Yii 2.0 Deep Dive: Performance Tuning & Optimization
Strategies to improve Yii 2.0 application performance include: 1. Database query optimization, using Query Builder and Active Record to select specific fields and limit result sets; 2. Caching strategy, rational use of data, query and page caching; 3. Code-level optimization, reducing object creation and using efficient algorithms. Through these methods, the performance of Yii 2.0 applications can be significantly improved.
introduction
In today's web development world, performance optimization is a challenge that every developer must face. Especially for developers using the Yii 2.0 framework, how to make full use of its features to improve application performance is a topic worth discussing in depth. This article will take you into the deep understanding of Yii 2.0's performance tuning and optimization strategies. By reading this article, you will learn how to improve the performance of Yii 2.0 applications from multiple perspectives, including database query optimization, caching strategies, code-level optimization, etc.
Review of basic knowledge
Yii 2.0 is a high-performance PHP framework that takes into account performance and efficiency from the beginning of its design. It provides rich features such as Active Record, Query Builder, Caching, etc., which are important tools for us to optimize performance. Understanding these basic concepts is crucial for subsequent optimization strategies.
For example, Active Record is an ORM tool for database operations in Yii 2.0, which can simplify database interactions, but can also cause performance issues if used improperly. Query Builder provides a more flexible query method, which can help us control database queries more accurately.
Core concept or function analysis
Database query optimization
In Yii 2.0, database query is one of the key points of performance optimization. By using the right way to use Query Builder and Active Record, we can significantly improve query performance.
// Use Query Builder for optimization $query = (new \yii\db\Query()) ->select(['id', 'name']) ->from('user') ->where(['status' => 1]) ->limit(10); $users = $query->all(); // Use Active Record for optimization $users = User::find() ->select(['id', 'name']) ->where(['status' => 1]) ->limit(10) ->all();
These two examples show how to optimize a query by selecting a specific field and limiting the result set. Using Query Builder allows for more granular control of queries, while Active Record provides a cleaner syntax.
Cache Policy
Yii 2.0 provides a variety of caching mechanisms, including data caching, query caching and page caching. Using these caches rationally can greatly improve the response speed of your application.
// Data cache example $cache = Yii::$app->cache; $data = $cache->get('my_data'); if ($data === false) { $data = // Get data from the database or elsewhere $cache->set('my_data', $data, 3600); // Cache for one hour} // Query cache example $users = User::find() ->cache(3600) // Cache for one hour ->all();
The key to a cache strategy is to find the right cache time and cache content. Overcache may lead to data inconsistency, while too short cache time will not fully utilize the performance improvements brought by cache.
Code-level optimization
In Yii 2.0, code-level optimization mainly includes reducing unnecessary object creation, using more efficient algorithms and data structures, etc.
// Avoid unnecessary object creation $users = User::find()->all(); foreach ($users as $user) { echo $user->name; // Avoid repeated creation of User objects in loops} // Use more efficient algorithm $array = [1, 2, 3, 4, 5]; $sum = array_sum($array); // Use built-in functions instead of manual loop
Code-level optimization requires developers to have a deep understanding of the PHP language and the Yii 2.0 framework. Through continuous practice and learning, we can gradually master these skills.
Example of usage
Basic usage
In actual projects, we can combine the above strategies for performance optimization. For example, optimize the query and cache of a user list page:
// Optimize user list page $users = User::find() ->select(['id', 'name', 'email']) ->where(['status' => 1]) ->orderBy('created_at DESC') ->limit(20) ->cache(3600) ->all(); // Render page return $this->render('index', ['users' => $users]);
This example shows how to optimize the performance of user list pages by selecting specific fields, limiting result sets, sorting, and cache.
Advanced Usage
For more complex scenarios, we can use the extension of Yii 2.0 to further optimize performance. For example, use Redis as the cache backend:
// Configure Redis as cache backend 'components' => [ 'cache' => [ 'class' => 'yii\redis\Cache', 'redis' => [ 'hostname' => 'localhost', 'port' => 6379, 'database' => 0, ], ], ], // Use Redis to cache $cache = Yii::$app->cache; $data = $cache->get('my_data'); if ($data === false) { $data = // Get data from the database or elsewhere $cache->set('my_data', $data, 3600); // Cache for one hour}
Using Redis as the cache backend can significantly improve cache performance, especially in high concurrency scenarios.
Common Errors and Debugging Tips
Common errors when performing performance optimization include over-optimization, ignoring cache failures, abuse of Active Record, etc. Here are some debugging tips:
- Use Yii's debug toolbar to view database queries and cache hits.
- Identify performance bottlenecks through logging and analysis.
- Use performance analysis tools such as Xdebug and Blackfire for detailed performance analysis.
Performance optimization and best practices
In practical applications, performance optimization is a continuous process. We need to continuously monitor and adjust our optimization strategies to adapt to changing needs and environments.
- Compare performance differences between different methods: For example, compare the performance differences between Query Builder and Active Record and choose the most suitable method for the current scenario.
- Give examples to illustrate the effect of optimization: through actual data display performance comparison before and after optimization, help the team understand the value of optimization.
- Programming habits and best practices: Keep code readable and maintained, and avoid increasing code complexity caused by over-optimization.
