The C language function definition includes the specified return value type, function name, parameter list and function body. To call a function, just add the parameter with the function name. Parameter passing is passed by value by default, except pointer parameters. Function prototype declares function information to improve readability. Recursive function calls itself and requires a termination condition. Performance optimization can reduce function call overhead using inline functions or macro definitions.
C language functions: definitions and calls, details that you may not know
Many beginners think that the definition and call of C functions are simple, aren’t it just int func(int a){...}
and func(5);
;? That's right, it's the case, but the devil is hidden in the details. In this article, let’s dig deeper and take a look at the unknown "secrets" of C language functions. After reading it, you will not only be able to master function definitions and calls, but also to a higher level in performance optimization and code elegance.
Let’s start with the most basic ones. A C function is essentially a reusable block of code. It receives input (parameter), processes it, and returns the result (return value). To define a function, you need to specify its return value type, function name, parameter list, and function body. For example:
<code class="c">int add(int a, int b) { return ab; }</code>
This code defines a function called add
that takes two integers a
and b
as input and returns their sum. Note that int
means that the return value type is an integer. If no return value is used, use void
.
Function calls are even simpler. Just use the function name and parameters, just like using a built-in function:
<code class="c">int sum = add(5, 3); // 调用add函数,并将结果赋值给sum</code>
It looks simple, right? But things are much more than that.
The secret of parameter passing
The method of parameter passing determines whether the modification of parameters inside the function will affect variables outside the function. C language uses value passing, which means that the function receives a copy of the parameters, not the parameters themselves. Therefore, modifying the value of the parameter inside the function will not change the value of the external variable of the function.
<code class="c">void modify(int x) { x = 10; // 修改局部变量x } int main() { int y = 5; modify(y); printf("%d\n", y); // 输出5,y的值没有改变return 0; }</code>
However, pointer parameters are an exception. The pointer passes a memory address, so the value of the original variable can be modified through the pointer inside the function.
<code class="c">void modify_ptr(int *x) { *x = 10; // 修改x指向的内存中的值} int main() { int y = 5; modify_ptr(&y); printf("%d\n", y); // 输出10,y的值被改变了return 0; }</code>
Understanding pointer parameters is crucial to mastering C language and is also a place where many beginners are prone to make mistakes.
The importance of function prototypes
Before calling a function, the compiler needs to know the function's return value type and parameter list. This is what the function prototype does. A good programming habit is to declare function prototypes in header files, which can improve the readability and maintainability of the code and avoid many potential errors.
<code class="c">// 在头文件中声明函数原型int add(int a, int b); // 在源文件中定义函数int add(int a, int b) { return ab; }</code>
Recursive functions
A recursive function refers to a function that calls itself within a function. Recursive functions are elegant and concise, but improper use can easily cause stack overflow. Make sure your recursive function has the correct terminating conditions and avoid infinite recursion.
<code class="c">int factorial(int n) { if (n == 0) { return 1; // 终止条件} else { return n * factorial(n - 1); } }</code>
Performance optimization
Function calls will have some overhead, especially small functions that are frequently called. For programs with high performance requirements, you can consider using inline functions or macro definitions to reduce the overhead of function calls. But remember that inline functions and macro definitions also have some disadvantages, such as code bloating and potential debugging difficulties. Choosing the right optimization method requires weighing the pros and cons based on actual conditions.
In short, the definition and call of C functions seem simple, but they contain rich details and techniques. Only by mastering these details can you write more efficient and elegant C language code. Remember, programming is a practical art. Only by practicing more and thinking more can you become a real master of programming.
The above is the detailed content of A brief list of definitions and calls of c language functions. For more information, please follow other related articles on the PHP Chinese website!
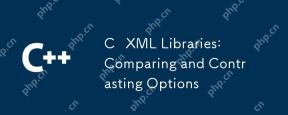
There are four commonly used XML libraries in C: TinyXML-2, PugiXML, Xerces-C, and RapidXML. 1.TinyXML-2 is suitable for environments with limited resources, lightweight but limited functions. 2. PugiXML is fast and supports XPath query, suitable for complex XML structures. 3.Xerces-C is powerful, supports DOM and SAX resolution, and is suitable for complex processing. 4. RapidXML focuses on performance and parses extremely fast, but does not support XPath queries.
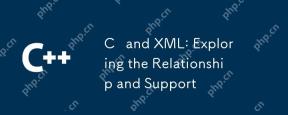
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
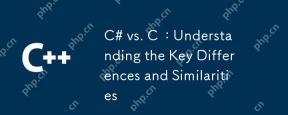
The main differences between C# and C are syntax, performance and application scenarios. 1) The C# syntax is more concise, supports garbage collection, and is suitable for .NET framework development. 2) C has higher performance and requires manual memory management, which is often used in system programming and game development.
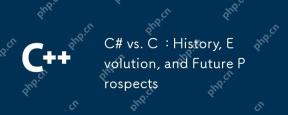
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
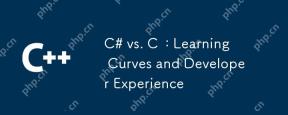
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
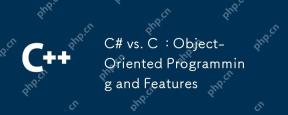
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
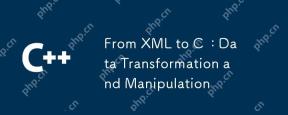
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
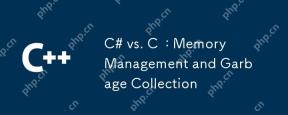
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor
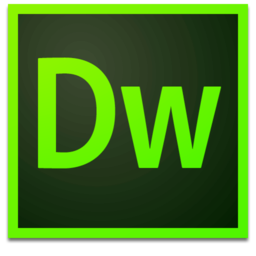
Dreamweaver Mac version
Visual web development tools
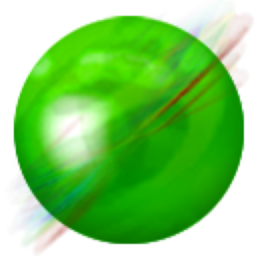
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software