


* Go language calls DLL to return char type data: a safe and efficient memory management policy**
Directly processing char*
type data returned by DLLs in Go language, which can easily cause memory leakage and concurrency security issues. This article will explore in-depth how to solve these problems safely and effectively.
Problem analysis:
Suppose a DLL library provides a function named echo
, and its C language implementation is as follows:
char *echo() { return "123123"; }
If the Go code directly calls the function using syscall
package and processes the return value, it will face the following challenges:
- Memory Leak: The string memory returned by the DLL is not released on the Go side, resulting in a memory leak. The string returned by the
echo
function is stored in the memory allocated inside the DLL, and cannot be released by the Go program after use. - Concurrency security: multiple goroutines call
echo
functions simultaneously may cause race conditions, raise data errors or program crashes. -
unsafe.Pointer
risk: Direct operation ofunsafe.Pointer
poses potential memory security risks and is prone to errors. - Lack of error handling: The code lacks a robust error handling mechanism, which reduces reliability.
Solution: Advantages of Cgo
It is more risky to use syscall
package to deal with char*
directly. It is recommended to use cgo
, which allows Go code to interact seamlessly with C code. Through cgo
, we can write a C language wrapper function that is responsible for getting data from DLLs and freeing memory on the Go side. cgo
provides functions such as C.CString
, C.GoString
, C.free
, etc. to simplify Go and C type conversion and memory management.
Advantages of using cgo
:
- Avoid
unsafe.Pointer
: Reduce memory security risks and improve code readability and maintainability. - Fine memory management: Ensure that the memory allocated by the DLL is released correctly to avoid memory leakage.
- Enhance concurrency security: perform necessary synchronization processing (such as mutex locks) on the Go side to ensure data consistency and program stability.
Best Practice: Write wrapper functions using Cgo
Here is an example of using cgo
to handle DLL returning char*
:
/* #include<stdlib.h> #include "my_dll.h" // Assume that the header file of the DLL is char* wrapEcho() { char* result = echo(); // Call the DLL function return result; } void freeString(char* str) { free(str); // Free memory} */ import "C" import ( "fmt" "unsafe" "sync" ) var mu sync.Mutex // for concurrent control func Echo() (string, error) { mu.Lock() defer mu.Unlock() cStr := C.wrapEcho() defer C.free(unsafe.Pointer(cStr)) // Free memory goStr := C.GoString(cStr) return goStr, nil } func main() { str, err := Echo() if err != nil { fmt.Println("Error:", err) } else { fmt.Println("Result:", str) } }</stdlib.h>
In this example, the wrapEcho
function is a wrapper function in C language, which is responsible for calling the echo
function of the DLL and returning the result. freeString
function is responsible for freeing memory. The Go code uses C.free
to free memory and adds the mutex sync.Mutex
to ensure concurrency security. Remember to handle errors correctly and adjust the synchronization mechanism according to actual conditions. Read the cgo
documentation carefully and it is crucial to understand the memory management differences between Go and C.
Through cgo
, we can process char*
type data returned by DLL more securely and efficiently, avoid memory leaks and concurrency security issues, and significantly improve the reliability and stability of our code.
The above is the detailed content of How to avoid memory leaks and concurrency security issues when calling DLL to return char* type data?. For more information, please follow other related articles on the PHP Chinese website!
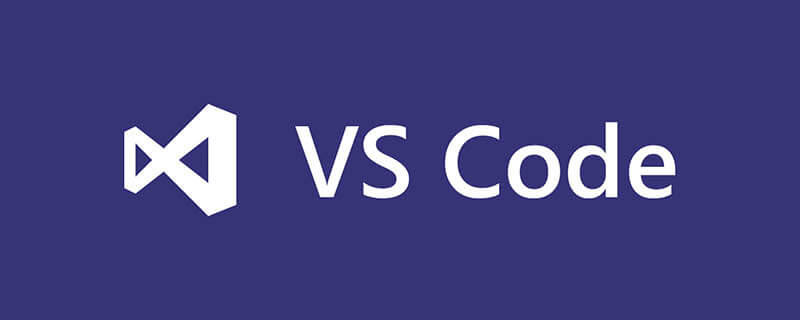
VScode中怎么配置C语言环境?下面本篇文章给大家介绍一下VScode配置C语言环境的方法(超详细),希望对大家有所帮助!
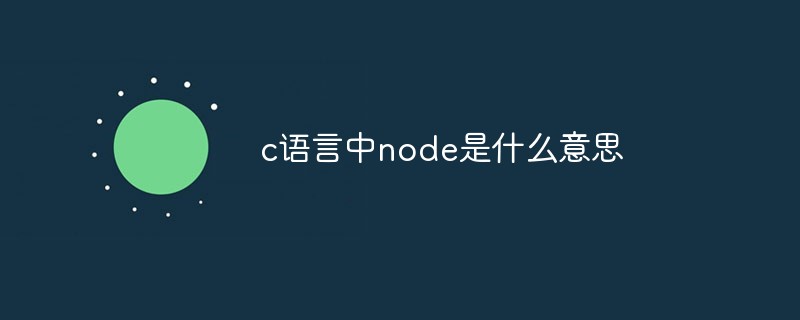
在C语言中,node是用于定义链表结点的名称,通常在数据结构中用作结点的类型名,语法为“struct Node{...};”;结构和类在定义出名称以后,直接用该名称就可以定义对象,C语言中还存在“Node * a”和“Node* &a”。

