


How to solve the problem of the return value of the C language function being ignored?
The return value of C language function is ignored, which will lead to the failure to obtain the execution result and cover up the error. The solutions include: carefully reading the function document, explicitly using the return value, turning on the compiler warning, and using assertions. Develop good programming habits, pay attention to function return values, and write robust and reliable code.
Is the return value of C function ignored? Don't panic, let's talk about this!
Many novices, even some veterans, will encounter this problem: C language functions work hard to calculate the result, but because the caller ignores the return value, the program runs incorrectly and even crashes. It’s like carefully preparing a full table of Manchu and Han banquets, but the guests were just chatting and didn’t even touch a single bite of the dishes. Isn’t that a pity?
In this article, let’s analyze the root cause of this problem and how to avoid it gracefully. After reading it, you will be able to better understand the mechanism of C language functions and write more robust and reliable code.
Let’s start with the basics: What is the return value of the function?
A function, you can understand it as a "black box". You throw some data (parameters) into it, and it spits out a result (return value) after a series of operations. This return value is the embodiment of the function execution result and is crucial! Functions without return values are usually used to perform some side effects, such as modifying global variables, printing information, etc. But for the most part, we need the function to return a meaningful value.
The consequences of the return value being ignored
Ignoring the return value, the most direct consequence is that you cannot get the execution result of the function. It's like you ordered a takeaway and the delivery guy delivered it, but you didn't open the door, and the takeaway would get cold and you didn't eat it. What's more serious is that the return value of some functions is not only the result, but may also contain error codes or status information. Ignoring this information, you may not know what errors occurred during the function operation, which leads to difficult bugs in the program.
How to avoid ignoring the return value?
This is actually very simple, but it requires good programming habits.
- Read function documents carefully: Each function should have clear documentation instructions, including its functions, parameters, return values and possible error codes. Developing the habit of reading documents can help you understand the purpose of a function and how to use its return value correctly.
- Use the return value explicitly: Don't let the return value disappear silently. Even if you don't need to use the return value for the time being, you should explicitly assign it to a variable, or at least print it out with a statement, which can help you discover potential problems.
<code class="c">#include <stdio.h> #include <stdlib.h> int my_function(int a, int b) { if (b == 0) { fprintf(stderr, "Error: Division by zero!\n"); //报错信息输出到标准错误流return -1; // 返回错误码} return a / b; } int main() { int result = my_function(10, 2); //正确使用返回值if (result == -1) { fprintf(stderr, "Function execution failed!\n"); } else { printf("Result: %d\n", result); } my_function(10, 0); //忽略返回值,程序会输出错误信息,但不会被main函数捕获处理// 更优雅的处理方式,使用一个变量接收返回值int anotherResult = my_function(10,0); if (anotherResult == -1){ //进行错误处理} return 0; }</stdlib.h></stdio.h></code>
In this example, the my_function
function returns -1 when the divisor is 0, indicating an error. main
function should check this return value and take corresponding measures based on the return value. Ignore the return value. Although the program can run, the result is incorrect and it is easy to cover up the error.
- Compiler Warning: Many compilers provide warning options to detect situations where the return value is ignored. Turn on these warning options to help you spot potential problems during the compilation phase. For example, GCC's
-Wall
option is a good choice.
Some more advanced tips
For complex programs, consider using assert to check the return value of the function. Assertions can help you detect problems early in the development phase and avoid unexpected situations at runtime.
<code class="c">#include <assert.h> // ... (前面的代码) ... int main() { int result = my_function(10, 2); assert(result != -1); // 断言返回值不等于错误码printf("Result: %d\n", result); return 0; }</assert.h></code>
Remember, writing high-quality C code is not only to make the program run, but also to make the program robust, reliable and easy to maintain. Paying attention to function return values is an important part of writing high-quality C code. Don’t let your “Manhan Banquet” be done in vain!
The above is the detailed content of How to solve the problem of the return value of the C language function being ignored?. For more information, please follow other related articles on the PHP Chinese website!
