What does the return value of 56 or 65 mean by C language function?
When a C function returns 56 or 65, it indicates a specific event. These numerical meanings are defined by the function developer and may indicate success, file not found, or read errors. Replace these "magic numbers" with enumerations or macro definitions can improve readability and maintainability, such as: READ_SUCCESS, FILE_NOT_FOUND, and READ_ERROR.
C function returns value 56 or 65: those hidden signals
Many beginners may be confused when they see that the C function returns 56 or 65. This is not a magic number, but a "signal light" carefully designed by programmers, conveying information about the execution results of the function. They themselves have no fixed meaning, and they all depend on how the function developers define it. In this article, we will explore the story behind these numbers and how to avoid falling into the trap of "magic numbers".
Background: The conventional "code"
C language does not have rich exception handling mechanisms like some high-level languages. In many cases, the return value of a function is the only feedback channel. Therefore, programmers will agree on some specific values to represent different situations of function execution: success, failure, certain specific error, etc. 56 and 65 are two examples of this conventional "code". They may represent a certain state code or they may be just numbers that programmers choose at will to distinguish different results. The key is that you have to consult the documentation or code comments of the function to understand the true meaning of these numbers.
Peeking into the inner: the perspective of functions
Let's assume a simple example: a function is responsible for reading data from a file. If the read is successful, it may return 0; if the file does not exist, it may return 56; if other errors occur during the read process, it may return 65. It depends entirely on the designer of the function.
<code class="c">#include <stdio.h> int readFile(const char* filename) { FILE *fp = fopen(filename, "r"); if (fp == NULL) { return 56; // File not found } // ... 读取文件内容... if (/* 读取过程中发生错误*/) { fclose(fp); return 65; // Read error } fclose(fp); return 0; // Success } int main() { int result = readFile("mydata.txt"); if (result == 56) { printf("File not found!\n"); } else if (result == 65) { printf("Read error!\n"); } else { printf("File read successfully!\n"); } return 0; }</stdio.h></code>
In this code, 56 and 65 represent two different error cases. Note that the readFile
function does not throw an exception, but instead informs the caller of the execution result of the function by returning the value. This is the typical style of C language, which is simple and direct, but also requires programmers to be more careful.
Traps and warnings: Avoid "magic numbers"
Using numbers like 56 and 65 as status codes directly can easily make the code difficult to understand and maintain. Imagine that in a few months, you or someone else needs to modify this function, and it will be very troublesome if you forget the meaning of these numbers.
A better approach is to use enums or macro definitions instead of these "magic numbers".
<code class="c">#include <stdio.h> typedef enum { READ_SUCCESS = 0, FILE_NOT_FOUND = 56, READ_ERROR = 65 } ReadResult; ReadResult readFile(const char* filename) { // ... (函数体不变) ... } int main() { ReadResult result = readFile("mydata.txt"); if (result == FILE_NOT_FOUND) { printf("File not found!\n"); } else if (result == READ_ERROR) { printf("Read error!\n"); } else { printf("File read successfully!\n"); } return 0; }</stdio.h></code>
In this way, the readability and maintainability of the code will be greatly improved. Even if the meaning of 56 and 65 is forgotten, FILE_NOT_FOUND
and READ_ERROR
can clearly express their meanings. This is a good programming habit and is worth learning and reference by all C language programmers.
Experience: Code readability is better than anything else
Remember, the code is written for people to see, and the second is executed for machines. Clear, concise and easy to understand code can not only improve development efficiency, but also reduce maintenance costs and avoid unnecessary errors. Therefore, when writing C language code, be sure to pay attention to the readability of the code, try to avoid using "magic numbers", and use enumerations or macro definitions to improve the comprehensibility of the code. This not only prevents you from falling into a pit, but also makes you a better programmer.
The above is the detailed content of What does the return value of 56 or 65 mean by C language function?. For more information, please follow other related articles on the PHP Chinese website!
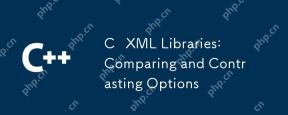
There are four commonly used XML libraries in C: TinyXML-2, PugiXML, Xerces-C, and RapidXML. 1.TinyXML-2 is suitable for environments with limited resources, lightweight but limited functions. 2. PugiXML is fast and supports XPath query, suitable for complex XML structures. 3.Xerces-C is powerful, supports DOM and SAX resolution, and is suitable for complex processing. 4. RapidXML focuses on performance and parses extremely fast, but does not support XPath queries.
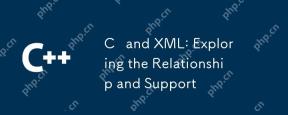
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
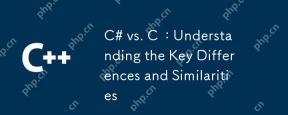
The main differences between C# and C are syntax, performance and application scenarios. 1) The C# syntax is more concise, supports garbage collection, and is suitable for .NET framework development. 2) C has higher performance and requires manual memory management, which is often used in system programming and game development.
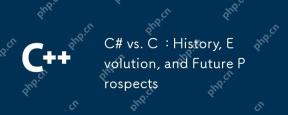
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
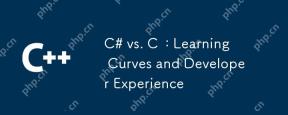
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
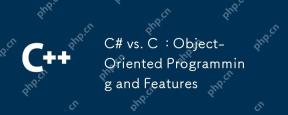
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
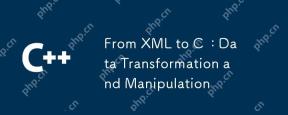
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
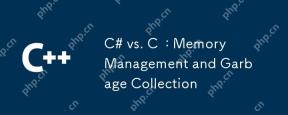
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Zend Studio 13.0.1
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.