


Explain covariance and contravariance in PHP method parameters and return types.
PHP supports covariance and inversion since version 7.4. 1. Covariance allows subclass methods to return more specific types, such as the parent class returns Animal, and the subclass can return Dog. 2. Inverter allows subclass methods to accept wider parameter types, such as the parent class accepts Dog, and the subclass can accept Animal.
introduction
Today we are going to explore covariance and inverse in method parameters and return types in PHP. This may sound a bit abstract, but trust me, understanding them will greatly improve your ability in object-oriented programming. Through this article, you will not only master these concepts, but also understand their applications and potential pitfalls in actual programming.
Review of basic knowledge
In PHP, covariance and inversion are important concepts in type systems. They affect how we override the methods of the parent class in a subclass. Simply put, covariance means that the return type of a subclass method can be a child of the parent method return type, while inversion allows the parameter type of the subclass method to be the supertype of the parent method parameter type.
For example, if you are familiar with inheritance and polymorphism in object-oriented programming, it will be easier to understand these concepts. PHP supports covariance and inversion since version 7.4, which greatly enhances language flexibility and type safety.
Core concept or function analysis
Definition and function of covariance and inversion
Covariance allows the return type of a child class method to be more specific than the return type of a parent class method. For example, if the parent class method returns an Animal
type, the subclass can return a Dog
type because Dog
is a subclass of Animal
.
class Animal {} class Dog extends Animal {} class ParentClass { public function getAnimal(): Animal { return new Animal(); } } class ChildClass extends ParentClass { public function getAnimal(): Dog { return new Dog(); } }
Contravariance allows the parameter types of subclass methods to be broader than those of parent methods. For example, if the parent class method accepts Dog
type, the subclass can accept Animal
type.
class Animal {} class Dog extends Animal {} class ParentClass { public function feedDog(Dog $dog) { // Feed the dog} } class ChildClass extends ParentClass { public function feedDog(Animal $animal) { // Feed animals} }
How it works
The working principle of covariance and inversion is based on the hierarchy of type systems. Covariance utilizes subtype relationships, allowing for more specific return types, because subtypes can replace parent types. Inverting utilizes supertype relationships, allowing for broader parameter types, because the parent type can accept objects of child types.
In PHP, these features are implemented through type prompts. Covariance and inversion improve code flexibility while also increasing type safety. They allow developers to rewrite parent class methods more freely in subclasses without having to strictly follow the parent class's method signature.
Example of usage
Basic usage
Let's take a look at a simple example of covariation. In this example, the parent class returns Animal
, and the child class returns Dog
:
class Animal { public function sound() { return "Animal sound"; } } class Dog extends Animal { public function sound() { return "Woof"; } } class ParentClass { public function getAnimal(): Animal { return new Animal(); } } class ChildClass extends ParentClass { public function getAnimal(): Dog { return new Dog(); } } $child = new ChildClass(); $animal = $child->getAnimal(); echo $animal->sound(); // Output "Woof"
This example shows how to return more specific types in a subclass while keeping them type safe.
Advanced Usage
Now let's look at an example of inverse change. In this example, the parent class accepts Dog
, while the child class accepts Animal
:
class Animal { public function eat() { return "Eating"; } } class Dog extends Animal { public function eat() { return "Eating dog food"; } } class ParentClass { public function feedDog(Dog $dog) { echo $dog->eat(); // Output "Eating dog food" } } class ChildClass extends ParentClass { public function feedDog(Animal $animal) { echo $animal->eat(); // Output "Eating" } } $child = new ChildClass(); $child->feedDog(new Dog()); // Output "Eating dog food" $child->feedDog(new Animal()); // Output "Eating"
This example shows how to accept a wider parameter type in a subclass while maintaining method flexibility.
Common Errors and Debugging Tips
Common errors when using covariance and inversion include:
- Type mismatch : Make sure that the return type of the child class method is a child of the parent class method return type, or the parameter type is a supertype of the parent class method parameter type.
- Type prompt error : Make sure to use the type prompt correctly, otherwise it will cause type errors.
Debugging skills include:
- Type checking with IDE : Modern IDEs usually support type checking to help you discover types mismatch problems.
- Test-driven development : Write test cases to verify the type safety of methods.
Performance optimization and best practices
In practical applications, covariance and inversion can help optimize the readability and maintenance of the code. Here are some best practices:
- Maintain type consistency : Ensure that the type of the subclass method remains consistent with the type of the parent class method and avoid type errors.
