


Explain different error types in PHP (Notice, Warning, Fatal Error, Parse Error).
There are four main error types in PHP: 1. Notice: the slightest, will not interrupt the program, such as accessing undefined variables; 2. Warning: serious than Notice, will not terminate the program, such as containing files that do not exist; 3. Fatal Error: the most serious, will terminate the program, such as calling a function that does not exist; 4. Parse Error: syntax error, will prevent the program from being executed, such as forgetting to add the end tag.
introduction
In the world of PHP programming, errors are like various signposts we encounter on our journey, guiding us to correct our code and improve the robustness of our programs. Today, we will dive into four main error types in PHP: Notice, Warning, Fatal Error, and Parse Error. Through this article, you will not only understand the definition and role of these errors, but also master how to identify and process them in actual development, thereby improving your programming skills.
Review of basic knowledge
Before we start to dive into it, let's review the error handling mechanism in PHP. PHP provides a flexible error handling system that allows developers to customize error handling functions to catch and handle different types of errors. Understanding these error types is essential for debugging and optimizing code.
Core concept or function analysis
Notice
Definition and Function : Notice is the slightest error type in PHP and usually does not interrupt the execution of the program. They remind developers that there may be potential problems, but will not affect the normal operation of the program. For example, when you try to access an undefined variable, PHP throws an Notice.
Example :
<?php echo $undefinedVariable; // This will trigger an Notice ?>
How it works : Notice is usually triggered by the PHP engine when a potential problem is detected while executing code. They do not cause the program to terminate, but if not processed, it may lead to more serious errors at some point in the future.
Warning
Definition and Effect : Warning is more serious than Notice, but it still does not cause the program to terminate. They indicate that there are errors in the code that can cause problems. For example, when you try to include a file that does not exist, PHP throws a Warning.
Example :
<?php include 'non_existent_file.php'; // This will trigger a Warning ?>
How it works : Warning is usually triggered by the PHP engine when executing code to detect errors that may cause problems. They do not terminate the program immediately, but if not processed, it may cause abnormal program behavior.
Fatal Error
Definition and Function : Fatal Error is one of the most serious error types in PHP and will cause the program to terminate immediately. They indicate that there is an unrecoverable error in the code. For example, when you try to call a function that does not exist, PHP will throw a Fatal Error.
Example :
<?php nonExistentFunction(); // This will trigger a Fatal Error ?>
How it works : Fatal Error is usually triggered by the PHP engine when an error that cannot be recovered is detected while executing code. They will immediately terminate the execution of the program, so special attention and handling is required.
Parse Error
Definition and function : Parse Error is another serious error type in PHP, which will be triggered during the code parsing stage, causing the program to be unable to execute. They indicate a syntax error in the code. For example, when you forget to add an end tag to the PHP code block, PHP will throw a Parse Error.
Example :
<?php echo "Hello, World!"; // Forgot to add?> The end tag will trigger Parse Error
How it works : Parse Error is triggered when a syntax error is detected when the PHP engine parses the code. They block the execution of the program and therefore require special attention during development.
Example of usage
Basic usage
In actual development, it is very important to identify and deal with these error types. Here are some basic usage examples:
Notice :
<?php error_reporting(E_ALL); ini_set('display_errors', 1); $undefinedVariable = null; echo $undefinedVariable; // This will trigger an Notice ?>
Warning :
<?php error_reporting(E_ALL); ini_set('display_errors', 1); include 'non_existent_file.php'; // This will trigger a Warning ?>
Fatal Error :
<?php error_reporting(E_ALL); ini_set('display_errors', 1); nonExistentFunction(); // This will trigger a Fatal Error ?>
Parse Error :
<?php echo "Hello, World!"; // Forgot to add?> The end tag will trigger Parse Error
Advanced Usage
In more complex scenarios, we can use custom error handling functions to capture and handle these errors. For example:
<?php function customErrorHandler($errno, $errstr, $errfile, $errline) { if (!(error_reporting() & $errno)) { // The error code is closed in this script and will not be processed; } switch ($errno) { case E_NOTICE: case E_USER_NOTICE: echo "Notice: [$errno] $errstr - $errfile:$errline\n"; break; case E_WARNING: case E_USER_WARNING: echo "Warning: [$errno] $errstr - $errfile:$errline\n"; break; case E_ERROR: case E_USER_ERROR: echo "Fatal Error: [$errno] $errstr - $errfile:$errline\n"; break; default: echo "Unknown error type: [$errno] $errstr - $errfile:$errline\n"; break; } /* Do not execute the error handler inside PHP*/ return true; } set_error_handler("customErrorHandler"); // Trigger different types of error echo $undefinedVariable; // Notice include 'non_existent_file.php'; // Warning nonExistentFunction(); // Fatal Error ?>
Common Errors and Debugging Tips
In actual development, we may encounter the following common errors:
- Notice : Access undefined variables or array keys. Debugging tips: Use
isset()
orempty()
functions to check whether the variable exists. - Warning : Contains files that do not exist. Debugging tips: Use
file_exists()
function to check whether the file exists. - Fatal Error : Calling a function that does not exist. Debugging tips: Use
function_exists()
function to check whether the function exists. - Parse Error : Syntax error. Debugging tips: Check the code carefully to make sure the syntax is correct.
Performance optimization and best practices
Here are some performance optimization and best practice suggestions when dealing with PHP errors:
- Error Reporting Level : In the development environment, set
error_reporting(E_ALL)
to catch all types of errors. In a production environment, adjust the error reporting level according to requirements to avoid exposure of sensitive information. - Custom error handling : Use custom error handling functions to handle errors more flexibly and provide more detailed error information.
- Logging : Log error information into a log file for subsequent analysis and debugging.
- Code review : Regular code reviews are conducted to ensure code quality and reduce errors.
By deeply understanding and handling different error types in PHP, we can not only improve the robustness of our code, but also improve development efficiency. In actual projects, using this knowledge flexibly will make you a better PHP developer.
The above is the detailed content of Explain different error types in PHP (Notice, Warning, Fatal Error, Parse Error).. For more information, please follow other related articles on the PHP Chinese website!
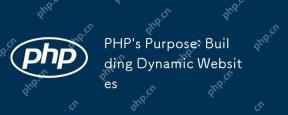
PHP is used to build dynamic websites, and its core functions include: 1. Generate dynamic content and generate web pages in real time by connecting with the database; 2. Process user interaction and form submissions, verify inputs and respond to operations; 3. Manage sessions and user authentication to provide a personalized experience; 4. Optimize performance and follow best practices to improve website efficiency and security.