In short, the performance tuning and optimization of Yii 2.0 is a multi-level and multi-angle process. By deeply understanding and flexibly applying various features of Yii 2.0, we can significantly improve the performance of our application and provide a better user experience.
The above is the detailed content of Yii 2.0 Deep Dive: Performance Tuning & Optimization. For more information, please follow other related articles on the PHP Chinese website!
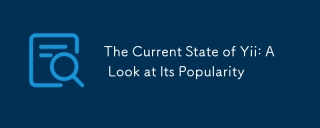
YiiremainspopularbutislessfavoredthanLaravel,withabout14kGitHubstars.ItexcelsinperformanceandActiveRecord,buthasasteeperlearningcurveandasmallerecosystem.It'sidealfordevelopersprioritizingefficiencyoveravastecosystem.
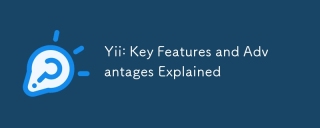
Yii is a high-performance PHP framework that is unique in its componentized architecture, powerful ORM and excellent security. 1. The component-based architecture allows developers to flexibly assemble functions. 2. Powerful ORM simplifies data operation. 3. Built-in multiple security functions to ensure application security.
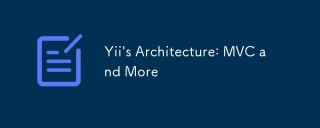
Yii framework adopts an MVC architecture and enhances its flexibility and scalability through components, modules, etc. 1) The MVC mode divides the application logic into model, view and controller. 2) Yii's MVC implementation uses action refinement request processing. 3) Yii supports modular development and improves code organization and management. 4) Use cache and database query optimization to improve performance.
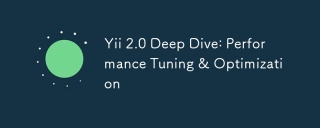
Strategies to improve Yii2.0 application performance include: 1. Database query optimization, using QueryBuilder and ActiveRecord to select specific fields and limit result sets; 2. Caching strategy, rational use of data, query and page cache; 3. Code-level optimization, reducing object creation and using efficient algorithms. Through these methods, the performance of Yii2.0 applications can be significantly improved.
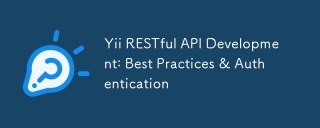
Developing a RESTful API in the Yii framework can be achieved through the following steps: Defining a controller: Use yii\rest\ActiveController to define a resource controller, such as UserController. Configure authentication: Ensure the security of the API by adding HTTPBearer authentication mechanism. Implement paging and sorting: Use yii\data\ActiveDataProvider to handle complex business logic. Error handling: Configure yii\web\ErrorHandler to customize error responses, such as handling when authentication fails. Performance optimization: Use Yii's caching mechanism to optimize frequently accessed resources and improve API performance.
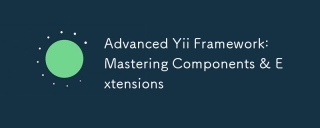
In the Yii framework, components are reusable objects, and extensions are plugins added through Composer. 1. Components are instantiated through configuration files or code, and use dependency injection containers to improve flexibility and testability. 2. Expand the management through Composer to quickly enhance application functions. Using these tools can improve development efficiency and application performance.
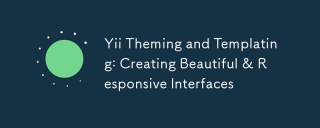
Theming and Tempting of the Yii framework achieve website style and content generation through theme directories and views and layout files: 1. Theming manages website style and layout by setting theme directories, 2. Tempting generates HTML content through views and layout files, 3. Embed complex UI components using the Widget system, 4. Optimize performance and follow best practices to improve user experience and development efficiency.
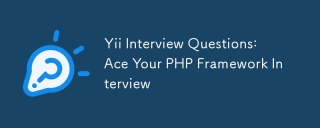
When preparing for an interview with Yii framework, you need to know the following key knowledge points: 1. MVC architecture: Understand the collaborative work of models, views and controllers. 2. ActiveRecord: Master the use of ORM tools and simplify database operations. 3. Widgets and Helpers: Familiar with built-in components and helper functions, and quickly build the user interface. Mastering these core concepts and best practices will help you stand out in the interview.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
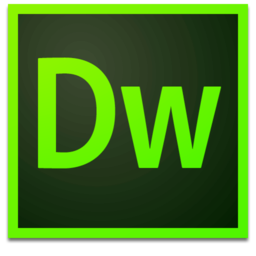
Dreamweaver Mac version
Visual web development tools
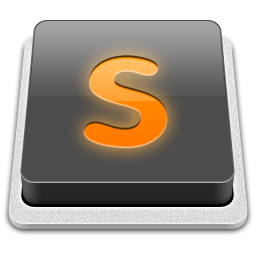
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Linux new version
SublimeText3 Linux latest version
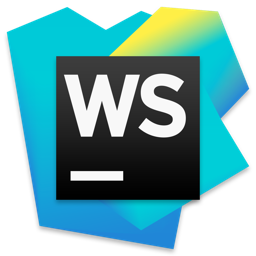
WebStorm Mac version
Useful JavaScript development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.