- Use type tips : Use type tips whenever possible to improve the type safety of your code.
- Performance Considerations : While covariance and inversion do not directly affect performance, they can help you write clearer and easier to maintain code that will indirectly improve performance.
By understanding and applying covariance and inversion, you can write more flexible and safer code in PHP. I hope this article can help you better grasp these concepts and flexibly apply them in actual projects.
The above is the detailed content of Explain covariance and contravariance in PHP method parameters and return types.. For more information, please follow other related articles on the PHP Chinese website!
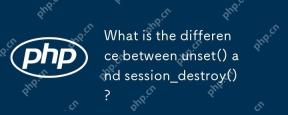
Thedifferencebetweenunset()andsession_destroy()isthatunset()clearsspecificsessionvariableswhilekeepingthesessionactive,whereassession_destroy()terminatestheentiresession.1)Useunset()toremovespecificsessionvariableswithoutaffectingthesession'soveralls
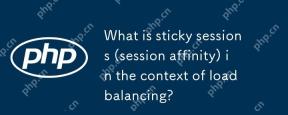
Stickysessionsensureuserrequestsareroutedtothesameserverforsessiondataconsistency.1)SessionIdentificationassignsuserstoserversusingcookiesorURLmodifications.2)ConsistentRoutingdirectssubsequentrequeststothesameserver.3)LoadBalancingdistributesnewuser
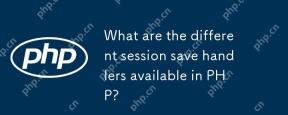
PHPoffersvarioussessionsavehandlers:1)Files:Default,simplebutmaybottleneckonhigh-trafficsites.2)Memcached:High-performance,idealforspeed-criticalapplications.3)Redis:SimilartoMemcached,withaddedpersistence.4)Databases:Offerscontrol,usefulforintegrati
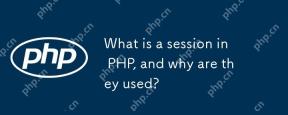
Session in PHP is a mechanism for saving user data on the server side to maintain state between multiple requests. Specifically, 1) the session is started by the session_start() function, and data is stored and read through the $_SESSION super global array; 2) the session data is stored in the server's temporary files by default, but can be optimized through database or memory storage; 3) the session can be used to realize user login status tracking and shopping cart management functions; 4) Pay attention to the secure transmission and performance optimization of the session to ensure the security and efficiency of the application.
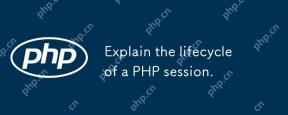
PHPsessionsstartwithsession_start(),whichgeneratesauniqueIDandcreatesaserverfile;theypersistacrossrequestsandcanbemanuallyendedwithsession_destroy().1)Sessionsbeginwhensession_start()iscalled,creatingauniqueIDandserverfile.2)Theycontinueasdataisloade
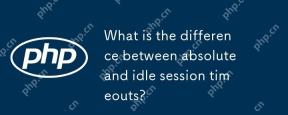
Absolute session timeout starts at the time of session creation, while an idle session timeout starts at the time of user's no operation. Absolute session timeout is suitable for scenarios where strict control of the session life cycle is required, such as financial applications; idle session timeout is suitable for applications that want users to keep their session active for a long time, such as social media.
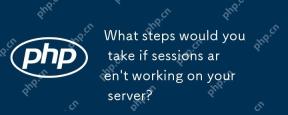
The server session failure can be solved through the following steps: 1. Check the server configuration to ensure that the session is set correctly. 2. Verify client cookies, confirm that the browser supports it and send it correctly. 3. Check session storage services, such as Redis, to ensure that they are running normally. 4. Review the application code to ensure the correct session logic. Through these steps, conversation problems can be effectively diagnosed and repaired and user experience can be improved.
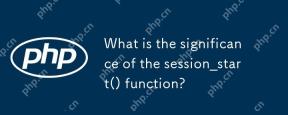
session_start()iscrucialinPHPformanagingusersessions.1)Itinitiatesanewsessionifnoneexists,2)resumesanexistingsession,and3)setsasessioncookieforcontinuityacrossrequests,enablingapplicationslikeuserauthenticationandpersonalizedcontent.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Chinese version
Chinese version, very easy to use
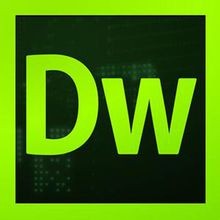
Dreamweaver CS6
Visual web development tools

Atom editor mac version download
The most popular open source editor