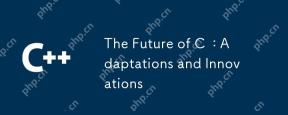
The future of C will focus on parallel computing, security, modularization and AI/machine learning: 1) Parallel computing will be enhanced through features such as coroutines; 2) Security will be improved through stricter type checking and memory management mechanisms; 3) Modulation will simplify code organization and compilation; 4) AI and machine learning will prompt C to adapt to new needs, such as numerical computing and GPU programming support.
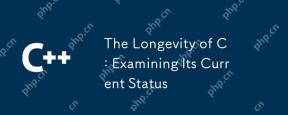
C is still important in modern programming because of its efficient, flexible and powerful nature. 1)C supports object-oriented programming, suitable for system programming, game development and embedded systems. 2) Polymorphism is the highlight of C, allowing the call to derived class methods through base class pointers or references to enhance the flexibility and scalability of the code.
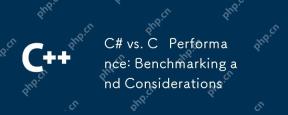
The performance differences between C# and C are mainly reflected in execution speed and resource management: 1) C usually performs better in numerical calculations and string operations because it is closer to hardware and has no additional overhead such as garbage collection; 2) C# is more concise in multi-threaded programming, but its performance is slightly inferior to C; 3) Which language to choose should be determined based on project requirements and team technology stack.
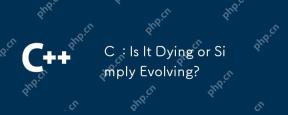
C isnotdying;it'sevolving.1)C remainsrelevantduetoitsversatilityandefficiencyinperformance-criticalapplications.2)Thelanguageiscontinuouslyupdated,withC 20introducingfeatureslikemodulesandcoroutinestoimproveusabilityandperformance.3)Despitechallen
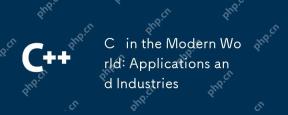
C is widely used and important in the modern world. 1) In game development, C is widely used for its high performance and polymorphism, such as UnrealEngine and Unity. 2) In financial trading systems, C's low latency and high throughput make it the first choice, suitable for high-frequency trading and real-time data analysis.
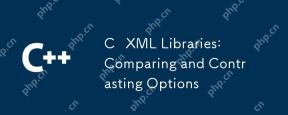
There are four commonly used XML libraries in C: TinyXML-2, PugiXML, Xerces-C, and RapidXML. 1.TinyXML-2 is suitable for environments with limited resources, lightweight but limited functions. 2. PugiXML is fast and supports XPath query, suitable for complex XML structures. 3.Xerces-C is powerful, supports DOM and SAX resolution, and is suitable for complex processing. 4. RapidXML focuses on performance and parses extremely fast, but does not support XPath queries.
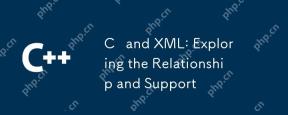
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
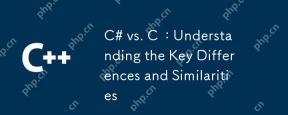
The main differences between C# and C are syntax, performance and application scenarios. 1) The C# syntax is more concise, supports garbage collection, and is suitable for .NET framework development. 2) C has higher performance and requires manual memory management, which is often used in system programming and game development.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
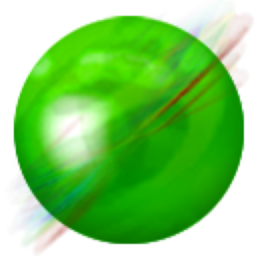
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
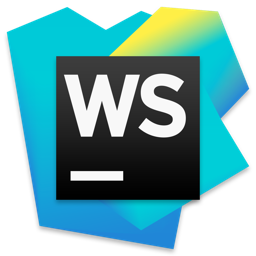
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 Chinese version
Chinese version, very easy to use