PHP uses MySQLi and PDO extensions to interact in database operations and server-side logic processing, and processes server-side logic through functions such as session management. 1) Use MySQLi or PDO to connect to the database and execute SQL queries. 2) Handle HTTP requests and user status through session management and other functions. 3) Use transactions to ensure the atomicity of database operations. 4) Prevent SQL injection, use exception handling and closing connections for debugging. 5) Optimize performance through indexing and cache, write highly readable code and perform error handling.
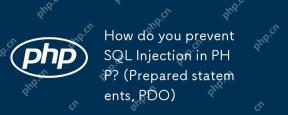
Using preprocessing statements and PDO in PHP can effectively prevent SQL injection attacks. 1) Use PDO to connect to the database and set the error mode. 2) Create preprocessing statements through the prepare method and pass data using placeholders and execute methods. 3) Process query results and ensure the security and performance of the code.
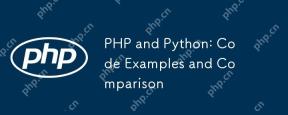
PHP and Python have their own advantages and disadvantages, and the choice depends on project needs and personal preferences. 1.PHP is suitable for rapid development and maintenance of large-scale web applications. 2. Python dominates the field of data science and machine learning.
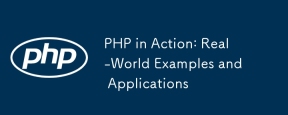
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
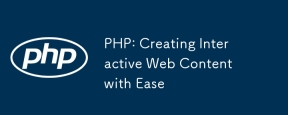
PHP makes it easy to create interactive web content. 1) Dynamically generate content by embedding HTML and display it in real time based on user input or database data. 2) Process form submission and generate dynamic output to ensure that htmlspecialchars is used to prevent XSS. 3) Use MySQL to create a user registration system, and use password_hash and preprocessing statements to enhance security. Mastering these techniques will improve the efficiency of web development.
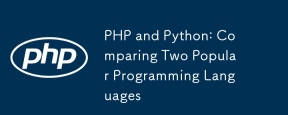
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
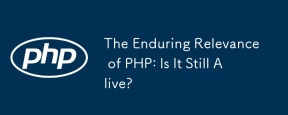
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

Zend Studio 13.0.1
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.