c语言将数字转换成字符串的方法:1、ascii码操作,在原数字的基础上加“0x30”,语法“数字+0x30”,会存储数字对应的字符ascii码;2、使用itoa(),可以把整型数转换成字符串,语法“itoa(number1,string,数字);”;3、使用sprintf(),可以能够根据指定的需求,格式化内容,存储至指针指向的字符串。
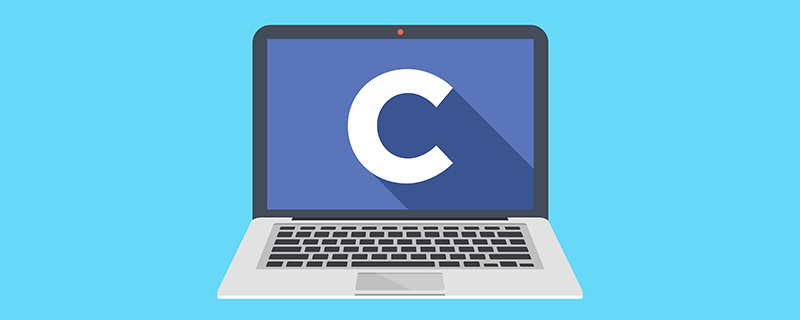
在c语言中,没有开根号运算符,开根号使用的是内置函数“sqrt()”,使用语法“sqrt(数值x)”;例如“sqrt(4)”,就是对4进行平方根运算,结果为2。sqrt()是c语言内置的开根号运算函数,其运算结果是函数变量的算术平方根;该函数既不能运算负数值,也不能输出虚数结果。

C语言数组初始化的三种方式:1、在定义时直接赋值,语法“数据类型 arrayName[index] = {值};”;2、利用for循环初始化,语法“for (int i=0;i<3;i++) {arr[i] = i;}”;3、使用memset()函数初始化,语法“memset(arr, 0, sizeof(int) * 3)”。
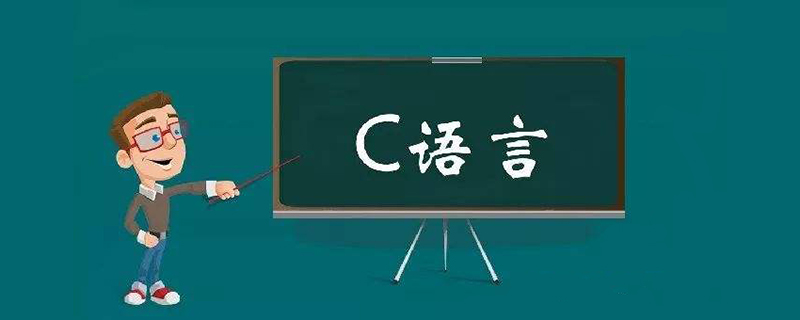
c语言合法标识符的要求是:1、标识符只能由字母(A~Z, a~z)、数字(0~9)和下划线(_)组成;2、第一个字符必须是字母或下划线,不能是数字;3、标识符中的大小写字母是有区别的,代表不同含义;4、标识符不能是关键字。
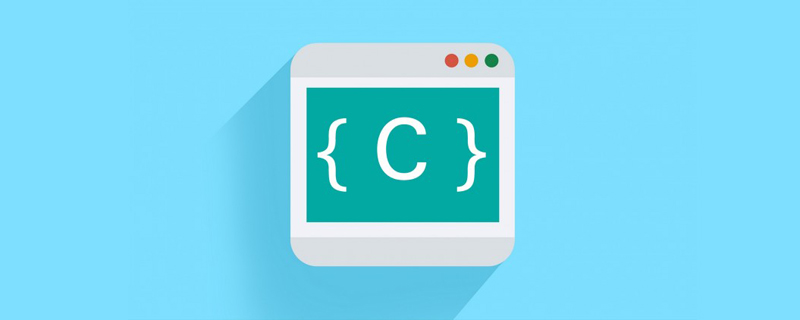
c语言编译后生成“.OBJ”的二进制文件(目标文件)。在C语言中,源程序(.c文件)经过编译程序编译之后,会生成一个后缀为“.OBJ”的二进制文件(称为目标文件);最后还要由称为“连接程序”(Link)的软件,把此“.OBJ”文件与c语言提供的各种库函数连接在一起,生成一个后缀“.EXE”的可执行文件。
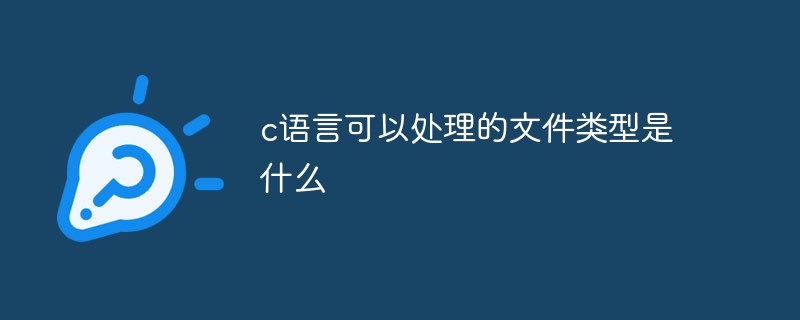
c语言可以处理的文件类型是:文本文件和二进制文件。C语言所能够处理文件是按照存放形式分为文本文件和二进制文件:1、文本文件存储的是一个ASCII码,文件的内容可以直接进行输入输出;2、二进制文件直接将字符存储,不能将二进制文件的内容直接输出到屏幕上。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
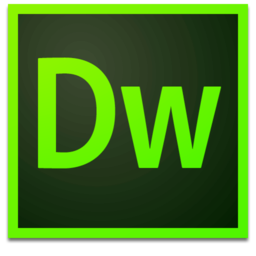
Dreamweaver Mac version
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),