom timers can flexibly measure the execution time of a specific code segment. These methods help to fully understand thread performance and optimize code.
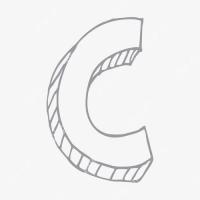
Using the chrono library in C can allow you to control time and time intervals more accurately. Let's explore the charm of this library. C's chrono library is part of the standard library, which provides a modern way to deal with time and time intervals. For programmers who have suffered from time.h and ctime, chrono is undoubtedly a boon. It not only improves the readability and maintainability of the code, but also provides higher accuracy and flexibility. Let's start with the basics. The chrono library mainly includes the following key components: std::chrono::system_clock: represents the system clock, used to obtain the current time. std::chron
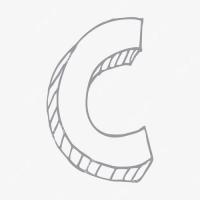
C performs well in real-time operating system (RTOS) programming, providing efficient execution efficiency and precise time management. 1) C Meet the needs of RTOS through direct operation of hardware resources and efficient memory management. 2) Using object-oriented features, C can design a flexible task scheduling system. 3) C supports efficient interrupt processing, but dynamic memory allocation and exception processing must be avoided to ensure real-time. 4) Template programming and inline functions help in performance optimization. 5) In practical applications, C can be used to implement an efficient logging system.
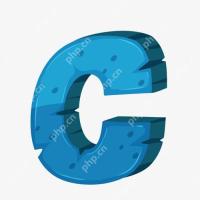
ABI compatibility in C refers to whether binary code generated by different compilers or versions can be compatible without recompilation. 1. Function calling conventions, 2. Name modification, 3. Virtual function table layout, 4. Structure and class layout are the main aspects involved.
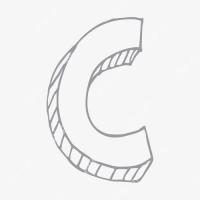
DMA in C refers to DirectMemoryAccess, a direct memory access technology, allowing hardware devices to directly transmit data to memory without CPU intervention. 1) DMA operation is highly dependent on hardware devices and drivers, and the implementation method varies from system to system. 2) Direct access to memory may bring security risks, and the correctness and security of the code must be ensured. 3) DMA can improve performance, but improper use may lead to degradation of system performance. Through practice and learning, we can master the skills of using DMA and maximize its effectiveness in scenarios such as high-speed data transmission and real-time signal processing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
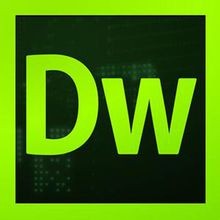
Dreamweaver CS6
Visual web development tools
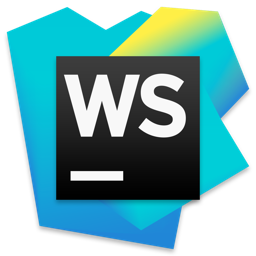
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor
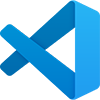
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
